kget
#include <kget.h>
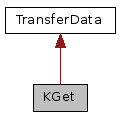
Public Types | |
enum | AfterFinishAction { Quit = 0, Shutdown = 1, Hibernate = 2, Suspend = 3 } |
enum | DeleteMode { AutoDelete, DeleteFiles } |
Public Member Functions | |
~KGet () | |
TransferData (const KUrl &src, const KUrl &dest, const QString &groupName=QString(), bool start=false, const QDomElement *e=0) | |
Static Public Member Functions | |
static KActionCollection * | actionCollection () |
static bool | addGroup (const QString &groupName) |
static TransferHandler * | addTransfer (KUrl srcUrl, QString destDir=QString(), QString suggestedFileName=QString(), QString groupName=QString(), bool start=false) |
static const QList < TransferHandler * > | addTransfer (KUrl::List srcUrls, QString destDir=QString(), QString groupName=QString(), bool start=false) |
static QList< TransferHandler * > | addTransfers (const QList< QDomElement > &elements, const QString &groupName=QString()) |
static QList < TransferGroupHandler * > | allTransferGroups () |
static QList< TransferHandler * > | allTransfers () |
static void | calculateGlobalDownloadLimit () |
static void | calculateGlobalSpeedLimits () |
static void | calculateGlobalUploadLimit () |
static void | checkSystemTray () |
static TransferDataSource * | createTransferDataSource (const KUrl &src, const QDomElement &type=QDomElement(), QObject *parent=0) |
static void | delGroup (TransferGroupHandler *group, bool askUser=true) |
static void | delGroups (QList< TransferGroupHandler * > groups, bool askUser=true) |
static bool | delTransfer (TransferHandler *transfer, DeleteMode mode=AutoDelete) |
static bool | delTransfers (const QList< TransferHandler * > &transfers, DeleteMode mode=AutoDelete) |
static QList< TransferFactory * > | factories () |
static TransferFactory * | factory (TransferHandler *transfer) |
static TransferGroupHandler * | findGroup (const QString &name) |
static TransferHandler * | findTransfer (const KUrl &src) |
static QList< TransferHandler * > | finishedTransfers () |
static QString | generalDestDir (bool preferXDGDownloadDir=false) |
static QList < TransferGroupHandler * > | groupsFromExceptions (const KUrl &filename) |
static void | load (QString filename=QString()) |
static void | loadPlugins () |
static bool | matchesExceptions (const KUrl &sourceUrl, const QStringList &patterns) |
static TransferTreeModel * | model () |
static void | moveTransfer (TransferHandler *transfer, const QString &groupName) |
static void | redownloadTransfer (TransferHandler *transfer) |
static void | renameGroup (const QString &oldName, const QString &newName) |
static void | save (QString filename=QString(), bool plain=false) |
static bool | schedulerRunning () |
static QList < TransferGroupHandler * > | selectedTransferGroups () |
static QList< TransferHandler * > | selectedTransfers () |
static TransferTreeSelectionModel * | selectionModel () |
static KGet * | self (MainWindow *mainWindow=0) |
static void | setGlobalDownloadLimit (int limit) |
static void | setGlobalUploadLimit (int limit) |
static void | setSchedulerRunning (bool running=true) |
static void | setSuspendScheduler (bool isSuspended) |
static void | settingsChanged () |
static KNotification * | showNotification (QWidget *parent, const QString &eventType, const QString &text, const QString &icon=QString("dialog-error"), const QString &title=i18n("KGet"), const KNotification::NotificationFlags &flags=KNotification::CloseOnTimeout) |
static QStringList | transferGroupNames () |
Public Attributes | |
KUrl | dest |
const QDomElement * | e |
QString | groupName |
KUrl | src |
bool | start |
Detailed Description
This is our KGet class.
This is where the user's transfers and searches are stored and organized. Use this class from the views to add or remove transfers or searches In order to organize the transfers inside categories we have a TransferGroup class. By definition, a transfer must always belong to a TransferGroup. If we don't want it to be displayed by the gui inside a specific group, we will put it in the group named "Not grouped" (better name?).
Member Enumeration Documentation
enum KGet::DeleteMode |
Constructor & Destructor Documentation
Member Function Documentation
|
static |
|
static |
|
static |
Adds a new transfer to the KGet.
- Parameters
-
srcUrl The url to be downloaded destDir The destination directory. If empty we show a dialog where the user can choose it. suggestedFileName a suggestion of a simple filename to be saved in destDir groupName The name of the group the new transfer will belong to start Specifies if the newly added transfers should be started. If the group queue is already in a running state, this flag does nothing
|
static |
Adds new transfers to the KGet.
- Parameters
-
srcUrls The urls to be downloaded destDir The destination directory. If empty we show a dialog where the user can choose it. groupName The name of the group the new transfer will belong to start Specifies if the newly added transfers should be started. If the group queue is already in a running state, this flag does nothing
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
Scans for all the available plugins and creates the proper transfer DataSource object for transfers Containers.
- Parameters
-
src Source Url type the type of the DataSource that should be created e.g. <TransferDataSource type="search"> this is only needed when creating a "special" TransferDataSource like the search for Urls you can set additional information and the TransferDataSource will use it if it can
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
Returns a download directory.
- Parameters
-
preferXDGDownloadDir if true the XDG_DOWNLOAD_DIR will be taken if it is not empty
- Note
- depending if the directories exist it will return them in the following order: (preferXDGDownloadDirectory >) lastDirectory > XDG_DOWNLOAD_DIR
|
static |
|
static |
|
static |
|
static |
- Returns
- a pointer to the TransferTreeModel object
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
|
static |
- Returns
- a pointer to the QItemSelectionModel object
|
static |
This is our KGet class.
This is where the user's transfers and searches are stored and organized. Use this class from the views to add or remove transfers or searches In order to organize the transfers inside categories we have a TransferGroup class. By definition, a transfer must always belong to a TransferGroup. If we don't want it to be displayed by the gui inside a specific group, we will put it in the group named "Not grouped" (better name?).
|
static |
|
static |
|
static |
|
static |
true suspends the scheduler, any events that would result in a reschedule are ignored false wakes up the scheduler, events result in reschedule again NOTE this is a HACK for cases where the scheduler is the bottleneck, e.g.
when stopping a lot of running transfers, or starting a lot transfers
|
static |
|
static |
KGet::TransferData | ( | const KUrl & | src, |
const KUrl & | dest, | ||
const QString & | groupName = QString() , |
||
bool | start = false , |
||
const QDomElement * | e = 0 |
||
) |
|
static |
Member Data Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.