console/kabcclient
#include <kabcclient.h>
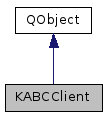
Public Types | |
enum | Operation { List = 0, Add, Remove, Merge, Search } |
Public Member Functions | |
KABCClient (Operation operation, FormatFactory *factory) | |
virtual | ~KABCClient () |
bool | initOperation () |
void | setAllowSaving (bool on) |
bool | setInputCodec (const QByteArray &name) |
bool | setInputFormat (const QByteArray &name) |
bool | setInputOptions (const QByteArray &options) |
void | setInputStream (std::istream *stream) |
void | setMatchCaseSensitivity (Qt::CaseSensitivity sensitivity) |
bool | setOutputCodec (const QByteArray &name) |
bool | setOutputFormat (const QByteArray &name) |
bool | setOutputOptions (const QByteArray &options) |
Detailed Description
Main handler of the program.
This class is the "program", it gets configured with the options passed by the user at the command line, retrieves the necessary components from its factories (see FormatFactory) and then executes the desired Operation
Definition at line 50 of file kabcclient.h.
Member Enumeration Documentation
List of supported operations.
Enumerator | |
---|---|
List |
Writes all contacts of the address book. Does not consume any input |
Add |
Adds the input to the address book. Reads contacts from the input stream in a loop and tries to add each one to the address book. Writes the each contacts data to the output stream.
|
Remove |
Removes matching contact from the address book. Reads contacts from the input stream in a loop and checks for each which entries in the address book match. If there is more than one match, it will not remove any of them. Else (only one match) it will remove the match from the address book. Can use SearchInput and DialogInput Writes the remove contact's data to the output stream
|
Merge |
Merges input data into the address book. Reads contacts from the input stream in a loop and checks for each which entries in the address book match. If there is more than one match, it will not attempt to merge. Else (only one match) it will use the found contact and merge information from the input contact into it and then replace the one inside the address book with the merged one. Writes the merged contact's data to the output stream.
|
Search |
Searches for matching entries in the address book. Reads contacts from the input stream in a loop and checks for each which entries in the address book match. Can use SearchInput and DialogInput Writes all matches per input to the output stream. |
Definition at line 58 of file kabcclient.h.
Constructor & Destructor Documentation
KABCClient::KABCClient | ( | Operation | operation, |
FormatFactory * | factory | ||
) |
Creates and initializes the instance.
- Parameters
-
operation the operation to perform on the address book factory the factory to get the input and output format handlers from
Definition at line 54 of file kabcclient.cpp.
|
virtual |
Destroys the instance.
Definition at line 71 of file kabcclient.cpp.
Member Function Documentation
bool KABCClient::initOperation | ( | ) |
Checks if Operation setup is correct and schedules execution.
Loads the KABC::StandardAddressBook syncronously and deactivates its "auto save", so the user can operate the program in "simulate" mode
- Returns
true
when Operation can start, otherwisefalse
Definition at line 174 of file kabcclient.cpp.
|
inline |
Sets the save behavior.
When saving is on
to address book data will be written to the address book storage, otherwise they will just be performed on the in-memory data.
Default is true
- Parameters
-
on when true
write data to address book store, whenfalse
operate in "simulate" mode
Definition at line 277 of file kabcclient.h.
bool KABCClient::setInputCodec | ( | const QByteArray & | name | ) |
Sets the text codec for reading the input data.
Translates utf
, utf8
, utf-8
to UTF-8
and local
, locale
the codec for the current locale.
- Parameters
-
name the name of the QTextCodec. See QTextCodec::codecForName()
- Returns
- return value of QTextCodec::codecForName()
- See also
- setInputFormat()
Definition at line 139 of file kabcclient.cpp.
bool KABCClient::setInputFormat | ( | const QByteArray & | name | ) |
Sets the input format to use.
Checks if the given name
is a valid input format for the Operation specified at the construction. If it is not blacklisted, the respective InputFormat will be retrieved from the FormatFactory.
- Parameters
-
name the name of an InputFormat to use for parsing the input data
- Returns
true
if the format is allowed for the selected Operation and its format parser can be created, otherwisefalse
Definition at line 79 of file kabcclient.cpp.
bool KABCClient::setInputOptions | ( | const QByteArray & | options | ) |
Sets the options for the input format.
Passes the options
to the InputFormat set with setInputFormat()
- Parameters
-
options the options for the InputFormat
- Returns
true
if the InputFormat accepts the options,false
if it doesn't or if there is not InputFormat set
Definition at line 119 of file kabcclient.cpp.
void KABCClient::setInputStream | ( | std::istream * | stream | ) |
Sets the input stream to read data from.
Depending on the input mode this can be either a stringstream
on the additional command arguments or cin
- Parameters
-
stream the input stream for reading
Definition at line 167 of file kabcclient.cpp.
|
inline |
Sets the string matching mode.
Default if Qt::CaseInsensitive
- Parameters
-
sensitivity whether to do string comparisons case sensitive or not
Definition at line 260 of file kabcclient.h.
bool KABCClient::setOutputCodec | ( | const QByteArray & | name | ) |
Sets the text codec for writing the output data.
Translates utf
, utf8
, utf-8
to UTF-8
and local
, locale
the codec for the current locale.
- Parameters
-
name the name of the QTextCodec. See QTextCodec::codecForName()
- Returns
- return value of QTextCodec::codecForName()
- See also
- setOutputFormat()
Definition at line 154 of file kabcclient.cpp.
bool KABCClient::setOutputFormat | ( | const QByteArray & | name | ) |
Sets the output format to use.
Retrieves the respective OutputFormat from the FormatFactory
- Parameters
-
name the name of an OutputFormat to use for formatting the output data
- Returns
true
if the format has been created, otherwisefalse
Definition at line 110 of file kabcclient.cpp.
bool KABCClient::setOutputOptions | ( | const QByteArray & | options | ) |
Sets the options for the output format.
Passes the options
to the OutputFormat set with setOutputFormat()
- Parameters
-
options the options for the OutputFormat
- Returns
true
if the OutputFormat accepts the options,false
if it doesn't or if there is not OutputFormat set
Definition at line 130 of file kabcclient.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.