kget
#include <transfergroup.h>
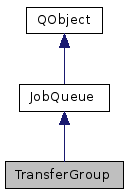
Public Types | |
typedef int | ChangesFlags |
enum | GroupChange { Gc_None = 0x00000000, Gc_GroupName = 0x00000001, Gc_Status = 0x00000002, Gc_TotalSize = 0x00000004, Gc_Percent = 0x00000008, Gc_UploadSpeed = 0x00000010, Gc_DownloadSpeed = 0x00000020, Gc_ProcessedSize = 0x00010000 } |
![]() | |
enum | Status { Running, Stopped } |
Public Member Functions | |
TransferGroup (TransferTreeModel *model, Scheduler *parent, const QString &name=QString()) | |
virtual | ~TransferGroup () |
void | append (Transfer *transfer) |
void | append (const QList< Transfer * > &transfer) |
void | calculateDownloadLimit () |
void | calculateSpeedLimits () |
void | calculateUploadLimit () |
QString | defaultFolder () |
int | downloadedSize () const |
int | downloadLimit (Transfer::SpeedLimit limit) const |
int | downloadSpeed () |
Transfer * | findTransfer (const KUrl &src) |
Transfer * | findTransferByDestination (const KUrl &dest) |
TransferGroupHandler * | handler () const |
QString | iconName () const |
void | insert (Transfer *transfer, Transfer *after) |
void | load (const QDomElement &e) |
TransferTreeModel * | model () |
void | move (Transfer *transfer, Transfer *after) |
const QString & | name () |
Transfer * | operator[] (int i) const |
int | percent () const |
QPixmap | pixmap () |
void | prepend (Transfer *transfer) |
QRegExp | regExp () |
void | remove (Transfer *transfer) |
void | remove (const QList< Transfer * > &transfers) |
void | save (QDomElement e) |
void | setDefaultFolder (QString folder) |
void | setDownloadLimit (int dlLimit, Transfer::SpeedLimit limit) |
void | setIconName (const QString &name) |
void | setName (const QString &name) |
void | setRegExp (const QRegExp ®Exp) |
void | setStatus (Status queueStatus) |
void | setUploadLimit (int ulLimit, Transfer::SpeedLimit limit) |
bool | supportsSpeedLimits () |
int | totalSize () const |
int | uploadedSize () const |
int | uploadLimit (Transfer::SpeedLimit limit) const |
int | uploadSpeed () |
![]() | |
JobQueue (Scheduler *parent) | |
virtual | ~JobQueue () |
iterator | begin () |
iterator | end () |
int | indexOf (Job *job) const |
Job * | last () |
int | maxSimultaneousJobs () const |
Job * | operator[] (int i) const |
const QList< Job * > | runningJobs () |
void | setMaxSimultaneousJobs (int n) |
int | size () const |
Status | status () const |
Additional Inherited Members | |
![]() | |
void | append (Job *job) |
void | append (const QList< Job * > &jobs) |
void | insert (Job *job, Job *after) |
void | move (Job *job, Job *after) |
void | prepend (Job *job) |
void | remove (Job *job) |
void | remove (const QList< Job * > jobs) |
Scheduler * | scheduler () |
Detailed Description
class TransferGroup:
This class abstracts the concept of transfer group by means of which the user can sort his transfers into categories. By definition, we want each TransferGroup (transfer group) to be a JobQueue. Moreover this class calculates information such as:
- the size obtained by the sum of all the transfer's size
- the size obtained by the sum of all the transfer's processed size
- the global progress percentage within the group
- the global speed within the group
Definition at line 46 of file transfergroup.h.
Member Typedef Documentation
typedef int TransferGroup::ChangesFlags |
Definition at line 64 of file transfergroup.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Gc_None | |
Gc_GroupName | |
Gc_Status | |
Gc_TotalSize | |
Gc_Percent | |
Gc_UploadSpeed | |
Gc_DownloadSpeed | |
Gc_ProcessedSize |
Definition at line 50 of file transfergroup.h.
Constructor & Destructor Documentation
TransferGroup::TransferGroup | ( | TransferTreeModel * | model, |
Scheduler * | parent, | ||
const QString & | name = QString() |
||
) |
Definition at line 28 of file transfergroup.cpp.
|
virtual |
Definition at line 40 of file transfergroup.cpp.
Member Function Documentation
void TransferGroup::append | ( | Transfer * | transfer | ) |
Appends a new transfer to the list of the transfers.
- Parameters
-
transfer the transfer to append
Definition at line 102 of file transfergroup.cpp.
void TransferGroup::append | ( | const QList< Transfer * > & | transfer | ) |
Appends new transfers to the list of the transfers.
- Parameters
-
transfers to append
Definition at line 109 of file transfergroup.cpp.
void TransferGroup::calculateDownloadLimit | ( | ) |
Calculates the DownloadLimits.
Definition at line 238 of file transfergroup.cpp.
void TransferGroup::calculateSpeedLimits | ( | ) |
Calculates the whole SpeedLimits.
Definition at line 231 of file transfergroup.cpp.
void TransferGroup::calculateUploadLimit | ( | ) |
Calculates the DownloadLimits.
Definition at line 281 of file transfergroup.cpp.
|
inline |
- Returns
- the groups default folder
Definition at line 209 of file transfergroup.h.
|
inline |
- Returns
- the sum of the downloaded sizes of the transfers belonging to this group
Definition at line 175 of file transfergroup.h.
int TransferGroup::downloadLimit | ( | Transfer::SpeedLimit | limit | ) | const |
- Returns
- the group's Download-Limit
Definition at line 223 of file transfergroup.cpp.
int TransferGroup::downloadSpeed | ( | ) |
- Returns
- the sum of the download speeds of the running transfers belonging this group
Definition at line 44 of file transfergroup.cpp.
Transfer * TransferGroup::findTransfer | ( | const KUrl & | src | ) |
Finds the first transfer with source src.
- Parameters
-
src the url of the source location
- Returns
- the transfer pointer if the transfer has been found. Otherwise it returns 0
Definition at line 161 of file transfergroup.cpp.
Transfer * TransferGroup::findTransferByDestination | ( | const KUrl & | dest | ) |
Finds the first transfer with destination dest.
- Parameters
-
dest the url of the destination location
- Returns
- the transfer pointer if the transfer has been found, else return 0
Definition at line 175 of file transfergroup.cpp.
|
inline |
- Returns
- the handler associated with this group
Definition at line 283 of file transfergroup.h.
|
inline |
- Returns
- the group's icon's name
Definition at line 273 of file transfergroup.h.
inserts a transfer to the current group after the given transfer
- Parameters
-
transfer The transfer to add in the current Group after The transfer after which to add the transfer
Definition at line 128 of file transfergroup.cpp.
void TransferGroup::load | ( | const QDomElement & | e | ) |
Adds all the groups in the given QDomNode * to the group.
- Parameters
-
n The QDomNode where the group will look for the transfers to add
Definition at line 361 of file transfergroup.cpp.
|
inline |
- Returns
- the TransferTreeModel that owns this group
Definition at line 288 of file transfergroup.h.
Moves a transfer in the list.
- Parameters
-
transfer The transfer to move. Note that this transfer can belong to other groups. In this situation this transfer is deleted from the previous group and moved inside this one. after The transfer after which we have to move the given one
Definition at line 153 of file transfergroup.cpp.
|
inline |
- Returns
- the group name
Definition at line 163 of file transfergroup.h.
|
inline |
- Returns
- the Job in the queue at the given index i
Definition at line 153 of file transfergroup.h.
|
inline |
- Returns
- the progress percentage
Definition at line 186 of file transfergroup.h.
|
inline |
- Returns
- the group's icon
Definition at line 278 of file transfergroup.h.
void TransferGroup::prepend | ( | Transfer * | transfer | ) |
Prepends a new transfer to the list of the transfers.
- Parameters
-
transfer the transfer to prepend
Definition at line 121 of file transfergroup.cpp.
|
inline |
- Returns
- the regular expression of the group
Definition at line 220 of file transfergroup.h.
void TransferGroup::remove | ( | Transfer * | transfer | ) |
Removes the given transfer from the list of the transfers.
- Parameters
-
transfer the transfer to remove
Definition at line 135 of file transfergroup.cpp.
void TransferGroup::remove | ( | const QList< Transfer * > & | transfers | ) |
Removes the given transfers from the list of the transfers.
- Parameters
-
transfers the transfers to remove
Definition at line 142 of file transfergroup.cpp.
void TransferGroup::save | ( | QDomElement | e | ) |
Saves this group object to the given QDomNode.
- Parameters
-
n The QDomNode where the group will be saved
Definition at line 324 of file transfergroup.cpp.
|
inline |
Set a default Folder for the group.
- Parameters
-
folder the new default folder
Definition at line 204 of file transfergroup.h.
void TransferGroup::setDownloadLimit | ( | int | dlLimit, |
Transfer::SpeedLimit | limit | ||
) |
Set a Download-Limit for the group.
- Parameters
-
limit the new download-limit
- Note
- if limit is 0, no download-limit is set
Definition at line 202 of file transfergroup.cpp.
|
inline |
Set the group's icon.
- Parameters
-
name the icon's name
Definition at line 268 of file transfergroup.h.
|
inline |
|
inline |
Sets the regular expression of the group.
- Parameters
-
regexp the regular expression
Definition at line 215 of file transfergroup.h.
|
virtual |
This function is reimplemented by JobQueue::setStatus.
- Parameters
-
queueStatus the new JobQueue status
Reimplemented from JobQueue.
Definition at line 95 of file transfergroup.cpp.
void TransferGroup::setUploadLimit | ( | int | ulLimit, |
Transfer::SpeedLimit | limit | ||
) |
Set a Upload-Limit for the group.
- Parameters
-
limit the new upload-limit
- Note
- if limit is 0, no upload-limit is set
Definition at line 189 of file transfergroup.cpp.
bool TransferGroup::supportsSpeedLimits | ( | ) |
- Returns
- true if the group supports SpeedLimits
Definition at line 81 of file transfergroup.cpp.
|
inline |
- Returns
- the sum of the sizes of the transfers belonging to this group
Definition at line 169 of file transfergroup.h.
|
inline |
- Returns
- the sum of the uploaded sizes of the transfers belonging to this group
Definition at line 181 of file transfergroup.h.
int TransferGroup::uploadLimit | ( | Transfer::SpeedLimit | limit | ) | const |
- Returns
- the group's Upload-Limit
Definition at line 215 of file transfergroup.cpp.
int TransferGroup::uploadSpeed | ( | ) |
- Returns
- the sum of the download speeds of the running transfers belonging this group
Definition at line 56 of file transfergroup.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.