kleopatra
#include <assuancommand.h>
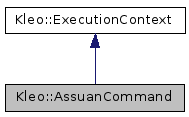
Classes | |
class | Memento |
class | TypedMemento |
Public Types | |
enum | CheckProtocolOption { AllowProtocolMissing =0x01 } |
enum | Mode { NoMode, EMail, FileManager } |
Public Member Functions | |
AssuanCommand () | |
virtual | ~AssuanCommand () |
void | applyWindowID (QWidget *w) const |
void | canceled () |
Mode | checkMode () const |
GpgME::Protocol | checkProtocol (Mode mode, int options=0) const |
void | done (const GpgME::Error &err=GpgME::Error()) |
void | done (const GpgME::Error &err, const QString &details) |
void | done (int err) |
void | done (int err, const QString &details) |
QStringList | fileNames () const |
bool | hasMemento (const QByteArray &tag) const |
bool | hasOption (const char *opt) const |
bool | informativeRecipients () const |
bool | informativeSenders () const |
const std::vector < boost::shared_ptr< Input > > & | inputs () const |
int | inquire (const char *keyword, QObject *receiver, const char *slot, unsigned int maxSize=0) |
bool | isDone () const |
bool | isNohup () const |
boost::shared_ptr< Memento > | memento (const QByteArray &tag) const |
template<typename T > | |
boost::shared_ptr< T > | mementoAs (const QByteArray &tag) const |
template<typename T > | |
T | mementoContent (const QByteArray &tag) const |
const std::vector < boost::shared_ptr< Input > > & | messages () const |
virtual const char * | name () const =0 |
unsigned int | numFiles () const |
QVariant | option (const char *opt) const |
const std::map< std::string, QVariant > & | options () const |
const std::vector < boost::shared_ptr< Output > > & | outputs () const |
WId | parentWId () const |
const std::vector < KMime::Types::Mailbox > & | recipients () const |
QByteArray | registerMemento (const boost::shared_ptr< Memento > &mem) |
QByteArray | registerMemento (const QByteArray &tag, const boost::shared_ptr< Memento > &mem) |
void | removeMemento (const QByteArray &tag) |
void | sendData (const QByteArray &data, bool moreToCome=false) |
const std::vector < KMime::Types::Mailbox > & | senders () const |
void | sendStatus (const char *keyword, const QString &text) |
void | sendStatusEncoded (const char *keyword, const std::string &text) |
unsigned int | sessionId () const |
QString | sessionTitle () const |
void | setNohup (bool on) |
int | start () |
![]() | |
virtual | ~ExecutionContext () |
Static Public Member Functions | |
template<typename T > | |
static boost::shared_ptr < TypedMemento< T > > | make_typed_memento (const T &t) |
static int | makeError (int code) |
Detailed Description
Base class for GnuPG UI Server commands.
- Note
- large parts of this are outdated by now!
Implementing a new AssuanCommand
You do not directly inherit AssuanCommand, unless you want to deal with implementing low-level, repetetive things like name() in terms of staticName(). Assuming you don't, then you inherit your command class from AssuanCommandMixin, passing your class as the template argument to AssuanCommandMixin, like this:
(http://en.wikipedia.org/wiki/Curiously_recurring_template_pattern)
You then choose a command name, and return that from the static method staticName(), which is by convention queried by both AssuanCommandMixin<> and GenericAssuanCommandFactory<>:
The string should be all-uppercase by convention, but the UiServer implementation doesn't enforce this.
The next step is to implement start(), the starting point of command execution:
Executing the command
This should set everything up and check the parameters in line and any options this command understands. If there's an error, choose one of the gpg-error codes and create a gpg_error_t from it using the protected makeError() function:
But usually, you will want to create a dialog, or call some GpgME function from here. In case of errors from GpgME, you shouldn't pipe them through makeError(), but return them as-is. This will preserve the error source. Error created using makeError() will have Kleopatra as their error source, so watch out what you're doing :)
In addition to options and the command line, your command might require {bulk data} input or output. That's what the bulk input and output channels are for. You can check whether the client handed you an input channel by checking that bulkInputDevice() isn't NULL, likewise for bulkOutputDevice().
If everything is ok, you return 0. This indicates to the client that the command has been accepted and is now in progress.
In this mode (start() returned 0), there are a bunch of options for your command to do. Some commands may require additional information from the client. The options passed to start() are designed to be persistent across commands, and rather limited in length (there's a strict line length limit in the assuan protocol with no line continuation mechanism). The same is true for command line arguments, which, in addition, you have to parse yourself. Those usually apply only to this command, and not to following ones.
If you need data that might be larger than the line length limit, you can either expect it on the bulkInputDevice(), or, if you have the need for more than one such data channel, or the data is optional or conditional on some condition that can only be determined during command execution, you can inquire the missing information from the client.
As an example, a VERIFY command would expect the signed data on the bulkInputDevice(). But if the input stream doesn't contain an embedded (opaque) signature, indicating a detached signature, it would go and inquire that data from the client. Here's how it works:
This should be self-explanatory: You give a slot to call when the data has arrived. The slot's first argument is an error code. The second the data (if any), and the third is just repeating what you gave as inquire()'s first argument. As usual, you can leave argument off of the end, if you are not interested in them.
You can do as many inquiries as you want, but only one at a time.
You should peridocally send status updates to the client. You do that by calling sendStatus().
Once your command has finished executing, call done(). If it's with an error code, call done(err) like above. {Do not forget to call done() when done!}. It will close bulkInputDevice(), bulkOutputDevice(), and send an OK or ERR message back to the client.
At that point, your command has finished executing, and a new one can be accepted, or the connection closed.
Apropos connection closed. The only way for the client to cancel an operation is to shut down the connection. In this case, the canceled() function will be called. At that point, the connection to the client will have been broken already, and all you can do is pack your things and go down gracefully.
If you detect that the user has canceled (your dialog contains a cancel button, doesn't it?), then you should instead call done( GPG_ERR_CANCELED ), like for normal operation.
Registering the command with UiServer
To register a command, you implement a AssuanCommandFactory for your AssuanCommand subclass, and register it with the UiServer. This can be made considerably easier using GenericAssuanCommandFactory:
Definition at line 213 of file assuancommand.h.
Member Enumeration Documentation
Enumerator | |
---|---|
AllowProtocolMissing |
Definition at line 250 of file assuancommand.h.
Enumerator | |
---|---|
NoMode | |
FileManager |
Definition at line 247 of file assuancommand.h.
Constructor & Destructor Documentation
AssuanCommand::AssuanCommand | ( | ) |
Definition at line 1104 of file assuanserverconnection.cpp.
|
virtual |
Definition at line 1110 of file assuanserverconnection.cpp.
Member Function Documentation
|
inlinevirtual |
Implements Kleo::ExecutionContext.
Definition at line 256 of file assuancommand.h.
void AssuanCommand::canceled | ( | ) |
Definition at line 1139 of file assuanserverconnection.cpp.
AssuanCommand::Mode AssuanCommand::checkMode | ( | ) | const |
Checks the –mode
parameter.
- Returns
- The parameter as an AssuanCommand::Mode enum value.
If no –mode
was given, or it's value wasn't recognized, throws an Kleo::Exception.
Definition at line 1508 of file assuanserverconnection.cpp.
GpgME::Protocol AssuanCommand::checkProtocol | ( | Mode | mode, |
int | options = 0 |
||
) | const |
Checks the –protocol
parameter.
- Returns
- The parameter as a GpgME::Protocol enum value.
If –protocol
was given, but has an invalid value, throws an Kleo::Exception.
If no –protocol
was given, checks the connection bias, if available, otherwise, in FileManager mode, returns GpgME::UnknownProtocol, but if mode == EMail
, throws an Kleo::Exception instead.
Definition at line 1533 of file assuanserverconnection.cpp.
void AssuanCommand::done | ( | const GpgME::Error & | err = GpgME::Error() | ) |
Definition at line 1307 of file assuanserverconnection.cpp.
void AssuanCommand::done | ( | const GpgME::Error & | err, |
const QString & | details | ||
) |
Definition at line 1297 of file assuanserverconnection.cpp.
|
inline |
Definition at line 310 of file assuancommand.h.
|
inline |
Definition at line 311 of file assuancommand.h.
QStringList AssuanCommand::fileNames | ( | ) | const |
Definition at line 1244 of file assuanserverconnection.cpp.
bool AssuanCommand::hasMemento | ( | const QByteArray & | tag | ) | const |
Definition at line 1183 of file assuanserverconnection.cpp.
bool AssuanCommand::hasOption | ( | const char * | opt | ) | const |
Definition at line 1149 of file assuanserverconnection.cpp.
bool AssuanCommand::informativeRecipients | ( | ) | const |
Definition at line 1374 of file assuanserverconnection.cpp.
bool AssuanCommand::informativeSenders | ( | ) | const |
Definition at line 1370 of file assuanserverconnection.cpp.
const std::vector< shared_ptr< Input > > & AssuanCommand::inputs | ( | ) | const |
Definition at line 1232 of file assuanserverconnection.cpp.
int AssuanCommand::inquire | ( | const char * | keyword, |
QObject * | receiver, | ||
const char * | slot, | ||
unsigned int | maxSize = 0 |
||
) |
Definition at line 1273 of file assuanserverconnection.cpp.
bool AssuanCommand::isDone | ( | ) | const |
Definition at line 1358 of file assuanserverconnection.cpp.
bool AssuanCommand::isNohup | ( | ) | const |
Definition at line 1354 of file assuanserverconnection.cpp.
|
inlinestatic |
Definition at line 240 of file assuancommand.h.
|
static |
Definition at line 1145 of file assuanserverconnection.cpp.
shared_ptr< AssuanCommand::Memento > AssuanCommand::memento | ( | const QByteArray & | tag | ) | const |
Definition at line 1190 of file assuanserverconnection.cpp.
|
inline |
Definition at line 277 of file assuancommand.h.
|
inline |
Definition at line 284 of file assuancommand.h.
const std::vector< shared_ptr< Input > > & AssuanCommand::messages | ( | ) | const |
Definition at line 1236 of file assuanserverconnection.cpp.
|
pure virtual |
unsigned int AssuanCommand::numFiles | ( | ) | const |
Definition at line 1248 of file assuanserverconnection.cpp.
QVariant AssuanCommand::option | ( | const char * | opt | ) | const |
Definition at line 1153 of file assuanserverconnection.cpp.
const std::map< std::string, QVariant > & AssuanCommand::options | ( | ) | const |
Definition at line 1161 of file assuanserverconnection.cpp.
const std::vector< shared_ptr< Output > > & AssuanCommand::outputs | ( | ) | const |
Definition at line 1240 of file assuanserverconnection.cpp.
WId AssuanCommand::parentWId | ( | ) | const |
Definition at line 1559 of file assuanserverconnection.cpp.
const std::vector< KMime::Types::Mailbox > & AssuanCommand::recipients | ( | ) | const |
Definition at line 1378 of file assuanserverconnection.cpp.
QByteArray AssuanCommand::registerMemento | ( | const boost::shared_ptr< Memento > & | mem | ) |
Definition at line 1205 of file assuanserverconnection.cpp.
QByteArray AssuanCommand::registerMemento | ( | const QByteArray & | tag, |
const boost::shared_ptr< Memento > & | mem | ||
) |
Definition at line 1210 of file assuanserverconnection.cpp.
void AssuanCommand::removeMemento | ( | const QByteArray & | tag | ) |
Definition at line 1222 of file assuanserverconnection.cpp.
void AssuanCommand::sendData | ( | const QByteArray & | data, |
bool | moreToCome = false |
||
) |
Definition at line 1263 of file assuanserverconnection.cpp.
const std::vector< KMime::Types::Mailbox > & AssuanCommand::senders | ( | ) | const |
Definition at line 1382 of file assuanserverconnection.cpp.
void AssuanCommand::sendStatus | ( | const char * | keyword, |
const QString & | text | ||
) |
Definition at line 1252 of file assuanserverconnection.cpp.
void AssuanCommand::sendStatusEncoded | ( | const char * | keyword, |
const std::string & | text | ||
) |
Definition at line 1256 of file assuanserverconnection.cpp.
unsigned int AssuanCommand::sessionId | ( | ) | const |
Definition at line 1366 of file assuanserverconnection.cpp.
QString AssuanCommand::sessionTitle | ( | ) | const |
Definition at line 1362 of file assuanserverconnection.cpp.
void AssuanCommand::setNohup | ( | bool | on | ) |
Definition at line 1350 of file assuanserverconnection.cpp.
int AssuanCommand::start | ( | ) |
Definition at line 1114 of file assuanserverconnection.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.