kopete/libkopete
#include <kopeteaccount.h>
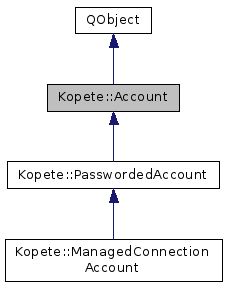
Public Types | |
enum | AddMode { ChangeKABC = 0, DontChangeKABC = 1, Temporary = 2 } |
enum | DisconnectReason { OtherClient = -4, BadPassword = -3, BadUserName = -2, InvalidHost = -1, Manual = 0, ConnectionReset = 1, Unknown = 99 } |
enum | OnlineStatusOption { None = 0x00, KeepSpecialFlags = 0x01 } |
Public Slots | |
virtual void | block (const QString &contactId) |
virtual void | connect (const Kopete::OnlineStatus &initialStatus=OnlineStatus())=0 |
virtual void | disconnect ()=0 |
void | editAccount (QWidget *parent=0L) |
bool | resume () |
virtual void | setOnlineStatus (const Kopete::OnlineStatus &status, const Kopete::StatusMessage &reason=Kopete::StatusMessage(), const OnlineStatusOptions &options=None)=0 |
virtual void | setStatusMessage (const Kopete::StatusMessage &statusMessage)=0 |
bool | suspend (const Kopete::StatusMessage &reason=Kopete::StatusMessage()) |
virtual void | unblock (const QString &contactId) |
Signals | |
void | accountDestroyed (const Kopete::Account *account) |
void | colorChanged (const QColor &) |
void | isConnectedChanged () |
Public Member Functions | |
Account (Protocol *parent, const QString &accountID) | |
~Account () | |
QPixmap | accountIcon (const int size=0) const |
QString | accountIconPath (const KIconLoader::Group size) const |
QString | accountId () const |
QString | accountLabel () const |
MetaContact * | addContact (const QString &contactId, const QString &displayName=QString(), Group *group=0, AddMode mode=DontChangeKABC) |
bool | addContact (const QString &contactId, MetaContact *parent, AddMode mode=DontChangeKABC) |
BlackLister * | blackLister () |
const QColor | color () const |
KConfigGroup * | configGroup () const |
const QHash< QString, Contact * > & | contacts () |
QString | customIcon () const |
bool | excludeConnect () const |
virtual void | fillActionMenu (KActionMenu *actionMenu) |
virtual bool | hasCustomStatusMenu () const |
Identity * | identity () const |
bool | isAway () const |
virtual bool | isBlocked (const QString &contactId) |
bool | isBusy () const |
bool | isConnected () const |
Contact * | myself () const |
uint | priority () const |
Protocol * | protocol () const |
bool | registerContact (Contact *c) |
virtual bool | removeAccount () |
void | setColor (const QColor &color) |
void | setCustomIcon (const QString &) |
void | setExcludeConnect (bool) |
virtual bool | setIdentity (Kopete::Identity *ident) |
void | setPriority (uint priority) |
bool | suppressStatusNotification () const |
Protected Slots | |
virtual void | disconnected (Kopete::Account::DisconnectReason reason) |
void | networkingStatusChanged (const Solid::Networking::Status status) |
void | setAllContactsStatus (const Kopete::OnlineStatus &status) |
Protected Member Functions | |
virtual bool | createContact (const QString &contactId, MetaContact *parentContact)=0 |
void | setAccountLabel (const QString &label) |
void | setMyself (Contact *myself) |
Properties | |
QPixmap | accountIcon |
QString | accountId |
QColor | color |
bool | excludeConnect |
bool | isAway |
bool | isConnected |
uint | priority |
bool | suppressStatusNotification |
Detailed Description
The Kopete::Account class handles one account.
Each protocol implementation should subclass this class in its own custom account class. There are a few pure virtual methods that must be implemented. Examples are:
The accountId is an constant unique id, which represents the login. The myself() contact is one of the most important contacts, which represents the user tied to this account. You must create this contact in the contructor of your account and pass it to setMyself().
All account data is saved to KConfig. This includes the accountId, the autoconnect flag and the color. You can save more data using configGroup()
When you create a new account, you have to register it with the account manager by calling AccountManager::registerAccount.
Definition at line 72 of file kopeteaccount.h.
Member Enumeration Documentation
Describes what should be done when the contact is added to a metacontact.
- See also
- addContact()
Enumerator | |
---|---|
ChangeKABC |
The KDE Address book may be updated. |
DontChangeKABC |
The KDE Address book will not be changed. |
Temporary |
The contact will not be added on the contact list. |
Definition at line 109 of file kopeteaccount.h.
Describes how the account was disconnected.
Manual means that the disconnection was done by the user and no reconnection will take place. Any other value will reconnect the account on disconnection. The case where the password is wrong will be handled differently.
- See also
- disconnected
Definition at line 95 of file kopeteaccount.h.
Describes what should be done when we set new OnlineStatus.
- See also
- setOnlineStatus()
Enumerator | |
---|---|
None |
Use the online status. |
KeepSpecialFlags |
Use the online status but keep special flags, e.g. Invisible. |
Definition at line 119 of file kopeteaccount.h.
Constructor & Destructor Documentation
Kopete::Account::Account | ( | Protocol * | parent, |
const QString & | accountID | ||
) |
Constructor for the Account object.
- Parameters
-
parent the protocol for this account. The account is a child object of the protocol, so it will be automatically deleted when the protocol is. accountID the unique ID of this account. name the name of this QObject.
Definition at line 101 of file kopeteaccount.cpp.
Kopete::Account::~Account | ( | ) |
Destroy the Account object.
Definition at line 128 of file kopeteaccount.cpp.
Member Function Documentation
|
signal |
Emitted when the account is deleted.
- Warning
- emitted in the Account destructor. It is not safe to call any functions on
account
.
QPixmap Kopete::Account::accountIcon | ( | const int | size = 0 | ) | const |
Get the icon for this account.
Generates an image of size size
representing this account. The result is not cached.
- Parameters
-
size the size of the icon. If the size is 0, the default size is used.
- Returns
- the icon for this account, colored if needed
Definition at line 251 of file kopeteaccount.cpp.
QString Kopete::Account::accountIconPath | ( | const KIconLoader::Group | size | ) | const |
Definition at line 273 of file kopeteaccount.cpp.
QString Kopete::Account::accountId | ( | ) | const |
- Returns
- the unique ID of this account used as the login
QString Kopete::Account::accountLabel | ( | ) | const |
The label for this account.
This is used in the GUI.
- Returns
- The label of this account
Definition at line 290 of file kopeteaccount.cpp.
Kopete::MetaContact * Kopete::Account::addContact | ( | const QString & | contactId, |
const QString & | displayName = QString() , |
||
Group * | group = 0 , |
||
AddMode | mode = DontChangeKABC |
||
) |
Create a contact (creating a new metacontact if necessary)
If a contact for this account with ID contactId
is not already on the contact list, a new contact with that ID is created, and added to a new metacontact.
If mode
is ChangeKABC
, MetaContact::updateKABC will be called on the resulting metacontact. If mode
is Temporary
, MetaContact::setTemporary will be called on the resulting metacontact, and the metacontact will not be added to group
. If mode
is DontChangeKABC
, no additional action is carried out.
- Parameters
-
contactId the Contact::contactId of the contact to create displayName the displayname (alias) of the new metacontact. Leave as QString() if no alias is known, then by default, the nick will be taken as alias and tracked if changed. group the group to add the created metacontact to, or 0 for the top-level group. mode the mode used to add the contact. Use DontChangeKABC when deserializing.
- Returns
- the new created metacontact or 0L if the operation failed
Definition at line 337 of file kopeteaccount.cpp.
bool Kopete::Account::addContact | ( | const QString & | contactId, |
MetaContact * | parent, | ||
AddMode | mode = DontChangeKABC |
||
) |
Create a new contact, adding it to an existing metacontact.
If a contact for this account with ID contactId
is not already on the contact list, a new contact with that ID is created, and added to the metacontact parent
.
- Parameters
-
contactId the Contact::contactId of the contact to create parent the parent metacontact (must not be 0) mode the mode used to add the contact. See addContact(const QString&,const QString&,Group*,AddMode) for details.
- Returns
true
if creation of the contact succeeded or the contact was already in the list,false
otherwise.
Definition at line 412 of file kopeteaccount.cpp.
BlackLister * Kopete::Account::blackLister | ( | ) |
- Returns
- a pointer to the blacklist of the account
- Todo:
- remove or implement correctly (BlackLister)
Definition at line 667 of file kopeteaccount.cpp.
|
virtualslot |
Add a user to the blacklist.
The default implementation calls blackList()->addContact( contactId )
- Parameters
-
contactId the contact to be added to the blacklist
- Todo:
- remove or implement correctly (BlackLister)
Definition at line 672 of file kopeteaccount.cpp.
const QColor Kopete::Account::color | ( | ) | const |
Get the color for this account.
The color will be used to visually differentiate this account from other accounts on the same protocol.
- Returns
- the user color for this account
|
signal |
The color of the account has been changed.
also emitted when the icon change
- Todo:
- probably rename to accountIconChanged
KConfigGroup * Kopete::Account::configGroup | ( | ) | const |
Return the KConfigGroup used to write and read special properties.
"Protocol", "AccountId" , "Color", "AutoConnect", "Priority", "Enabled" , "Icon" are reserved keyword already in use in that group.
for compatibility, try to not use key that start with a uppercase
Definition at line 280 of file kopeteaccount.cpp.
|
pure virtualslot |
Go online for this service.
- Parameters
-
initialStatus is the status to connect with. If it is an invalid status for this account, the default online for the account should be used.
- Todo:
- probably deprecate in favor of setOnlineStatus
Implemented in Kopete::PasswordedAccount.
const QHash< QString, Contact * > & Kopete::Account::contacts | ( | ) |
Retrieve the list of contacts for this account (except myself contact)
The list is guaranteed to contain only contacts for this account, so you can safely use static_cast to your own derived contact class if needed.
Definition at line 331 of file kopeteaccount.cpp.
|
protectedpure virtual |
Create a new contact in the specified metacontact.
You shouldn't ever call this method yourself. To add contacts, use addContact().
This method is called by addContact(). In this method, you should create the new custom Contact, using parentContact
as the parent.
If the metacontact is not temporary and the protocol supports it, you can add the contact to the server.
- Parameters
-
contactId the ID of the contact to create parentContact the metacontact to add this contact to
- Returns
true
if creating the contact succeeded,false
on failure.
QString Kopete::Account::customIcon | ( | ) | const |
return the icon base This is the custom account icon set with setIcon.
if this icon is null, then the protocol icon is used don't use this function to get the icon that need to be displayed, use accountIcon
Definition at line 731 of file kopeteaccount.cpp.
|
pure virtualslot |
Go offline for this service.
If the service is connecting, you should abort the connection.
You should call the disconnected function from this function.
- Todo:
- probably deprecate in favor of setOnlineStatus
|
protectedvirtualslot |
The service has been disconnected.
You have to call this method when you are disconnected. Depending on the value of reason
, this function may attempt to reconnect to the server.
- BadPassword will ask again for the password
- OtherClient will show a message box
- Parameters
-
reason the reason for the disconnection.
Definition at line 177 of file kopeteaccount.cpp.
|
slot |
Display the edit account widget for the account.
Definition at line 687 of file kopeteaccount.cpp.
bool Kopete::Account::excludeConnect | ( | ) | const |
Get if the account should not log in.
- Returns
true
if the account should not be connected when connectAll at startup,false
otherwise.
|
virtual |
Fill the menu with actions for this account.
You have to reimplement this method to add custom actions to the actionMenu
which will be shown in the statusbar. It is the caller's responsibility to ensure the menu is deleted.
The default implementation provides a generic menu, with actions generated from the protocol's registered statuses, and an action to show the account's settings dialog.
You should call the default implementation from your reimplementation, and add more actions you require to the resulting action menu.
Definition at line 463 of file kopeteaccount.cpp.
|
virtual |
Return true if account has custom status menu.
You have to reimplement this method and return true if you don't want to have status menu in menu which will be shown in the statusbar
The default implementation returns false.
Definition at line 490 of file kopeteaccount.cpp.
Identity * Kopete::Account::identity | ( | ) | const |
Retrieve the identity this account belongs to.
- Returns
- a pointer to the Identity object this account belongs to.
- See also
- setIdentity().
Definition at line 512 of file kopeteaccount.cpp.
bool Kopete::Account::isAway | ( | ) | const |
Indicate whether the account is away.
This is a convenience method that queries Contact::onlineStatus() on myself(). This function is safe to call if setMyself() has not been called yet.
- See also
- isBusy()
|
virtual |
- Returns
true
if the contact with IDcontactId
is in the blacklist,false
otherwise.
- Todo:
- remove or implement correctly (BlackLister)
Definition at line 682 of file kopeteaccount.cpp.
bool Kopete::Account::isBusy | ( | ) | const |
Indicate whether the account is busy.
In busy mode all visible and sound events should be disabled.
This is a convenience method that queries Contact::onlineStatus() on myself(). This function is safe to call if setMyself() has not been called yet.
- See also
- isAway()
Definition at line 507 of file kopeteaccount.cpp.
bool Kopete::Account::isConnected | ( | ) | const |
Indicate whether the account is connected at all.
This is a convenience method that calls Contact::isOnline() on myself(). This function is safe to call if setMyself() has not been called yet.
- See also
- isConnectedChanged()
|
signal |
Emitted whenever isConnected() changes.
Contact * Kopete::Account::myself | ( | ) | const |
Retrieve the 'myself' contact.
- Returns
- a pointer to the Contact object for this account
- See also
- setMyself().
Definition at line 535 of file kopeteaccount.cpp.
|
protectedslot |
React to network status changes.
- Parameters
-
status the new network status.
Definition at line 154 of file kopeteaccount.cpp.
uint Kopete::Account::priority | ( | ) | const |
Get the priority of this account.
Used for sorting and determining the preferred account to message a contact.
Protocol * Kopete::Account::protocol | ( | ) | const |
- Returns
- the Protocol for this account
Definition at line 214 of file kopeteaccount.cpp.
bool Kopete::Account::registerContact | ( | Contact * | c | ) |
Register a new Contact with the account. This should be called only from the Contact constructor, not from anywhere else (not even a derived class).
Definition at line 308 of file kopeteaccount.cpp.
|
virtual |
Remove the account from the server.
Reimplement this if your protocol supports removing the accounts from the server. This function is called by AccountManager::removeAccount typically when you remove the account on the account config page.
You should add a confirmation message box before removing the account. The default implementation does nothing.
- Returns
false
only if the user requested for the account to be deleted, and deleting the account failed. Returnstrue
in all other cases.
Reimplemented in Kopete::PasswordedAccount.
Definition at line 660 of file kopeteaccount.cpp.
|
slot |
Sets account to the online status that was active when suspend() was called.
Returns false if account has not been suspended or status has changed to something other than Offline in the meantime.
Definition at line 612 of file kopeteaccount.cpp.
|
protected |
Sets the account label.
- Parameters
-
label The label to set
Definition at line 285 of file kopeteaccount.cpp.
|
protectedslot |
Sets the online status of all contacts in this account to the same value.
Some protocols do not provide status-changed events for all contacts when an account becomes connected or disconnected. For such protocols, this function may be useful to set all contacts offline.
Calls Kopete::Contact::setOnlineStatus on all contacts of this account (except the myself() contact), passing status
as the status.
- Parameters
-
status the status to set all contacts of this account except myself() to.
Definition at line 623 of file kopeteaccount.cpp.
void Kopete::Account::setColor | ( | const QColor & | color | ) |
Set the color for this account.
This is called by Kopete's account config page; you don't have to set the color yourself.
- See also
- color()
Definition at line 229 of file kopeteaccount.cpp.
void Kopete::Account::setCustomIcon | ( | const QString & | i | ) |
change the account icon.
by default the icon of an account is the protocol one, but it may be overide it. Set QString() to go back to the default (the protocol icon)
this call will emit colorChanged()
Definition at line 721 of file kopeteaccount.cpp.
void Kopete::Account::setExcludeConnect | ( | bool | b | ) |
Set if the account should not log in automatically.
This function can be used by the EditAccountPage. Kopete handles connection automatically.
- See also
- excludeConnect
Definition at line 297 of file kopeteaccount.cpp.
|
virtual |
Sets the identity this account belongs to.
Setting the account to a new identity implies it to be removed from the identity it was previously associated.
- Parameters
-
ident The identity this account should be associated to
- Returns
true
if the identity was changed,false
otherwise
- Note
- You should call the default implementation from your reimplementation
Definition at line 517 of file kopeteaccount.cpp.
|
protected |
Set the 'myself' contact.
This contact must be defined for every account, because it holds the online status of the account. You must call this function in the constructor of your account.
The myself contact can't be deleted as long as the account still exists. The myself contact is used as a member of every ChatSession involving this account. myself's contactId should be the accountID. The online status of the myself contact represents the account's status.
The myself should have the ContactList::myself() as parent metacontact
Definition at line 540 of file kopeteaccount.cpp.
|
pure virtualslot |
Reimplement this function to set the online status.
- Parameters
-
status is the new status reason is the status message to set. options specify how the status should be set
- Note
- If needed, you need to connect. if the offline status is given, you should disconnect
void Kopete::Account::setPriority | ( | uint | priority | ) |
Set the priority of this account.
- Note
- This method is called by the UI, and should not be called elsewhere.
Definition at line 239 of file kopeteaccount.cpp.
|
pure virtualslot |
Reimplement this function to set the status message(with metadata).
You should use this method to set the status message instead of using setOnlineStatus.
- Parameters
-
statusMessage is the status message to set. (Use Kopete::StatusMessage).
bool Kopete::Account::suppressStatusNotification | ( | ) | const |
Indicates whether or not we should suppress status notifications for contacts belonging to this account.
This is used when we just connected or disconnected, and every contact has their initial status set.
- Returns
true
if notifications should not be used,false
otherwise
|
slot |
Disconnects account, required before resume() Returns false if account is already suspended.
Definition at line 599 of file kopeteaccount.cpp.
|
virtualslot |
Remove a user from the blacklist.
The default implementation calls blackList()->removeContact( contactId )
- Parameters
-
contactId the contact to be removed from the blacklist
- Todo:
- remove or implement correctly (BlackLister)
Definition at line 677 of file kopeteaccount.cpp.
Property Documentation
|
read |
Definition at line 80 of file kopeteaccount.h.
|
read |
Definition at line 77 of file kopeteaccount.h.
|
readwrite |
Definition at line 79 of file kopeteaccount.h.
|
readwrite |
Definition at line 78 of file kopeteaccount.h.
|
read |
Definition at line 82 of file kopeteaccount.h.
|
read |
Definition at line 81 of file kopeteaccount.h.
|
readwrite |
Definition at line 84 of file kopeteaccount.h.
|
read |
Definition at line 83 of file kopeteaccount.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:51 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.