kopete/libkopete
#include <kopetecommandhandler.h>
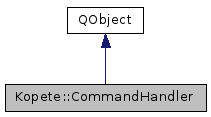
Public Types | |
enum | CommandType { Normal, SystemAlias, UserAlias, Undefined } |
Public Member Functions | |
bool | commandHandled (const QString &command) |
bool | commandHandledByProtocol (const QString &command, Protocol *protocol) |
bool | processMessage (Message &msg, ChatSession *manager) |
bool | processMessage (const QString &msg, ChatSession *manager) |
void | registerAlias (QObject *parent, const QString &alias, const QString &formatString, const QString &help=QString(), CommandType=SystemAlias, uint minArgs=0, int maxArgs=-1, const KShortcut &cut=KShortcut(), const QString &pix=QString()) |
void | registerCommand (QObject *parent, const QString &command, const char *handlerSlot, const QString &help=QString(), uint minArgs=0, int maxArgs=-1, const KShortcut &cut=KShortcut(), const QString &pix=QString()) |
void | unregisterAlias (QObject *parent, const QString &alias) |
void | unregisterCommand (QObject *parent, const QString &command) |
Static Public Member Functions | |
static CommandHandler * | commandHandler () |
static QStringList | parseArguments (const QString &args) |
Detailed Description
The Kopete::CommandHandler can handle /action like messages
Definition at line 49 of file kopetecommandhandler.h.
Member Enumeration Documentation
an enum defining the type of a command
Enumerator | |
---|---|
Normal | |
SystemAlias | |
UserAlias | |
Undefined |
Definition at line 59 of file kopetecommandhandler.h.
Member Function Documentation
bool Kopete::CommandHandler::commandHandled | ( | const QString & | command | ) |
Check if a command is already handled.
- Parameters
-
command The command to check
- Returns
- True if the command is already being handled, False if not
Definition at line 438 of file kopetecommandhandler.cpp.
bool Kopete::CommandHandler::commandHandledByProtocol | ( | const QString & | command, |
Kopete::Protocol * | protocol | ||
) |
Check if a command is already handled by a spesific protocol.
- Parameters
-
command The command to check protocol The protocol to check
- Returns
- True if the command is already being handled, False if not
Definition at line 449 of file kopetecommandhandler.cpp.
|
static |
Returns a pointer to the command handler.
Definition at line 184 of file kopetecommandhandler.cpp.
|
static |
Parses a string of command arguments into a QStringList.
Quoted blocks within the arguments string are treated as one argument.
Definition at line 419 of file kopetecommandhandler.cpp.
bool Kopete::CommandHandler::processMessage | ( | Kopete::Message & | msg, |
Kopete::ChatSession * | manager | ||
) |
Process a message to see if any commands should be handled.
- Parameters
-
msg The message to process manager The manager who owns this message
- Returns
- True if the command was handled, false if not
Definition at line 259 of file kopetecommandhandler.cpp.
bool Kopete::CommandHandler::processMessage | ( | const QString & | msg, |
Kopete::ChatSession * | manager | ||
) |
Process a message to see if any commands should be handled.
- Parameters
-
msg A QString contain the message manager the Kopete::ChatSession who will own the message
- Returns
- true if the command was handled, false if the command was not handled.
Definition at line 227 of file kopetecommandhandler.cpp.
void Kopete::CommandHandler::registerAlias | ( | QObject * | parent, |
const QString & | alias, | ||
const QString & | formatString, | ||
const QString & | help = QString() , |
||
CommandType | type = SystemAlias , |
||
uint | minArgs = 0 , |
||
int | maxArgs = -1 , |
||
const KShortcut & | cut = KShortcut() , |
||
const QString & | pix = QString() |
||
) |
Register a command alias.
- Parameters
-
parent The plugin who owns this alias alias The command for the alias formatString This is the string that will be transformed into another command. The formatString should begin with an already existing command, followed by any other arguments. The variables %1, %2... %9 will be substituted with the arguments passed into the alias. The variable s will be substituted with the entire argument string help An optional help string to be shown when the user uses /help <command> minArgs the minimum number of arguments for this command maxArgs the maximum number of arguments this command takes cut a default keyboard shortcut pix icon name, the icon will be shown in menus
Definition at line 211 of file kopetecommandhandler.cpp.
void Kopete::CommandHandler::registerCommand | ( | QObject * | parent, |
const QString & | command, | ||
const char * | handlerSlot, | ||
const QString & | help = QString() , |
||
uint | minArgs = 0 , |
||
int | maxArgs = -1 , |
||
const KShortcut & | cut = KShortcut() , |
||
const QString & | pix = QString() |
||
) |
Register a command with the command handler.
Command matching is case insensitive. All commands are registered, regardless of whether or not they are already handled by another handler. This is so that if the first plugin is unloaded, the next handler in the sequence will handle the command. However, there are certain commands which are reserved (internally handled by the Kopete::CommandHandler). These commands can also be overridden by registering a new duplicate command.
- Parameters
-
parent The plugin who owns this command command The command we want to handle, not including the '/' handlerSlot The slot used to handle the command. This slot must accept two parameters, a QString of arguments, and a Kopete::ChatSession pointer to the manager under which the command was sent. help An optional help string to be shown when the user uses /help <command> minArgs the minimum number of arguments for this command maxArgs the maximum number of arguments this command takes cut a default keyboard shortcut pix icon name, the icon will be shown in menus
Definition at line 195 of file kopetecommandhandler.cpp.
void Kopete::CommandHandler::unregisterAlias | ( | QObject * | parent, |
const QString & | alias | ||
) |
Unregister an alias.
- Parameters
-
parent The plugin who owns this alias alias The alais to unload
Definition at line 221 of file kopetecommandhandler.cpp.
void Kopete::CommandHandler::unregisterCommand | ( | QObject * | parent, |
const QString & | command | ||
) |
Unregister a command.
When a plugin unloads, all commands are automaticlly unregistered and deleted. This function should only be called in the case of a plugin which loads and unloads commands dynamically.
- Parameters
-
parent The plugin who owns this command command The command to unload
Definition at line 205 of file kopetecommandhandler.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:51 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.