Plasma
#include <Plasma/Applet>
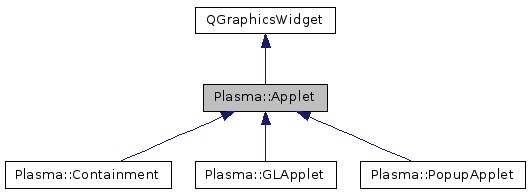
Public Types | |
enum | { Type = Plasma::AppletType } |
enum | BackgroundHint { NoBackground = 0, StandardBackground = 1, TranslucentBackground = 2, DefaultBackground = StandardBackground } |
typedef QHash< QString, Applet * > | Dict |
typedef QList< Applet * > | List |
Public Slots | |
virtual void | configChanged () |
virtual void | destroy () |
void | flushPendingConstraintsEvents () |
virtual void | init () |
bool | isPublished () const |
bool | isUserConfiguring () const |
void | lower () |
void | publish (Plasma::AnnouncementMethods methods, const QString &resourceName) |
void | raise () |
void | runAssociatedApplication () |
void | setBusy (bool busy) |
void | setImmutability (const ImmutabilityType immutable) |
void | setStatus (const ItemStatus stat) |
virtual void | showConfigurationInterface () |
void | showConfigurationInterface (QWidget *widget) |
QVariantList | startupArguments () const |
ItemStatus | status () const |
void | unpublish () |
Signals | |
void | activate () |
void | appletDestroyed (Plasma::Applet *applet) |
void | appletTransformedByUser () |
void | appletTransformedItself () |
void | configNeedsSaving () |
void | extenderItemRestored (Plasma::ExtenderItem *item) |
void | geometryChanged () |
void | immutabilityChanged (Plasma::ImmutabilityType immutable) |
void | messageButtonPressed (const Plasma::MessageButton button) |
void | newStatus (Plasma::ItemStatus status) |
void | releaseVisualFocus () |
void | sizeHintChanged (Qt::SizeHint which) |
Public Member Functions | |
Applet (QGraphicsItem *parent=0, const QString &serviceId=QString(), uint appletId=0) | |
Applet (const KPluginInfo &info, QGraphicsItem *parent=0, uint appletId=0) | |
Applet (QGraphicsItem *parent, const QString &serviceId, uint appletId, const QVariantList &args) | |
~Applet () | |
Q_INVOKABLE QAction * | action (QString name) const |
void | addAction (QString name, QAction *action) |
virtual void | addAssociatedWidget (QWidget *widget) |
Plasma::AspectRatioMode | aspectRatioMode () const |
QString | associatedApplication () const |
KUrl::List | associatedApplicationUrls () const |
BackgroundHints | backgroundHints () const |
QString | category () const |
KConfigGroup | config () const |
KConfigGroup | config (const QString &group) const |
ConfigLoader * | configScheme () const |
bool | configurationRequired () const |
Containment * | containment () const |
Context * | context () const |
virtual QList< QAction * > | contextualActions () |
virtual void | createConfigurationInterface (KConfigDialog *parent) |
QStringList | customCategories () |
Q_INVOKABLE DataEngine * | dataEngine (const QString &name) const |
bool | destroyed () const |
QFont | font () const |
virtual FormFactor | formFactor () const |
KConfigGroup | globalConfig () const |
KShortcut | globalShortcut () const |
bool | hasAuthorization (const QString &constraint) const |
bool | hasConfigurationInterface () const |
bool | hasFailedToLaunch () const |
bool | hasValidAssociatedApplication () const |
QString | icon () const |
uint | id () const |
ImmutabilityType | immutability () const |
virtual void | initExtenderItem (ExtenderItem *item) |
bool | isBusy () const |
bool | isContainment () const |
virtual bool | isPopupShowing () const |
virtual Location | location () const |
QRectF | mapFromView (const QGraphicsView *view, const QRect &rect) const |
QRect | mapToView (const QGraphicsView *view, const QRectF &rect) const |
QString | name () const |
const Package * | package () const |
virtual void | paintInterface (QPainter *painter, const QStyleOptionGraphicsItem *option, const QRect &contentsRect) |
void | paintWindowFrame (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
QString | pluginName () const |
QPoint | popupPosition (const QSize &s) const |
QPoint | popupPosition (const QSize &s, Qt::AlignmentFlag alignment) const |
virtual void | removeAssociatedWidget (QWidget *widget) |
virtual void | restore (KConfigGroup &group) |
virtual void | save (KConfigGroup &group) const |
QRect | screenRect () const |
void | setAspectRatioMode (Plasma::AspectRatioMode) |
void | setAssociatedApplication (const QString &string) |
void | setAssociatedApplicationUrls (const KUrl::List &urls) |
void | setBackgroundHints (const BackgroundHints hints) |
void | setCustomCategories (const QStringList &categories) |
void | setGlobalShortcut (const KShortcut &shortcut) |
bool | shouldConserveResources () const |
int | type () const |
void | updateConstraints (Plasma::Constraints constraints=Plasma::AllConstraints) |
QGraphicsView * | view () const |
Static Public Member Functions | |
static QString | category (const KPluginInfo &applet) |
static QString | category (const QString &appletName) |
static KPluginInfo::List | listAppletInfo (const QString &category=QString(), const QString &parentApp=QString()) |
static KPluginInfo::List | listAppletInfoForMimetype (const QString &mimetype) |
static KPluginInfo::List | listAppletInfoForUrl (const QUrl &url) |
static QStringList | listCategories (const QString &parentApp=QString(), bool visibleOnly=true) |
static Applet * | load (const QString &name, uint appletId=0, const QVariantList &args=QVariantList()) |
static Applet * | load (const KPluginInfo &info, uint appletId=0, const QVariantList &args=QVariantList()) |
static Applet * | loadPlasmoid (const QString &path, uint appletId=0, const QVariantList &args=QVariantList()) |
static PackageStructure::Ptr | packageStructure () |
Protected Member Functions | |
Applet (QObject *parent, const QVariantList &args) | |
virtual void | constraintsEvent (Plasma::Constraints constraints) |
bool | eventFilter (QObject *o, QEvent *e) |
Extender * | extender () const |
void | focusInEvent (QFocusEvent *event) |
void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
bool | isRegisteredAsDragHandle (QGraphicsItem *item) |
QVariant | itemChange (GraphicsItemChange change, const QVariant &value) |
void | mouseMoveEvent (QGraphicsSceneMouseEvent *event) |
void | registerAsDragHandle (QGraphicsItem *item) |
void | resizeEvent (QGraphicsSceneResizeEvent *event) |
virtual void | saveState (KConfigGroup &config) const |
bool | sceneEventFilter (QGraphicsItem *watched, QEvent *event) |
void | setConfigurationRequired (bool needsConfiguring, const QString &reason=QString()) |
void | setFailedToLaunch (bool failed, const QString &reason=QString()) |
void | setHasConfigurationInterface (bool hasInterface) |
QPainterPath | shape () const |
void | showMessage (const QIcon &icon, const QString &message, const Plasma::MessageButtons buttons) |
QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
void | timerEvent (QTimerEvent *event) |
void | unregisterAsDragHandle (QGraphicsItem *item) |
Properties | |
BackgroundHints | backgroundHints |
bool | busy |
QString | category |
bool | configurationRequired |
QRectF | geometry |
bool | hasConfigurationInterface |
bool | hasFailedToLaunch |
uint | id |
ImmutabilityType | immutability |
bool | isBusy |
QString | name |
QString | pluginName |
bool | shouldConserveResources |
bool | userConfiguring |
Detailed Description
The base Applet class.
Applet provides several important roles for add-ons widgets in Plasma.
First, it is the base class for the plugin system and therefore is the interface to applets for host applications. It also handles the life time management of data engines (e.g. all data engines accessed via Applet::dataEngine(const QString&) are properly deref'd on Applet destruction), background painting (allowing for consistent and complex look and feel in just one line of code for applets), loading and starting of scripting support for each applet, providing access to the associated plasmoid package (if any) and access to configuration data.
See techbase.kde.org for tutorials on writing Applets using this class.
Member Typedef Documentation
typedef QHash<QString, Applet*> Plasma::Applet::Dict |
typedef QList<Applet*> Plasma::Applet::List |
Member Enumeration Documentation
Description on how draw a background for the applet.
Constructor & Destructor Documentation
Plasma::Applet::~Applet | ( | ) |
Definition at line 207 of file applet.cpp.
|
explicit |
- Parameters
-
parent the QGraphicsItem this applet is parented to serviceId the name of the .desktop file containing the information about the widget appletId a unique id used to differentiate between multiple instances of the same Applet type
Definition at line 136 of file applet.cpp.
|
explicit |
- Parameters
-
parent the QGraphicsItem this applet is parented to info the plugin information object for this Applet appletId a unique id used to differentiate between multiple instances of the same Applet type
- Since
- 4.6
Definition at line 127 of file applet.cpp.
|
explicit |
- Parameters
-
parent the QGraphicsItem this applet is parented to serviceId the name of the .desktop file containing the information about the widget appletId a unique id used to differentiate between multiple instances of the same Applet type args a list of strings containing two entries: the service id and the applet id
- Since
- 4.3
Definition at line 145 of file applet.cpp.
|
protected |
This constructor is to be used with the plugin loading systems found in KPluginInfo and KService.
The argument list is expected to have two elements: the KService service ID for the desktop entry and an applet ID which must be a base 10 number.
- Parameters
-
parent a QObject parent; you probably want to pass in 0 args a list of strings containing two entries: the service id and the applet id
Definition at line 169 of file applet.cpp.
Member Function Documentation
QAction * Plasma::Applet::action | ( | QString | name | ) | const |
Returns the QAction with the given name from our collection.
Definition at line 1390 of file applet.cpp.
|
signal |
Emitted when activation is requested due to, for example, a global keyboard shortcut.
By default the wiget is given focus.
void Plasma::Applet::addAction | ( | QString | name, |
QAction * | action | ||
) |
Adds the action to our collection under the given name.
Definition at line 1395 of file applet.cpp.
|
virtual |
associate actions with this widget, including ones added after this call.
needed to make keyboard shortcuts work.
Reimplemented in Plasma::Containment.
Definition at line 1608 of file applet.cpp.
|
signal |
Emitted when the applet is deleted.
|
signal |
Emitted when the user completes a transformation of the applet.
|
signal |
Emitted when the applet changes its own geometry or transform.
Plasma::AspectRatioMode Plasma::Applet::aspectRatioMode | ( | ) | const |
- Returns
- the preferred aspect ratio mode for placement and resizing
Definition at line 1631 of file applet.cpp.
QString Plasma::Applet::associatedApplication | ( | ) | const |
- Returns
- the application associated to this applet
- Since
- 4.4
Definition at line 2247 of file applet.cpp.
KUrl::List Plasma::Applet::associatedApplicationUrls | ( | ) | const |
BackgroundHints Plasma::Applet::backgroundHints | ( | ) | const |
- Returns
- BackgroundHints flags combination telling if the standard background is shown and if it has a drop shadow
|
static |
Get the category of the given applet.
- Parameters
-
applet a KPluginInfo object for the applet
Definition at line 870 of file applet.cpp.
|
static |
Get the category of the given applet.
- Parameters
-
appletName the name of the applet
Definition at line 875 of file applet.cpp.
QString Plasma::Applet::category | ( | ) | const |
Returns the category the applet is in, as specified in the .desktop file.
KConfigGroup Plasma::Applet::config | ( | ) | const |
Returns the KConfigGroup to access the applets configuration.
This config object will write to an instance specific config file named <appletname><instanceid>rc in the Plasma appdata directory.
Definition at line 450 of file applet.cpp.
KConfigGroup Plasma::Applet::config | ( | const QString & | group | ) | const |
Returns a config group with the name provided.
This ensures that the group name is properly namespaced to avoid collision with other applets that may be sharing this config file
- Parameters
-
group the name of the group to access
Definition at line 440 of file applet.cpp.
|
virtualslot |
Called when applet configuration values have changed.
Definition at line 2198 of file applet.cpp.
|
signal |
Emitted when an applet has changed values in its configuration and wishes for them to be saved at the next save point.
As this implies disk activity, this signal should be used with care.
- Note
- This does not need to be emitted from saveState by individual applets.
ConfigLoader * Plasma::Applet::configScheme | ( | ) | const |
Returns the config skeleton object from this applet's package, if any.
- Returns
- config skeleton object, or 0 if none
Definition at line 675 of file applet.cpp.
bool Plasma::Applet::configurationRequired | ( | ) | const |
- Returns
- true if the applet currently needs to be configured, otherwise, false
|
protectedvirtual |
Definition at line 755 of file applet.cpp.
Containment * Plasma::Applet::containment | ( | ) | const |
- Returns
- the Containment, if any, this applet belongs to
Definition at line 1518 of file applet.cpp.
Context * Plasma::Applet::context | ( | ) | const |
Returns the workspace context which the applet is operating in.
Definition at line 1624 of file applet.cpp.
|
virtual |
Returns a list of context-related QAction instances.
This is used e.g. within the DesktopView to display a contextmenu.
- Returns
- A list of actions. The default implementation returns an empty list.
Definition at line 1384 of file applet.cpp.
|
virtual |
Reimplement this method so provide a configuration interface, parented to the supplied widget.
Ownership of the widgets is passed to the parent widget.
Typically one would add custom pages to the config dialog parent
and then connect to the applyClicked() and okClicked() signals or parent
to react on config changes:
With this approach it makes sense to store the custom pages in member variables to make their fields accessible from the myConfigAccepted() slot.
Use config() to store your configuration.
- Parameters
-
parent the dialog which is the parent of the configuration widgets
Definition at line 2208 of file applet.cpp.
QStringList Plasma::Applet::customCategories | ( | ) |
- Returns
- the list of custom categories known to libplasma
- Since
- 4.3
Definition at line 2399 of file applet.cpp.
DataEngine * Plasma::Applet::dataEngine | ( | const QString & | name | ) | const |
Loads the given DataEngine.
Tries to load the data engine given by name
. Each engine is only loaded once, and that instance is re-used on all subsequent requests.
If the data engine was not found, an invalid data engine is returned (see DataEngine::isValid()).
Note that you should not delete the returned engine.
- Parameters
-
name Name of the data engine to load
- Returns
- pointer to the data engine if it was loaded, or an invalid data engine if the requested engine could not be loaded
Definition at line 680 of file applet.cpp.
|
virtualslot |
Destroys the applet; it will be removed nicely and deleted.
Its configuration will also be deleted.
Definition at line 479 of file applet.cpp.
bool Plasma::Applet::destroyed | ( | ) | const |
- Returns
- true if destroy() was called; useful for Applets which should avoid certain tasks if they are about to be deleted permanently
Definition at line 497 of file applet.cpp.
|
protected |
event filter; used for focus watching
Definition at line 1774 of file applet.cpp.
|
protected |
- Returns
- the extender of this applet.
Definition at line 782 of file applet.cpp.
|
signal |
Emitted when an ExtenderItem in a scripting applet needs to be initialized.
|
slot |
Sends all pending contraints updates to the applet.
Will usually be called automatically, but can also be called manually if needed.
Definition at line 1204 of file applet.cpp.
|
protected |
Reimplemented from QGraphicsItem.
Definition at line 1869 of file applet.cpp.
QFont Plasma::Applet::font | ( | ) | const |
- Returns
- the font currently set for this widget
Definition at line 829 of file applet.cpp.
|
virtual |
Returns the current form factor the applet is being displayed in.
- See also
- Plasma::FormFactor
Definition at line 1479 of file applet.cpp.
|
signal |
Emitted whenever the applet makes a geometry change, so that views can coordinate themselves with these changes if they desire.
KConfigGroup Plasma::Applet::globalConfig | ( | ) | const |
Returns a KConfigGroup object to be shared by all applets of this type.
This config object will write to an applet-specific config object named plasma_<appletname>rc in the local config directory.
Definition at line 463 of file applet.cpp.
KShortcut Plasma::Applet::globalShortcut | ( | ) | const |
- Returns
- the global shortcut associated with this wiget, or an empty shortcut if no global shortcut is associated.
Definition at line 1594 of file applet.cpp.
bool Plasma::Applet::hasAuthorization | ( | const QString & | constraint | ) | const |
Returns true if the applet is allowed to perform functions covered by the given constraint eg.
hasAuthorization("FileDialog") returns true if applets are allowed to show filedialogs.
- Since
- 4.3
Definition at line 2215 of file applet.cpp.
bool Plasma::Applet::hasConfigurationInterface | ( | ) | const |
- Returns
- true if this plasmoid provides a GUI configuration
bool Plasma::Applet::hasFailedToLaunch | ( | ) | const |
If for some reason, the applet fails to get up on its feet (the library couldn't be loaded, necessary hardware support wasn't found, etc..) this method returns true.
bool Plasma::Applet::hasValidAssociatedApplication | ( | ) | const |
- Returns
- true if the applet has a valid associated application or urls
- Since
- 4.4
Definition at line 2264 of file applet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 2543 of file applet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 2548 of file applet.cpp.
QString Plasma::Applet::icon | ( | ) | const |
Returns the icon related to this applet.
Definition at line 834 of file applet.cpp.
uint Plasma::Applet::id | ( | ) | const |
- Returns
- the id of this applet
ImmutabilityType Plasma::Applet::immutability | ( | ) | const |
- Returns
- The type of immutability of this applet
|
signal |
Emitted when the immutability changes.
- Since
- 4.4
|
virtualslot |
This method is called once the applet is loaded and added to a Corona.
If the applet requires a QGraphicsScene or has an particularly intensive set of initialization routines to go through, consider implementing it in this method instead of the constructor.
Note: paintInterface may get called before init() depending on initialization order. Painting is managed by the canvas (QGraphisScene), and may schedule a paint event prior to init() being called.
Reimplemented in Plasma::Containment.
Definition at line 243 of file applet.cpp.
|
virtual |
Gets called when an extender item has to be initialized after a plasma restart.
If you create ExtenderItems in your applet, you should implement this function to again create the widget that should be shown in this extender item. This function might look something like this:
You can also add one or more custom qactions to this extender item in this function.
Note that by default, not all ExtenderItems are persistent. Only items that are detached, will have their configuration stored when plasma exits.
Definition at line 769 of file applet.cpp.
bool Plasma::Applet::isBusy | ( | ) | const |
- Returns
- true if the applet is busy and is showing an indicator widget for that
bool Plasma::Applet::isContainment | ( | ) | const |
- Returns
- true if this Applet is currently being used as a Containment, false otherwise
Definition at line 2647 of file applet.cpp.
|
virtual |
- Returns
- true is there is a popup assoiated with this Applet showing, such as the dialog of a PopupApplet. May be reimplemented for custom popup implementations.
Reimplemented in Plasma::PopupApplet.
Definition at line 1603 of file applet.cpp.
|
slot |
Definition at line 1711 of file applet.cpp.
|
protected |
- Parameters
-
item the item to look for if it is registered or not
- Returns
- true if it is registered, false otherwise
Definition at line 1670 of file applet.cpp.
|
slot |
- Returns
- true when the configuration interface is being shown
- Since
- 4.5
Definition at line 1893 of file applet.cpp.
|
protected |
Reimplemented from QGraphicsItem.
Definition at line 2436 of file applet.cpp.
|
static |
Returns a list of all known applets.
This may skip applets based on security settings and ExcludeCategories in the application's config.
- Parameters
-
category Only applets matchin this category will be returned. Useful in conjunction with knownCategories. If "Misc" is passed in, then applets without a Categories= entry are also returned. If an empty string is passed in, all applets are returned. parentApp the application to filter applets on. Uses the X-KDE-ParentApp entry (if any) in the plugin info. The default value of QString() will result in a list containing only applets not specifically registered to an application.
- Returns
- list of applets
Definition at line 2312 of file applet.cpp.
|
static |
Returns a list of all known applets associated with a certain mimetype.
- Returns
- list of applets
Definition at line 2317 of file applet.cpp.
|
static |
Returns a list of all known applets associated with a certain URL.
- Since
- 4.4
- Returns
- list of applets
Definition at line 2327 of file applet.cpp.
|
static |
Returns a list of all the categories used by installed applets.
- Parameters
-
parentApp the application to filter applets on. Uses the X-KDE-ParentApp entry (if any) in the plugin info. The default value of QString() will result in a list containing only applets not specifically registered to an application.
- Returns
- list of categories
- Parameters
-
visibleOnly true if it should only return applets that are marked as visible
Definition at line 2351 of file applet.cpp.
|
static |
Attempts to load an applet.
Returns a pointer to the applet if successful. The caller takes responsibility for the applet, including deleting it when no longer needed.
- Parameters
-
name the plugin name, as returned by KPluginInfo::pluginName() appletId unique ID to assign the applet, or zero to have one assigned automatically. args to send the applet extra arguments
- Returns
- a pointer to the loaded applet, or 0 on load failure
Definition at line 2422 of file applet.cpp.
|
static |
Attempts to load an applet.
Returns a pointer to the applet if successful. The caller takes responsibility for the applet, including deleting it when no longer needed.
- Parameters
-
info KPluginInfo object for the desired applet appletId unique ID to assign the applet, or zero to have one assigned automatically. args to send the applet extra arguments
- Returns
- a pointer to the loaded applet, or 0 on load failure
Definition at line 2427 of file applet.cpp.
|
static |
Attempts to load an applet from a package.
Returns a pointer to the applet if successful. The caller takes responsibility for the applet, including deleting it when no longer needed.
- Parameters
-
path the path to the package appletId unique ID to assign the applet, or zero to have one assigned automatically. args to send the applet extra arguments
- Returns
- a pointer to the loaded applet, or 0 on load failure
- Since
- 4.3
Definition at line 2404 of file applet.cpp.
|
virtual |
Returns the location of the scene which is displaying applet.
- See also
- Plasma::Location
Definition at line 1618 of file applet.cpp.
|
slot |
Causes this applet to lower below all the other applets.
Definition at line 2622 of file applet.cpp.
QRectF Plasma::Applet::mapFromView | ( | const QGraphicsView * | view, |
const QRect & | rect | ||
) | const |
Maps a QRect from a view's coordinates to local coordinates.
- Parameters
-
view the view from which rect should be mapped rect the rect to be mapped
Definition at line 725 of file applet.cpp.
QRect Plasma::Applet::mapToView | ( | const QGraphicsView * | view, |
const QRectF & | rect | ||
) | const |
Maps a QRectF from local coordinates to a view's coordinates.
- Parameters
-
view the view to which rect should be mapped rect the rect to be mapped
Definition at line 731 of file applet.cpp.
|
signal |
Emitted when the user clicked on a button of the message overlay.
- See also
- showMessage
- Plasma::MessageButton
- Since
- 4.3
|
protected |
manage the mouse movement to drag the applet around
Definition at line 1862 of file applet.cpp.
QString Plasma::Applet::name | ( | ) | const |
Returns the user-visible name for the applet, as specified in the .desktop file.
- Returns
- the user-visible name for the applet.
|
signal |
Emitted when the applet status changes.
- Since
- 4.4
const Package * Plasma::Applet::package | ( | ) | const |
Accessor for the associated Package object if any.
Generally, only Plasmoids come in a Package.
- Returns
- the Package object, or 0 if none
Definition at line 691 of file applet.cpp.
|
static |
- Returns
- a package structure representing an Applet
Definition at line 234 of file applet.cpp.
|
virtual |
This method is called when the interface should be painted.
- Parameters
-
painter the QPainter to use to do the paintiner option the style options object contentsRect the rect to paint within; automatically adjusted for the background, if any
Definition at line 1470 of file applet.cpp.
void Plasma::Applet::paintWindowFrame | ( | QPainter * | painter, |
const QStyleOptionGraphicsItem * | option, | ||
QWidget * | widget | ||
) |
Definition at line 994 of file applet.cpp.
QString Plasma::Applet::pluginName | ( | ) | const |
Returns the plugin name for the applet.
QPoint Plasma::Applet::popupPosition | ( | const QSize & | s | ) | const |
Reccomended position for a popup window like a menu or a tooltip given its size.
- Parameters
-
s size of the popup
- Returns
- reccomended position
Definition at line 737 of file applet.cpp.
QPoint Plasma::Applet::popupPosition | ( | const QSize & | s, |
Qt::AlignmentFlag | alignment | ||
) | const |
- Since
- 4.4 Reccomended position for a popup window like a menu or a tooltip given its size
- Parameters
-
s size of the popup alignment alignment of the popup, valid flags are Qt::AlignLeft, Qt::AlignRight and Qt::AlignCenter
- Returns
- reccomended position
Definition at line 742 of file applet.cpp.
|
slot |
Publishes and optionally announces this applet on the network for remote access.
- Parameters
-
methods the methods to use for announcing this applet. resourceName the name under which this applet will be published (has to be unique for each machine)
Definition at line 1680 of file applet.cpp.
|
slot |
Causes this applet to raise above all other applets.
Definition at line 2617 of file applet.cpp.
|
protected |
Register the widgets that manage mouse clicks but you still want to be able to drag the applet around when holding the mouse pointer on that widget.
Calling this results in an eventFilter being places on the widget.
- Parameters
-
item the item to watch for mouse move
Definition at line 1647 of file applet.cpp.
|
signal |
This signal indicates that an application launch, window creation or window focus event was triggered.
This is used, for instance, to ensure that the Dashboard view in Plasma Desktop hides when such an event is triggered by an item it is displaying.
|
virtual |
un-associate actions from this widget, including ones added after this call.
needed to make keyboard shortcuts work.
Reimplemented in Plasma::Containment.
Definition at line 1613 of file applet.cpp.
|
protected |
Reimplemented from QGraphicsItem.
Definition at line 1879 of file applet.cpp.
|
virtual |
Restores state information about this applet saved previously in save(KConfigGroup&).
This method does not need to be reimplmented by Applet subclasses, but can be useful for Applet specializations (such as Containment) to do so.
Reimplemented in Plasma::Containment.
Definition at line 310 of file applet.cpp.
|
slot |
Open the application associated to this applet, if it's not set but some urls are, open those urls with the proper application for their mimetype.
- Since
- 4.4
Definition at line 2257 of file applet.cpp.
|
virtual |
Saves state information about this applet that will be accessed when next instantiated in the restore(KConfigGroup&) method.
This method does not need to be reimplmented by Applet subclasses, but can be useful for Applet specializations (such as Containment) to do so.
Applet subclasses may instead want to reimplement saveState().
Reimplemented in Plasma::Containment.
Definition at line 260 of file applet.cpp.
|
protectedvirtual |
When called, the Applet should write any information needed as part of the Applet's running state to the configuration object in config() and/or globalConfig().
Applets that always sync their settings/state with the config objects when these settings/states change do not need to reimplement this method.
Definition at line 426 of file applet.cpp.
|
protected |
scene event filter; used to manage applet dragging
Definition at line 1779 of file applet.cpp.
QRect Plasma::Applet::screenRect | ( | ) | const |
This method returns screen coordinates for the widget; this method can be somewhat expensive and should ONLY be called when screen coordinates are required.
For example when positioning top level widgets on top of the view to create the appearance of unit. This should NOT be used for popups (
- See also
- popupPosition) or for normal widget use (use Plasma:: widgets or QGraphicsProxyWidget instead).
- Returns
- a rect of the applet in screen coordinates.
Definition at line 2598 of file applet.cpp.
void Plasma::Applet::setAspectRatioMode | ( | Plasma::AspectRatioMode | mode | ) |
Sets the preferred aspect ratio mode for placement and resizing.
Definition at line 1636 of file applet.cpp.
void Plasma::Applet::setAssociatedApplication | ( | const QString & | string | ) |
Sets an application associated to this applet, that will be regarded as a full view of what is represented in the applet.
- Parameters
-
string the name of the application. it can be - a name understood by KService::serviceByDesktopName (e.g. "konqueror")
- a command in $PATH
- or an absolute path to an executable
- Since
- 4.4
Definition at line 2221 of file applet.cpp.
void Plasma::Applet::setAssociatedApplicationUrls | ( | const KUrl::List & | urls | ) |
Sets a list of urls associated to this application, they will be used as parameters for the associated application.
- See also
- setAssociatedApplication()
- Parameters
-
urls
Definition at line 2234 of file applet.cpp.
void Plasma::Applet::setBackgroundHints | ( | const BackgroundHints | hints | ) |
Sets the BackgroundHints for this applet.
- See also
- BackgroundHint
- Parameters
-
hints the BackgroundHint combination for this applet
Definition at line 938 of file applet.cpp.
|
slot |
Shows a busy indicator that overlays the applet.
- Parameters
-
busy show or hide the busy indicator
Definition at line 791 of file applet.cpp.
|
protected |
When the applet needs to be configured before being usable, this method can be called to show a standard interface prompting the user to configure the applet.
- Parameters
-
needsConfiguring true if the applet needs to be configured, or false if it doesn't reason a translated message for the user explaining that the applet needs configuring; this should note what needs to be configured
Definition at line 1010 of file applet.cpp.
void Plasma::Applet::setCustomCategories | ( | const QStringList & | categories | ) |
Sets the list of custom categories that are used in addition to the default set of categories known to libplasma for Applets.
- Parameters
-
categories a list of categories
- Since
- 4.3
Definition at line 2394 of file applet.cpp.
|
protected |
Call this method when the applet fails to launch properly.
An optional reason can be provided.
Not that all children items will be deleted when this method is called. If you have pointers to these items, you will need to reset them after calling this method.
- Parameters
-
failed true when the applet failed, false when it succeeded reason an optional reason to show the user why the applet failed to launch
Definition at line 366 of file applet.cpp.
void Plasma::Applet::setGlobalShortcut | ( | const KShortcut & | shortcut | ) |
Sets the global shorcut to associate with this widget.
Definition at line 1556 of file applet.cpp.
|
protected |
Sets whether or not this applet provides a user interface for configuring the applet.
It defaults to false, and if true is passed in you should also reimplement createConfigurationInterface()
- Parameters
-
hasInterface whether or not there is a user interface available
Definition at line 1724 of file applet.cpp.
|
slot |
Sets the immutability type for this applet (not immutable, user immutable or system immutable)
- Parameters
-
immutable the new immutability type of this applet
Definition at line 919 of file applet.cpp.
|
slot |
|
protected |
Reimplemented from QGraphicsItem.
Definition at line 2500 of file applet.cpp.
bool Plasma::Applet::shouldConserveResources | ( | ) | const |
Whether the applet should conserve resources.
If true, try to avoid doing stuff which is computationally heavy. Try to conserve power and resources.
- Returns
- true if it should conserve resources, false if it does not.
|
virtualslot |
Lets the user interact with the plasmoid options.
Called when the user selects the configure entry from the context menu.
Unless there is good reason for overriding this method, Applet subclasses should actually override createConfigurationInterface instead. A good example of when this isn't plausible is when using a dialog prepared by another library, such as KPropertiesDialog from libkfile. You probably want to call showConfigurationInterface(QWidget*) with the custom widget you created to actually show your interface
Definition at line 1898 of file applet.cpp.
|
slot |
Actually show your custom configuration interface Use this only if you reimplemented showConfigurationInterface()
- Parameters
-
widget the widget representing your configuration interface
- Since
- 4.5
Definition at line 2007 of file applet.cpp.
|
protected |
Shows a message as an overlay of the applet: the message has an icon, text and (optional) buttons.
- Parameters
-
icon the icon that will be shown message the message string that will be shown. If the message is empty nothng will be shown and if there was a message already it will be hidden buttons an OR combination of all the buttons needed
- See also
- Plasma::MessageButtons
- messageButtonPressed
- Since
- 4.3
Definition at line 1062 of file applet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 2509 of file applet.cpp.
|
signal |
Emitted by Applet subclasses when they change a sizeHint and wants to announce the change.
|
slot |
- Returns
- the list of arguments which the applet was called with
- Since
- KDE4.3
Definition at line 1188 of file applet.cpp.
|
slot |
|
protected |
Reimplemented from QObject.
Definition at line 2553 of file applet.cpp.
int Plasma::Applet::type | ( | ) | const |
Reimplemented from QGraphicsItem.
Definition at line 1379 of file applet.cpp.
|
slot |
Definition at line 1700 of file applet.cpp.
|
protected |
Unregister a widget registered with registerAsDragHandle.
- Parameters
-
item the item to unregister
Definition at line 1657 of file applet.cpp.
void Plasma::Applet::updateConstraints | ( | Plasma::Constraints | constraints = Plasma::AllConstraints | ) |
Called when any of the geometry constraints have been updated.
This method calls constraintsEvent, which may be reimplemented, once the Applet has been prepared for updating the constraints.
- Parameters
-
constraints the type of constraints that were updated
Definition at line 750 of file applet.cpp.
QGraphicsView * Plasma::Applet::view | ( | ) | const |
Returns the view this widget is visible on, or 0 if none can be found.
- Warning
- do NOT assume this will always return a view! a null view probably means that either plasma isn't finished loading, or your applet is on an activity that's not being shown anywhere.
Definition at line 696 of file applet.cpp.
Property Documentation
|
readwrite |
|
readwrite |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:34 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.