kopete/libkopete
#include <kopeteidentity.h>
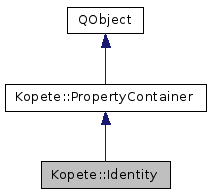
Public Types | |
typedef QList< Identity * > | List |
Public Slots | |
void | removeAccount (const Kopete::Account *account) |
void | setOnlineStatus (uint category, const Kopete::StatusMessage &statusMessage) |
void | setStatusMessage (const Kopete::StatusMessage &statusMessage) |
void | updateOnlineStatus () |
Signals | |
void | identityChanged (Kopete::Identity *identity) |
void | identityDestroyed (const Kopete::Identity *identity) |
void | onlineStatusChanged (Kopete::Identity *) |
void | toolTipChanged (Kopete::Identity *) |
![]() | |
void | propertyChanged (Kopete::PropertyContainer *container, const QString &key, const QVariant &oldValue, const QVariant &newValue) |
Public Member Functions | |
Identity (const QString &label) | |
Identity (const QString &id, const QString &label) | |
~Identity () | |
QList< Account * > | accounts () const |
void | addAccount (Kopete::Account *account) |
Identity * | clone () const |
KConfigGroup * | configGroup () const |
QString | customIcon () const |
bool | excludeConnect () const |
QString | id () const |
QString | label () const |
void | load () |
OnlineStatus::StatusType | onlineStatus () const |
void | save () |
void | setLabel (const QString &label) |
Kopete::StatusMessage | statusMessage () const |
QString | toolTip () const |
![]() | |
PropertyContainer (QObject *parent=0) | |
virtual | ~PropertyContainer () |
void | deserializeProperties (const QMap< QString, QString > &serializedData) |
bool | hasProperty (const QString &key) const |
QStringList | properties () const |
const Kopete::Property & | property (const QString &key) const |
const Kopete::Property & | property (const Kopete::PropertyTmpl &tmpl) const |
void | removeProperty (const Kopete::PropertyTmpl &tmpl) |
void | serializeProperties (QMap< QString, QString > &serializedData) const |
void | setProperty (const Kopete::PropertyTmpl &tmpl, const QVariant &value) |
Protected Slots | |
void | slotSaveProperty (Kopete::PropertyContainer *container, const QString &key, const QVariant &oldValue, const QVariant &newValue) |
Detailed Description
An identity that might contain one or more accounts associated to it
Definition at line 41 of file kopeteidentity.h.
Member Typedef Documentation
typedef QList<Identity*> Kopete::Identity::List |
Definition at line 45 of file kopeteidentity.h.
Constructor & Destructor Documentation
Kopete::Identity::Identity | ( | const QString & | label | ) |
The main constructor for Kopete Identities.
This will create an empty identity with a random id
- Parameters
-
label the label to use for this identity
Definition at line 64 of file kopeteidentity.cpp.
Kopete::Identity::Identity | ( | const QString & | id, |
const QString & | label | ||
) |
Constructor for deserialising stored Identities.
Definition at line 56 of file kopeteidentity.cpp.
Kopete::Identity::~Identity | ( | ) |
Definition at line 83 of file kopeteidentity.cpp.
Member Function Documentation
QList< Account * > Kopete::Identity::accounts | ( | ) | const |
Returns the accounts assigned to this identity.
Definition at line 189 of file kopeteidentity.cpp.
void Kopete::Identity::addAccount | ( | Kopete::Account * | account | ) |
Adds an account to the identity.
- Parameters
-
account the account to be added
Definition at line 194 of file kopeteidentity.cpp.
Identity * Kopete::Identity::clone | ( | ) | const |
Duplicates an existing identity.
This will create a new identity name
- Parameters
-
id,that clones all properties
- Returns
- duplicate Identity
Definition at line 71 of file kopeteidentity.cpp.
KConfigGroup * Kopete::Identity::configGroup | ( | ) | const |
Returns the KConfigGroup that should be used to read/write settings of this identity.
Definition at line 229 of file kopeteidentity.cpp.
QString Kopete::Identity::customIcon | ( | ) | const |
Return the icon for this identity.
Definition at line 180 of file kopeteidentity.cpp.
bool Kopete::Identity::excludeConnect | ( | ) | const |
This identity should be connected when connect all is called?
Definition at line 107 of file kopeteidentity.cpp.
QString Kopete::Identity::id | ( | ) | const |
The id is a unique internal handle and should not be exposed in the UI.
- Returns
- the identity's id
Definition at line 91 of file kopeteidentity.cpp.
|
signal |
|
signal |
QString Kopete::Identity::label | ( | ) | const |
The label is used to identify the identity in the UI.
- Returns
- the identity's label
Definition at line 96 of file kopeteidentity.cpp.
void Kopete::Identity::load | ( | ) |
Load the identity information.
Definition at line 234 of file kopeteidentity.cpp.
OnlineStatus::StatusType Kopete::Identity::onlineStatus | ( | ) | const |
Get the online status of the identity.
- Returns
- the online status of the identity
Definition at line 142 of file kopeteidentity.cpp.
|
signal |
|
slot |
Removes an account from the identity.
- Parameters
-
account the account to be removed
Definition at line 216 of file kopeteidentity.cpp.
void Kopete::Identity::save | ( | ) |
Save the identity information.
Definition at line 242 of file kopeteidentity.cpp.
void Kopete::Identity::setLabel | ( | const QString & | label | ) |
Sets the label.
- Parameters
-
newLabel a new label for the identity
Definition at line 101 of file kopeteidentity.cpp.
|
slot |
Sets the online status for this identity Sets the online status for each account in this identity, except those which are set to 'Exclude from connect all' (Kopete::Account::excludeConnect()).
- Parameters
-
category generic OnlineStatusManager::Categories identifying status to set statusMessage is the new status message to use in this onlinestatus
Definition at line 113 of file kopeteidentity.cpp.
|
slot |
Sets the status message for this identity Sets the status message for each account in this identity, except those which are set to 'Exclude from connect all' (Kopete::Account::excludeConnect()).
- Parameters
-
statusMessage is the new status message to use
Definition at line 126 of file kopeteidentity.cpp.
|
protectedslot |
Definition at line 276 of file kopeteidentity.cpp.
Kopete::StatusMessage Kopete::Identity::statusMessage | ( | ) | const |
Get the current status message of the identity.
Definition at line 147 of file kopeteidentity.cpp.
QString Kopete::Identity::toolTip | ( | ) | const |
Get the tooltip for this identity.
- Returns
- an RTF tooltip depending on Kopete::AppearanceSettings settings
Definition at line 152 of file kopeteidentity.cpp.
|
signal |
|
slot |
Definition at line 254 of file kopeteidentity.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:53:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.