Plasma
#include <Plasma/RunnerManager>
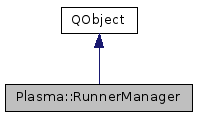
Public Slots | |
bool | execQuery (const QString &term, const QString &runnerName) |
bool | execQuery (const QString &term) |
void | launchQuery (const QString &term, const QString &runnerId) |
void | launchQuery (const QString &term) |
void | matchSessionComplete () |
void | reset () |
void | setupMatchSession () |
Signals | |
void | matchesChanged (const QList< Plasma::QueryMatch > &matches) |
void | queryFinished () |
Public Member Functions | |
RunnerManager (QObject *parent=0) | |
RunnerManager (KConfigGroup &config, QObject *parent=0) | |
~RunnerManager () | |
QList< QAction * > | actionsForMatch (const QueryMatch &match) |
QStringList | allowedRunners () const |
void | loadRunner (const KService::Ptr service) |
void | loadRunner (const QString &path) |
QList< QueryMatch > | matches () const |
QMimeData * | mimeDataForMatch (const QueryMatch &match) const |
QMimeData * | mimeDataForMatch (const QString &id) const |
QString | query () const |
void | reloadConfiguration () |
void | run (const QueryMatch &match) |
void | run (const QString &id) |
AbstractRunner * | runner (const QString &name) const |
QString | runnerName (const QString &id) const |
QList< AbstractRunner * > | runners () const |
RunnerContext * | searchContext () const |
void | setAllowedRunners (const QStringList &runners) |
void | setSingleMode (bool singleMode) |
void | setSingleModeRunnerId (const QString &id) |
bool | singleMode () const |
QStringList | singleModeAdvertisedRunnerIds () const |
AbstractRunner * | singleModeRunner () const |
QString | singleModeRunnerId () const |
Static Public Member Functions | |
static KPluginInfo::List | listRunnerInfo (const QString &parentApp=QString()) |
Detailed Description
The RunnerManager class decides what installed runners are runnable, and their ratings.
It is the main proxy to the runners.
Definition at line 49 of file runnermanager.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 441 of file runnermanager.cpp.
|
explicit |
Definition at line 449 of file runnermanager.cpp.
Plasma::RunnerManager::~RunnerManager | ( | ) |
Definition at line 460 of file runnermanager.cpp.
Member Function Documentation
QList< QAction * > Plasma::RunnerManager::actionsForMatch | ( | const QueryMatch & | match | ) |
Retrieves the list of actions, if any, for a match.
Definition at line 625 of file runnermanager.cpp.
QStringList Plasma::RunnerManager::allowedRunners | ( | ) | const |
|
slot |
Execute a query, this method will only return when the query is executed This means that the method may be dangerous as it wait a variable amount of time for the runner to finish.
The runner parameter is mandatory, to avoid launching unwanted runners.
- Parameters
-
term the term we want to find matches for runner the runner we will use, it is mandatory
- Returns
- 0 if nothing was launched, 1 if launched.
Definition at line 769 of file runnermanager.cpp.
|
slot |
Convenience version of above.
Definition at line 764 of file runnermanager.cpp.
|
slot |
Launch a query, this will create threads and return inmediately.
When the information will be available can be known using the matchesChanged signal.
- Parameters
-
term the term we want to find matches for runnerId optional, if only one specific runner is to be used; providing an id will put the manager into single runner mode
Definition at line 707 of file runnermanager.cpp.
|
slot |
Convenience version of above.
Definition at line 702 of file runnermanager.cpp.
|
static |
Returns a list of all known Runner implementations.
- Parameters
-
parentApp the application to filter applets on. Uses the X-KDE-ParentApp entry (if any) in the plugin info. The default value of QString() will result in a list containing only applets not specifically registered to an application.
- Returns
- list of AbstractRunners
- Since
- 4.6
Definition at line 657 of file runnermanager.cpp.
void Plasma::RunnerManager::loadRunner | ( | const KService::Ptr | service | ) |
Attempts to add the AbstractRunner plugin represented by the KService passed in.
Usually one can simply let the configuration of plugins handle loading Runner plugins, but in cases where specific runners should be loaded this allows for that to take place
- Parameters
-
service the service to use to load the plugin
- Since
- 4.5
Definition at line 492 of file runnermanager.cpp.
void Plasma::RunnerManager::loadRunner | ( | const QString & | path | ) |
Attempts to add the AbstractRunner from a Plasma::Package on disk.
Usually one can simply let the configuration of plugins handle loading Runner plugins, but in cases where specific runners should be loaded this allows for that to take place
- Parameters
-
path the path to a Runner package to load
- Since
- 4.5
Definition at line 504 of file runnermanager.cpp.
QList< QueryMatch > Plasma::RunnerManager::matches | ( | ) | const |
Retrieves all available matches found so far for the previously launched query.
- Returns
- List of matches
Definition at line 591 of file runnermanager.cpp.
|
signal |
Emitted each time a new match is added to the list.
|
slot |
Call this method when the query session is finished for the time being.
- Since
- 4.4
- See also
- prepareForMatchSession
Definition at line 692 of file runnermanager.cpp.
QMimeData * Plasma::RunnerManager::mimeDataForMatch | ( | const QueryMatch & | match | ) | const |
QMimeData * Plasma::RunnerManager::mimeDataForMatch | ( | const QString & | id | ) | const |
QString Plasma::RunnerManager::query | ( | ) | const |
- Returns
- the current query term
Definition at line 809 of file runnermanager.cpp.
|
signal |
Emitted when the launchQuery finish.
- Since
- 4.5
void Plasma::RunnerManager::reloadConfiguration | ( | ) |
Causes a reload of the current configuration.
Definition at line 469 of file runnermanager.cpp.
|
slot |
Reset the current data and stops the query.
Definition at line 814 of file runnermanager.cpp.
void Plasma::RunnerManager::run | ( | const QueryMatch & | match | ) |
Runs a given match.
- Parameters
-
match the match to be executed
Definition at line 601 of file runnermanager.cpp.
void Plasma::RunnerManager::run | ( | const QString & | id | ) |
Runs a given match.
- Parameters
-
id the id of the match to run
Definition at line 596 of file runnermanager.cpp.
AbstractRunner * Plasma::RunnerManager::runner | ( | const QString & | name | ) | const |
Finds and returns a loaded runner or NULL.
- Parameters
-
name the name of the runner
- Returns
- Pointer to the runner
Definition at line 513 of file runnermanager.cpp.
QString Plasma::RunnerManager::runnerName | ( | const QString & | id | ) | const |
Returns the translated name of a runner.
- Parameters
-
id the id of the runner
- Since
- 4.4
Definition at line 576 of file runnermanager.cpp.
QList< AbstractRunner * > Plasma::RunnerManager::runners | ( | ) | const |
- Returns
- the list of all currently loaded runners
Definition at line 566 of file runnermanager.cpp.
RunnerContext * Plasma::RunnerManager::searchContext | ( | ) | const |
Retrieves the current context.
- Returns
- pointer to the current context
Definition at line 585 of file runnermanager.cpp.
void Plasma::RunnerManager::setAllowedRunners | ( | const QStringList & | runners | ) |
Sets a whitelist for the plugins that can be loaded.
- Parameters
-
plugins the plugin names of allowed runners
- Since
- 4.4
Definition at line 475 of file runnermanager.cpp.
void Plasma::RunnerManager::setSingleMode | ( | bool | singleMode | ) |
Sets whether or not the manager is in single mode.
- Parameters
-
singleMode true if the manager should be in single mode, false otherwise
- Since
- 4.4
Definition at line 543 of file runnermanager.cpp.
void Plasma::RunnerManager::setSingleModeRunnerId | ( | const QString & | id | ) |
Puts the manager into "single runner" mode using the given runner; if the runner does not exist or can not be loaded then the single runner mode will not be started and singleModeRunner() will return NULL.
- Parameters
-
id the id of the runner to use
- Since
- 4.4
Definition at line 527 of file runnermanager.cpp.
|
slot |
Call this method when the runners should be prepared for a query session.
Call matchSessionComplete when the query session is finished for the time being.
- Since
- 4.4
- See also
- matchSessionComplete
Definition at line 662 of file runnermanager.cpp.
bool Plasma::RunnerManager::singleMode | ( | ) | const |
- Returns
- true if the manager is set to run in single runner mode
- Since
- 4.4
Definition at line 538 of file runnermanager.cpp.
QStringList Plasma::RunnerManager::singleModeAdvertisedRunnerIds | ( | ) | const |
- Returns
- the names of all runners that advertise single query mode
- Since
- 4.4
Definition at line 571 of file runnermanager.cpp.
AbstractRunner * Plasma::RunnerManager::singleModeRunner | ( | ) | const |
- Returns
- the currently active "single mode" runner, or null if none
- Since
- 4.4
Definition at line 522 of file runnermanager.cpp.
QString Plasma::RunnerManager::singleModeRunnerId | ( | ) | const |
- Returns
- the id of the runner to use in single mode
- Since
- 4.4
Definition at line 533 of file runnermanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.