kdgantt2
#include <kdganttdatetimegrid.h>
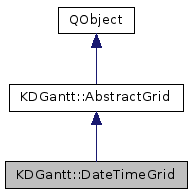
Classes | |
class | Private |
Public Types | |
enum | Scale { ScaleAuto, ScaleHour, ScaleDay, ScaleWeek, ScaleMonth, ScaleUserDefined } |
Public Member Functions | |
DateTimeGrid () | |
virtual | ~DateTimeGrid () |
qreal | dayWidth () const |
QSet< Qt::DayOfWeek > | freeDays () const |
bool | mapFromChart (const Span &span, const QModelIndex &idx, const QList< Constraint > &constraints=QList< Constraint >()) const |
qreal | mapFromDateTime (const QDateTime &dt) const |
Span | mapToChart (const QModelIndex &idx) const |
QDateTime | mapToDateTime (qreal x) const |
QBrush | noInformationBrush () const |
void | paintGrid (QPainter *painter, const QRectF &sceneRect, const QRectF &exposedRect, AbstractRowController *rowController=0, QWidget *widget=0) |
void | paintHeader (QPainter *painter, const QRectF &headerRect, const QRectF &exposedRect, qreal offset, QWidget *widget=0) |
bool | rowSeparators () const |
Scale | scale () const |
void | setDayWidth (qreal) |
void | setFreeDays (const QSet< Qt::DayOfWeek > &fd) |
void | setNoInformationBrush (const QBrush &brush) |
void | setRowSeparators (bool enable) |
void | setScale (Scale s) |
void | setStartDateTime (const QDateTime &dt) |
void | setUserDefinedLowerScale (DateTimeScaleFormatter *lower) |
void | setUserDefinedUpperScale (DateTimeScaleFormatter *upper) |
void | setWeekStart (Qt::DayOfWeek) |
QDateTime | startDateTime () const |
DateTimeScaleFormatter * | userDefinedLowerScale () const |
DateTimeScaleFormatter * | userDefinedUpperScale () const |
Qt::DayOfWeek | weekStart () const |
![]() | |
AbstractGrid (QObject *parent=0) | |
virtual | ~AbstractGrid () |
bool | isSatisfiedConstraint (const Constraint &c) const |
QAbstractItemModel * | model () const |
QModelIndex | rootIndex () const |
Protected Member Functions | |
virtual void | paintDayScaleHeader (QPainter *painter, const QRectF &headerRect, const QRectF &exposedRect, qreal offset, QWidget *widget=0) |
virtual void | paintHourScaleHeader (QPainter *painter, const QRectF &headerRect, const QRectF &exposedRect, qreal offset, QWidget *widget=0) |
virtual void | paintUserDefinedHeader (QPainter *painter, const QRectF &headerRect, const QRectF &exposedRect, qreal offset, const DateTimeScaleFormatter *formatter, QWidget *widget=0) |
Additional Inherited Members | |
![]() | |
virtual void | setModel (QAbstractItemModel *model) |
virtual void | setRootIndex (const QModelIndex &idx) |
![]() | |
void | gridChanged () |
Detailed Description
This implementation of AbstractGrid works with QDateTime and shows days and week numbers in the header
Definition at line 69 of file kdganttdatetimegrid.h.
Member Enumeration Documentation
Enumerator | |
---|---|
ScaleAuto | |
ScaleHour | |
ScaleDay | |
ScaleWeek | |
ScaleMonth | |
ScaleUserDefined |
Definition at line 73 of file kdganttdatetimegrid.h.
Constructor & Destructor Documentation
|
inline |
Definition at line 287 of file kdganttdatetimegrid.cpp.
|
virtual |
Definition at line 291 of file kdganttdatetimegrid.cpp.
Member Function Documentation
qreal DateTimeGrid::dayWidth | ( | ) | const |
- Returns
- The width in pixels for each day in the grid.
The default is 100 pixels.
Definition at line 319 of file kdganttdatetimegrid.cpp.
QSet< Qt::DayOfWeek > DateTimeGrid::freeDays | ( | ) | const |
- Returns
- The days marked as free in the grid.
Definition at line 443 of file kdganttdatetimegrid.cpp.
|
virtual |
Maps the supplied Span to QDateTimes, and puts them as start time and end time for the supplied index.
- Parameters
-
span The span used to map from. idx The index used for setting the start time and end time in the model. constraints A list of hard constraints to match against the start time and end time mapped from the span.
- Returns
- true if the start time and time was successfully added to the model, or false if unsucessful. Also returns false if any of the constraints isn't satisfied. That is, if the start time of the constrained index is before the end time of the dependency index, or the end time of the constrained index is before the start time of the dependency index.
Implements KDGantt::AbstractGrid.
Definition at line 538 of file kdganttdatetimegrid.cpp.
qreal DateTimeGrid::mapFromDateTime | ( | const QDateTime & | dt | ) | const |
Maps a given point in time dt to an X value in the scene.
Definition at line 326 of file kdganttdatetimegrid.cpp.
|
virtual |
- Parameters
-
idx The index to get the Span for.
- Returns
- The start and end pixels, in a Span, of the specified index.
Implements KDGantt::AbstractGrid.
Definition at line 479 of file kdganttdatetimegrid.cpp.
QDateTime DateTimeGrid::mapToDateTime | ( | qreal | x | ) | const |
Maps a given X value x in scene coordinates to a point in time.
Definition at line 333 of file kdganttdatetimegrid.cpp.
QBrush DateTimeGrid::noInformationBrush | ( | ) | const |
- Returns
- the brush used to mark rows with no information.
Definition at line 471 of file kdganttdatetimegrid.cpp.
|
protectedvirtual |
Paints the day scale header.
- See also
- paintHeader()
Definition at line 890 of file kdganttdatetimegrid.cpp.
|
virtual |
Implement this to paint the background of the view – typically with some grid lines.
- Parameters
-
painter – the QPainter to paint with. sceneRect – the total bounding rectangle of the scene. exposedRect – the rectangle that needs to be painted. rowController – the row controller used by the view – may be 0. widget – the widget used by the view – may be 0.
Implements KDGantt::AbstractGrid.
Definition at line 641 of file kdganttdatetimegrid.cpp.
|
virtual |
Implement this to paint the header part of the view.
- Parameters
-
painter – the QPainter to paint with. headerRect – the total rectangle occupied by the header. exposedRect – the rectangle that needs to be painted. offset – the horizontal scroll offset of the view. widget – the widget used by the view – may be 0.
Implements KDGantt::AbstractGrid.
Definition at line 747 of file kdganttdatetimegrid.cpp.
|
protectedvirtual |
Paints the hour scale header.
- See also
- paintHeader()
Definition at line 854 of file kdganttdatetimegrid.cpp.
|
protectedvirtual |
Definition at line 824 of file kdganttdatetimegrid.cpp.
bool DateTimeGrid::rowSeparators | ( | ) | const |
- Returns
- true if row separators are used.
Definition at line 449 of file kdganttdatetimegrid.cpp.
DateTimeGrid::Scale DateTimeGrid::scale | ( | ) | const |
- Returns
- The scale used to paint the grid.
The default is ScaleAuto, which means the day scale will be used as long as the day width is less or equal to 500.
- See also
- Scale
Definition at line 366 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setDayWidth | ( | qreal | w | ) |
- Parameters
-
w The width in pixels for each day in the grid.
The signal gridChanged() is emitted after the day width is changed.
Definition at line 342 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setFreeDays | ( | const QSet< Qt::DayOfWeek > & | fd | ) |
- Parameters
-
fd A set of days to mark as free in the grid.
Free days are filled with the alternate base brush of the palette used by the view. The signal gridChanged() is emitted after the free days are changed.
Definition at line 436 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setNoInformationBrush | ( | const QBrush & | brush | ) |
Sets the brush used to display rows where no data is found. Default is a red pattern. If set to QBrush() rows with no information will not be marked.
Definition at line 463 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setRowSeparators | ( | bool | enable | ) |
- Parameters
-
enable Whether to use row separators or not.
Definition at line 454 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setScale | ( | Scale | s | ) |
- Parameters
-
s The scale to be used to paint the grid.
The signal gridChanged() is emitted after the scale has changed.
- See also
- Scale
Definition at line 354 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setStartDateTime | ( | const QDateTime & | dt | ) |
- Parameters
-
dt The start date of the grid. It is used as the beginning of the horizontal scrollbar in the view.
Emits gridChanged() after the start date has changed.
Definition at line 309 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setUserDefinedLowerScale | ( | DateTimeScaleFormatter * | lower | ) |
Sets the scale formatter for the lower part of the header to the user defined formatter to lower. The DateTimeGrid object takes ownership of the formatter, which has to be allocated with new.
You have to set the scale to ScaleUserDefined for this setting to take effect.
- See also
- DateTimeScaleFormatter
Definition at line 378 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setUserDefinedUpperScale | ( | DateTimeScaleFormatter * | upper | ) |
Sets the scale formatter for the upper part of the header to the user defined formatter to upper. The DateTimeGrid object takes ownership of the formatter, which has to be allocated with new.
You have to set the scale to ScaleUserDefined for this setting to take effect.
- See also
- DateTimeScaleFormatter
Definition at line 392 of file kdganttdatetimegrid.cpp.
void DateTimeGrid::setWeekStart | ( | Qt::DayOfWeek | ws | ) |
- Parameters
-
ws The start day of the week.
A solid line is drawn on the grid to mark the beginning of a new week. Emits gridChanged() after the start day has changed.
Definition at line 418 of file kdganttdatetimegrid.cpp.
QDateTime DateTimeGrid::startDateTime | ( | ) | const |
- Returns
- The QDateTime used as start date for the grid.
The default is three days before the current date.
Definition at line 299 of file kdganttdatetimegrid.cpp.
DateTimeScaleFormatter * DateTimeGrid::userDefinedLowerScale | ( | ) | const |
- Returns
- The DateTimeScaleFormatter being used to render the lower scale.
Definition at line 401 of file kdganttdatetimegrid.cpp.
DateTimeScaleFormatter * DateTimeGrid::userDefinedUpperScale | ( | ) | const |
- Returns
- The DateTimeScaleFormatter being used to render the upper scale.
Definition at line 408 of file kdganttdatetimegrid.cpp.
Qt::DayOfWeek DateTimeGrid::weekStart | ( | ) | const |
- Returns
- The start day of the week
Definition at line 425 of file kdganttdatetimegrid.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.