calendarsupport
#include <kcalprefs_base.h>
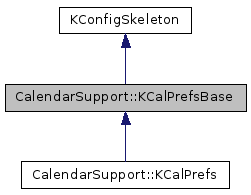
Public Types | |
enum | EnumArchiveAction { actionDelete, actionArchive } |
enum | EnumExpiryUnit { UnitDays, UnitWeeks, UnitMonths } |
Public Member Functions | |
KCalPrefsBase () | |
~KCalPrefsBase () | |
QStringList | activeDesignerFields () const |
ItemStringList * | activeDesignerFieldsItem () |
QStringList | additionalMails () const |
ItemStringList * | additionalMailsItem () |
int | archiveAction () const |
ItemEnum * | archiveActionItem () |
bool | archiveEvents () const |
ItemBool * | archiveEventsItem () |
QString | archiveFile () const |
ItemString * | archiveFileItem () |
bool | archiveTodos () const |
ItemBool * | archiveTodosItem () |
QString | audioFilePath () const |
ItemPath * | audioFilePathItem () |
bool | autoArchive () const |
ItemBool * | autoArchiveItem () |
bool | defaultAudioFileReminders () const |
ItemBool * | defaultAudioFileRemindersItem () |
QDateTime | defaultDuration () const |
ItemDateTime * | defaultDurationItem () |
bool | defaultEventReminders () const |
ItemBool * | defaultEventRemindersItem () |
bool | defaultTodoReminders () const |
ItemBool * | defaultTodoRemindersItem () |
bool | emailControlCenter () const |
ItemBool * | emailControlCenterItem () |
QStringList | eventTemplates () const |
ItemStringList * | eventTemplatesItem () |
bool | excludeHolidays () const |
ItemBool * | excludeHolidaysItem () |
int | expiryTime () const |
ItemInt * | expiryTimeItem () |
int | expiryUnit () const |
ItemEnum * | expiryUnitItem () |
QString | holidays () const |
ItemString * | holidaysItem () |
QStringList | journalTemplates () const |
ItemStringList * | journalTemplatesItem () |
int | reminderTime () const |
ItemInt * | reminderTimeItem () |
int | reminderTimeUnits () const |
ItemInt * | reminderTimeUnitsItem () |
void | setActiveDesignerFields (const QStringList &v) |
void | setAdditionalMails (const QStringList &v) |
void | setArchiveAction (int v) |
void | setArchiveEvents (bool v) |
void | setArchiveFile (const QString &v) |
void | setArchiveTodos (bool v) |
void | setAudioFilePath (const QString &v) |
void | setAutoArchive (bool v) |
void | setDefaultAudioFileReminders (bool v) |
void | setDefaultDuration (const QDateTime &v) |
void | setDefaultEventReminders (bool v) |
void | setDefaultTodoReminders (bool v) |
void | setEmailControlCenter (bool v) |
void | setEventTemplates (const QStringList &v) |
void | setExcludeHolidays (bool v) |
void | setExpiryTime (int v) |
void | setExpiryUnit (int v) |
void | setHolidays (const QString &v) |
void | setJournalTemplates (const QStringList &v) |
void | setReminderTime (int v) |
void | setReminderTimeUnits (int v) |
void | setShowTimeZoneSelectorInIncidenceEditor (bool v) |
void | setStartTime (const QDateTime &v) |
void | setTodoTemplates (const QStringList &v) |
void | setUnsetCategoryColor (const QColor &v) |
void | setUseGroupwareCommunication (bool v) |
void | setUserEmail (const QString &v) |
void | setUserName (const QString &v) |
void | setWorkWeekMask (int v) |
bool | showTimeZoneSelectorInIncidenceEditor () const |
ItemBool * | showTimeZoneSelectorInIncidenceEditorItem () |
QDateTime | startTime () const |
ItemDateTime * | startTimeItem () |
QStringList | todoTemplates () const |
ItemStringList * | todoTemplatesItem () |
QColor | unsetCategoryColor () const |
ItemColor * | unsetCategoryColorItem () |
bool | useGroupwareCommunication () const |
ItemBool * | useGroupwareCommunicationItem () |
QString | userEmail () const |
ItemString * | userEmailItem () |
QString | userName () const |
ItemString * | userNameItem () |
int | workWeekMask () const |
ItemInt * | workWeekMaskItem () |
Public Attributes | |
QStringList | mActiveDesignerFields |
QStringList | mAdditionalMails |
int | mArchiveAction |
bool | mArchiveEvents |
QString | mArchiveFile |
bool | mArchiveTodos |
QString | mAudioFilePath |
bool | mAutoArchive |
bool | mDefaultAudioFileReminders |
QDateTime | mDefaultDuration |
bool | mDefaultEventReminders |
bool | mDefaultTodoReminders |
bool | mEmailControlCenter |
QStringList | mEventTemplates |
bool | mExcludeHolidays |
int | mExpiryTime |
int | mExpiryUnit |
QString | mHolidays |
QStringList | mJournalTemplates |
int | mReminderTime |
int | mReminderTimeUnits |
bool | mShowTimeZoneSelectorInIncidenceEditor |
QDateTime | mStartTime |
QStringList | mTodoTemplates |
QColor | mUnsetCategoryColor |
bool | mUseGroupwareCommunication |
QString | mUserEmail |
QString | mUserName |
int | mWorkWeekMask |
Detailed Description
Definition at line 15 of file kcalprefs_base.h.
Member Enumeration Documentation
Enumerator | |
---|---|
actionDelete | |
actionArchive |
Definition at line 19 of file kcalprefs_base.h.
Enumerator | |
---|---|
UnitDays | |
UnitWeeks | |
UnitMonths |
Definition at line 18 of file kcalprefs_base.h.
Constructor & Destructor Documentation
KCalPrefsBase::KCalPrefsBase | ( | ) |
Definition at line 10 of file kcalprefs_base.cpp.
KCalPrefsBase::~KCalPrefsBase | ( | ) |
Definition at line 177 of file kcalprefs_base.cpp.
Member Function Documentation
|
inline |
Get ActiveDesignerFields.
Definition at line 711 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ActiveDesignerFields()
Definition at line 719 of file kcalprefs_base.h.
|
inline |
Get AdditionalMails.
Definition at line 336 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to AdditionalMails()
Definition at line 344 of file kcalprefs_base.h.
|
inline |
Get What to do when archiving.
Definition at line 536 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ArchiveAction()
Definition at line 544 of file kcalprefs_base.h.
|
inline |
Get Archive events.
Definition at line 486 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ArchiveEvents()
Definition at line 494 of file kcalprefs_base.h.
|
inline |
Get URL of the file where old events should be archived.
Definition at line 461 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ArchiveFile()
Definition at line 469 of file kcalprefs_base.h.
|
inline |
Get Archive to-dos.
Definition at line 511 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ArchiveTodos()
Definition at line 519 of file kcalprefs_base.h.
|
inline |
Get Default audio file.
Definition at line 136 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to audioFilePath()
Definition at line 144 of file kcalprefs_base.h.
|
inline |
Get Regularly archive events.
Definition at line 386 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to AutoArchive()
Definition at line 394 of file kcalprefs_base.h.
|
inline |
Get Enable a default sound file for audio reminders.
Definition at line 111 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to defaultAudioFileReminders()
Definition at line 119 of file kcalprefs_base.h.
|
inline |
Get Default duration of new appointment (HH:MM)
Definition at line 86 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to DefaultDuration()
Definition at line 94 of file kcalprefs_base.h.
|
inline |
Get Enable reminders for new Events.
Definition at line 161 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to defaultEventReminders()
Definition at line 169 of file kcalprefs_base.h.
|
inline |
Get Enable reminders for new To-dos.
Definition at line 186 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to defaultTodoReminders()
Definition at line 194 of file kcalprefs_base.h.
|
inline |
Get Use email settings from System Settings.
Definition at line 36 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to EmailControlCenter()
Definition at line 44 of file kcalprefs_base.h.
|
inline |
Get EventTemplates.
Definition at line 636 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to EventTemplates()
Definition at line 644 of file kcalprefs_base.h.
|
inline |
Get Exclude holidays.
Definition at line 261 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ExcludeHolidays()
Definition at line 269 of file kcalprefs_base.h.
|
inline |
Get If auto-archiving is enabled, events older than this amount will be archived.
The unit of this value is specified in another field.
Definition at line 411 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ExpiryTime()
Definition at line 419 of file kcalprefs_base.h.
|
inline |
Get The unit in which the expiry time is expressed.
Definition at line 436 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ExpiryUnit()
Definition at line 444 of file kcalprefs_base.h.
|
inline |
Get Use holiday region:
Definition at line 286 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to Holidays()
Definition at line 294 of file kcalprefs_base.h.
|
inline |
Get JournalTemplates.
Definition at line 686 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to JournalTemplates()
Definition at line 694 of file kcalprefs_base.h.
|
inline |
Get Default reminder time.
Definition at line 211 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ReminderTime()
Definition at line 219 of file kcalprefs_base.h.
|
inline |
Get Default Reminder Time Units.
Definition at line 236 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ReminderTimeUnits()
Definition at line 244 of file kcalprefs_base.h.
|
inline |
Set ActiveDesignerFields.
Definition at line 702 of file kcalprefs_base.h.
|
inline |
Set AdditionalMails.
Definition at line 327 of file kcalprefs_base.h.
|
inline |
Set What to do when archiving.
Definition at line 527 of file kcalprefs_base.h.
|
inline |
Set Archive events.
Definition at line 477 of file kcalprefs_base.h.
|
inline |
Set URL of the file where old events should be archived.
Definition at line 452 of file kcalprefs_base.h.
|
inline |
Set Archive to-dos.
Definition at line 502 of file kcalprefs_base.h.
|
inline |
Set Default audio file.
Definition at line 127 of file kcalprefs_base.h.
|
inline |
Set Regularly archive events.
Definition at line 377 of file kcalprefs_base.h.
|
inline |
Set Enable a default sound file for audio reminders.
Definition at line 102 of file kcalprefs_base.h.
|
inline |
Set Default duration of new appointment (HH:MM)
Definition at line 77 of file kcalprefs_base.h.
|
inline |
Set Enable reminders for new Events.
Definition at line 152 of file kcalprefs_base.h.
|
inline |
Set Enable reminders for new To-dos.
Definition at line 177 of file kcalprefs_base.h.
|
inline |
Set Use email settings from System Settings.
Definition at line 27 of file kcalprefs_base.h.
|
inline |
Set EventTemplates.
Definition at line 627 of file kcalprefs_base.h.
|
inline |
Set Exclude holidays.
Definition at line 252 of file kcalprefs_base.h.
|
inline |
Set If auto-archiving is enabled, events older than this amount will be archived.
The unit of this value is specified in another field.
Definition at line 402 of file kcalprefs_base.h.
|
inline |
Set The unit in which the expiry time is expressed.
Definition at line 427 of file kcalprefs_base.h.
|
inline |
Set Use holiday region:
Definition at line 277 of file kcalprefs_base.h.
|
inline |
Set JournalTemplates.
Definition at line 677 of file kcalprefs_base.h.
|
inline |
Set Default reminder time.
Definition at line 202 of file kcalprefs_base.h.
|
inline |
Set Default Reminder Time Units.
Definition at line 227 of file kcalprefs_base.h.
|
inline |
Set Show timezone selectors in the event and todo editor dialog.
Definition at line 352 of file kcalprefs_base.h.
|
inline |
Set Default appointment time.
Definition at line 52 of file kcalprefs_base.h.
|
inline |
Set TodoTemplates.
Definition at line 652 of file kcalprefs_base.h.
|
inline |
Set "No category" color (for "Only category" drawing schemes)
Definition at line 727 of file kcalprefs_base.h.
|
inline |
Set Use Groupware communication.
Definition at line 302 of file kcalprefs_base.h.
|
inline |
Set E&mail address.
Definition at line 602 of file kcalprefs_base.h.
|
inline |
Set Full &name.
Definition at line 577 of file kcalprefs_base.h.
|
inline |
Set Work Week Mask.
Definition at line 552 of file kcalprefs_base.h.
|
inline |
Get Show timezone selectors in the event and todo editor dialog.
Definition at line 361 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to ShowTimeZoneSelectorInIncidenceEditor()
Definition at line 369 of file kcalprefs_base.h.
|
inline |
Get Default appointment time.
Definition at line 61 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to StartTime()
Definition at line 69 of file kcalprefs_base.h.
|
inline |
Get TodoTemplates.
Definition at line 661 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to TodoTemplates()
Definition at line 669 of file kcalprefs_base.h.
|
inline |
Get "No category" color (for "Only category" drawing schemes)
Definition at line 736 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to UnsetCategoryColor()
Definition at line 744 of file kcalprefs_base.h.
|
inline |
Get Use Groupware communication.
Definition at line 311 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to UseGroupwareCommunication()
Definition at line 319 of file kcalprefs_base.h.
|
inline |
Get E&mail address.
Definition at line 611 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to UserEmail()
Definition at line 619 of file kcalprefs_base.h.
|
inline |
Get Full &name.
Definition at line 586 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to UserName()
Definition at line 594 of file kcalprefs_base.h.
|
inline |
Get Work Week Mask.
Definition at line 561 of file kcalprefs_base.h.
|
inline |
Get Item object corresponding to WorkWeekMask()
Definition at line 569 of file kcalprefs_base.h.
Member Data Documentation
QStringList CalendarSupport::KCalPrefsBase::mActiveDesignerFields |
Definition at line 792 of file kcalprefs_base.h.
QStringList CalendarSupport::KCalPrefsBase::mAdditionalMails |
Definition at line 771 of file kcalprefs_base.h.
int CalendarSupport::KCalPrefsBase::mArchiveAction |
Definition at line 783 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mArchiveEvents |
Definition at line 781 of file kcalprefs_base.h.
QString CalendarSupport::KCalPrefsBase::mArchiveFile |
Definition at line 780 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mArchiveTodos |
Definition at line 782 of file kcalprefs_base.h.
QString CalendarSupport::KCalPrefsBase::mAudioFilePath |
Definition at line 759 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mAutoArchive |
Definition at line 777 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mDefaultAudioFileReminders |
Definition at line 758 of file kcalprefs_base.h.
QDateTime CalendarSupport::KCalPrefsBase::mDefaultDuration |
Definition at line 757 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mDefaultEventReminders |
Definition at line 760 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mDefaultTodoReminders |
Definition at line 761 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mEmailControlCenter |
Definition at line 753 of file kcalprefs_base.h.
QStringList CalendarSupport::KCalPrefsBase::mEventTemplates |
Definition at line 789 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mExcludeHolidays |
Definition at line 766 of file kcalprefs_base.h.
int CalendarSupport::KCalPrefsBase::mExpiryTime |
Definition at line 778 of file kcalprefs_base.h.
int CalendarSupport::KCalPrefsBase::mExpiryUnit |
Definition at line 779 of file kcalprefs_base.h.
QString CalendarSupport::KCalPrefsBase::mHolidays |
Definition at line 767 of file kcalprefs_base.h.
QStringList CalendarSupport::KCalPrefsBase::mJournalTemplates |
Definition at line 791 of file kcalprefs_base.h.
int CalendarSupport::KCalPrefsBase::mReminderTime |
Definition at line 762 of file kcalprefs_base.h.
int CalendarSupport::KCalPrefsBase::mReminderTimeUnits |
Definition at line 763 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mShowTimeZoneSelectorInIncidenceEditor |
Definition at line 774 of file kcalprefs_base.h.
QDateTime CalendarSupport::KCalPrefsBase::mStartTime |
Definition at line 756 of file kcalprefs_base.h.
QStringList CalendarSupport::KCalPrefsBase::mTodoTemplates |
Definition at line 790 of file kcalprefs_base.h.
QColor CalendarSupport::KCalPrefsBase::mUnsetCategoryColor |
Definition at line 795 of file kcalprefs_base.h.
bool CalendarSupport::KCalPrefsBase::mUseGroupwareCommunication |
Definition at line 770 of file kcalprefs_base.h.
QString CalendarSupport::KCalPrefsBase::mUserEmail |
Definition at line 788 of file kcalprefs_base.h.
QString CalendarSupport::KCalPrefsBase::mUserName |
Definition at line 787 of file kcalprefs_base.h.
int CalendarSupport::KCalPrefsBase::mWorkWeekMask |
Definition at line 786 of file kcalprefs_base.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:54:59 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.