kalarm/lib
#include <messagebox.h>
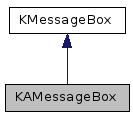
Public Types | |
enum | AskType { CONT_CANCEL_DEF_CONT, CONT_CANCEL_DEF_CANCEL, YES_NO_DEF_NO } |
Static Public Member Functions | |
static void | detailedError (QWidget *parent, const QString &text, const QString &details, const QString &caption=QString(), Options options=Options(Notify|WindowModal)) |
static void | detailedSorry (QWidget *parent, const QString &text, const QString &details, const QString &caption=QString(), Options options=Options(Notify|WindowModal)) |
static void | error (QWidget *parent, const QString &text, const QString &caption=QString(), Options options=Options(Notify|WindowModal)) |
static ButtonCode | getContinueDefault (const QString &dontAskAgainName) |
static void | information (QWidget *parent, const QString &text, const QString &caption=QString(), const QString &dontShowAgainName=QString(), Options options=Options(Notify|WindowModal)) |
static int | questionYesNo (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|WindowModal)) |
static int | questionYesNoCancel (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|WindowModal)) |
static void | saveDontShowAgainContinue (const QString &dontShowAgainName, bool dontShow=true) |
static void | saveDontShowAgainYesNo (const QString &dontShowAgainName, bool dontShow=true, ButtonCode result=No) |
static void | setContinueDefault (const QString &dontAskAgainName, ButtonCode defaultButton) |
static bool | setDefaultShouldBeShownContinue (const QString &dontShowAgainName, bool defaultShow) |
static bool | shouldBeShownContinue (const QString &dontShowAgainName) |
static void | sorry (QWidget *parent, const QString &text, const QString &caption=QString(), Options options=Options(Notify|WindowModal)) |
static int | warningContinueCancel (QWidget *parent, ButtonCode defaultButton, const QString &text, const QString &caption=QString(), const KGuiItem &buttonContinue=KStandardGuiItem::cont(), const QString &dontAskAgainName=QString()) |
static int | warningContinueCancel (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonContinue=KStandardGuiItem::cont(), const KGuiItem &buttonCancel=KStandardGuiItem::cancel(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|WindowModal)) |
static int | warningYesNo (QWidget *parent, const QString &text, const QString &caption=QString(), const KGuiItem &buttonYes=KStandardGuiItem::yes(), const KGuiItem &buttonNo=KStandardGuiItem::no(), const QString &dontAskAgainName=QString(), Options options=Options(Notify|Dangerous|WindowModal)) |
Static Public Attributes | |
static const Options | NoAppModal = KMessageBox::Options(KMessageBox::Notify | KAMessageBox::WindowModal) |
static const Option | WindowModal = Notify |
Detailed Description
Enhanced KMessageBox.
The KAMessageBox class provides an extension to KMessageBox, including the option for Continue/Cancel message boxes to have a default button of Cancel.
Note that this class is not called simply MessageBox due to a name clash on Windows.
Definition at line 38 of file messagebox.h.
Member Enumeration Documentation
KAMessageBox types.
- CONT_CANCEL_DEF_CONT - Continue/Cancel, with Continue as the default button.
- CONT_CANCEL_DEF_CANCEL - Continue/Cancel, with Cancel as the default button.
- YES_NO_DEF_NO - Yes/No, with No as the default button.
Enumerator | |
---|---|
CONT_CANCEL_DEF_CONT | |
CONT_CANCEL_DEF_CANCEL | |
YES_NO_DEF_NO |
Definition at line 46 of file messagebox.h.
Member Function Documentation
|
inlinestatic |
Same as KMessageBox::detailedError() except that it defaults to window-modal, not application-modal.
Definition at line 107 of file messagebox.h.
|
inlinestatic |
Same as KMessageBox::detailedSorry() except that it defaults to window-modal, not application-modal.
Definition at line 113 of file messagebox.h.
|
inlinestatic |
Same as KMessageBox::error() except that it defaults to window-modal, not application-modal.
Definition at line 119 of file messagebox.h.
|
static |
Gets the default button for the Continue/Cancel message box with the specified "don't ask again" name.
- Parameters
-
dontAskAgainName The identifier controlling whether the message box is suppressed.
Definition at line 46 of file messagebox.cpp.
|
inlinestatic |
Same as KMessageBox::information() except that it defaults to window-modal, not application-modal.
Definition at line 125 of file messagebox.h.
|
inlinestatic |
Same as KMessageBox::questionYesNo() except that it defaults to window-modal, not application-modal.
Definition at line 137 of file messagebox.h.
|
inlinestatic |
Same as KMessageBox::questionYesNoCancel() except that it defaults to window-modal, not application-modal.
Definition at line 145 of file messagebox.h.
|
static |
Stores whether a non-Yes/No message box should or should not be shown again.
If the message box has Cancel as the default button, either setContinueDefault() or warningContinueCancel() must have been called previously to set this for the specified dontShowAgainName
value.
- Parameters
-
dontShowAgainName The identifier controlling whether the message box is suppressed. dontShow If true, the message box will be suppressed and will return Continue.
Definition at line 142 of file messagebox.cpp.
|
static |
Stores whether the Yes/No message box should or should not be shown again.
- Parameters
-
dontShowAgainName The identifier controlling whether the message box is suppressed. dontShow If true, the message box will be suppressed and will return result
.result The button code to return if the message box is suppressed.
Definition at line 129 of file messagebox.cpp.
|
static |
Sets the default button for the Continue/Cancel message box with the specified "don't ask again" name.
- Parameters
-
dontAskAgainName The identifier controlling whether the message box is suppressed. defaultButton The default button for the message box. Valid values are Continue or Cancel.
Definition at line 37 of file messagebox.cpp.
|
static |
If there is no current setting for whether a non-Yes/No message box should be shown, sets it to defaultShow
.
If a Continue/Cancel message box has Cancel as the default button, either setContinueDefault() or warningContinueCancel() must have been called previously to set this for the specified dontShowAgainName
value.
- Returns
- true if
defaultShow
was written.
Definition at line 94 of file messagebox.cpp.
|
static |
Returns whether a non-Yes/No message box should be shown.
If the message box has Cancel as the default button, either setContinueDefault() or warningContinueCancel() must have been called previously to set this for the specified dontShowAgainName
value.
- Parameters
-
dontShowAgainName The identifier controlling whether the message box is suppressed.
Definition at line 114 of file messagebox.cpp.
|
inlinestatic |
Same as KMessageBox::sorry() except that it defaults to window-modal, not application-modal.
Definition at line 131 of file messagebox.h.
|
static |
Displays a Continue/Cancel message box with the option as to which button is the default.
- Parameters
-
parent Parent widget defaultButton The default button for the message box. Valid values are Continue or Cancel. text Message string caption Caption (window title) of the message box buttonContinue The text for the first button (default = "Continue") dontAskAgainName If specified, the message box will only be suppressed if the user chose Continue last time.
Definition at line 63 of file messagebox.cpp.
|
inlinestatic |
Same as KMessageBox::warningContinueCancel() except that it defaults to window-modal, not application-modal.
Definition at line 154 of file messagebox.h.
|
inlinestatic |
Same as KMessageBox::warningYesNo() except that it defaults to window-modal, not application-modal.
Definition at line 162 of file messagebox.h.
Member Data Documentation
|
static |
Shortcut to represent Options(Notify | WindowModal).
Definition at line 169 of file messagebox.h.
|
static |
Definition at line 172 of file messagebox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.