ThreadWeaver
#include <ThreadWeaver.h>
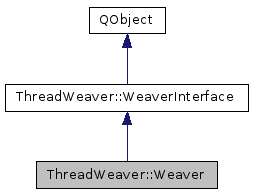
Public Member Functions | |
Weaver (QObject *parent=0) | |
virtual | ~Weaver () |
int | currentNumberOfThreads () const |
virtual bool | dequeue (Job *) |
virtual void | dequeue () |
virtual void | enqueue (Job *) |
virtual void | finish () |
bool | isEmpty () const |
bool | isIdle () const |
int | maximumNumberOfThreads () const |
int | queueLength () const |
void | registerObserver (WeaverObserver *) |
void | requestAbort () |
virtual void | resume () |
void | setMaximumNumberOfThreads (int cap) |
const State & | state () const |
virtual void | suspend () |
![]() | |
WeaverInterface (QObject *parent=0) | |
virtual | ~WeaverInterface () |
Static Public Member Functions | |
static ThreadWeaver::Weaver * | instance () |
Protected Member Functions | |
virtual WeaverInterface * | makeWeaverImpl () |
Additional Inherited Members | |
![]() | |
void | finished () |
void | jobDone (ThreadWeaver::Job *) |
void | stateChanged (ThreadWeaver::State *) |
void | suspended () |
Detailed Description
The Weaver class provides the public implementation of the WeaverInterface.
Weaver provides a static instance that can be used to perform jobs in threads without managing a weaver object. The static instance will only be created when it is first accessed. Also, Weaver objects will create the threads only when the first jobs are queued. Therefore, the creation of a Weaver object is a rather cheap operation.
The WeaverImpl class provides two parts of API - one for the threads that are handled by it, and one for the ThreadWeaver users (application developers). To separate those two different API parts, Weaver only provides the interface supposed to be used by developers of multithreaded applications.
Weaver creates and destroys WeaverImpl objects. It hides the implementation details of the WeaverImpl class. It is strongly discouraged to use the WeaverImpl class in programs, as its API will be changed without notice. Also, Weaver provides a factory method for this purpose that can be overloaded to create derived WeaverImpl objects.
Definition at line 65 of file ThreadWeaver.h.
Constructor & Destructor Documentation
|
explicit |
Construct a Weaver object.
Definition at line 48 of file ThreadWeaver.cpp.
|
virtual |
Destruct a Weaver object.
Definition at line 59 of file ThreadWeaver.cpp.
Member Function Documentation
|
virtual |
Returns the current number of threads in the inventory.
Implements ThreadWeaver::WeaverInterface.
Definition at line 147 of file ThreadWeaver.cpp.
|
virtual |
Remove a job from the queue.
If the job was queued but not started so far, it is simply removed from the queue. For now, it is unsupported to dequeue a job once its execution has started.
For that case, you will have to provide a method to interrupt your job's execution (and receive the done signal). Returns true if the job has been dequeued, false if the job has already been started or is not found in the queue.
Implements ThreadWeaver::WeaverInterface.
Definition at line 102 of file ThreadWeaver.cpp.
|
virtual |
Remove all queued jobs.
Please note that this will not kill the threads, therefore all jobs that are being processed will be continued.
Implements ThreadWeaver::WeaverInterface.
Definition at line 107 of file ThreadWeaver.cpp.
|
virtual |
Add a job to be executed.
It depends on the state if execution of the job will be attempted immediately. In suspended state, jobs can be added to the queue, but the threads remain suspended. In WorkongHard state, an idle thread may immediately execute the job, or it might be queued if all threads are busy.
Implements ThreadWeaver::WeaverInterface.
Definition at line 97 of file ThreadWeaver.cpp.
|
virtual |
Finish all queued operations, then return.
This method is used in imperative (not event driven) programs that cannot react on events to have the controlling (main) thread wait wait for the jobs to finish. The call will block the calling thread and return when all queued jobs have been processed.
Warning: This will suspend your thread! Warning: If one of your jobs enters an infinite loop, this will never return!
Implements ThreadWeaver::WeaverInterface.
Definition at line 112 of file ThreadWeaver.cpp.
|
static |
Return the global Weaver instance.
In some cases, a global Weaver object per application is sufficient for the applications purpose. If this is the case, query instance() to get a pointer to a global instance. If instance is never called, a global Weaver object will not be created.
The application-global Weaver instance.
This instance will only be created if this method is actually called in the lifetime of the application.
Definition at line 80 of file ThreadWeaver.cpp.
|
virtual |
Is the queue empty? The queue is empty if no more jobs are queued.
Implements ThreadWeaver::WeaverInterface.
Definition at line 127 of file ThreadWeaver.cpp.
|
virtual |
Is the weaver idle? The weaver is idle if no jobs are queued and no jobs are processed by the threads.
Implements ThreadWeaver::WeaverInterface.
Definition at line 132 of file ThreadWeaver.cpp.
|
protectedvirtual |
The factory method to create the actual Weaver implementation.
Overload this method to use a different or adapted implementation.
Definition at line 65 of file ThreadWeaver.cpp.
|
virtual |
Get the maximum number of threads this Weaver may start.
Implements ThreadWeaver::WeaverInterface.
Definition at line 152 of file ThreadWeaver.cpp.
|
virtual |
Returns the number of pending jobs.
This will return the number of queued jobs. Jobs that are currently being executed are not part of the queue. All jobs in the queue are waiting to be executed.
Implements ThreadWeaver::WeaverInterface.
Definition at line 137 of file ThreadWeaver.cpp.
|
virtual |
Register an observer.
Observers provides signals on different weaver events that are otherwise only available through objects of different classes (threads, jobs). Usually, access to the signals of those objects is not provided through the weaver API. Use an observer to reveice notice, for example, on thread activity.
To unregister, simply delete the observer.
Implements ThreadWeaver::WeaverInterface.
Definition at line 75 of file ThreadWeaver.cpp.
|
virtual |
Request aborts of the currently executed jobs.
It is important to understand that aborts are requested, but cannot be guaranteed, as not all Job classes support it. It is up to the application to decide if and how job aborts are necessary.
Implements ThreadWeaver::WeaverInterface.
Definition at line 157 of file ThreadWeaver.cpp.
|
virtual |
Resume job queueing.
- See also
- suspend
Implements ThreadWeaver::WeaverInterface.
Definition at line 122 of file ThreadWeaver.cpp.
|
virtual |
Set the maximum number of threads this Weaver object may start.
Implements ThreadWeaver::WeaverInterface.
Definition at line 142 of file ThreadWeaver.cpp.
|
virtual |
Return the state of the weaver object.
Implements ThreadWeaver::WeaverInterface.
Definition at line 70 of file ThreadWeaver.cpp.
|
virtual |
Suspend job execution.
When suspending, all threads are allowed to finish the currently assigned job but will not receive a new assignment. When all threads are done processing the assigned job, the signal suspended will() be emitted. If you call suspend() and there are no jobs left to be done, you will immediately receive the suspended() signal.
Implements ThreadWeaver::WeaverInterface.
Definition at line 117 of file ThreadWeaver.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:53 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.