kget
#include <transferhandler.h>
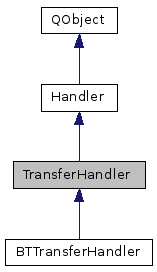
Public Types | |
typedef Transfer::ChangesFlags | ChangesFlags |
Public Slots | |
virtual void | start () |
virtual void | stop () |
Signals | |
void | capabilitiesChanged () |
void | transferChangedEvent (TransferHandler *transfer, TransferHandler::ChangesFlags flags) |
Public Member Functions | |
TransferHandler (Transfer *parent, Scheduler *scheduler) | |
virtual | ~TransferHandler () |
QHash< KUrl, QPair< bool, int > > | availableMirrors (const KUrl &file) const |
int | averageDownloadSped () const |
Transfer::Capabilities | capabilities () const |
ChangesFlags | changesFlags () const |
void | checkShareRatio () |
int | columnCount () const |
QList< QAction * > | contextActions () |
QVariant | data (int column) |
QString | dBusObjectPath () |
const KUrl & | dest () const |
KUrl | directory () const |
KIO::filesize_t | downloadedSize () const |
int | downloadLimit (Transfer::SpeedLimit limit) const |
int | downloadSpeed () const |
int | elapsedTime () const |
Job::Error | error () const |
QList< QAction * > | factoryActions () |
virtual FileModel * | fileModel () |
QList< KUrl > | files () const |
TransferGroupHandler * | group () const |
bool | isSelected () const |
KGetKJobAdapter * | kJobAdapter () |
double | maximumShareRatio () |
int | percent () const |
int | remainingTime () const |
bool | repair (const KUrl &file=KUrl()) |
void | resolveError (int errorId) |
void | setAvailableMirrors (const KUrl &file, const QHash< KUrl, QPair< bool, int > > &mirrors) |
bool | setDirectory (const KUrl &newDirectory) |
void | setDownloadLimit (int dlLimit, Transfer::SpeedLimit limit) |
void | setMaximumShareRatio (double ratio) |
void | setSelected (bool select) |
void | setUploadLimit (int ulLimit, Transfer::SpeedLimit limit) |
virtual Signature * | signature (const KUrl &file) |
const KUrl & | source () const |
Job::Status | startStatus () const |
Job::Status | status () const |
QPixmap | statusPixmap () const |
QString | statusText () const |
KIO::filesize_t | totalSize () const |
KIO::filesize_t | uploadedSize () const |
int | uploadLimit (Transfer::SpeedLimit limit) const |
int | uploadSpeed () const |
virtual Verifier * | verifier (const KUrl &file) |
![]() | |
Handler (Scheduler *scheduler, QObject *parent) | |
virtual | ~Handler () |
virtual void | start ()=0 |
virtual void | stop ()=0 |
Additional Inherited Members | |
![]() | |
Scheduler * | m_scheduler |
Detailed Description
Class TransferHandler:
— Overview — This class is the representation of a Transfer object from the views' perspective (proxy pattern). In fact the views never handle directly the Transfer objects themselves (because this would break the model/view policy). As a general rule, all the code strictly related to the views should placed here (and not in the transfer implementation). Here we provide the same api available in the transfer class, but we change the implementation of some methods. Let's give an example about this: The start() function of a specific Transfer (let's say TransferKio) is a low level command that really makes the transfer start and should therefore be executed only by the scheduler. The start() function in this class is implemented in another way, since it requests the scheduler to execute the start command to this specific transfer. At this point the scheduler will evaluate this request and execute, if possible, the start() function directly in the TransferKio.
Definition at line 48 of file transferhandler.h.
Member Typedef Documentation
Definition at line 58 of file transferhandler.h.
Constructor & Destructor Documentation
Definition at line 29 of file transferhandler.cpp.
|
virtual |
Definition at line 42 of file transferhandler.cpp.
Member Function Documentation
|
inline |
The mirrors that are available bool if it is used, int how many paralell connections are allowed to the mirror.
- Parameters
-
file the file for which the availableMirrors should be get
Definition at line 123 of file transferhandler.h.
int TransferHandler::averageDownloadSped | ( | ) | const |
- Returns
- the average download speed of the transfer in bytes/sec
Definition at line 111 of file transferhandler.cpp.
Transfer::Capabilities TransferHandler::capabilities | ( | ) | const |
Returns the capabilities the Transfer supports.
Definition at line 46 of file transferhandler.cpp.
|
signal |
Emitted when the capabilities of the Transfer change.
Transfer::ChangesFlags TransferHandler::changesFlags | ( | ) | const |
Returns the changes flags.
Definition at line 175 of file transferhandler.cpp.
|
inline |
Recalculate the share ratio.
Definition at line 205 of file transferhandler.h.
|
inline |
- Returns
- the number of columns associated to the transfer's data
Definition at line 226 of file transferhandler.h.
QList< QAction * > TransferHandler::contextActions | ( | ) |
- Returns
- a list of a actions, which are associated with this TransferHandler
Definition at line 205 of file transferhandler.cpp.
|
virtual |
- Returns
- the data associated to this Transfer item. This is necessary to make the interview model/view work
Implements Handler.
Definition at line 121 of file transferhandler.cpp.
|
inline |
- Returns
- the object path that will be shown in the DBUS interface
Definition at line 262 of file transferhandler.h.
|
inline |
- Returns
- the dest url
Definition at line 98 of file transferhandler.h.
|
inline |
- Returns
- the directory the Transfer will be stored to
Definition at line 108 of file transferhandler.h.
KIO::filesize_t TransferHandler::downloadedSize | ( | ) | const |
- Returns
- the downloaded size of the transfer in bytes
Definition at line 91 of file transferhandler.cpp.
|
inline |
- Returns
- the download Limit of the transfer in KiB
Definition at line 189 of file transferhandler.h.
int TransferHandler::downloadSpeed | ( | ) | const |
- Returns
- the download speed of the transfer in bytes/sec
Definition at line 106 of file transferhandler.cpp.
int TransferHandler::elapsedTime | ( | ) | const |
Definition at line 76 of file transferhandler.cpp.
|
inline |
Definition at line 65 of file transferhandler.h.
QList< QAction * > TransferHandler::factoryActions | ( | ) |
- Returns
- a list of the transfer's factory's actions
Definition at line 220 of file transferhandler.cpp.
|
inlinevirtual |
- Returns
- a pointer to the FileModel containing all files of this download
Definition at line 272 of file transferhandler.h.
|
inline |
- Returns
- all files of this transfer
Definition at line 103 of file transferhandler.h.
|
inline |
- Returns
- the transfer's group handler
Definition at line 88 of file transferhandler.h.
bool TransferHandler::isSelected | ( | ) | const |
- Returns
- true if the current transfer is selected
- false otherwise
Definition at line 170 of file transferhandler.cpp.
|
inline |
- Returns
- the kJobAdapter object
Definition at line 267 of file transferhandler.h.
|
inline |
- Returns
- the maximum share-ratio
Definition at line 200 of file transferhandler.h.
int TransferHandler::percent | ( | ) | const |
- Returns
- the progress percentage of the transfer
Definition at line 101 of file transferhandler.cpp.
int TransferHandler::remainingTime | ( | ) | const |
Definition at line 81 of file transferhandler.cpp.
|
inline |
Tries to repair file.
- Parameters
-
file the file of a download that should be repaired, if not defined all files of a download are going to be repaird
- Returns
- true if a repair started, false if it was not nescessary
Definition at line 83 of file transferhandler.h.
|
inline |
Definition at line 70 of file transferhandler.h.
|
inline |
Set the mirrors, int the number of paralell connections to the mirror bool if the mirror should be used.
- Parameters
-
file the file for which the availableMirrors should be set
Definition at line 130 of file transferhandler.h.
|
inline |
Move the download to the new destination.
- Parameters
-
newDirectory is a directory where the download should be stored
- Returns
- true if newDestination can be used
Definition at line 115 of file transferhandler.h.
|
inline |
Set a DownloadLimit for the transfer.
- Note
- this DownloadLimit is not visible in the GUI
- Parameters
-
dlLimit download Limit
Definition at line 179 of file transferhandler.h.
|
inline |
Set the maximum share-ratio.
- Parameters
-
ratio the new maximum share-ratio
Definition at line 195 of file transferhandler.h.
void TransferHandler::setSelected | ( | bool | select | ) |
Selects the current transfer.
Selecting transfers means that all the actions executed from the gui will apply also to the current transfer.
- Parameters
-
select if true the current transfer is selected if false the current transfer is deselected
Definition at line 161 of file transferhandler.cpp.
|
inline |
Set an UploadLimit for the transfer.
- Note
- this UploadLimit is not visible in the GUI
- Parameters
-
ulLimit upload Limit
Definition at line 172 of file transferhandler.h.
|
inlinevirtual |
- Parameters
-
file for which to get the signature
- Returns
- Signature that allows you to add signatures and verify them
Definition at line 284 of file transferhandler.h.
|
inline |
- Returns
- the source url
Definition at line 93 of file transferhandler.h.
|
virtualslot |
These are all Job-related functions.
Definition at line 51 of file transferhandler.cpp.
|
inline |
Definition at line 66 of file transferhandler.h.
|
inline |
Definition at line 64 of file transferhandler.h.
|
inline |
- Returns
- a pixmap associated with the current transfer status
Definition at line 215 of file transferhandler.h.
|
inline |
- Returns
- a string describing the current transfer status
Definition at line 210 of file transferhandler.h.
|
virtualslot |
Definition at line 64 of file transferhandler.cpp.
KIO::filesize_t TransferHandler::totalSize | ( | ) | const |
- Returns
- the total size of the transfer in bytes
Definition at line 86 of file transferhandler.cpp.
|
signal |
KIO::filesize_t TransferHandler::uploadedSize | ( | ) | const |
- Returns
- the uploaded size of the transfer in bytes
Definition at line 96 of file transferhandler.cpp.
|
inline |
- Returns
- the upload Limit of the transfer in KiB
Definition at line 184 of file transferhandler.h.
int TransferHandler::uploadSpeed | ( | ) | const |
- Returns
- the upload speed of the transfer in bytes/sec
Definition at line 116 of file transferhandler.cpp.
|
inlinevirtual |
- Parameters
-
file for which to get the verifier
- Returns
- Verifier that allows you to add checksums manually verify a file etc.
Definition at line 278 of file transferhandler.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.