kaddressbook
#include <qcsvreader.h>
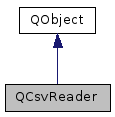
Public Member Functions | |
QCsvReader (QCsvBuilderInterface *builder) | |
~QCsvReader () | |
QChar | delimiter () const |
bool | read (QIODevice *device) |
void | setDelimiter (const QChar &delimiter) |
void | setStartRow (uint startRow) |
void | setTextCodec (QTextCodec *textCodec) |
void | setTextQuote (const QChar &textQuote) |
uint | startRow () const |
void | terminate () |
QTextCodec * | textCodec () const |
QChar | textQuote () const |
Properties | |
QChar | delimiter |
uint | startRow |
QChar | textQuote |
Detailed Description
A parser for comma separated value data.
QCsvReader is a class that reads a comma separated value list (csv) from a device and parses it into its fields. The parsed data are passed to a QCsvBuilderInterface instance, which can build up arbitrary data structures from it.
Definition at line 91 of file qcsvreader.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new csv reader.
- Parameters
-
builder The builder to use.
Definition at line 78 of file qcsvreader.cpp.
QCsvReader::~QCsvReader | ( | ) |
Destroys the csv reader.
Definition at line 84 of file qcsvreader.cpp.
Member Function Documentation
QChar QCsvReader::delimiter | ( | ) | const |
Returns the delimiter that is used as delimiter for fields.
bool QCsvReader::read | ( | QIODevice * | device | ) |
Parses the csv data from device
.
- Returns
- true on success, false otherwise.
We use the following state machine to parse CSV:
digraph { StartLine -> StartLine [label="\\r\\n"] StartLine -> QuotedField [label="Quote"] StartLine -> EmptyField [label="Delimiter"] StartLine -> NormalField [label="Other Char"]
QuotedField -> QuotedField [label="\\r\\n"] QuotedField -> QuotedFieldEnd [label="Quote"] QuotedField -> QuotedField [label="Delimiter"] QuotedField -> QuotedField [label="Other Char"]
QuotedFieldEnd -> StartLine [label="\\r\\n"] QuotedFieldEnd -> QuotedField [label="Quote"] QuotedFieldEnd -> EmptyField [label="Delimiter"] QuotedFieldEnd -> EmptyField [label="Other Char"]
EmptyField -> StartLine [label="\\r\\n"] EmptyField -> QuotedField [label="Quote"] EmptyField -> EmptyField [label="Delimiter"] EmptyField -> NormalField [label="Other Char"]
NormalField -> StartLine [label="\\r\\n"] NormalField -> NormalField [label="Quote"] NormalField -> EmptyField [label="Delimiter"] NormalField -> NormalField [label="Other Char"] }
Definition at line 89 of file qcsvreader.cpp.
void QCsvReader::setDelimiter | ( | const QChar & | delimiter | ) |
Sets the character that is used as delimiter for fields.
The default is ' '.
Definition at line 277 of file qcsvreader.cpp.
void QCsvReader::setStartRow | ( | uint | startRow | ) |
Sets the row from where the parsing shall be started.
Some csv files have some kind of header in the first line with the column titles. To retrieve only the real data, set the start row to '1' in this case.
The default start row is 0.
Definition at line 287 of file qcsvreader.cpp.
void QCsvReader::setTextCodec | ( | QTextCodec * | textCodec | ) |
Sets the text codec that shall be used for parsing the csv list.
The default is the system locale.
Definition at line 297 of file qcsvreader.cpp.
void QCsvReader::setTextQuote | ( | const QChar & | textQuote | ) |
Sets the character that is used for quoting.
The default is '"'.
Definition at line 267 of file qcsvreader.cpp.
uint QCsvReader::startRow | ( | ) | const |
Returns the start row.
void QCsvReader::terminate | ( | ) |
Terminates the parsing of the csv data.
Definition at line 307 of file qcsvreader.cpp.
QTextCodec * QCsvReader::textCodec | ( | ) | const |
Returns the text codec that is used for parsing the csv list.
Definition at line 302 of file qcsvreader.cpp.
QChar QCsvReader::textQuote | ( | ) | const |
Returns the character that is used for quoting.
Property Documentation
|
readwrite |
Definition at line 96 of file qcsvreader.h.
|
readwrite |
Definition at line 97 of file qcsvreader.h.
|
readwrite |
Definition at line 95 of file qcsvreader.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.