kalarm/lib
#include <timeperiod.h>
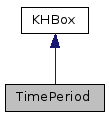
Public Types | |
enum | Units { Minutes, HoursMinutes, Days, Weeks } |
Signals | |
void | valueChanged (const KCal::Duration &period) |
Public Member Functions | |
TimePeriod (bool allowMinute, QWidget *parent) | |
bool | isDateOnly () const |
bool | isReadOnly () const |
KCal::Duration | period () const |
void | setDateOnly (bool dateOnly) |
void | setFocusOnCount () |
void | setMaximum (int hourmin, int days) |
void | setPeriod (const KCal::Duration &period, bool dateOnly, Units defaultUnits) |
virtual void | setReadOnly (bool readOnly) |
void | setSelectOnStep (bool select) |
void | setUnits (Units units) |
void | setWhatsThises (const QString &units, const QString &dayWeek, const QString &hourMin=QString()) |
Units | units () const |
Detailed Description
Time period entry widget.
The TimePeriod class provides a widget for entering a time period as a number of weeks, days, hours and minutes, or minutes.
It displays a combo box to select the time units (weeks, days, hours and minutes, or minutes) alongside a spin box to enter the number of units. The type of spin box displayed alters according to the units selection: day, week and minute values are entered in a normal spin box, while hours and minutes are entered in a time spin box (with two pairs of spin buttons, one for hours and one for minutes).
The widget may be set as read-only. This has the same effect as disabling it, except that its appearance is unchanged.
Definition at line 55 of file timeperiod.h.
Member Enumeration Documentation
enum TimePeriod::Units |
Units for the time period.
- Minutes - the time period is entered as a number of minutes.
- HoursMinutes - the time period is entered as an hours/minutes value.
- Days - the time period is entered as a number of days.
- Weeks - the time period is entered as a number of weeks.
Enumerator | |
---|---|
Minutes | |
HoursMinutes | |
Days | |
Weeks |
Definition at line 65 of file timeperiod.h.
Constructor & Destructor Documentation
TimePeriod::TimePeriod | ( | bool | allowMinute, |
QWidget * | parent | ||
) |
Constructor.
- Parameters
-
allowMinute Set false to prevent hours/minutes or minutes from being allowed as units; only days and weeks can ever be used, regardless of other method calls. Set true to allow minutes, hours/minutes, days or weeks as units. parent The parent object of this widget.
Definition at line 53 of file timeperiod.cpp.
Member Function Documentation
|
inline |
Returns true if minutes and hours/minutes units are disabled.
Definition at line 106 of file timeperiod.h.
|
inline |
Returns true if the widget is read only.
Definition at line 76 of file timeperiod.h.
Duration TimePeriod::period | ( | ) | const |
Gets the entered time period.
Definition at line 152 of file timeperiod.cpp.
|
inline |
Enables or disables minutes and hours/minutes units in the combo box.
To disable minutes and hours/minutes, set dateOnly
true; to enable minutes and hours/minutes, set dateOnly
false. But note that minutes and hours/minutes cannot be enabled if it was disallowed in the constructor.
Definition at line 112 of file timeperiod.h.
void TimePeriod::setFocusOnCount | ( | ) |
Sets the input focus to the count field.
Definition at line 122 of file timeperiod.cpp.
void TimePeriod::setMaximum | ( | int | hourmin, |
int | days | ||
) |
Sets the maximum values for the minutes and hours/minutes, and days/weeks spin boxes.
Set hourmin
= 0 to leave the minutes and hours/minutes maximum unchanged.
Definition at line 131 of file timeperiod.cpp.
void TimePeriod::setPeriod | ( | const KCal::Duration & | period, |
bool | dateOnly, | ||
Units | defaultUnits | ||
) |
Initialises the time period value.
- Parameters
-
period The value of the time period to set. If zero, the time period is left unchanged. dateOnly True to restrict the units available in the combo box to days or weeks. defaultUnits The units to display initially in the combo box.
Definition at line 175 of file timeperiod.cpp.
|
virtual |
Sets whether the widget is read-only for the user.
If read-only, the time period cannot be edited and the units combo box is inactive.
- Parameters
-
readOnly True to set the widget read-only, false to set it read-write.
Definition at line 98 of file timeperiod.cpp.
void TimePeriod::setSelectOnStep | ( | bool | select | ) |
Sets whether the editor text is to be selected whenever spin buttons are clicked.
The default is to select it.
Definition at line 113 of file timeperiod.cpp.
void TimePeriod::setUnits | ( | Units | units | ) |
Sets the time units.
Note that this changes the value.
Definition at line 337 of file timeperiod.cpp.
void TimePeriod::setWhatsThises | ( | const QString & | units, |
const QString & | dayWeek, | ||
const QString & | hourMin = QString() |
||
) |
Sets separate WhatsThis texts for the count spin boxes and the units combo box.
If hourMin
is omitted, both spin boxes are set to the same WhatsThis text.
Definition at line 420 of file timeperiod.cpp.
TimePeriod::Units TimePeriod::units | ( | ) | const |
Gets the currently selected time units.
Definition at line 361 of file timeperiod.cpp.
|
signal |
This signal is emitted whenever the value held in the widget changes.
- Parameters
-
period The current value of the time period.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.