kopete/libkopete
#include <kopetemetacontact.h>
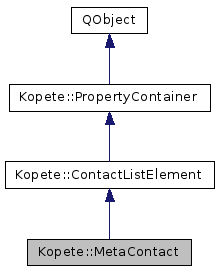
Classes | |
class | Private |
Public Types | |
typedef QList< MetaContact * > | List |
enum | PropertySource { SourceContact, SourceKABC, SourceCustom } |
![]() | |
typedef QMap< QString, QString > | ContactData |
typedef QList< ContactData > | ContactDataList |
typedef QMap< IconState, QString > | IconMap |
enum | IconState { None, Open, Closed, Online, Away, Offline, Unknown } |
typedef QMap< QString, QMap < QString, QString > > | PluginDataMap |
Public Slots | |
void | addToGroup (Kopete::Group *to) |
Contact * | execute () |
void | moveToGroup (Kopete::Group *from, Kopete::Group *to) |
void | removeFromGroup (Kopete::Group *from) |
void | sendFile (const KUrl &sourceURL, const QString &altFileName=QString(), unsigned long fileSize=0L) |
Contact * | sendMessage () |
void | serialize () |
void | setTemporary (bool b=true, Kopete::Group *group=0L) |
void | slotAllPluginsLoaded () |
void | slotPluginLoaded (Kopete::Plugin *plugin) |
void | slotProtocolLoaded (Kopete::Protocol *p) |
Contact * | startChat () |
Public Member Functions | |
MetaContact () | |
~MetaContact () | |
void | addContact (Contact *c) |
QString | addressBookField (Plugin *p, const QString &app, const QString &key) const |
bool | canAcceptFiles () const |
QList< Contact * > | contacts () const |
QString | customDisplayName () const |
KUrl | customPhoto () const |
QString | displayName () const |
PropertySource | displayNameSource () const |
Contact * | displayNameSourceContact () const |
Contact * | findContact (const QString &protocolId, const QString &accountId, const QString &contactId) |
QList< Group * > | groups () const |
quint32 | idleTime () const |
bool | isAlwaysVisible () const |
bool | isOnline () const |
bool | isPhotoSyncedWithKABC () const |
bool | isReachable () const |
bool | isTemporary () const |
QString | kabcId () const |
QUuid | metaContactId () const |
KDE_DEPRECATED QImage | photo () const |
PropertySource | photoSource () const |
Contact * | photoSourceContact () const |
Picture & | picture () const |
Contact * | preferredContact () |
void | removeContact (Contact *c, bool deleted=false) |
void | setAddressBookField (Plugin *p, const QString &app, const QString &key, const QString &value) |
void | setDisplayName (const QString &name) |
void | setDisplayNameSource (PropertySource source) |
void | setDisplayNameSource (const QString &nameSourcePID, const QString &nameSourceAID, const QString &nameSourceCID) |
void | setDisplayNameSourceContact (Contact *contact) |
void | setKabcId (const QString &newKabcId) |
void | setMetaContactId (const QUuid &newMetaContactId) |
void | setPhoto (const KUrl &url) |
void | setPhotoSource (PropertySource source) |
void | setPhotoSource (const QString &photoSourcePID, const QString &photoSourceAID, const QString &photoSourceCID) |
void | setPhotoSourceContact (Contact *contact) |
void | setPhotoSyncedWithKABC (bool b) |
OnlineStatus::StatusType | status () const |
QString | statusIcon () const |
StatusMessage | statusMessage () const |
QString | statusString () const |
![]() | |
void | appendPluginContactData (const QString &pluginId, const ContactData &data) |
void | clearPluginContactData () |
QString | icon (IconState state=None) const |
const IconMap | icons () const |
bool | loading () const |
QMap< QString, ContactDataList > | pluginContactData () const |
ContactDataList | pluginContactData (Plugin *plugin) const |
QMap< QString, QString > | pluginData (Plugin *plugin) const |
QString | pluginData (Plugin *plugin, const QString &key) const |
const PluginDataMap | pluginData () const |
void | setIcon (const QString &icon, IconState=None) |
void | setLoading (bool value) |
void | setPluginContactData (Plugin *plugin, const ContactDataList &dataList) |
void | setPluginData (Plugin *plugin, const QMap< QString, QString > &value) |
void | setPluginData (const QString &pluginId, const QMap< QString, QString > &pluginData) |
void | setPluginData (Plugin *plugin, const QString &key, const QString &value) |
void | setUseCustomIcon (bool useCustomIcon) |
bool | useCustomIcon () const |
![]() | |
PropertyContainer (QObject *parent=0) | |
virtual | ~PropertyContainer () |
void | deserializeProperties (const QMap< QString, QString > &serializedData) |
bool | hasProperty (const QString &key) const |
QStringList | properties () const |
const Kopete::Property & | property (const QString &key) const |
const Kopete::Property & | property (const Kopete::PropertyTmpl &tmpl) const |
void | removeProperty (const Kopete::PropertyTmpl &tmpl) |
void | serializeProperties (QMap< QString, QString > &serializedData) const |
void | setProperty (const Kopete::PropertyTmpl &tmpl, const QVariant &value) |
Protected Member Functions | |
void | onlineStatusNotification (Kopete::Contact *c) |
QImage | photoFromCustom () const |
![]() | |
ContactListElement (QObject *parent=0L) | |
~ContactListElement () | |
Properties | |
bool | canAcceptFiles |
QString | displayName |
bool | isOnline |
bool | isReachable |
bool | isTemporary |
QUuid | metaContactId |
bool | photoSyncedWithKABC |
QString | statusIcon |
QString | statusString |
Detailed Description
A metacontact represent a person. This is a kind of entry to the contact list. All information of a contact is contained in the metacontact. Plugins can store data in it with all ContactListElement methods
Definition at line 54 of file kopetemetacontact.h.
Member Typedef Documentation
typedef QList<MetaContact *> Kopete::MetaContact::List |
Definition at line 70 of file kopetemetacontact.h.
Member Enumeration Documentation
Enumeration of possible sources for a property (which may be photos, see setPhotoSource() for instance).
Enumerator | |
---|---|
SourceContact |
Data comes from the contact itself. |
SourceKABC |
Data comes from KABC (addressbook). |
SourceCustom |
Data comes from somewhere else. |
Definition at line 75 of file kopetemetacontact.h.
Constructor & Destructor Documentation
Kopete::MetaContact::MetaContact | ( | ) |
constructor
Definition at line 50 of file kopetemetacontact.cpp.
Kopete::MetaContact::~MetaContact | ( | ) |
destructor
Definition at line 75 of file kopetemetacontact.cpp.
Member Function Documentation
void Kopete::MetaContact::addContact | ( | Contact * | c | ) |
Add a contact which has just been deserialised to the meta contact.
- Parameters
-
c The Contact being added
Definition at line 90 of file kopetemetacontact.cpp.
|
signal |
The contact was added to another group.
QString Kopete::MetaContact::addressBookField | ( | Kopete::Plugin * | p, |
const QString & | app, | ||
const QString & | key | ||
) | const |
Get or set a field for the KDE address book backend.
Fields not registered during the call to Plugin::addressBookFields() cannot be altered!
- Parameters
-
p The Plugin by which uses this field app refers to the application id in the libkabc database. This should be a standardized format to make sense in the address book in the first place - if you could use "" as application then probably you should use the plugin data API instead of the address book fields. key The name of the address book field to get or set
- Todo:
- : In the code the requirement that fields are registered first is already lifted, but the API needs some review before we can remove it here too. Probably it requires once more some rewrites to get it working properly :( - Martijn
Definition at line 1071 of file kopetemetacontact.cpp.
|
slot |
Add a contact to another group.
Definition at line 1037 of file kopetemetacontact.cpp.
bool Kopete::MetaContact::canAcceptFiles | ( | ) | const |
Returns whether this contact can accept files.
- Returns
- True if the user is online with a file capable protocol, false otherwise
|
signal |
a contact has been added into this metacontact
This signal is emitted when a contact is added to this metacontact
|
signal |
One of the subcontacts' idle status has changed.
As with online status, this can occur without the metacontact changing idle state
|
signal |
a contact has been removed from this metacontact
This signal is emitted when a contact is removed from this metacontact
QList< Contact * > Kopete::MetaContact::contacts | ( | ) | const |
Retrieve the list of contacts that are part of the meta contact.
Definition at line 1270 of file kopetemetacontact.cpp.
|
signal |
A contact's online status changed.
this signal differs from onlineStatusChanged because a contact can change his status without changing MetaContact status. It is mainly used to update the small icons in the contact list
QString Kopete::MetaContact::customDisplayName | ( | ) | const |
Returns the custom display name.
- See also
- displayName()
- displayNameSource()
Definition at line 657 of file kopetemetacontact.cpp.
KUrl Kopete::MetaContact::customPhoto | ( | ) | const |
Returns the custom display photo.
- See also
- photo()
- photoSource()
Definition at line 723 of file kopetemetacontact.cpp.
QString Kopete::MetaContact::displayName | ( | ) | const |
the display name showed in the contact list window
The displayname is the name which should be shown almost everywere to represent the metacontact. (in the contact list, in the chatwindow, ...)
This is a kind of alias, set by the kopete user, as opposed to a nickname set by the contact itself.
If the protocol support alias serverside, the metacontact displayname should probably be synchronized with the alias on the server.
This displayName is obtained from the source set with setDisplayNameSource
|
signal |
The meta contact's display name changed.
MetaContact::PropertySource Kopete::MetaContact::displayNameSource | ( | ) | const |
get the source of metacontact display name
This method obtains the current name source for one of the sources defined in PropertySource
- See also
- PropertySource
Definition at line 296 of file kopetemetacontact.cpp.
Contact * Kopete::MetaContact::displayNameSourceContact | ( | ) | const |
get the subcontact being tracked for its displayname (null if not set)
The MetaContact will adjust its displayName() every time the "nameSource" changes its nickname property.
Definition at line 814 of file kopetemetacontact.cpp.
|
slot |
Contact another user.
Depending on the config settings, call sendMessage() or startChat()
returns the Contact that was chosen as the preferred
Definition at line 419 of file kopetemetacontact.cpp.
Contact * Kopete::MetaContact::findContact | ( | const QString & | protocolId, |
const QString & | accountId, | ||
const QString & | contactId | ||
) |
Find the Contact to a given contact.
If contact is not found, a null pointer is returned. if protocolId
or accountId
are null, it is searched over all protocols/accounts
Definition at line 250 of file kopetemetacontact.cpp.
QList< Group * > Kopete::MetaContact::groups | ( | ) | const |
The groups the contact is stored in.
Definition at line 1061 of file kopetemetacontact.cpp.
quint32 Kopete::MetaContact::idleTime | ( | ) | const |
return the time in second the contact is idle.
Definition at line 438 of file kopetemetacontact.cpp.
bool Kopete::MetaContact::isAlwaysVisible | ( | ) | const |
Returns whether this contact is visible even if offline.
Definition at line 526 of file kopetemetacontact.cpp.
bool Kopete::MetaContact::isOnline | ( | ) | const |
Returns whether this contact can be reached online for at least one FIXME: Make that an enum, because status can be unknown for certain protocols.
bool Kopete::MetaContact::isPhotoSyncedWithKABC | ( | ) | const |
- Returns
- true if when a subcontact change his photo, the photo will be set to the kabc contact.
Definition at line 1202 of file kopetemetacontact.cpp.
bool Kopete::MetaContact::isReachable | ( | ) | const |
Like isOnline, but returns true even if the contact is not online, but can be reached trough offline-messages.
it it return false, you are unable to open a chatwindow
- Todo:
: Here too, use preference order, not append order!
: Here too an enum.
bool Kopete::MetaContact::isTemporary | ( | ) | const |
Temporary contacts will not be serialized.
If they are added to the contact list, they appears in a special "Not in your contact list" group. (the Group::temporary group)
QString Kopete::MetaContact::kabcId | ( | ) | const |
Get the KABC id for this metacontact.
Definition at line 1161 of file kopetemetacontact.cpp.
QUuid Kopete::MetaContact::metaContactId | ( | ) | const |
Returns this metacontact's ID.
Every metacontact has a unique id, set when creating the contact, or reading the contactlist
|
signal |
The contact was moved.
|
slot |
Move a contact from one group to another.
Definition at line 975 of file kopetemetacontact.cpp.
|
signal |
The MetaContact online status changed.
|
protected |
Definition at line 1275 of file kopetemetacontact.cpp.
|
signal |
Some part of this object's persistent data (as returned by toXML) has changed.
QImage Kopete::MetaContact::photo | ( | ) | const |
the photo showed in the contact list window
Returns a image for the metacontact. If the metacontact photo source is the KDE addressbook. it will return the picture stored in the addressbook It can also use a subcontact as the photo source.
This photo is obtained from the source set with setPhotoSource
- Deprecated:
- Use picture().image() instead.
Definition at line 739 of file kopetemetacontact.cpp.
|
signal |
The meta contact's photo changed.
|
protected |
Definition at line 758 of file kopetemetacontact.cpp.
MetaContact::PropertySource Kopete::MetaContact::photoSource | ( | ) | const |
get the source of metacontact photo
This method obtains the current photo source for one of the sources defined in PropertySource
- See also
- PropertySource
Definition at line 318 of file kopetemetacontact.cpp.
Contact * Kopete::MetaContact::photoSourceContact | ( | ) | const |
get the subcontact being tracked for its photo
Definition at line 819 of file kopetemetacontact.cpp.
Picture & Kopete::MetaContact::picture | ( | ) | const |
Return the correct Kopete::Picture object depending of the metacontact photo source.
This photo is obtained from the source set with setPhotoSource
KDE4 TODO: Rename this to photo() and use the new object.
Definition at line 744 of file kopetemetacontact.cpp.
Contact * Kopete::MetaContact::preferredContact | ( | ) |
- Returns
- the preferred child Contact for communication, or 0 if none is suitable (all unreachable).
Definition at line 360 of file kopetemetacontact.cpp.
void Kopete::MetaContact::removeContact | ( | Contact * | c, |
bool | deleted = false |
||
) |
remove the contact from this metacontact
set 'deleted' to true if the Contact is already deleted
- Parameters
-
c is the contact to remove deleted : if it is false, it will disconnect the old contact, and call some method.
Definition at line 161 of file kopetemetacontact.cpp.
|
signal |
The contact was removed from group.
|
slot |
Remove a contact from one group.
Definition at line 1014 of file kopetemetacontact.cpp.
|
slot |
Send a file to this metacontact.
This is the MetaContact level slot for sending files. It may be called through the "Send File" entry in the GUI, or over DCOP. If the function is called through the GUI, no parameters are sent and they assume default values. This slot calls the slotSendFile with identical params of the highest ranked contact capable of sending files (if any)
- Parameters
-
sourceURL The actual KUrl of the file you are sending altFileName (Optional) An alternate name for the file - what the receiver will see fileSize (Optional) Size of the file being sent. Used when sending a nondeterminate file size (such as over a socket)
Definition at line 569 of file kopetemetacontact.cpp.
|
slot |
Send a single message, classic ICQ style.
The actual sending is done by the Contact, but the meta contact does the GUI side of things. This is a slot to allow being called easily from e.g. a GUI.
returns the Contact that was chosen as the preferred
Definition at line 324 of file kopetemetacontact.cpp.
|
slot |
Serialize this metaContact This causes each Kopete::Protocol subclass to serialise its contacts' data into the metacontact's plugin data.
Definition at line 589 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setAddressBookField | ( | Kopete::Plugin * | p, |
const QString & | app, | ||
const QString & | key, | ||
const QString & | value | ||
) |
set an address book field
- See also
- also addressBookField()
- Parameters
-
p The Plugin by which uses this field app The application ID in the KABC database key The name of the address book field to set value The value of the address book field to set
Definition at line 1076 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setDisplayName | ( | const QString & | name | ) |
Set the custom displayName.
This display name is used when name source is Custom this metohd may emit displayNameChanged signal. And will call Kopete::Contact::sync
- See also
- displayName()
- displayNameSource()
Definition at line 614 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setDisplayNameSource | ( | PropertySource | source | ) |
Set the source of metacontact displayName.
This method selects the display name source for one of the sources defined in PropertySource
- See also
- PropertySource
Definition at line 276 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setDisplayNameSource | ( | const QString & | nameSourcePID, |
const QString & | nameSourceAID, | ||
const QString & | nameSourceCID | ||
) |
Definition at line 289 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setDisplayNameSourceContact | ( | Contact * | contact | ) |
set the subcontact whose name is to be tracked (set to null to disable tracking)
- See also
- nameSource
Definition at line 824 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setKabcId | ( | const QString & | newKabcId | ) |
Set the KABC id for this metacontact Use with care! You could create a one to many relationship.
Definition at line 1175 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setMetaContactId | ( | const QUuid & | newMetaContactId | ) |
Set the meta contact id for this meta contact to use. It should only be used by the contact list loading mechanism
Definition at line 85 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setPhoto | ( | const KUrl & | url | ) |
Set the custom photo.
This photo is used when photo source is set toCustom this metohd may emit photoChanged signal.
- See also
- photo()
- photoSource()
Definition at line 728 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setPhotoSource | ( | PropertySource | source | ) |
Set the source of metacontact photo.
This method selects the photo source for one of the sources defined in PropertySource
- See also
- PropertySource
Definition at line 301 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setPhotoSource | ( | const QString & | photoSourcePID, |
const QString & | photoSourceAID, | ||
const QString & | photoSourceCID | ||
) |
Definition at line 311 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setPhotoSourceContact | ( | Contact * | contact | ) |
set the subcontact to use for SourceContact source
Definition at line 836 of file kopetemetacontact.cpp.
void Kopete::MetaContact::setPhotoSyncedWithKABC | ( | bool | b | ) |
Set if the photo should be synced with the adressbook when the photosource change his photo.
If b
is true, the photo will be synced immediately if possible
Definition at line 1207 of file kopetemetacontact.cpp.
|
slot |
Set if this is a temporary contact.
(see isTemporary)
- Parameters
-
b if the contact is or not temporary group if the contact was temporary and b is false, then the contact will be moved to this group. if group is null, it will be moved to top-level
Definition at line 1142 of file kopetemetacontact.cpp.
|
slot |
When all the plugins are loaded, set the Contact Source.
Definition at line 1108 of file kopetemetacontact.cpp.
|
slot |
If a plugin is loaded, maybe data about this plugin are already cached in the metacontact.
Definition at line 1086 of file kopetemetacontact.cpp.
|
slot |
If a protocol is loaded, deserialize cached data.
Definition at line 1098 of file kopetemetacontact.cpp.
|
slot |
Start a chat in a persistent chat window.
Like sendMessage, but this time a full-blown chat will be opened. Most protocols can't distinguish between the two and are either completely session based like MSN or completely message based like ICQ the only true difference is the GUI shown to the user.
returns the Contact that was chosen as the preferred
Definition at line 342 of file kopetemetacontact.cpp.
OnlineStatus::StatusType Kopete::MetaContact::status | ( | ) | const |
Return a more fine-grained status.
Online means at least one sub-contact is online, away means at least one is away, but nobody is online and offline speaks for itself
Definition at line 509 of file kopetemetacontact.cpp.
QString Kopete::MetaContact::statusIcon | ( | ) | const |
The name of the icon associated with the contact's status.
- Todo:
- improve with OnlineStatus
Kopete::StatusMessage Kopete::MetaContact::statusMessage | ( | ) | const |
The status message of metacontact.
Definition at line 1081 of file kopetemetacontact.cpp.
|
signal |
Status title or message has changed.
QString Kopete::MetaContact::statusString | ( | ) | const |
Property Documentation
|
read |
Definition at line 64 of file kopetemetacontact.h.
|
readwrite |
Definition at line 58 of file kopetemetacontact.h.
|
read |
Definition at line 61 of file kopetemetacontact.h.
|
read |
Definition at line 62 of file kopetemetacontact.h.
|
read |
Definition at line 63 of file kopetemetacontact.h.
|
readwrite |
Definition at line 66 of file kopetemetacontact.h.
|
readwrite |
Definition at line 67 of file kopetemetacontact.h.
|
read |
Definition at line 60 of file kopetemetacontact.h.
|
read |
Definition at line 59 of file kopetemetacontact.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.