kget
#include <scheduler.h>
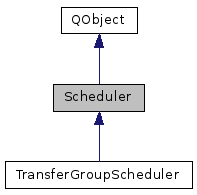
Classes | |
class | JobFailure |
Public Types | |
enum | FailureStatus { None = 0, AboutToStall = 1, Stall = 2, StallTimeout = 3, Abort = 4, AbortTimeout = 5, Error = 6 } |
Public Member Functions | |
Scheduler (QObject *parent=0) | |
~Scheduler () | |
void | addQueue (JobQueue *queue) |
int | countRunningJobs () const |
void | delQueue (JobQueue *queue) |
bool | hasRunningJobs () const |
virtual void | jobChangedEvent (Job *job, Job::Status status) |
virtual void | jobChangedEvent (Job *job, Job::Policy status) |
virtual void | jobChangedEvent (Job *job, JobFailure failure) |
virtual void | jobQueueAddedJobEvent (JobQueue *queue, Job *job) |
virtual void | jobQueueAddedJobsEvent (JobQueue *queue, const QList< Job * > jobs) |
virtual void | jobQueueChangedEvent (JobQueue *queue, JobQueue::Status status) |
virtual void | jobQueueMovedJobEvent (JobQueue *queue, Job *job) |
virtual void | jobQueueRemovedJobEvent (JobQueue *queue, Job *job) |
virtual void | jobQueueRemovedJobsEvent (JobQueue *queue, const QList< Job * > jobs) |
void | setHasNetworkConnection (bool hasConnection) |
void | setIsSuspended (bool isSuspended) |
void | settingsChanged () |
void | start () |
void | stop () |
Protected Member Functions | |
bool | shouldBeRunning (Job *job) |
void | updateQueue (JobQueue *queue) |
Detailed Description
Scheduler class: what handle all the jobs in kget.
This class handles all the jobs in kget. See job.h for further details. When we want a job to be executed in kget, we have to add the queue that owns the job in the scheduler calling the addQueue(JobQueue *) function.
Definition at line 32 of file scheduler.h.
Member Enumeration Documentation
Enumerator | |
---|---|
None | |
AboutToStall | |
Stall | |
StallTimeout | |
Abort | |
AbortTimeout | |
Error |
Definition at line 40 of file scheduler.h.
Constructor & Destructor Documentation
Scheduler::Scheduler | ( | QObject * | parent = 0 | ) |
Definition at line 22 of file scheduler.cpp.
Scheduler::~Scheduler | ( | ) |
Definition at line 34 of file scheduler.cpp.
Member Function Documentation
void Scheduler::addQueue | ( | JobQueue * | queue | ) |
Adds a queue to the scheduler.
- Parameters
-
queue The queue that should be added
Definition at line 73 of file scheduler.cpp.
int Scheduler::countRunningJobs | ( | ) | const |
- Returns
- the number of jobs that are currently in a Running state
Definition at line 99 of file scheduler.cpp.
void Scheduler::delQueue | ( | JobQueue * | queue | ) |
Deletes a queue from the scheduler.
If some jobs in the given queue are being executed, they are first stopped, then removed from the scheduler.
- Parameters
-
queue The queue that should be removed
Definition at line 79 of file scheduler.cpp.
bool Scheduler::hasRunningJobs | ( | ) | const |
- Returns
- true if there is at least one Job in the Running state
Definition at line 89 of file scheduler.cpp.
|
virtual |
Definition at line 170 of file scheduler.cpp.
|
virtual |
Definition at line 181 of file scheduler.cpp.
|
virtual |
Definition at line 188 of file scheduler.cpp.
Definition at line 141 of file scheduler.cpp.
Definition at line 148 of file scheduler.cpp.
|
virtual |
Definition at line 117 of file scheduler.cpp.
Definition at line 134 of file scheduler.cpp.
Definition at line 156 of file scheduler.cpp.
Definition at line 163 of file scheduler.cpp.
void Scheduler::setHasNetworkConnection | ( | bool | hasConnection | ) |
The JobQueues will be informed of changes in the network connection If there is no network connection then the Scheduler won't act on the timerEvent or updateQueue.
Definition at line 50 of file scheduler.cpp.
void Scheduler::setIsSuspended | ( | bool | isSuspended | ) |
Can be used to suspend the scheduler before doing lenghty operations and activating it later again.
NOTE does not stop running jobs, just prevents changes to jobs HACK this is needed since the scheduler would constantly update the queue when stopping starting multiple transfers, this slows down that operation a lot and could result in transfers finishing before they are stopped etc.
Definition at line 39 of file scheduler.cpp.
void Scheduler::settingsChanged | ( | ) |
This function gets called by the KGet class whenever the settings have changed.
Definition at line 109 of file scheduler.cpp.
|
protected |
- Returns
- true if the given job should be running (and this depends on the job policy and on its jobQueue status)
- Parameters
-
job the job to evaluate
Definition at line 329 of file scheduler.cpp.
void Scheduler::start | ( | ) |
Starts globally the execution of the jobs.
- See also
- stop()
Definition at line 234 of file scheduler.cpp.
void Scheduler::stop | ( | ) |
Stops globally the execution of the jobs.
- See also
- start()
Definition at line 239 of file scheduler.cpp.
|
protected |
Updates the given queue, starting the jobs that come first in the queue and stopping all the other.
- Parameters
-
queue the queue to update
Implemented behaviour
The scheduler allows a maximum number of runningJobs equal to the queue->maxSimultaneousJobs() setting. If that number is not reached because of stallTimeout transfers, the scheduler allows that: (runningJobs + waitingJobs) < 2 * queue->maxSimultaneousJobs() Examples (with maxSimultaneousJobs = 2): These are if the running jobs come first in the queue 1) 2 runningJobs - 0 waitingJobs 2) 1 runningJobs - up to 3 waitingJobs 3) 0 runningJobs - up to 4 waitingJobs These are if the waiting jobs come first in the queue 1) 1 waitingJobs - 2 runningJobs 2) 2 waitingJobs - 2 runningJobs 3) 3 waitingJobs - 1 runningJobs 4) 4 waitingJobs - 0 runningJobs
Definition at line 244 of file scheduler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.