Plasma
#include <Plasma/Wallpaper>
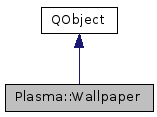
Public Types | |
enum | ResizeMethod { ScaledResize, CenteredResize, ScaledAndCroppedResize, TiledResize, CenterTiledResize, MaxpectResize, LastResizeMethod = MaxpectResize } |
Signals | |
void | configNeedsSaving () |
void | configurationRequired (bool needsConfig) |
void | configureRequested () |
void | renderCompleted (const QImage &image) |
void | renderHintsChanged () |
void | update (const QRectF &exposedArea) |
void | urlDropped (const KUrl &url) |
Public Member Functions | |
Wallpaper (QObject *parent=0) | |
~Wallpaper () | |
QRectF | boundingRect () const |
bool | configurationRequired () const |
QList< QAction * > | contextualActions () const |
virtual QWidget * | createConfigurationInterface (QWidget *parent) |
Q_INVOKABLE DataEngine * | dataEngine (const QString &name) const |
QString | icon () const |
bool | isInitialized () const |
bool | isPreviewing () const |
bool | isUsingRenderingCache () const |
QList< KServiceAction > | listRenderingModes () const |
virtual void | mouseMoveEvent (QGraphicsSceneMouseEvent *event) |
virtual void | mousePressEvent (QGraphicsSceneMouseEvent *event) |
virtual void | mouseReleaseEvent (QGraphicsSceneMouseEvent *event) |
QString | name () const |
bool | needsPreviewDuringConfiguration () const |
const Package * | package () const |
virtual void | paint (QPainter *painter, const QRectF &exposedRect)=0 |
QString | pluginName () const |
KServiceAction | renderingMode () const |
Wallpaper::ResizeMethod | resizeMethodHint () const |
void | restore (const KConfigGroup &config) |
virtual void | save (KConfigGroup &config) |
void | setBoundingRect (const QRectF &boundingRect) |
void | setPreviewing (bool previewing) |
void | setRenderingMode (const QString &mode) |
void | setResizeMethodHint (Wallpaper::ResizeMethod resizeMethod) |
void | setTargetSizeHint (const QSizeF &targetSize) |
void | setUrls (const KUrl::List &urls) |
bool | supportsMimetype (const QString &mimetype) const |
QSizeF | targetSizeHint () const |
virtual void | wheelEvent (QGraphicsSceneWheelEvent *event) |
Static Public Member Functions | |
static KPluginInfo::List | listWallpaperInfo (const QString &formFactor=QString()) |
static KPluginInfo::List | listWallpaperInfoForMimetype (const QString &mimetype, const QString &formFactor=QString()) |
static Wallpaper * | load (const QString &name, const QVariantList &args=QVariantList()) |
static Wallpaper * | load (const KPluginInfo &info, const QVariantList &args=QVariantList()) |
static PackageStructure::Ptr | packageStructure (Wallpaper *paper=0) |
Protected Slots | |
void | addUrls (const KUrl::List &urls) |
Protected Member Functions | |
Wallpaper (QObject *parent, const QVariantList &args) | |
bool | findInCache (const QString &key, QImage &image, unsigned int lastModified=0) |
virtual void | init (const KConfigGroup &config) |
void | insertIntoCache (const QString &key, const QImage &image) |
void | render (const QString &sourceImagePath, const QSize &size, Wallpaper::ResizeMethod resizeMethod=ScaledResize, const QColor &color=QColor(0, 0, 0)) |
void | render (const QImage &image, const QSize &size, Wallpaper::ResizeMethod resizeMethod=ScaledResize, const QColor &color=QColor(0, 0, 0)) |
void | setConfigurationRequired (bool needsConfiguring, const QString &reason=QString()) |
void | setContextualActions (const QList< QAction * > &actions) |
void | setPreviewDuringConfiguration (const bool preview) |
void | setUsingRenderingCache (bool useCache) |
Protected Attributes | |
QList< QAction * > | contextActions |
Properties | |
QRectF | boundingRect |
QString | icon |
QList< KServiceAction > | listRenderingModes |
QString | name |
QString | pluginName |
bool | previewing |
KServiceAction | renderingMode |
ResizeMethod | resizeMethod |
QSizeF | targetSize |
bool | usingRenderingCache |
Detailed Description
The base Wallpaper class.
"Wallpapers" are components that paint the background for Containments that do not provide their own background rendering.
Wallpaper plugins are registered using .desktop files. These files should be named using the following naming scheme:
plasma-wallpaper-\<pluginname\>.desktop
If a wallpaper plugin provides more than on mode (e.g. Single Image, Wallpaper) it should include a Actions= entry in the .desktop file, listing the possible actions. An actions group should be included to provide for translatable names.
Definition at line 56 of file wallpaper.h.
Member Enumeration Documentation
Various resize modes supported by the built in image renderer.
Definition at line 74 of file wallpaper.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor for an empty or null wallpaper.
Definition at line 102 of file wallpaper.cpp.
Plasma::Wallpaper::~Wallpaper | ( | ) |
Definition at line 123 of file wallpaper.cpp.
|
protected |
This constructor is to be used with the plugin loading systems found in KPluginInfo and KService.
The argument list is expected to have one element: the KService service ID for the desktop entry.
- Parameters
-
parent a QObject parent; you probably want to pass in 0 args a list of strings containing one entry: the service id
Definition at line 108 of file wallpaper.cpp.
Member Function Documentation
|
protectedslot |
This method is invoked by setUrls(KUrl::List) Can be Overriden by Plugins which want to support setting Image URLs Will be changed to virtual method in libplasma2/KDE5.
- Since
- 4.7
Definition at line 128 of file wallpaper.cpp.
QRectF Plasma::Wallpaper::boundingRect | ( | ) | const |
Returns bounding rectangle.
|
signal |
Emitted when the configuration of the wallpaper needs to be saved to disk.
- Since
- 4.3
bool Plasma::Wallpaper::configurationRequired | ( | ) | const |
- Returns
- true if the wallpaper currently needs to be configured, otherwise, false
- Since
- 4.3
Definition at line 389 of file wallpaper.cpp.
|
signal |
Emitted when the state of the wallpaper requiring configuration changes.
- Since
- 4.3
|
signal |
Emitted when the user wants to configure/change the wallpaper.
- Since
- 4.3
QList< QAction * > Plasma::Wallpaper::contextualActions | ( | ) | const |
Returns a list of wallpaper contextual actions (nothing by default)
Definition at line 652 of file wallpaper.cpp.
Returns a widget that can be used to configure the options (if any) associated with this wallpaper.
It will be deleted by the caller when it complete. The default implementation returns a null pointer.
To signal that settings have changed connect to settingsChanged(bool modified) in parent
.
Emit settingsChanged(true) when the settings are changed and false when the original state is restored.
Implementation detail note: for best visual results, use a QGridLayout with two columns, with the option labels in column 0
Definition at line 347 of file wallpaper.cpp.
DataEngine * Plasma::Wallpaper::dataEngine | ( | const QString & | name | ) | const |
Loads the given DataEngine.
Tries to load the data engine given by name
. Each engine is only loaded once, and that instance is re-used on all subsequent requests.
If the data engine was not found, an invalid data engine is returned (see DataEngine::isValid()).
Note that you should not delete the returned engine.
- Parameters
-
name Name of the data engine to load
- Returns
- pointer to the data engine if it was loaded, or an invalid data engine if the requested engine could not be loaded
- Since
- 4.3
Definition at line 384 of file wallpaper.cpp.
|
protected |
Tries to load pixmap with the specified key from cache.
- Parameters
-
key the name to use in the cache for this image image the image object to populate with the resulting data if found lastModified if non-zero, the time stamp is also checked on the file, and must be newer than the timestamp to be loaded
- Returns
- true when pixmap was found and loaded from cache, false otherwise
- Since
- 4.3
Definition at line 611 of file wallpaper.cpp.
QString Plasma::Wallpaper::icon | ( | ) | const |
Returns the icon related to this wallpaper.
|
protectedvirtual |
This method is called once the wallpaper is loaded or mode is changed.
The mode can be retrieved using the renderingMode() method.
- Parameters
-
config Config group to load settings
Definition at line 332 of file wallpaper.cpp.
|
protected |
Insert specified pixmap into the cache if usingRenderingCache.
If the cache already contains pixmap with the specified key then it is overwritten.
- Parameters
-
key the name use in the cache for this image; if the image is specific to this wallpaper plugin, consider including the name() as part of the cache key to avoid collisions with other plugins. image the image to store in the cache; passing in a null image will cause the cached image to be removed from the cache
- Since
- 4.3
Definition at line 631 of file wallpaper.cpp.
bool Plasma::Wallpaper::isInitialized | ( | ) | const |
- Returns
- true if initialized (usually by calling restore), false otherwise
Definition at line 288 of file wallpaper.cpp.
bool Plasma::Wallpaper::isPreviewing | ( | ) | const |
- Returns
- true if in preview mode, such as in a configuation dialog
- Since
- 4.5
Definition at line 662 of file wallpaper.cpp.
bool Plasma::Wallpaper::isUsingRenderingCache | ( | ) | const |
QList<KServiceAction> Plasma::Wallpaper::listRenderingModes | ( | ) | const |
Returns modes the wallpaper has, as specified in the .desktop file.
|
static |
Returns a list of all known wallpapers.
- Parameters
-
formFactor the format of the wallpaper being search for (e.g. desktop)
- Returns
- list of wallpapers
Definition at line 151 of file wallpaper.cpp.
|
static |
Returns a list of all known wallpapers that can accept the given mimetype.
- Parameters
-
mimetype the mimetype to search for formFactor the format of the wallpaper being search for (e.g. desktop)
- Returns
- list of wallpapers
Definition at line 162 of file wallpaper.cpp.
|
static |
Attempts to load a wallpaper.
Returns a pointer to the wallpaper if successful. The caller takes responsibility for the wallpaper, including deleting it when no longer needed.
- Parameters
-
name the plugin name, as returned by KPluginInfo::pluginName() args to send the wallpaper extra arguments
- Returns
- a pointer to the loaded wallpaper, or 0 on load failure
Definition at line 180 of file wallpaper.cpp.
|
static |
Attempts to load a wallpaper.
Returns a pointer to the wallpaper if successful. The caller takes responsibility for the wallpaper, including deleting it when no longer needed.
- Parameters
-
info KPluginInfo object for the desired wallpaper args to send the wallpaper extra arguments
- Returns
- a pointer to the loaded wallpaper, or 0 on load failure
Definition at line 220 of file wallpaper.cpp.
|
virtual |
Mouse move event.
To prevent further propagation of the event, the event must be accepted.
- Parameters
-
event the mouse event object
Definition at line 356 of file wallpaper.cpp.
|
virtual |
Mouse press event.
To prevent further propagation of the even, and to receive mouseMoveEvents, the event must be accepted.
- Parameters
-
event the mouse event object
Definition at line 363 of file wallpaper.cpp.
|
virtual |
Mouse release event.
To prevent further propagation of the event, the event must be accepted.
- Parameters
-
event the mouse event object
Definition at line 370 of file wallpaper.cpp.
QString Plasma::Wallpaper::name | ( | ) | const |
Returns the user-visible name for the wallpaper, as specified in the .desktop file.
- Returns
- the user-visible name for the wallpaper.
bool Plasma::Wallpaper::needsPreviewDuringConfiguration | ( | ) | const |
- Returns
- true if the wallpaper needs a live preview in the configuration UI
- Since
- 4.6
Definition at line 672 of file wallpaper.cpp.
const Package * Plasma::Wallpaper::package | ( | ) | const |
Accessor for the associated Package object if any.
- Returns
- the Package object, or 0 if none
Definition at line 682 of file wallpaper.cpp.
|
static |
Returns the Package specialization for wallpapers.
May be queried for 'preferred' which will return the preferred wallpaper image path given the associated Wallpaper object, if any.
- Parameters
-
paper the Wallpaper object to associated the PackageStructure with, which will then use the Wallpaper object to define things such as default size and resize methods.
Definition at line 228 of file wallpaper.cpp.
|
pure virtual |
This method is called when the wallpaper should be painted.
- Parameters
-
painter the QPainter to use to do the painting exposedRect the rect to paint within
QString Plasma::Wallpaper::pluginName | ( | ) | const |
Returns the plugin name for the wallpaper.
|
protected |
Renders the wallpaper asyncronously with the given parameters.
When the rendering is complete, the renderCompleted signal is emitted.
- Parameters
-
sourceImagePath the path to the image file on disk. Common image formats such as PNG, JPEG and SVG are supported size the size to render the image as resizeMethod the method to use when resizing the image to fit size,
- See also
- ResizeMethod
- Parameters
-
color the color to use to pad the rendered image if it doesn't take up the entire size with the given ResizeMethod
- Since
- 4.3
Definition at line 455 of file wallpaper.cpp.
|
protected |
Renders the wallpaper asyncronously with the given parameters.
When the rendering is complete, the renderCompleted signal is emitted.
- Parameters
-
image the raw QImage size the size to render the image as resizeMethod the method to use when resizing the image to fit size,
- See also
- ResizeMethod
- Parameters
-
color the color to use to pad the rendered image if it doesn't take up the entire size with the given ResizeMethod
- Since
- 4.7.4
Definition at line 445 of file wallpaper.cpp.
|
signal |
Emitted when a wallpaper image render is completed.
- Since
- 4.3
|
signal |
KServiceAction Plasma::Wallpaper::renderingMode | ( | ) | const |
- Returns
- the currently active rendering mode
Wallpaper::ResizeMethod Plasma::Wallpaper::resizeMethodHint | ( | ) | const |
void Plasma::Wallpaper::restore | ( | const KConfigGroup & | config | ) |
This method should be called once the wallpaper is loaded or mode is changed.
- Parameters
-
config Config group to load settings
- See also
- init
Definition at line 322 of file wallpaper.cpp.
|
virtual |
This method is called when settings need to be saved.
- Parameters
-
config Config group to save settings
Definition at line 340 of file wallpaper.cpp.
void Plasma::Wallpaper::setBoundingRect | ( | const QRectF & | boundingRect | ) |
Sets bounding rectangle.
Definition at line 293 of file wallpaper.cpp.
|
protected |
When the wallpaper needs to be configured before being usable, this method can be called to denote that action is required.
- Parameters
-
needsConfiguring true if the applet needs to be configured, or false if it doesn't reason a translated message for the user explaining that the applet needs configuring; this should note what needs to be configured
- Since
- 4.3
Definition at line 394 of file wallpaper.cpp.
|
protected |
Sets the contextual actions for this wallpaper.
- Parameters
-
actions A list of contextual actions for this wallpaper
- Since
- 4.4
Definition at line 657 of file wallpaper.cpp.
|
protected |
Sets whether the configuration user interface of the wallpaper should have a live preview rendered by a Wallpaper instance.
note: this is just an hint, the configuration user interface can choose to ignore it
- Parameters
-
preview true if a preview should be shown
- Since
- 4.6
Definition at line 677 of file wallpaper.cpp.
void Plasma::Wallpaper::setPreviewing | ( | bool | previewing | ) |
void Plasma::Wallpaper::setRenderingMode | ( | const QString & | mode | ) |
Sets the rendering mode for this wallpaper.
- Parameters
-
mode One of the modes supported by the plugin, or an empty string for the default mode.
Definition at line 303 of file wallpaper.cpp.
void Plasma::Wallpaper::setResizeMethodHint | ( | Wallpaper::ResizeMethod | resizeMethod | ) |
Allows one to set rendering hints that may differ from the actualities of the Wallpaper's current state, allowing for better selection of papers from packages, for instance.
- Parameters
-
resizeMethod The resize method to assume will be used for future wallpaper scaling; may later be changed by calls to render()
- Since
- 4.3
Definition at line 418 of file wallpaper.cpp.
void Plasma::Wallpaper::setTargetSizeHint | ( | const QSizeF & | targetSize | ) |
Allows one to set rendering hints that may differ from the actualities of the Wallpaper's current state, allowing for better selection of papers from packages, for instance.
- Parameters
-
targetSize The size to assume will be used for future wallpaper scaling
- Since
- 4.3
Definition at line 432 of file wallpaper.cpp.
void Plasma::Wallpaper::setUrls | ( | const KUrl::List & | urls | ) |
Sets the urls for the wallpaper.
- Parameters
-
urls Urls of the selected images
- Since
- 4.7
Definition at line 140 of file wallpaper.cpp.
|
protected |
Sets whether or not to cache on disk the results of calls to render.
If the wallpaper changes often or is innexpensive to render, then it's probably best not to cache them.
The default is not to cache.
- See also
- render
- Parameters
-
useCache true to cache rendered papers on disk, false not to cache
- Since
- 4.3
Definition at line 413 of file wallpaper.cpp.
bool Plasma::Wallpaper::supportsMimetype | ( | const QString & | mimetype | ) | const |
- Returns
- true if the mimetype is supported by this wallpaper and can be used in renderering. Uses the MimeType= entry from the .desktop file, and can include mimetypes that may not be suitable for drag-and-drop purposes.
- Since
- 4.7
Definition at line 174 of file wallpaper.cpp.
QSizeF Plasma::Wallpaper::targetSizeHint | ( | ) | const |
|
signal |
This signal indicates that wallpaper needs to be repainted.
|
signal |
Emitted when a URL matching X-Plasma-DropMimeTypes is dropped on the wallpaper.
- Parameters
-
url the URL of the dropped file
- Since
- 4.4
|
virtual |
Mouse wheel event.
To prevent further propagation of the event, the event must be accepted.
- Parameters
-
event the wheel event object
Definition at line 377 of file wallpaper.cpp.
Member Data Documentation
|
protected |
Definition at line 555 of file wallpaper.h.
Property Documentation
|
readwrite |
Definition at line 59 of file wallpaper.h.
|
read |
Definition at line 62 of file wallpaper.h.
|
read |
Definition at line 64 of file wallpaper.h.
|
read |
Definition at line 60 of file wallpaper.h.
|
read |
Definition at line 61 of file wallpaper.h.
|
readwrite |
Definition at line 66 of file wallpaper.h.
|
read |
Definition at line 63 of file wallpaper.h.
|
readwrite |
Definition at line 67 of file wallpaper.h.
|
readwrite |
Definition at line 68 of file wallpaper.h.
|
readwrite |
Definition at line 65 of file wallpaper.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.