kopete/libkopete
#include <kopeteplugin.h>
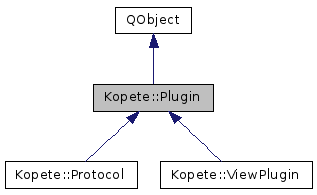
Public Types | |
enum | AddressBookFieldAddMode { AddOnly, MakeIndexField } |
Public Slots | |
virtual void | deserialize (MetaContact *metaContact, const QMap< QString, QString > &data) |
Signals | |
void | readyForUnload () |
void | settingsChanged () |
Public Member Functions | |
Plugin (const KComponentData &instance, QObject *parent) | |
virtual | ~Plugin () |
virtual void | aboutToUnload () |
void | addAddressBookField (const QString &field, AddressBookFieldAddMode mode=AddOnly) |
QStringList | addressBookFields () const |
QString | addressBookIndexField () const |
QString | displayName () const |
QString | pluginIcon () const |
QString | pluginId () const |
KPluginInfo | pluginInfo () const |
Q_INVOKABLE bool | shouldExitOnclose () |
Q_INVOKABLE bool | showCloseWindowMessage () |
Detailed Description
Base class for all plugins or protocols.
To create a plugin, you need to create a .desktop file which looks like that:
[Desktop Entry] Encoding=UTF-8 Type=Service X-Kopete-Version=1000900 Icon=icon ServiceTypes=Kopete/Plugin X-KDE-Library=kopete_myplugin X-KDE-PluginInfo-Author=Your Name X-KDE-PluginInfo-Email=your@mail.com X-KDE-PluginInfo-Name=kopete_myplugin X-KDE-PluginInfo-Version=0.0.1 X-KDE-PluginInfo-Website=http://yoursite.com X-KDE-PluginInfo-Category=Plugins X-KDE-PluginInfo-Depends= X-KDE-PluginInfo-License=GPL X-KDE-PluginInfo-EnabledByDefault=false Name=MyPlugin Comment=Plugin that do some nice stuff
The constructor of your plugin should looks like this:
Kopete::Plugin inherits from KXMLGUIClient. That client is added to the Kopete's mainwindow KXMLGUIFactory. So you may add actions on the main window (for hinstance in the meta contact popup menu). Please note that the client is added right after the plugin is created, so you have to create every actions in the constructor.
Definition at line 84 of file kopeteplugin.h.
Member Enumeration Documentation
Mode for an address book field as used by addAddressBookField()
Enumerator | |
---|---|
AddOnly | |
MakeIndexField |
Definition at line 139 of file kopeteplugin.h.
Constructor & Destructor Documentation
Kopete::Plugin::Plugin | ( | const KComponentData & | instance, |
QObject * | parent | ||
) |
Definition at line 34 of file kopeteplugin.cpp.
|
virtual |
Definition at line 41 of file kopeteplugin.cpp.
Member Function Documentation
|
virtual |
Prepare for unloading a plugin.
When unloading a plugin the plugin manager first calls aboutToUnload() to indicate the pending unload. Some plugins need time to shutdown asynchronously and thus can't be simply deleted in the destructor.
The default implementation immediately emits the readyForUnload() signal, which basically makes the shutdown immediate and synchronous. If you need more time you can reimplement this method and fire the signal whenever you're ready. (you have 3 seconds)
Kopete::Protocol reimplement it.
Reimplemented in Kopete::Protocol, and Kopete::ViewPlugin.
Definition at line 78 of file kopeteplugin.cpp.
void Kopete::Plugin::addAddressBookField | ( | const QString & | field, |
AddressBookFieldAddMode | mode = AddOnly |
||
) |
Add a field to the list of address book fields.
See also addressBookFields() for a description of the fields.
Set mode to MakeIndexField to make this the index field. Index fields are currently used by Kopete::Contact::serialize to autoset the index when possible.
Only one field can be index field. Calling this method multiple times as index field will reset the value of index field!
Definition at line 93 of file kopeteplugin.cpp.
QStringList Kopete::Plugin::addressBookFields | ( | ) | const |
Return the list of all keys from the address book in which the plugin is interested.
Those keys are monitored for changes upon load and during runtime. When the key actually changes, the plugin's addressBookKeyChanged( Kopete::MetaContact *mc, const QString &key ) is called. You can add fields to the list using addAddressBookField()
Definition at line 100 of file kopeteplugin.cpp.
QString Kopete::Plugin::addressBookIndexField | ( | ) | const |
Return the index field as set by addAddressBookField()
Definition at line 105 of file kopeteplugin.cpp.
|
virtualslot |
deserialize() and tell the plugin to apply the previously stored data again.
This method is also responsible for retrieving the settings from the address book. Settings that were registered can be retrieved with Kopete::MetaContact::addressBookField().
The default implementation does nothing.
- Todo:
- we probably should think to another way to save the contacltist.
Reimplemented in Kopete::Protocol.
Definition at line 85 of file kopeteplugin.cpp.
QString Kopete::Plugin::displayName | ( | ) | const |
Returns the display name of this plugin.
This is a convenience method that simply calls pluginInfo()->name().
Definition at line 52 of file kopeteplugin.cpp.
QString Kopete::Plugin::pluginIcon | ( | ) | const |
Get the name of the icon for this plugin.
The icon name is taken from the .desktop file.
May return an empty string if the .desktop file for this plugin specifies no icon name to use.
This is a convenience method that simply calls pluginInfo()->icon().
Definition at line 57 of file kopeteplugin.cpp.
QString Kopete::Plugin::pluginId | ( | ) | const |
Get the plugin id.
- Returns
- the plugin's id which is gotten by calling QObject::metaObject()->className().
Definition at line 46 of file kopeteplugin.cpp.
KPluginInfo Kopete::Plugin::pluginInfo | ( | ) | const |
Returns the KPluginInfo object associated with this plugin.
Definition at line 63 of file kopeteplugin.cpp.
|
signal |
Indicate when we're ready for unload.
- See also
- aboutToUnload()
|
signal |
Notify that the settings of a plugin were changed.
These changes are passed on from the new KCDialog code in kdelibs/kutils.
bool Kopete::Plugin::shouldExitOnclose | ( | ) |
Called when the application is about to exit.
Return false if you want to prevent exit. If you do so you may want to display an explanation message by reimplementing showCloseWindowMessage().
Default implementation returns true.
Not implemented as a virtual method to keep binary compatibility. You must mark the method as Q_INVOKABLE for it to be called.
Definition at line 73 of file kopeteplugin.cpp.
bool Kopete::Plugin::showCloseWindowMessage | ( | ) |
Called when user is about to close the main-window.
If your plugin is going to return false to shouldExitOnclose(), then you can show a message here.
Default implementation returns false.
- Returns
- true if a message was shown (so that the user is not spammed with further messages), false otherwise
Not implemented as a virtual method to keep binary compatibility. You must mark the method as Q_INVOKABLE for it to be called.
Definition at line 68 of file kopeteplugin.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.