kopete/libkopete
#include <kopeteprotocol.h>
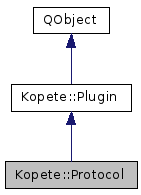
Public Types | |
enum | Capability { BaseFgColor = 0x1, BaseBgColor = 0x2, RichFgColor = 0x4, RichBgColor = 0x8, BaseFont = 0x10, RichFont = 0x20, BaseUFormatting = 0x40, BaseIFormatting = 0x80, BaseBFormatting = 0x100, RichUFormatting = 0x200, RichIFormatting = 0x400, RichBFormatting = 0x800, Alignment = 0x1000, BaseFormatting = BaseIFormatting | BaseUFormatting | BaseBFormatting, RichFormatting = RichIFormatting | RichUFormatting | RichBFormatting, RichColor = RichBgColor | RichFgColor, BaseColor = BaseBgColor | BaseFgColor, FullRTF = RichFormatting | Alignment | RichFont | RichFgColor | RichBgColor, CanSendOffline = 0x10000 } |
![]() | |
enum | AddressBookFieldAddMode { AddOnly, MakeIndexField } |
Public Member Functions | |
virtual | ~Protocol () |
virtual void | aboutToUnload () |
Kopete::OnlineStatus | accountOfflineStatus () const |
bool | canAddMyself () const |
Capabilities | capabilities () const |
virtual AddContactPage * | createAddContactWidget (QWidget *parent, Account *account)=0 |
virtual KopeteEditAccountWidget * | createEditAccountWidget (Account *account, QWidget *parent)=0 |
virtual Account * | createNewAccount (const QString &accountId)=0 |
virtual KJob * | createProtocolTask (const QString &taskType) |
virtual void | deserialize (MetaContact *metaContact, const QMap< QString, QString > &serializedData) |
virtual Contact * | deserializeContact (MetaContact *metaContact, const QMap< QString, QString > &serializedData, const QMap< QString, QString > &addressBookData) |
virtual void | deserializeContactList (MetaContact *metaContact, const QList< QMap< QString, QString > > &dataList) |
void | serialize (Kopete::MetaContact *metaContact) |
virtual bool | validatePassword (const QString &password) const |
![]() | |
Plugin (const KComponentData &instance, QObject *parent) | |
virtual | ~Plugin () |
void | addAddressBookField (const QString &field, AddressBookFieldAddMode mode=AddOnly) |
QStringList | addressBookFields () const |
QString | addressBookIndexField () const |
QString | displayName () const |
QString | pluginIcon () const |
QString | pluginId () const |
KPluginInfo | pluginInfo () const |
Q_INVOKABLE bool | shouldExitOnclose () |
Q_INVOKABLE bool | showCloseWindowMessage () |
Protected Member Functions | |
Protocol (const KComponentData &instance, QObject *parent, bool canAddMyself=false) | |
void | setCapabilities (Capabilities) |
Additional Inherited Members | |
![]() | |
![]() | |
void | readyForUnload () |
void | settingsChanged () |
Detailed Description
base class of every protocol.
A protocol is just a particular case of Plugin
Protocol is an abstract class, you need to reimplement createNewAccount, createAddContactPage, createEditAccountWidget
Definition at line 62 of file kopeteprotocol.h.
Member Enumeration Documentation
Available capabilities.
capabilities() returns an ORed list of these, which the edit widget interperts to determine what buttons to show
Definition at line 110 of file kopeteprotocol.h.
Constructor & Destructor Documentation
|
virtual |
- Todo:
- Ideally, the destructor should be protected. but we need it public to allow QPtrList<Protocol>
Definition at line 63 of file kopeteprotocol.cpp.
|
protected |
Constructor for Protocol.
- Parameters
-
instance The protocol's instance, every plugin needs to have a KComponentData of its own parent The protocol's parent object name The protocol's name
Definition at line 53 of file kopeteprotocol.cpp.
Member Function Documentation
|
virtual |
Reimplemented from Kopete::Plugin.
This method disconnects all accounts and deletes them, after which it will emit readyForUnload.
Note that this is an asynchronous operation that may take some time with active chats. It's no longer immediate as it used to be in Kopete 0.7.x and before. This also means that you can do a clean shutdown.
- Note
- The method is not private to allow subclasses to reimplement it even more, but if you need to do this please explain why on the list first. It might make more sense to add another virtual for protocols that's called instead, but for now I actually think protocols don't need their own implementation at all, so I left out the necessary hooks on purpose.
- Martijn
Reimplemented from Kopete::Plugin.
Definition at line 130 of file kopeteprotocol.cpp.
Kopete::OnlineStatus Kopete::Protocol::accountOfflineStatus | ( | ) | const |
Returns the status used for contacts when accounts of this protocol are offline.
Definition at line 94 of file kopeteprotocol.cpp.
bool Kopete::Protocol::canAddMyself | ( | ) | const |
true if account can add own contact into a contact list
Definition at line 89 of file kopeteprotocol.cpp.
Protocol::Capabilities Kopete::Protocol::capabilities | ( | ) | const |
a bitmask of the capabilities of this protocol
- See also
- setCapabilities
Definition at line 79 of file kopeteprotocol.cpp.
|
pure virtual |
Create a new AddContactPage widget to be shown in the Add Contact Wizard.
- Returns
- A new AddContactPage to be shown in the Add Contact Wizard
|
pure virtual |
Create a new KopeteEditAccountWidget.
- Returns
- A new KopeteEditAccountWidget to be shown in the account part of the configurations.
- Parameters
-
account is the KopeteAccount to edit. If it's 0L, then we create a new account parent The parent of the 'to be returned' widget
|
pure virtual |
Create an empty Account.
This method is called during the loading of the config file.
- Parameters
-
accountId - the account ID to create the account with. This is usually the login name of the account
you don't need to register the account to the AccountManager in this function. But if you want to use this function don't forget to call AccountManager::registerAccount
- Returns
- The new Account object created by this function
|
virtual |
Factory method to create a protocol Task.
Protocols Task are tasks needed for executing specific commands for the given protocol. This method creates the required task for the given task type.
If a task type is not available in the protocol, just return a null pointer, like the default implementation.
Example of a implementation of createProtocolTask()
- Parameters
-
taskType a task type as a string. Check each task for name.
Definition at line 311 of file kopeteprotocol.cpp.
|
virtual |
Deserialize the plugin data for a meta contact.
This method splits up the data into the independent Kopete::Contact objects and calls deserializeContact() for each contact.
Note that you can still reimplement this method if you prefer, but you are strongly recommended to use this version of the method instead, unless you want to do VERY special things with the data...
- Todo:
- we probably should think to another way to save the contacltist.
Reimplemented from Kopete::Plugin.
Definition at line 293 of file kopeteprotocol.cpp.
|
virtual |
Deserialize a single contact.
This method is called by deserialize() for each separate contact, so you don't need to add your own hooks for multiple contacts in a single meta contact yourself. serializedData
and addressBookData
will be the data the contact provided in Kopete::Contact::serialize.
The default implementation does nothing.
- Returns
- The contact created from the data
- See also
- Contact::serialize
- Todo:
- we probably should think to another way to save the contacltist.
Definition at line 299 of file kopeteprotocol.cpp.
|
virtual |
Deserialize the plugin data for a meta contact's contacts.
Definition at line 231 of file kopeteprotocol.cpp.
void Kopete::Protocol::serialize | ( | Kopete::MetaContact * | metaContact | ) |
Serialize meta contact into the metacontact's plugin data Call serialize() for all contained contacts for this protocol.
it's public because for example, Contact::setMetaContact uses it.
- Todo:
- we probably should think to another way to save the contacltist.
Definition at line 175 of file kopeteprotocol.cpp.
|
protected |
Sets the capabilities of this protcol.
The subclass contructor is a good place for calling it.
- See also
- capabilities()
Definition at line 84 of file kopeteprotocol.cpp.
|
virtual |
Check whether a password is valid for this protocol.
The default implementation validates every password
- Parameters
-
password The password to check
Definition at line 318 of file kopeteprotocol.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.