kopete/libkopete
#include <kopetegroup.h>
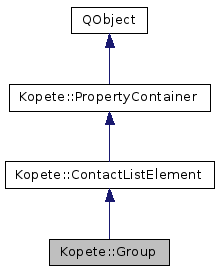
Classes | |
class | Private |
Public Types | |
enum | GroupType { Normal =0, Temporary, TopLevel, Offline } |
typedef QList< Group * > | List |
![]() | |
typedef QMap< QString, QString > | ContactData |
typedef QList< ContactData > | ContactDataList |
typedef QMap< IconState, QString > | IconMap |
enum | IconState { None, Open, Closed, Online, Away, Offline, Unknown } |
typedef QMap< QString, QMap < QString, QString > > | PluginDataMap |
Public Slots | |
void | sendMessage () |
Signals | |
void | displayNameChanged (Kopete::Group *group, const QString &oldName) |
![]() | |
void | iconAppearanceChanged () |
void | iconChanged (Kopete::ContactListElement::IconState, const QString &) |
void | pluginDataChanged () |
void | useCustomIconChanged (bool useCustomIcon) |
![]() | |
void | propertyChanged (Kopete::PropertyContainer *container, const QString &key, const QVariant &oldValue, const QVariant &newValue) |
Public Member Functions | |
Group () | |
Group (const QString &displayName) | |
~Group () | |
QString | displayName () const |
uint | groupId () const |
bool | isExpanded () const |
QList< MetaContact * > | members () const |
void | setDisplayName (const QString &newName) |
void | setExpanded (bool expanded) |
void | setGroupId (uint groupId) |
void | setUniqueGroupId (uint uniqueGroupId) |
GroupType | type () const |
uint | uniqueGroupId () const |
![]() | |
void | appendPluginContactData (const QString &pluginId, const ContactData &data) |
void | clearPluginContactData () |
QString | icon (IconState state=None) const |
const IconMap | icons () const |
bool | loading () const |
QMap< QString, ContactDataList > | pluginContactData () const |
ContactDataList | pluginContactData (Plugin *plugin) const |
QMap< QString, QString > | pluginData (Plugin *plugin) const |
QString | pluginData (Plugin *plugin, const QString &key) const |
const PluginDataMap | pluginData () const |
void | setIcon (const QString &icon, IconState=None) |
void | setLoading (bool value) |
void | setPluginContactData (Plugin *plugin, const ContactDataList &dataList) |
void | setPluginData (Plugin *plugin, const QMap< QString, QString > &value) |
void | setPluginData (const QString &pluginId, const QMap< QString, QString > &pluginData) |
void | setPluginData (Plugin *plugin, const QString &key, const QString &value) |
void | setUseCustomIcon (bool useCustomIcon) |
bool | useCustomIcon () const |
![]() | |
PropertyContainer (QObject *parent=0) | |
virtual | ~PropertyContainer () |
void | deserializeProperties (const QMap< QString, QString > &serializedData) |
bool | hasProperty (const QString &key) const |
QStringList | properties () const |
const Kopete::Property & | property (const QString &key) const |
const Kopete::Property & | property (const Kopete::PropertyTmpl &tmpl) const |
void | removeProperty (const Kopete::PropertyTmpl &tmpl) |
void | serializeProperties (QMap< QString, QString > &serializedData) const |
void | setProperty (const Kopete::PropertyTmpl &tmpl, const QVariant &value) |
Static Public Member Functions | |
static Group * | offline () |
static Group * | temporary () |
static Group * | topLevel () |
Properties | |
QString | displayName |
bool | expanded |
uint | groupId |
Additional Inherited Members | |
![]() | |
ContactListElement (QObject *parent=0L) | |
~ContactListElement () | |
Detailed Description
Class which represents the Group.
A Group is a ConstacListElement which means plugin can save data.
some static group are availavle from this class: topLevel and temporary
Definition at line 44 of file kopetegroup.h.
Member Typedef Documentation
typedef QList<Group*> Kopete::Group::List |
Definition at line 53 of file kopetegroup.h.
Member Enumeration Documentation
Kinds of groups.
Enumerator | |
---|---|
Normal | |
Temporary | |
TopLevel | |
Offline |
Definition at line 56 of file kopetegroup.h.
Constructor & Destructor Documentation
Kopete::Group::Group | ( | ) |
Create an empty group.
Note that the constructor will not add the group automatically to the contact list. Use ContactList::addGroup() to add it
Definition at line 79 of file kopetegroup.cpp.
|
explicit |
Create a group of the specified type.
Overloaded constructor to create a group with a display name and Normal type.
Definition at line 61 of file kopetegroup.cpp.
Kopete::Group::~Group | ( | ) |
Definition at line 87 of file kopetegroup.cpp.
Member Function Documentation
QString Kopete::Group::displayName | ( | ) | const |
Return the group's display name.
- Returns
- the display name of the group
|
signal |
Emitted when the group has been renamed.
uint Kopete::Group::groupId | ( | ) | const |
- Returns
- the unique id for this group
bool Kopete::Group::isExpanded | ( | ) | const |
- Returns
- true if the group is expanded.
- false otherwise
Definition at line 137 of file kopetegroup.cpp.
QList< MetaContact * > Kopete::Group::members | ( | ) | const |
child metacontact This function is not very efficient - it searches through all the metacontacts in the contact list
- Returns
- the members of this group
Definition at line 98 of file kopetegroup.cpp.
|
static |
- Returns
- a Group pointer to the offline group
Definition at line 51 of file kopetegroup.cpp.
|
slot |
Send a message to all contacts in the group.
Definition at line 165 of file kopetegroup.cpp.
void Kopete::Group::setDisplayName | ( | const QString & | newName | ) |
Rename the group.
Definition at line 110 of file kopetegroup.cpp.
void Kopete::Group::setExpanded | ( | bool | expanded | ) |
Set if the group is expanded.
Definition at line 132 of file kopetegroup.cpp.
void Kopete::Group::setGroupId | ( | uint | groupId | ) |
Definition at line 150 of file kopetegroup.cpp.
void Kopete::Group::setUniqueGroupId | ( | uint | uniqueGroupId | ) |
Definition at line 160 of file kopetegroup.cpp.
|
static |
- Returns
- a Group pointer to the temporary group
Definition at line 43 of file kopetegroup.cpp.
|
static |
- Returns
- a Group pointer to the toplevel group
Definition at line 35 of file kopetegroup.cpp.
Group::GroupType Kopete::Group::type | ( | ) | const |
- Returns
- the group type
Definition at line 127 of file kopetegroup.cpp.
uint Kopete::Group::uniqueGroupId | ( | ) | const |
Definition at line 155 of file kopetegroup.cpp.
Property Documentation
|
readwrite |
Definition at line 46 of file kopetegroup.h.
|
readwrite |
Definition at line 48 of file kopetegroup.h.
|
read |
Definition at line 47 of file kopetegroup.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.