kalarm/lib
#include <shellprocess.h>
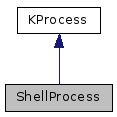
Public Types | |
enum | Status { INACTIVE, RUNNING, SUCCESS, UNAUTHORISED, DIED, NOT_FOUND, START_FAIL } |
Signals | |
void | receivedStderr (ShellProcess *) |
void | receivedStdout (ShellProcess *) |
void | shellExited (ShellProcess *) |
Public Member Functions | |
ShellProcess (const QString &command) | |
const QString & | command () const |
QString | errorMessage () const |
int | exitCode () const |
bool | normalExit () const |
bool | start (OpenMode=ReadWrite) |
Status | status () const |
void | stdinExit () |
void | writeStdin (const char *buffer, int bufflen) |
Static Public Member Functions | |
static bool | authorised () |
static const QByteArray & | shellName () |
static const QByteArray & | shellPath () |
Detailed Description
Enhanced KProcess to run a shell command.
The ShellProcess class runs a shell command and interprets the shell exit status as far as possible. It blocks execution if shell access is prohibited. It buffers data written to the process's stdin.
Before executing any command, ShellProcess checks whether shell commands are allowed at all. If not (e.g. if the user is running in kiosk mode), it blocks execution.
Derived from KProcess, this class additionally tries to interpret the shell exit status. Different shells use different exit codes. Currently, if bash or ksh report that the command could not be found or could not be executed, the NOT_FOUND status is returned.
Writes to the process's stdin are buffered, so that unlike with KProcess, there is no need to wait for the write to complete before writing again.
Definition at line 52 of file shellprocess.h.
Member Enumeration Documentation
enum ShellProcess::Status |
Current status of the shell process.
- INACTIVE - start() has not yet been called to run the command.
- RUNNING - the command is currently running.
- SUCCESS - the command appears to have exited successfully.
- UNAUTHORISED - shell commands are not authorised for this user.
- DIED - the command didn't exit cleanly, i.e. was killed or died.
- NOT_FOUND - the command was either not found or not executable.
- START_FAIL - the command couldn't be started for other reasons.
Enumerator | |
---|---|
INACTIVE | |
RUNNING | |
SUCCESS | |
UNAUTHORISED | |
DIED | |
NOT_FOUND | |
START_FAIL |
Definition at line 65 of file shellprocess.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
- Parameters
-
command The command line to be run when start() is called.
Definition at line 38 of file shellprocess.cpp.
Member Function Documentation
|
static |
Returns whether the user is authorised to run shell commands.
Shell commands may be prohibited in kiosk mode, for example.
Definition at line 215 of file shellprocess.cpp.
|
inline |
Returns the command configured to be run.
Definition at line 91 of file shellprocess.h.
QString ShellProcess::errorMessage | ( | ) | const |
Returns the error message corresponding to the command exit status.
- Returns
- Error message if an error occurred. Null string if the command has not yet exited, or if the command ran successfully.
Definition at line 153 of file shellprocess.cpp.
|
inline |
Returns the shell exit code.
Only valid if status() == SUCCESS or NOT_FOUND.
Definition at line 85 of file shellprocess.h.
|
inline |
Returns whether the command was run successfully.
- Returns
- True if the command has been run and appears to have exited successfully.
Definition at line 89 of file shellprocess.h.
|
signal |
Signal emitted when input is available from the process's stderr.
|
signal |
Signal emitted when input is available from the process's stdout.
|
signal |
Signal emitted when the shell process execution completes.
It is not emitted if start() did not attempt to start the command execution, e.g. in kiosk mode.
|
inlinestatic |
Determines which shell to use.
- Returns
- file name of shell, excluding path.
Definition at line 108 of file shellprocess.h.
|
static |
Determines which shell to use.
- Returns
- path name of shell.
Definition at line 180 of file shellprocess.cpp.
bool ShellProcess::start | ( | OpenMode | openMode = ReadWrite | ) |
Executes the configured command.
- Parameters
-
openMode WriteOnly for stdin only, ReadOnly for stdout/stderr only, else ReadWrite.
Definition at line 49 of file shellprocess.cpp.
|
inline |
Returns the current status of the shell process.
Definition at line 83 of file shellprocess.h.
void ShellProcess::stdinExit | ( | ) |
Tell the process to exit once any outstanding STDIN strings have been written.
Definition at line 141 of file shellprocess.cpp.
void ShellProcess::writeStdin | ( | const char * | buffer, |
int | bufflen | ||
) |
Writes a string to the process's STDIN.
Definition at line 104 of file shellprocess.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.