kopete/libkopete
#include <kopetecontactlist.h>
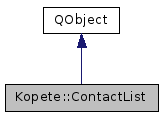
Public Slots | |
void | addGroup (Kopete::Group *) |
void | addGroups (QList< Group * > groups) |
void | addMetaContact (Kopete::MetaContact *c) |
void | addMetaContacts (QList< MetaContact * > metaContacts) |
void | load () |
bool | loaded () const |
void | mergeMetaContacts (QList< MetaContact * > src, Kopete::MetaContact *dst) |
void | removeGroup (Kopete::Group *) |
void | removeMetaContact (Kopete::MetaContact *contact) |
void | save () |
void | setSelectedItems (QList< MetaContact * > metaContacts, QList< Group * > groups) |
void | shutdown () |
Signals | |
void | contactListLoaded () |
void | groupAdded (Kopete::Group *) |
void | groupRemoved (Kopete::Group *) |
void | groupRenamed (Kopete::Group *, const QString &oldname) |
void | metaContactAdded (Kopete::MetaContact *mc) |
void | metaContactAddedToGroup (Kopete::MetaContact *mc, Kopete::Group *to) |
void | metaContactMovedToGroup (Kopete::MetaContact *mc, Kopete::Group *from, Kopete::Group *to) |
void | metaContactRemoved (Kopete::MetaContact *mc) |
void | metaContactRemovedFromGroup (Kopete::MetaContact *mc, Kopete::Group *from) |
void | metaContactSelected (bool) |
void | selectionChanged () |
Public Member Functions | |
~ContactList () | |
Contact * | findContact (const QString &protocolId, const QString &accountId, const QString &contactId) const |
Group * | findGroup (const QString &displayName, int type=0) |
MetaContact * | findMetaContactByContactId (const QString &contactId) const |
MetaContact * | findMetaContactByDisplayName (const QString &displayName) const |
Group * | group (unsigned int groupId) const |
QList< Group * > | groups () const |
MetaContact * | metaContact (const QString &metaContactId) const |
QList< MetaContact * > | metaContacts () const |
MetaContact * | myself () |
QList< Group * > | selectedGroups () const |
QList< MetaContact * > | selectedMetaContacts () const |
Static Public Member Functions | |
static ContactList * | self () |
Detailed Description
manage contacts and metacontact
The contactList is a singleton you can uses with ContactList::self()
it let you get a list of metacontact with metaContacts() Only metacontact which are on the contact list are returned.
Definition at line 49 of file kopetecontactlist.h.
Constructor & Destructor Documentation
Kopete::ContactList::~ContactList | ( | ) |
Definition at line 105 of file kopetecontactlist.cpp.
Member Function Documentation
|
slot |
Add a group each group must be added on the list after his creation.
Definition at line 302 of file kopetecontactlist.cpp.
|
slot |
Add groups each group must be added on the list after his creation.
Definition at line 296 of file kopetecontactlist.cpp.
|
slot |
Add the metacontact into the contact list When calling this method, the contact has to be already placed in the correct group.
If the contact is not in a group, it will be added to the top-level group. It is also better if the MetaContact could also be completely created, i.e: all contacts already in it
Definition at line 235 of file kopetecontactlist.cpp.
|
slot |
Add metacontacts into the contact list When calling this method, contacts have to be already placed in the correct group.
If contacts are not in a group, they will be added to the top-level group. It is also better if the MetaContacts could also be completely created, i.e: all contacts already in it
Definition at line 229 of file kopetecontactlist.cpp.
|
signal |
Contact * Kopete::ContactList::findContact | ( | const QString & | protocolId, |
const QString & | accountId, | ||
const QString & | contactId | ||
) | const |
find a contact in the contact list.
Browse in each metacontact of the list to find the contact with the given ID.
- Parameters
-
protocolId the Plugin::pluginId() of the protocol ("MSNProtocol") accountId the Account::accountId() contactId the Contact::contactId()
- Returns
- the contact with the parameters, or 0L if not found.
Definition at line 156 of file kopetecontactlist.cpp.
Group * Kopete::ContactList::findGroup | ( | const QString & | displayName, |
int | type = 0 |
||
) |
find a group with his displayName If a group already exists with the given name and the given type, the existing group will be returned.
Otherwise, a new group will be created.
- Parameters
-
displayName is the display name to search type is the Group::GroupType to search, the default value is group::Normal
- Returns
- always a valid Group
Definition at line 196 of file kopetecontactlist.cpp.
MetaContact * Kopete::ContactList::findMetaContactByContactId | ( | const QString & | contactId | ) | const |
Find a meta contact by its contact id.
Returns the first match.
Definition at line 182 of file kopetecontactlist.cpp.
MetaContact * Kopete::ContactList::findMetaContactByDisplayName | ( | const QString & | displayName | ) | const |
Find a contact by display name.
Returns the first match.
Definition at line 170 of file kopetecontactlist.cpp.
Group * Kopete::ContactList::group | ( | unsigned int | groupId | ) | const |
return the group with the given unique id.
if none is found return 0L
Definition at line 139 of file kopetecontactlist.cpp.
|
signal |
A group has just been added.
|
signal |
A group has just been removed.
|
signal |
A group has just been renamed.
QList< Group * > Kopete::ContactList::groups | ( | ) | const |
- Returns
- all groups
Definition at line 118 of file kopetecontactlist.cpp.
|
slot |
Load the contact list
Definition at line 357 of file kopetecontactlist.cpp.
|
slot |
Definition at line 379 of file kopetecontactlist.cpp.
|
slot |
Merge one or more metacontacts into another one.
Definition at line 279 of file kopetecontactlist.cpp.
MetaContact * Kopete::ContactList::metaContact | ( | const QString & | metaContactId | ) | const |
Return the metacontact referenced by the given id.
is none is found, return 0L
- See also
- MetaContact::metaContactId()
Definition at line 124 of file kopetecontactlist.cpp.
|
signal |
A meta contact was added to the contact list.
Interested classes ( like the listview widgets ) can connect to this signal to receive the newly added contacts.
|
signal |
A contact has been added to a group.
|
signal |
A contact has been moved from one group to another.
|
signal |
A metacontact has just been removed.
and will be soon deleted
|
signal |
A contact has been removed from a group.
QList< MetaContact * > Kopete::ContactList::metaContacts | ( | ) | const |
return a list of all metacontact of the contact list Retrieve the list of all available meta contacts.
The returned QPtrList is not the internally used variable, so changes to it won't propagate into the actual contact list. This can be useful if you need a subset of the contact list, because you can simply filter the result set as you wish without worrying about side effects. The contained MetaContacts are obviously not duplicates, so changing those will have the expected result :-)
Definition at line 112 of file kopetecontactlist.cpp.
|
signal |
This signal is emitted each time the selection has changed.
the bool is set to true if only one meta contact has been selected, and set to false if none, or several contacts are selected you can connect this signal to KAction::setEnabled if you have an action which is applied to only one contact
MetaContact * Kopete::ContactList::myself | ( | ) |
return the metacontact that represent the user itself.
This metacontact should be the parent of every Kopete::Account::myself() contacts.
This metacontact is not in the contact list.
Definition at line 349 of file kopetecontactlist.cpp.
|
slot |
Remove a group this method delete the group.
Definition at line 312 of file kopetecontactlist.cpp.
|
slot |
Remove a metacontact from the contact list.
This method delete itself the metacontact.
Definition at line 251 of file kopetecontactlist.cpp.
|
slot |
Definition at line 384 of file kopetecontactlist.cpp.
QList< Group * > Kopete::ContactList::selectedGroups | ( | ) | const |
return the list of groups actualy selected in the contact list UI
Definition at line 224 of file kopetecontactlist.cpp.
QList< MetaContact * > Kopete::ContactList::selectedMetaContacts | ( | ) | const |
return the list of metacontact actually selected in the contact list UI
Definition at line 219 of file kopetecontactlist.cpp.
|
signal |
This signal is emit when the selection has changed, it is emitted after the following slot Warning: Do not delete any contacts in slots connected to this signal.
(it is the warning in the QListView::selectionChanged() doc)
|
static |
The contact list is a singleton object.
Use this method to retrieve the instance.
Definition at line 71 of file kopetecontactlist.cpp.
|
slot |
Set which items are selected in the ContactList GUI.
This method has to be called by the contact list UI side. it stores the selected items, and emits signals
Definition at line 339 of file kopetecontactlist.cpp.
|
slot |
Save and shutdown the contact list
Definition at line 417 of file kopetecontactlist.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.