kopete/libkopete
#include <kopetecontact.h>
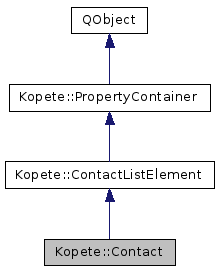
Public Types | |
enum | CanCreateFlags { CannotCreate =false, CanCreate =true } |
enum | Changed { MovedBetweenGroup = 0x01, DisplayNameChanged = 0x02 } |
![]() | |
typedef QMap< QString, QString > | ContactData |
typedef QList< ContactData > | ContactDataList |
typedef QMap< IconState, QString > | IconMap |
enum | IconState { None, Open, Closed, Online, Away, Offline, Unknown } |
typedef QMap< QString, QMap < QString, QString > > | PluginDataMap |
Public Slots | |
void | changeMetaContact () |
virtual KDE_DEPRECATED void | deleteContact () |
void | execute () |
virtual void | sendFile (const KUrl &sourceURL=KUrl(), const QString &fileName=QString(), uint fileSize=0L) |
void | sendMessage () |
virtual void | slotUserInfo () |
void | startChat () |
virtual void | sync (unsigned int changed=0xFF) |
void | toggleAlwaysVisible () |
Signals | |
void | canAcceptFilesChanged () |
void | contactDestroyed (Kopete::Contact *contact) |
void | idleStateChanged (Kopete::Contact *contact) |
void | onlineStatusChanged (Kopete::Contact *contact, const Kopete::OnlineStatus &status, const Kopete::OnlineStatus &oldStatus) |
void | statusMessageChanged (Kopete::Contact *contact) |
![]() | |
void | iconAppearanceChanged () |
void | iconChanged (Kopete::ContactListElement::IconState, const QString &) |
void | pluginDataChanged () |
void | useCustomIconChanged (bool useCustomIcon) |
![]() | |
void | propertyChanged (Kopete::PropertyContainer *container, const QString &key, const QVariant &oldValue, const QVariant &newValue) |
Public Member Functions | |
Contact (Account *account, const QString &id, MetaContact *parent, const QString &icon=QString()) | |
~Contact () | |
Account * | account () const |
bool | canAcceptFiles () const |
QString | contactId () const |
virtual QList< KAction * > * | customContextMenuActions () |
virtual KDE_DEPRECATED QList < KAction * > * | customContextMenuActions (ChatSession *manager) |
QString | formattedIdleTime () const |
QString | formattedName () const |
QString & | icon () const |
virtual unsigned long int | idleTime () const |
bool | isFileCapable () const |
bool | isOnline () const |
virtual bool | isReachable () |
virtual ChatSession * | manager (CanCreateFlags canCreate=CannotCreate)=0 |
MetaContact * | metaContact () const |
QString | nickName () const |
OnlineStatus | onlineStatus () const |
KMenu * | popupMenu () |
KDE_DEPRECATED KMenu * | popupMenu (ChatSession *manager) |
Protocol * | protocol () const |
virtual void | serialize (QMap< QString, QString > &serializedData, QMap< QString, QString > &addressBookData) |
void | setFileCapable (bool filecap) |
void | setIcon (const QString &icon) |
void | setIdleTime (unsigned long int) |
void | setMetaContact (MetaContact *m) |
void | setNickName (const QString &name) |
void | setOnlineStatus (const OnlineStatus &status) |
void | setPhoto (const QString &photoPath) |
void | setStatusMessage (const Kopete::StatusMessage &statusMessage) |
Kopete::StatusMessage | statusMessage () const |
QString | toolTip () const |
![]() | |
void | appendPluginContactData (const QString &pluginId, const ContactData &data) |
void | clearPluginContactData () |
QString | icon (IconState state=None) const |
const IconMap | icons () const |
bool | loading () const |
QMap< QString, ContactDataList > | pluginContactData () const |
ContactDataList | pluginContactData (Plugin *plugin) const |
QMap< QString, QString > | pluginData (Plugin *plugin) const |
QString | pluginData (Plugin *plugin, const QString &key) const |
const PluginDataMap | pluginData () const |
void | setIcon (const QString &icon, IconState=None) |
void | setLoading (bool value) |
void | setPluginContactData (Plugin *plugin, const ContactDataList &dataList) |
void | setPluginData (Plugin *plugin, const QMap< QString, QString > &value) |
void | setPluginData (const QString &pluginId, const QMap< QString, QString > &pluginData) |
void | setPluginData (Plugin *plugin, const QString &key, const QString &value) |
void | setUseCustomIcon (bool useCustomIcon) |
bool | useCustomIcon () const |
![]() | |
PropertyContainer (QObject *parent=0) | |
virtual | ~PropertyContainer () |
void | deserializeProperties (const QMap< QString, QString > &serializedData) |
bool | hasProperty (const QString &key) const |
QStringList | properties () const |
const Kopete::Property & | property (const QString &key) const |
const Kopete::Property & | property (const Kopete::PropertyTmpl &tmpl) const |
void | removeProperty (const Kopete::PropertyTmpl &tmpl) |
void | serializeProperties (QMap< QString, QString > &serializedData) const |
void | setProperty (const Kopete::PropertyTmpl &tmpl, const QVariant &value) |
Properties | |
bool | canAcceptFiles |
QString | contactId |
bool | fileCapable |
QString | formattedIdleTime |
QString | formattedName |
QString | icon |
bool | isOnline |
QString | nickName |
QString | toolTip |
Additional Inherited Members | |
![]() | |
ContactListElement (QObject *parent=0L) | |
~ContactListElement () | |
Detailed Description
This class abstracts a generic contact Use it for inserting contacts in the contact list for example.
Definition at line 58 of file kopetecontact.h.
Member Enumeration Documentation
Enumerator | |
---|---|
CannotCreate | |
CanCreate |
Definition at line 283 of file kopetecontact.h.
used in sync()
Enumerator | |
---|---|
MovedBetweenGroup |
the contact has been moved between groups |
DisplayNameChanged |
the displayname of the contact changed |
Definition at line 80 of file kopetecontact.h.
Constructor & Destructor Documentation
Kopete::Contact::Contact | ( | Account * | account, |
const QString & | id, | ||
MetaContact * | parent, | ||
const QString & | icon = QString() |
||
) |
Create new contact.
The parent MetaContact must not be NULL
- Note
- id is required to be unique per protocol and per account. Across those boundaries ids may occur multiple times. The id is solely for comparing items safely (using pointers is more crash-prone). DO NOT assume anything regarding the id's value! Even if it may look like an ICQ UIN or an MSN passport, this is undefined and may change at any time!
- Parameters
-
account is the parent account. this constructor automatically register the contact to the account id is the Contact's unique Id (mostly the user's login) parent is the parent MetaContact this Contact is part of icon is an optional icon
Definition at line 87 of file kopetecontact.cpp.
Kopete::Contact::~Contact | ( | ) |
Definition at line 124 of file kopetecontact.cpp.
Member Function Documentation
Account * Kopete::Contact::account | ( | ) | const |
Get the account that this contact belongs to.
- Returns
- the Account object for this contact
Definition at line 498 of file kopetecontact.cpp.
bool Kopete::Contact::canAcceptFiles | ( | ) | const |
Get whether or not this contact can accept file transfers.
This function checks to make sure that the contact is online as well as capable of sending files.
- See also
- isReachable()
- Returns
- true if this contact is online and is capable of receiving files
- Todo:
- have a capabilioties. or move to protocol capabilities
|
signal |
Emitted when the file transfer capability of this contact has changed.
|
slot |
Changes the MetaContact that this contact is a part of.
This function is called by the KAction changeMetaContact that is part of the context menu.
Definition at line 327 of file kopetecontact.cpp.
|
signal |
The contact is about to be destroyed.
Called when entering the destructor. Useful for cleanup, since metaContact() is still accessible at this point.
- Warning
- this signal is emit in the Contact destructor, so all virtual method are not available
QString Kopete::Contact::contactId | ( | ) | const |
Get the unique id that identifies a contact.
- Note
- Id is required to be unique per protocol and per account. Across those boundaries ids may occur multiple times. The id is solely for comparing items safely (using pointers is more crash-prone). DO NOT assume anything regarding the id's value! Even if it may look like an ICQ UIN or an MSN passport, this is undefined and may change at any time!
- Returns
- The unique id of the contact
|
virtual |
Get the set of custom menu items for this contact.
Returns a set of custom menu items for the context menu which is displayed in showContextMenu (private). Protocols should use this to add protocol-specific actions to the popup menu. Kopete take care of the deletion of the action collection. Actions should have the collection as parent.
- Returns
- Collection of menu items to be show on the context menu
- Todo:
- if possible, try to use KXMLGUI
Definition at line 521 of file kopetecontact.cpp.
|
virtual |
Definition at line 526 of file kopetecontact.cpp.
|
virtualslot |
- Deprecated:
- Use DeleteContactTask instead. Method to delete a contact from the contact list, should be implemented by protocol plugin to handle protocol-specific actions required to delete a contact (ie. messages to the server, etc) the default implementation simply call deleteLater()
Definition at line 476 of file kopetecontact.cpp.
|
slot |
The user clicked on the contact, do the default action.
Definition at line 446 of file kopetecontact.cpp.
QString Kopete::Contact::formattedIdleTime | ( | ) | const |
Returns a formatted string of idleTime().
Suitable for GUI display
QString Kopete::Contact::formattedName | ( | ) | const |
Returns a formatted string of "firstName" and/or "lastName" properties if present.
Suitable for GUI display
QString& Kopete::Contact::icon | ( | ) | const |
Returns the name of the icon to use for this contact If null, the protocol icon need to be used.
The icon is not colored, nor has the status icon overloaded
|
signal |
The contact's idle state changed.
You need to emit this signal to update the view. That mean when activity has been noticed
|
virtual |
Get the time (in seconds) this contact has been idle It will return the time set in setIdleTime() with an addition of the time since you set this last time.
- Returns
- time this contact has been idle for, in seconds
FIXME: Can we make this just 'unsigned long' ? QT Properties can't handle 'unsigned long int'
Definition at line 555 of file kopetecontact.cpp.
bool Kopete::Contact::isFileCapable | ( | ) | const |
Get whether or not this contact is capable of file transfers.
- See also
- setFileCapable()
- Returns
- true if the protocol for this contact is capable of file transfers
- false if the protocol for this contact is not capable of file transfers
- Todo:
- have a capabilioties. or move to protocol capabilities
Definition at line 536 of file kopetecontact.cpp.
bool Kopete::Contact::isOnline | ( | ) | const |
Get whether this contact is online.
- Returns
true
if the contact is online,false
otherwise.
|
virtual |
Get whether this contact can receive messages.
Used in determining if the contact is able to receive messages. This function must be defined by child classes
- Returns
- true if the contact can be reached
- false if the contact cannot be reached
Definition at line 424 of file kopetecontact.cpp.
|
pure virtual |
Returns the primary message manager affiliated with this contact Although a contact can have more than one active message manager (as is the case with MSN at least), only one message manager will ever be the contacts "primary" message manager.
. aka the 1 on 1 chat. This function should always return that instance.
- Parameters
-
canCreate If a new message manager can be created in addition to any existing managers. Currently, this is only set to true when a chat is initiated by the user by clicking the contact list.
MetaContact * Kopete::Contact::metaContact | ( | ) | const |
Get the metacontact for this contact.
- Returns
- The MetaContact object for this contact
Definition at line 483 of file kopetecontact.cpp.
QString Kopete::Contact::nickName | ( | ) | const |
Convenience method to retrieve the nickName property.
This method will return the contactId if there has been no nickName property set
OnlineStatus Kopete::Contact::onlineStatus | ( | ) | const |
Get the online status of the contact.
- Returns
- the online status of the contact
Definition at line 133 of file kopetecontact.cpp.
|
signal |
The contact's online status changed.
KMenu * Kopete::Contact::popupMenu | ( | ) |
Get the Context Menu for this contact.
This menu includes generic actions common to each protocol, and action defined in customContextMenuActions()
Definition at line 241 of file kopetecontact.cpp.
KMenu * Kopete::Contact::popupMenu | ( | ChatSession * | manager | ) |
Definition at line 236 of file kopetecontact.cpp.
Protocol * Kopete::Contact::protocol | ( | ) | const |
Get the protocol that the contact belongs to.
simply return account()->protocol()
- Returns
- the contact's protocol
Definition at line 493 of file kopetecontact.cpp.
|
virtualslot |
This is the Contact level slot for sending files.
It should be implemented by all contacts which have the setFileCapable() flag set to true. If the function is called through the GUI, no parameters are sent and they take on default values (the file is chosen with a file open dialog)
- Parameters
-
sourceURL The actual KUrl of the file you are sending fileName (Optional) An alternate name for the file - what the receiver will see fileSize (Optional) Size of the file being sent. Used when sending a nondeterminate file size (such as over asocket
Definition at line 220 of file kopetecontact.cpp.
|
slot |
Pops up an email type window.
Definition at line 439 of file kopetecontact.cpp.
|
virtual |
Serialize the contact for storage in the contact list.
The provided serializedData contain the contact id in the field "contactId". If you don't like this, or don't want to store these fields at all, you are free to remove them from the list.
Most plugins don't need more than these fields, so they only need to set the address book fields themselves. If you have nothing to save at all you can clear the QMap, an empty map is treated as 'nothing to save'.
The provided addressBookFields QMap contains the index field as marked with Plugin::addAddressBookField() with the contact id as value. If no index field is available the QMap is simply passed as an empty map.
- See also
- Protocol::deserializeContact
Definition at line 419 of file kopetecontact.cpp.
void Kopete::Contact::setFileCapable | ( | bool | filecap | ) |
Set the file transfer capability of this contact.
- Parameters
-
filecap The new file transfer capability setting
- Todo:
- have a capabilioties. or move to protocol capabilities
Definition at line 541 of file kopetecontact.cpp.
void Kopete::Contact::setIcon | ( | const QString & | icon | ) |
Change the icon to use for this contact If you don't want to have the protocol icon as icon for this contact, you may set another icon.
The icon doesn't need to be colored with the account icon as this operation will be performed later.
if you want to go back to the protocol icon, set a null string.
Definition at line 515 of file kopetecontact.cpp.
void Kopete::Contact::setIdleTime | ( | unsigned long int | t | ) |
Set the current idle time in seconds.
Kopete will automatically calculate the time in idleTime except if you set 0.
FIXME: Can we make this just 'unsigned long' ? QT Properties can't handle 'unsigned long int'
Definition at line 563 of file kopetecontact.cpp.
void Kopete::Contact::setMetaContact | ( | MetaContact * | m | ) |
Move this contact to a new MetaContact.
This basically reparents the contact and updates the internal data structures. If the old contact is going to be empty, the old contact will be removed.
- Parameters
-
m The new MetaContact to move this contact to
Definition at line 380 of file kopetecontact.cpp.
void Kopete::Contact::setNickName | ( | const QString & | name | ) |
Convenience method to set the nickName property to the specified value.
- Parameters
-
name The nickname to set
Definition at line 805 of file kopetecontact.cpp.
void Kopete::Contact::setOnlineStatus | ( | const OnlineStatus & | status | ) |
Set the contact's online status.
Definition at line 141 of file kopetecontact.cpp.
void Kopete::Contact::setPhoto | ( | const QString & | photoPath | ) |
Convience method to set the photo property.
- Parameters
-
photoPath Local path to the photo
Definition at line 819 of file kopetecontact.cpp.
void Kopete::Contact::setStatusMessage | ( | const Kopete::StatusMessage & | statusMessage | ) |
Set the contact's status message.
It sets also the "awayMessage" property so you don't need to do it.
Definition at line 183 of file kopetecontact.cpp.
|
inlinevirtualslot |
Method to retrieve user information.
Should be implemented by the protocols, and popup some sort of dialog box
reimplement it to show the informlation
- Todo:
- rename and make it pure virtual
Definition at line 411 of file kopetecontact.h.
|
slot |
This should typically pop up a KopeteChatWindow.
Definition at line 432 of file kopetecontact.cpp.
Kopete::StatusMessage Kopete::Contact::statusMessage | ( | ) | const |
Get the current status message of the contact.
- Returns
- the status in a Kopete::StatusMessage.
Definition at line 178 of file kopetecontact.cpp.
|
signal |
The contact's status message changed.
|
virtualslot |
Syncronise the server and the metacontact.
Protocols with server-side contact lists can implement this to sync the server groups with the metaContact groups. Or the server alias if any.
This method is called every time the metacontact has been moved or renamed.
default implementation does nothing
- Parameters
-
changed is a bitmask of the Changed enum which say why the call to this function is done.
Definition at line 505 of file kopetecontact.cpp.
|
slot |
Toggle the visibility of this contact even if offline.
This function is called by the KAction toggleAlwaysVisible that is part of the context menu.
Definition at line 320 of file kopetecontact.cpp.
QString Kopete::Contact::toolTip | ( | ) | const |
Get the tooltip for this contact Makes use of formattedName() and formattedIdleTime().
- Returns
- an RTF tooltip depending on Kopete::AppearanceSettings settings
Property Documentation
|
read |
Definition at line 68 of file kopetecontact.h.
|
read |
Definition at line 70 of file kopetecontact.h.
|
readwrite |
Definition at line 67 of file kopetecontact.h.
|
read |
Definition at line 65 of file kopetecontact.h.
|
read |
Definition at line 64 of file kopetecontact.h.
|
readwrite |
Definition at line 71 of file kopetecontact.h.
|
read |
Definition at line 66 of file kopetecontact.h.
|
readwrite |
Definition at line 73 of file kopetecontact.h.
|
read |
Definition at line 72 of file kopetecontact.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:51 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.