Plasma
#include <popupapplet.h>
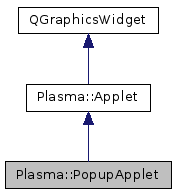
Public Slots | |
void | hidePopup () |
void | showPopup (uint displayTime=0) |
void | togglePopup () |
![]() | |
virtual void | configChanged () |
virtual void | destroy () |
void | flushPendingConstraintsEvents () |
virtual void | init () |
bool | isPublished () const |
bool | isUserConfiguring () const |
void | lower () |
void | publish (Plasma::AnnouncementMethods methods, const QString &resourceName) |
void | raise () |
void | runAssociatedApplication () |
void | setBusy (bool busy) |
void | setImmutability (const ImmutabilityType immutable) |
void | setStatus (const ItemStatus stat) |
virtual void | showConfigurationInterface () |
void | showConfigurationInterface (QWidget *widget) |
QVariantList | startupArguments () const |
ItemStatus | status () const |
void | unpublish () |
Public Member Functions | |
PopupApplet (QObject *parent, const QVariantList &args) | |
~PopupApplet () | |
virtual QGraphicsWidget * | graphicsWidget () |
bool | isIconified () const |
bool | isPassivePopup () const |
bool | isPopupShowing () const |
Qt::AlignmentFlag | popupAlignment () const |
QIcon | popupIcon () const |
Plasma::PopupPlacement | popupPlacement () const |
void | setGraphicsWidget (QGraphicsWidget *widget) |
void | setPassivePopup (bool passive) |
void | setPopupAlignment (Qt::AlignmentFlag alignment) |
void | setPopupIcon (const QIcon &icon) |
void | setPopupIcon (const QString &iconName) |
void | setWidget (QWidget *widget) |
virtual QWidget * | widget () |
![]() | |
Applet (QGraphicsItem *parent=0, const QString &serviceId=QString(), uint appletId=0) | |
Applet (const KPluginInfo &info, QGraphicsItem *parent=0, uint appletId=0) | |
Applet (QGraphicsItem *parent, const QString &serviceId, uint appletId, const QVariantList &args) | |
~Applet () | |
Q_INVOKABLE QAction * | action (QString name) const |
void | addAction (QString name, QAction *action) |
virtual void | addAssociatedWidget (QWidget *widget) |
Plasma::AspectRatioMode | aspectRatioMode () const |
QString | associatedApplication () const |
KUrl::List | associatedApplicationUrls () const |
BackgroundHints | backgroundHints () const |
QString | category () const |
KConfigGroup | config () const |
KConfigGroup | config (const QString &group) const |
ConfigLoader * | configScheme () const |
bool | configurationRequired () const |
Containment * | containment () const |
Context * | context () const |
virtual QList< QAction * > | contextualActions () |
virtual void | createConfigurationInterface (KConfigDialog *parent) |
QStringList | customCategories () |
Q_INVOKABLE DataEngine * | dataEngine (const QString &name) const |
bool | destroyed () const |
QFont | font () const |
virtual FormFactor | formFactor () const |
KConfigGroup | globalConfig () const |
KShortcut | globalShortcut () const |
bool | hasAuthorization (const QString &constraint) const |
bool | hasConfigurationInterface () const |
bool | hasFailedToLaunch () const |
bool | hasValidAssociatedApplication () const |
QString | icon () const |
uint | id () const |
ImmutabilityType | immutability () const |
virtual void | initExtenderItem (ExtenderItem *item) |
bool | isBusy () const |
bool | isContainment () const |
virtual Location | location () const |
QRectF | mapFromView (const QGraphicsView *view, const QRect &rect) const |
QRect | mapToView (const QGraphicsView *view, const QRectF &rect) const |
QString | name () const |
const Package * | package () const |
virtual void | paintInterface (QPainter *painter, const QStyleOptionGraphicsItem *option, const QRect &contentsRect) |
void | paintWindowFrame (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget) |
QString | pluginName () const |
QPoint | popupPosition (const QSize &s) const |
QPoint | popupPosition (const QSize &s, Qt::AlignmentFlag alignment) const |
virtual void | removeAssociatedWidget (QWidget *widget) |
virtual void | restore (KConfigGroup &group) |
virtual void | save (KConfigGroup &group) const |
QRect | screenRect () const |
void | setAspectRatioMode (Plasma::AspectRatioMode) |
void | setAssociatedApplication (const QString &string) |
void | setAssociatedApplicationUrls (const KUrl::List &urls) |
void | setBackgroundHints (const BackgroundHints hints) |
void | setCustomCategories (const QStringList &categories) |
void | setGlobalShortcut (const KShortcut &shortcut) |
bool | shouldConserveResources () const |
int | type () const |
void | updateConstraints (Plasma::Constraints constraints=Plasma::AllConstraints) |
QGraphicsView * | view () const |
Protected Member Functions | |
void | dragEnterEvent (QGraphicsSceneDragDropEvent *event) |
void | dragLeaveEvent (QGraphicsSceneDragDropEvent *event) |
void | dropEvent (QGraphicsSceneDragDropEvent *event) |
bool | eventFilter (QObject *watched, QEvent *event) |
void | mousePressEvent (QGraphicsSceneMouseEvent *event) |
void | mouseReleaseEvent (QGraphicsSceneMouseEvent *event) |
virtual void | popupEvent (bool show) |
QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
void | timerEvent (QTimerEvent *event) |
![]() | |
Applet (QObject *parent, const QVariantList &args) | |
virtual void | constraintsEvent (Plasma::Constraints constraints) |
bool | eventFilter (QObject *o, QEvent *e) |
Extender * | extender () const |
void | focusInEvent (QFocusEvent *event) |
void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
bool | isRegisteredAsDragHandle (QGraphicsItem *item) |
QVariant | itemChange (GraphicsItemChange change, const QVariant &value) |
void | mouseMoveEvent (QGraphicsSceneMouseEvent *event) |
void | registerAsDragHandle (QGraphicsItem *item) |
void | resizeEvent (QGraphicsSceneResizeEvent *event) |
virtual void | saveState (KConfigGroup &config) const |
bool | sceneEventFilter (QGraphicsItem *watched, QEvent *event) |
void | setConfigurationRequired (bool needsConfiguring, const QString &reason=QString()) |
void | setFailedToLaunch (bool failed, const QString &reason=QString()) |
void | setHasConfigurationInterface (bool hasInterface) |
QPainterPath | shape () const |
void | showMessage (const QIcon &icon, const QString &message, const Plasma::MessageButtons buttons) |
QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
void | timerEvent (QTimerEvent *event) |
void | unregisterAsDragHandle (QGraphicsItem *item) |
Properties | |
Qt::AlignmentFlag | popupAlignment |
![]() | |
BackgroundHints | backgroundHints |
bool | busy |
QString | category |
bool | configurationRequired |
QRectF | geometry |
bool | hasConfigurationInterface |
bool | hasFailedToLaunch |
uint | id |
ImmutabilityType | immutability |
bool | isBusy |
QString | name |
QString | pluginName |
bool | shouldConserveResources |
bool | userConfiguring |
Additional Inherited Members | |
![]() | |
enum | { Type = Plasma::AppletType } |
enum | BackgroundHint { NoBackground = 0, StandardBackground = 1, TranslucentBackground = 2, DefaultBackground = StandardBackground } |
typedef QHash< QString, Applet * > | Dict |
typedef QList< Applet * > | List |
![]() | |
void | activate () |
void | appletDestroyed (Plasma::Applet *applet) |
void | appletTransformedByUser () |
void | appletTransformedItself () |
void | configNeedsSaving () |
void | extenderItemRestored (Plasma::ExtenderItem *item) |
void | geometryChanged () |
void | immutabilityChanged (Plasma::ImmutabilityType immutable) |
void | messageButtonPressed (const Plasma::MessageButton button) |
void | newStatus (Plasma::ItemStatus status) |
void | releaseVisualFocus () |
void | sizeHintChanged (Qt::SizeHint which) |
![]() | |
static QString | category (const KPluginInfo &applet) |
static QString | category (const QString &appletName) |
static KPluginInfo::List | listAppletInfo (const QString &category=QString(), const QString &parentApp=QString()) |
static KPluginInfo::List | listAppletInfoForMimetype (const QString &mimetype) |
static KPluginInfo::List | listAppletInfoForUrl (const QUrl &url) |
static QStringList | listCategories (const QString &parentApp=QString(), bool visibleOnly=true) |
static Applet * | load (const QString &name, uint appletId=0, const QVariantList &args=QVariantList()) |
static Applet * | load (const KPluginInfo &info, uint appletId=0, const QVariantList &args=QVariantList()) |
static Applet * | loadPlasmoid (const QString &path, uint appletId=0, const QVariantList &args=QVariantList()) |
static PackageStructure::Ptr | packageStructure () |
Detailed Description
Allows applets to automatically 'collapse' into an icon when put in an panel, and is a convenient base class for any applet that wishes to use extenders.
Applets that subclass this class should implement either widget() or graphicsWidget() to return a widget that will be displayed in the applet if the applet is in a Planar or MediaCenter form factor. If the applet is put in a panel, an icon will be displayed instead, which shows the widget in a popup when clicked.
If you use this class as a base class for your extender using applet, the extender will automatically be used for the popup; reimplementing graphicsWidget() is unnecessary in this case. If you need a popup that does not steal window focus when openend or used, set window flag Qt::X11BypassWindowManagerHint the widget returned by widget() or graphicsWidget().
Definition at line 52 of file popupapplet.h.
Constructor & Destructor Documentation
Plasma::PopupApplet::PopupApplet | ( | QObject * | parent, |
const QVariantList & | args | ||
) |
Definition at line 59 of file popupapplet.cpp.
Plasma::PopupApplet::~PopupApplet | ( | ) |
Definition at line 71 of file popupapplet.cpp.
Member Function Documentation
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 546 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 558 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 577 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 504 of file popupapplet.cpp.
|
virtual |
Implement either this function or widget().
- Returns
- the widget that will get shown in either a layout, in the applet or in a Dialog, depending on the form factor of the applet. If you set a popup icon you must also set a minimum size of the applet. When the applet is smaller than this minimum size, it will be displayed as that icon.
Definition at line 163 of file popupapplet.cpp.
|
slot |
Hides the popup.
Definition at line 635 of file popupapplet.cpp.
bool Plasma::PopupApplet::isIconified | ( | ) | const |
- Returns
- true if the applet is collapsed to an icon
- Since
- 4.6
Definition at line 692 of file popupapplet.cpp.
bool Plasma::PopupApplet::isPassivePopup | ( | ) | const |
- Returns
- true if the dialog will be treated as a passive poup
Definition at line 682 of file popupapplet.cpp.
|
virtual |
- Returns
- true if the applet is popped up
Reimplemented from Plasma::Applet.
Definition at line 687 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 482 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 494 of file popupapplet.cpp.
Qt::AlignmentFlag Plasma::PopupApplet::popupAlignment | ( | ) | const |
- Returns
- the default alignment of the popup relative to the applet
- Since
- 4.6
|
protectedvirtual |
This event handler can be reimplemented in a subclass to receive an event before the popup is shown or hidden.
- Parameters
-
show true if the popup is going to be shown, false if the popup is going to be hidden. Note that showing and hiding the popup on click is already done in PopupApplet.
Definition at line 670 of file popupapplet.cpp.
QIcon Plasma::PopupApplet::popupIcon | ( | ) | const |
- Returns
- the icon that is displayed when the applet is in a panel.
Definition at line 122 of file popupapplet.cpp.
Plasma::PopupPlacement Plasma::PopupApplet::popupPlacement | ( | ) | const |
- Returns
- the placement of the popup relating to the applet
Definition at line 655 of file popupapplet.cpp.
void Plasma::PopupApplet::setGraphicsWidget | ( | QGraphicsWidget * | widget | ) |
Definition at line 172 of file popupapplet.cpp.
void Plasma::PopupApplet::setPassivePopup | ( | bool | passive | ) |
Sets whether or not the dialog popup that gets created should be a "passive" popup that does not steal focus from other windows or not.
- Parameters
-
passive true if the dialog should be treated as a passive popup
Definition at line 677 of file popupapplet.cpp.
void Plasma::PopupApplet::setPopupAlignment | ( | Qt::AlignmentFlag | alignment | ) |
Sets the default alignment of the popup relative to the applet.
- Parameters
-
alignment the alignment to use; Qt::AlignLeft or Qt::AlignRight
- Since
- 4.6
Definition at line 660 of file popupapplet.cpp.
void Plasma::PopupApplet::setPopupIcon | ( | const QIcon & | icon | ) |
- Parameters
-
icon the icon that has to be displayed when the applet is in a panel. Passing in a null icon means that the popup applet itself will provide an interface for when the PopupApplet is not showing the widget() or graphicsWidget() directly.
Definition at line 77 of file popupapplet.cpp.
void Plasma::PopupApplet::setPopupIcon | ( | const QString & | iconName | ) |
- Parameters
-
icon the icon that has to be displayed when the applet is in a panel. Passing in an empty QString() means that the popup applet itself will provide an interface for when the PopupApplet is not showing the widget() or graphicsWidget() directly.
If you set a popup icon you must also set a minimum size of the applet. When the applet is smaller than this minimum size, it will be displayed as that icon.
Definition at line 95 of file popupapplet.cpp.
void Plasma::PopupApplet::setWidget | ( | QWidget * | widget | ) |
Definition at line 132 of file popupapplet.cpp.
|
slot |
Shows the dialog showing the widget if the applet is in a panel.
- Parameters
-
displayTime the time in ms that the popup should be displayed, defaults to 0 which means always (until the user closes it again, that is).
Definition at line 589 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 462 of file popupapplet.cpp.
|
protected |
Reimplemented from QGraphicsLayoutItem.
Definition at line 607 of file popupapplet.cpp.
|
slot |
Toggles the popup.
Definition at line 650 of file popupapplet.cpp.
|
virtual |
Implement either this function or graphicsWidget().
- Returns
- the widget that will get shown in either a layout, in the applet or in a Dialog, depending on the form factor of the applet.
Definition at line 127 of file popupapplet.cpp.
Property Documentation
|
readwrite |
Definition at line 55 of file popupapplet.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.