ThreadWeaver
#include <Job.h>
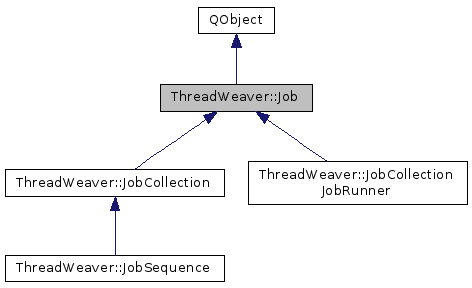
Signals | |
void | done (ThreadWeaver::Job *) |
void | failed (ThreadWeaver::Job *) |
void | started (ThreadWeaver::Job *) |
Public Member Functions | |
Job (QObject *parent=0) | |
virtual | ~Job () |
virtual void | aboutToBeDequeued (WeaverInterface *weaver) |
virtual void | aboutToBeQueued (WeaverInterface *weaver) |
void | assignQueuePolicy (QueuePolicy *) |
virtual bool | canBeExecuted () |
virtual void | execute (Thread *) |
bool | isFinished () const |
virtual int | priority () const |
void | removeQueuePolicy (QueuePolicy *) |
virtual void | requestAbort () |
virtual bool | success () const |
Protected Member Functions | |
void | freeQueuePolicyResources () |
virtual void | run ()=0 |
void | setFinished (bool status) |
Thread * | thread () |
Protected Attributes | |
Private * | d |
Detailed Description
A Job is a simple abstraction of an action that is to be executed in a thread context.
It is essential for the ThreadWeaver library that as a kind of convention, the different creators of Job objects do not touch the protected data members of the Job until somehow notified by the Job.
Also, please note that Jobs may not be executed twice. Create two different objects to perform two consecutive or parallel runs.
Jobs may declare dependencies. If Job B depends on Job A, B may not be executed before A is finished. To learn about dependencies, see DependencyPolicy.
Job objects finish by emitting the done(Job*) signal. Once this has been emitted, ThreadWeaver is no longer using the Job which may then be deleted.
Constructor & Destructor Documentation
|
explicit |
Member Function Documentation
|
virtual |
This Job is about the be dequeued from the weaver's job queue.
The job will be removed from the queue right after this method returns. Use this method to dequeue, if necessary, sub-operations (jobs) that this job has enqueued.
Note: When this method is called, the associated Weaver object's thread does hold a lock on the weaver's queue.
Note: The default implementation does nothing.
- Parameters
-
weaver the Weaver object from which the job will be dequeued
Reimplemented in ThreadWeaver::JobCollection, and ThreadWeaver::JobCollectionJobRunner.
|
virtual |
The job is about to be added to the weaver's job queue.
The job will be added right after this method finished. The default implementation does nothing. Use this method to, for example, queue sub-operations as jobs before the job itself is queued.
Note: When this method is called, the associated Weaver object's thread holds a lock on the weaver's queue. Therefore, it is save to assume that recursive queueing is atomic from the queues perspective.
- Parameters
-
weaver the Weaver object the job will be queued in
Reimplemented in ThreadWeaver::JobCollection, ThreadWeaver::JobCollectionJobRunner, and ThreadWeaver::JobSequence.
void Job::assignQueuePolicy | ( | QueuePolicy * | policy | ) |
Assign a queue policy.
Queue Policies customize the queueing (running) behaviour of sets of jobs. Examples for queue policies are dependencies and resource restrictions. Every queue policy object can only be assigned once to a job, multiple assignments will be IGNORED.
|
virtual |
canBeExecuted() returns true if all the jobs queue policies agree to it.
If it returns true, it expects that the job is executed right after that. The done() methods of the queue policies will be automatically called when the job is finished.
If it returns false, all queue policy resources have been freed, and the method can be called again at a later time.
Reimplemented in ThreadWeaver::JobCollection, and ThreadWeaver::JobCollectionJobRunner.
|
signal |
This signal is emitted when the job has been finished (no matter if it succeeded or not).
After this signal has been emitted, ThreadWeaver no longer references the Job internally and the Job can be deleted.
|
virtual |
Perform the job.
The thread in which this job is executed is given as a parameter. Do not overload this method to create your own Job implementation, overload run().
Reimplemented in ThreadWeaver::JobCollectionJobRunner.
|
signal |
This job has failed.
This signal is emitted when success() returns false after the job is executed.
|
protected |
bool Job::isFinished | ( | ) | const |
|
virtual |
The queueing priority of the job.
Jobs will be sorted by their queueing priority when enqueued. A higher queueing priority will place the job in front of all lower-priority jobs in the queue.
Note: A higher or lower priority does not influence queue policies. For example, a high-priority job that has an unresolved dependency will not be executed, which means an available lower-priority job will take precedence.
The default implementation returns zero. Only if this method is overloaded for some job classes, priorities will influence the execution order of jobs.
Reimplemented in ThreadWeaver::JobCollectionJobRunner.
void Job::removeQueuePolicy | ( | QueuePolicy * | policy | ) |
|
inlinevirtual |
Abort the execution of the job.
Call this method to ask the Job to abort if it is currently executed. Please note that the default implementation of the method does nothing (!). This is due to the fact that there is no generic method to abort a processing Job. Not even a default boolean flag makes sense, as Job could, for example, be in an event loop and will need to create an exit event. You have to reimplement the method to actually initiate an abort action. The method is not pure virtual because users are not supposed to be forced to always implement requestAbort(). Also, this method is supposed to return immediately, not after the abort has completed. It requests the abort, the Job has to act on the request.
|
protectedpure virtual |
The method that actually performs the job.
It is called from execute(). This method is the one to overload it with the job's task.
|
protected |
|
signal |
This signal is emitted when this job is being processed by a thread.
|
virtual |
Return whether the Job finished successfully or not.
The default implementation simply returns true. Overload in derived classes if the derived Job class can fail.
If a job fails (success() returns false), it will NOT resolve its dependencies when it finishes. This will make sure that Jobs that depend on the failed job will not be started.
There is an important gotcha: When a Job object is deleted, it will always resolve its dependencies. If dependent jobs should not be executed after a failure, it is important to dequeue those before deleting the failed Job.
A JobSequence may be helpful for that purpose.
|
protected |
Member Data Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.