kalarm/lib
#include <spinbox.h>
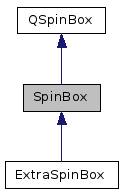
Signals | |
void | stepped (int step) |
Public Member Functions | |
SpinBox (QWidget *parent=0) | |
SpinBox (int minValue, int maxValue, QWidget *parent=0) | |
void | addValue (int change) |
int | bound (int val) const |
QRect | downRect () const |
void | initStyleOption (QStyleOptionSpinBox &) const |
bool | isReadOnly () const |
int | maximum () const |
int | minimum () const |
bool | selectOnStep () const |
void | setMaximum (int val) |
void | setMinimum (int val) |
void | setRange (int minValue, int maxValue) |
virtual void | setReadOnly (bool readOnly) |
void | setSelectOnStep (bool sel) |
void | setSingleShiftStep (int step) |
void | setSingleStep (int step) |
void | setUpDownOnly (bool only) |
int | singleShiftStep () const |
int | singleStep () const |
virtual void | stepBy (int steps) |
QRect | upDownRect () const |
QRect | upRect () const |
Protected Member Functions | |
virtual bool | eventFilter (QObject *, QEvent *) |
virtual void | focusOutEvent (QFocusEvent *) |
virtual void | keyPressEvent (QKeyEvent *) |
virtual void | keyReleaseEvent (QKeyEvent *) |
virtual void | mouseDoubleClickEvent (QMouseEvent *) |
virtual void | mouseMoveEvent (QMouseEvent *) |
virtual void | mousePressEvent (QMouseEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *) |
virtual void | paintEvent (QPaintEvent *) |
virtual int | shiftStepAdjustment (int oldValue, int shiftStep) |
virtual void | wheelEvent (QWheelEvent *) |
Detailed Description
Spin box with accelerated shift key stepping and read-only option.
The SpinBox class provides a QSpinBox with accelerated stepping using the shift key.
A separate step increment may optionally be specified for use when the shift key is held down. Typically this would be larger than the normal step. Then, when the user clicks the spin buttons, he/she can increment or decrement the value faster by holding the shift key down.
The widget may be set as read-only. This has the same effect as disabling it, except that its appearance is unchanged.
Constructor & Destructor Documentation
|
explicit |
Constructor.
- Parameters
-
parent The parent object of this widget.
Definition at line 36 of file spinbox.cpp.
SpinBox::SpinBox | ( | int | minValue, |
int | maxValue, | ||
QWidget * | parent = 0 |
||
) |
Constructor.
- Parameters
-
minValue The minimum value which the spin box can have. maxValue The maximum value which the spin box can have. parent The parent object of this widget.
Definition at line 45 of file spinbox.cpp.
Member Function Documentation
|
inline |
int SpinBox::bound | ( | int | val | ) | const |
Returns the specified value clamped to the range of the spin box.
Definition at line 87 of file spinbox.cpp.
QRect SpinBox::downRect | ( | ) | const |
Returns the rectangle containing the down arrow.
Definition at line 501 of file spinbox.cpp.
|
protectedvirtual |
Receives events destined for the spin widget or for the edit field.
Definition at line 189 of file spinbox.cpp.
|
protectedvirtual |
Definition at line 239 of file spinbox.cpp.
void SpinBox::initStyleOption | ( | QStyleOptionSpinBox & | so | ) | const |
Initialise a QStyleOptionSpinBox with this instance's details.
Definition at line 529 of file spinbox.cpp.
|
inline |
|
protectedvirtual |
Definition at line 328 of file spinbox.cpp.
|
protectedvirtual |
Definition at line 334 of file spinbox.cpp.
|
inline |
|
inline |
|
protectedvirtual |
Definition at line 255 of file spinbox.cpp.
|
protectedvirtual |
Definition at line 304 of file spinbox.cpp.
|
protectedvirtual |
Definition at line 249 of file spinbox.cpp.
|
protectedvirtual |
Definition at line 297 of file spinbox.cpp.
|
protectedvirtual |
Reimplemented in ExtraSpinBox.
Definition at line 516 of file spinbox.cpp.
|
inline |
void SpinBox::setMaximum | ( | int | val | ) |
Sets the maximum value of the spin box.
Definition at line 99 of file spinbox.cpp.
void SpinBox::setMinimum | ( | int | val | ) |
Sets the minimum value of the spin box.
Definition at line 92 of file spinbox.cpp.
|
inline |
|
virtual |
Sets whether the spin box can be changed by the user.
- Parameters
-
readOnly True to set the widget read-only, false to set it read-write.
Definition at line 76 of file spinbox.cpp.
|
inline |
void SpinBox::setSingleShiftStep | ( | int | step | ) |
Sets the shifted step increment, i.e.
the amount by which the spin box value changes when a spin button is clicked while the shift key is pressed.
Definition at line 113 of file spinbox.cpp.
void SpinBox::setSingleStep | ( | int | step | ) |
Sets the unshifted step increment, i.e.
the amount by which the spin box value changes when a spin button is clicked without the shift key being pressed.
Definition at line 106 of file spinbox.cpp.
|
inline |
|
protectedvirtual |
Returns the initial adjustment to the value for a shift step up or down.
The default is to step up or down to the nearest multiple of the shift increment, so the adjustment returned is for stepping up the decrement required to round down to a multiple of the shift increment <= current value, or for stepping down the increment required to round up to a multiple of the shift increment >= current value. This method's caller then adjusts the resultant value if necessary to cater for the widget's minimum/maximum value, and wrapping. This should really be a static method, but it needs to be virtual...
Definition at line 459 of file spinbox.cpp.
|
inline |
|
inline |
|
virtual |
Called whenever the user triggers a step, to adjust the value of the spin box by the unshifted increment.
Definition at line 120 of file spinbox.cpp.
|
signal |
Signal emitted when the spin box's value is stepped (by the shifted or unshifted increment).
- Parameters
-
step The requested step in the spin box's value. Note that the actual change in value may have been less than this.
QRect SpinBox::upDownRect | ( | ) | const |
Returns the rectangle containing the up and down arrows.
Definition at line 508 of file spinbox.cpp.
QRect SpinBox::upRect | ( | ) | const |
Returns the rectangle containing the up arrow.
Definition at line 494 of file spinbox.cpp.
|
protectedvirtual |
Definition at line 284 of file spinbox.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.