kleopatra
#include <signencryptwizard.h>
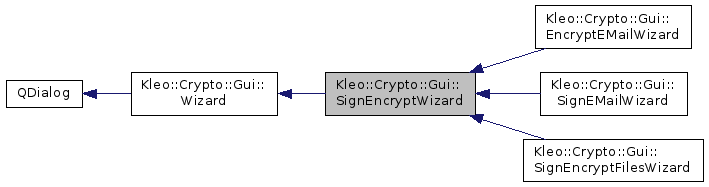
Public Types | |
enum | Page { ResolveSignerPage =0, ObjectsPage, ResolveRecipientsPage, ResultPage } |
![]() | |
enum | Page { InvalidPage =-1 } |
Signals | |
void | linkActivated (const QString &link) |
void | objectsResolved () |
void | recipientsResolved () |
void | signersResolved () |
![]() | |
void | canceled () |
Public Member Functions | |
SignEncryptWizard (QWidget *parent=0, Qt::WindowFlags f=0) | |
virtual | ~SignEncryptWizard () |
bool | isAsciiArmorEnabled () const |
bool | keepResultPageOpenWhenDone () const |
void | onNext (int currentId) |
GpgME::Protocol | presetProtocol () const |
bool | recipientsUserMutable () const |
bool | removeUnencryptedFile () const |
std::vector< GpgME::Key > | resolvedCertificates () const |
std::vector< GpgME::Key > | resolvedSigners () const |
GpgME::Protocol | selectedProtocol () const |
void | setAsciiArmorEnabled (bool enabled) |
void | setCommitPage (Page) |
void | setKeepResultPageOpenWhenDone (bool keep) |
void | setPresetProtocol (GpgME::Protocol proto) |
void | setRecipients (const std::vector< KMime::Types::Mailbox > &recipients, const std::vector< KMime::Types::Mailbox > &encryptoToSelfRecipients) |
void | setRecipientsUserMutable (bool isMutable) |
void | setRemoveUnencryptedFile (bool remove) |
void | setSignersAndCandidates (const std::vector< KMime::Types::Mailbox > &signers, const std::vector< std::vector< GpgME::Key > > &keys) |
void | setTaskCollection (const boost::shared_ptr< TaskCollection > &tasks) |
QFileInfoList | resolvedFiles () const |
void | setFiles (const QStringList &files) |
bool | signingSelected () const |
void | setSigningSelected (bool selected) |
bool | encryptionSelected () const |
void | setEncryptionSelected (bool selected) |
bool | isSigningUserMutable () const |
void | setSigningUserMutable (bool isMutable) |
bool | isEncryptionUserMutable () const |
void | setEncryptionUserMutable (bool isMutable) |
bool | isMultipleProtocolsAllowed () const |
void | setMultipleProtocolsAllowed (bool allowed) |
![]() | |
Wizard (QWidget *parent=0, Qt::WindowFlags f=0) | |
~Wizard () | |
bool | canGoToNextPage () const |
bool | canGoToPreviousPage () const |
int | currentPage () const |
const WizardPage * | currentPageWidget () const |
WizardPage * | currentPageWidget () |
const WizardPage * | page (int id) const |
WizardPage * | page (int id) |
void | setCurrentPage (int id) |
void | setPage (int id, WizardPage *page) |
void | setPageOrder (const std::vector< int > &pages) |
void | setPageVisible (int id, bool visible) |
Protected Member Functions | |
Gui::ObjectsPage * | objectsPage () |
Gui::ResolveRecipientsPage * | resolveRecipientsPage () |
Gui::ResultPage * | resultPage () |
void | setSignerResolvePageValidator (const boost::shared_ptr< SignerResolvePage::Validator > &validator) |
Gui::SignerResolvePage * | signerResolvePage () |
const Gui::SignerResolvePage * | signerResolvePage () const |
![]() | |
virtual void | onBack (int currentId) |
Additional Inherited Members | |
![]() | |
void | back () |
void | next () |
Detailed Description
Definition at line 70 of file signencryptwizard.h.
Member Enumeration Documentation
Enumerator | |
---|---|
ResolveSignerPage | |
ObjectsPage | |
ResolveRecipientsPage | |
ResultPage |
Definition at line 76 of file signencryptwizard.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 118 of file signencryptwizard.cpp.
|
virtual |
Definition at line 124 of file signencryptwizard.cpp.
Member Function Documentation
bool SignEncryptWizard::encryptionSelected | ( | ) | const |
Definition at line 214 of file signencryptwizard.cpp.
bool SignEncryptWizard::isAsciiArmorEnabled | ( | ) | const |
Definition at line 241 of file signencryptwizard.cpp.
bool SignEncryptWizard::isEncryptionUserMutable | ( | ) | const |
Definition at line 176 of file signencryptwizard.cpp.
bool SignEncryptWizard::isMultipleProtocolsAllowed | ( | ) | const |
Definition at line 182 of file signencryptwizard.cpp.
bool SignEncryptWizard::isSigningUserMutable | ( | ) | const |
Definition at line 166 of file signencryptwizard.cpp.
bool SignEncryptWizard::keepResultPageOpenWhenDone | ( | ) | const |
Definition at line 301 of file signencryptwizard.cpp.
|
signal |
|
protected |
Definition at line 291 of file signencryptwizard.cpp.
|
signal |
|
virtual |
Reimplemented from Kleo::Crypto::Gui::Wizard.
Definition at line 102 of file signencryptwizard.cpp.
GpgME::Protocol SignEncryptWizard::presetProtocol | ( | ) | const |
Definition at line 151 of file signencryptwizard.cpp.
|
signal |
bool SignEncryptWizard::recipientsUserMutable | ( | ) | const |
if true, the user is allowed to remove/add recipients via the UI.
Defaults to false
.
Definition at line 261 of file signencryptwizard.cpp.
bool SignEncryptWizard::removeUnencryptedFile | ( | ) | const |
Definition at line 251 of file signencryptwizard.cpp.
std::vector< Key > SignEncryptWizard::resolvedCertificates | ( | ) | const |
Definition at line 233 of file signencryptwizard.cpp.
QFileInfoList SignEncryptWizard::resolvedFiles | ( | ) | const |
SignOrEncryptFiles mode subinterface.
Definition at line 202 of file signencryptwizard.cpp.
std::vector< Key > SignEncryptWizard::resolvedSigners | ( | ) | const |
Definition at line 237 of file signencryptwizard.cpp.
|
protected |
Definition at line 286 of file signencryptwizard.cpp.
|
protected |
Definition at line 296 of file signencryptwizard.cpp.
GpgME::Protocol SignEncryptWizard::selectedProtocol | ( | ) | const |
Definition at line 146 of file signencryptwizard.cpp.
void SignEncryptWizard::setAsciiArmorEnabled | ( | bool | enabled | ) |
Definition at line 246 of file signencryptwizard.cpp.
void SignEncryptWizard::setCommitPage | ( | Page | page | ) |
Definition at line 126 of file signencryptwizard.cpp.
void SignEncryptWizard::setEncryptionSelected | ( | bool | selected | ) |
Definition at line 156 of file signencryptwizard.cpp.
void SignEncryptWizard::setEncryptionUserMutable | ( | bool | isMutable | ) |
Definition at line 193 of file signencryptwizard.cpp.
void SignEncryptWizard::setFiles | ( | const QStringList & | files | ) |
Definition at line 198 of file signencryptwizard.cpp.
void SignEncryptWizard::setKeepResultPageOpenWhenDone | ( | bool | keep | ) |
Definition at line 306 of file signencryptwizard.cpp.
void SignEncryptWizard::setMultipleProtocolsAllowed | ( | bool | allowed | ) |
Definition at line 187 of file signencryptwizard.cpp.
void SignEncryptWizard::setPresetProtocol | ( | GpgME::Protocol | proto | ) |
Definition at line 140 of file signencryptwizard.cpp.
void SignEncryptWizard::setRecipients | ( | const std::vector< KMime::Types::Mailbox > & | recipients, |
const std::vector< KMime::Types::Mailbox > & | encryptoToSelfRecipients | ||
) |
Definition at line 218 of file signencryptwizard.cpp.
void SignEncryptWizard::setRecipientsUserMutable | ( | bool | isMutable | ) |
Definition at line 266 of file signencryptwizard.cpp.
void SignEncryptWizard::setRemoveUnencryptedFile | ( | bool | remove | ) |
Definition at line 256 of file signencryptwizard.cpp.
|
protected |
Definition at line 271 of file signencryptwizard.cpp.
void SignEncryptWizard::setSignersAndCandidates | ( | const std::vector< KMime::Types::Mailbox > & | signers, |
const std::vector< std::vector< GpgME::Key > > & | keys | ||
) |
Definition at line 222 of file signencryptwizard.cpp.
void SignEncryptWizard::setSigningSelected | ( | bool | selected | ) |
Definition at line 161 of file signencryptwizard.cpp.
void SignEncryptWizard::setSigningUserMutable | ( | bool | isMutable | ) |
Definition at line 171 of file signencryptwizard.cpp.
void SignEncryptWizard::setTaskCollection | ( | const boost::shared_ptr< TaskCollection > & | tasks | ) |
Definition at line 227 of file signencryptwizard.cpp.
|
protected |
Definition at line 276 of file signencryptwizard.cpp.
|
protected |
Definition at line 281 of file signencryptwizard.cpp.
|
signal |
bool SignEncryptWizard::signingSelected | ( | ) | const |
Definition at line 210 of file signencryptwizard.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:43 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.