kalarm/lib
#include <timespinbox.h>
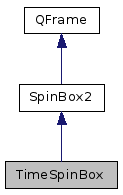
Public Slots | |
virtual void | setValue (int minutes) |
void | setValue (const QTime &t) |
![]() | |
virtual void | pageDown () |
virtual void | pageUp () |
void | selectAll () |
virtual void | setEnabled (bool enabled) |
void | setValue (int val) |
Public Member Functions | |
TimeSpinBox (bool use24hour, QWidget *parent=0) | |
TimeSpinBox (int minMinute, int maxMinute, QWidget *parent=0) | |
bool | isValid () const |
QTime | maxTime () const |
virtual QSize | minimumSizeHint () const |
virtual void | setMaximum (int minutes) |
void | setMaximum (const QTime &t) |
virtual void | setMinimum (int minutes) |
void | setValid (bool) |
virtual QSize | sizeHint () const |
virtual void | stepBy (int increment) |
QTime | time () const |
virtual QValidator::State | validate (QString &, int &pos) const |
![]() | |
SpinBox2 (QWidget *parent=0) | |
SpinBox2 (int minValue, int maxValue, int pageStep=1, QWidget *parent=0) | |
void | addPage () |
void | addSingle () |
void | addValue (int change) |
int | bound (int val) const |
QSpinBox::ButtonSymbols | buttonSymbols () const |
QString | cleanText () const |
QRect | down2Rect () const |
QRect | downRect () const |
bool | isReadOnly () const |
int | maximum () const |
int | minimum () const |
int | pageShiftStep () const |
int | pageStep () const |
QString | prefix () const |
bool | reverseButtons () const |
void | setAlignment (Qt::Alignment a) |
void | setButtonSymbols (QSpinBox::ButtonSymbols) |
void | setPrefix (const QString &text) |
void | setRange (int minValue, int maxValue) |
virtual void | setReadOnly (bool readOnly) |
void | setReverseWithLayout (bool reverse) |
void | setSelectOnStep (bool sel) |
void | setShiftSteps (int line, int page) |
void | setSingleStep (int step) |
void | setSpecialValueText (const QString &text) |
void | setSteps (int line, int page) |
void | setSuffix (const QString &text) |
void | setWrapping (bool on) |
int | singleShiftStep () const |
int | singleStep () const |
QString | specialValueText () const |
void | subtractPage () |
void | subtractSingle () |
QString | suffix () const |
QString | text () const |
QRect | up2Rect () const |
QRect | upRect () const |
int | value () const |
bool | wrapping () const |
Static Public Member Functions | |
static QString | shiftWhatsThis () |
Protected Member Functions | |
virtual QString | textFromValue (int v) const |
virtual int | valueFromText (const QString &) const |
![]() | |
virtual void | getMetrics () const |
virtual void | paintEvent (QPaintEvent *) |
virtual void | showEvent (QShowEvent *) |
virtual void | styleChange (QStyle &) |
Additional Inherited Members | |
![]() | |
void | valueChanged (int value) |
void | valueChanged (const QString &valueText) |
![]() | |
virtual void | stepPage (int) |
virtual void | valueChange () |
![]() | |
QPoint | mButtonPos |
int | wSpinboxHide |
int | wUpdown2 |
Detailed Description
Hours/minutes time entry widget.
The TimeSpinBox class provides a widget to enter a time consisting of an hours/minutes value. It can hold a time in any of 3 modes: a time of day using the 24-hour clock; a time of day using the 12-hour clock; or a length of time not restricted to 24 hours.
Derived from SpinBox2, it displays a spin box with two pairs of spin buttons, one for hours and one for minutes. It provides accelerated stepping using the spin buttons, when the shift key is held down (inherited from SpinBox2). The default shift steps are 5 minutes and 6 hours.
The widget may be set as read-only. This has the same effect as disabling it, except that its appearance is unchanged.
Definition at line 45 of file timespinbox.h.
Constructor & Destructor Documentation
|
explicit |
Constructor for a wrapping time spin box which can be used to enter a time of day.
- Parameters
-
use24hour True for entry of 24-hour clock times (range 00:00 to 23:59). False for entry of 12-hour clock times (range 12:00 to 11:59). parent The parent object of this widget.
Definition at line 43 of file timespinbox.cpp.
TimeSpinBox::TimeSpinBox | ( | int | minMinute, |
int | maxMinute, | ||
QWidget * | parent = 0 |
||
) |
Constructor for a non-wrapping time spin box which can be used to enter a length of time.
- Parameters
-
minMinute The minimum value which the spin box can hold, in minutes. maxMinute The maximum value which the spin box can hold, in minutes. parent The parent object of this widget.
Definition at line 62 of file timespinbox.cpp.
Member Function Documentation
bool TimeSpinBox::isValid | ( | ) | const |
Returns true if the spin box holds a valid value.
An invalid value is displayed as asterisks.
Definition at line 237 of file timespinbox.cpp.
|
inline |
Returns the maximum value which can be held in the spin box.
Definition at line 87 of file timespinbox.h.
|
virtual |
Reimplemented from SpinBox2.
Definition at line 254 of file timespinbox.cpp.
|
inlinevirtual |
Sets the maximum value which can be held in the spin box.
- Parameters
-
minutes The maximum value expressed in minutes.
Reimplemented from SpinBox2.
Definition at line 83 of file timespinbox.h.
|
inline |
Sets the maximum value which can be held in the spin box.
Definition at line 85 of file timespinbox.h.
|
virtual |
Sets the maximum value which can be held in the spin box.
- Parameters
-
minutes The maximum value expressed in minutes.
Reimplemented from SpinBox2.
Definition at line 194 of file timespinbox.cpp.
void TimeSpinBox::setValid | ( | bool | valid | ) |
Sets the spin box as holding a valid or invalid value.
If newly invalid, the value is displayed as asterisks. If newly valid, the value is set to the minimum value.
Definition at line 172 of file timespinbox.cpp.
|
virtualslot |
Sets the value of the spin box.
- Parameters
-
minutes The new value of the spin box, expressed in minutes.
Definition at line 203 of file timespinbox.cpp.
|
inlineslot |
Sets the value of the spin box.
Definition at line 106 of file timespinbox.h.
|
static |
Returns a text describing use of the shift key as an accelerator for the spin buttons, designed for incorporation into WhatsThis texts.
Definition at line 75 of file timespinbox.cpp.
|
virtual |
Reimplemented from SpinBox2.
Definition at line 247 of file timespinbox.cpp.
|
virtual |
Called whenever the user triggers a step, to adjust the value of the spin box.
If the value was previously invalid, the spin box is set to the minimum value.
Reimplemented from SpinBox2.
Definition at line 229 of file timespinbox.cpp.
|
protectedvirtual |
Reimplemented from SpinBox2.
Definition at line 85 of file timespinbox.cpp.
QTime TimeSpinBox::time | ( | ) | const |
Returns the current value held in the spin box.
If an invalid value is displayed, returns a value lower than the minimum value.
Definition at line 80 of file timespinbox.cpp.
|
virtual |
Determine whether the current input is valid.
Reimplemented from SpinBox2.
Definition at line 266 of file timespinbox.cpp.
|
protectedvirtual |
Reimplemented from SpinBox2.
Definition at line 106 of file timespinbox.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:59:21 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.