kmail
#include <kmcomposewin.h>
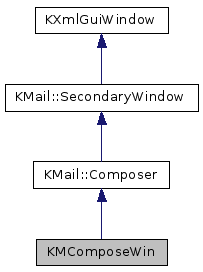
Public Slots | |
Q_SCRIPTABLE void | addAttachment (const KUrl &url, const QString &comment) |
Q_SCRIPTABLE void | addAttachment (const QString &name, KMime::Headers::contentEncoding cte, const QString &charset, const QByteArray &data, const QByteArray &mimeType) |
Q_SCRIPTABLE void | addAttachmentsAndSend (const KUrl::List &urls, const QString &comment, int how) |
void | autoSaveMessage (bool force=false) |
void | htmlToolBarVisibilityChanged (bool visible) |
Q_SCRIPTABLE void | send (int how) |
void | setModified (bool modified) |
void | slotFetchJob (KJob *) |
void | slotSendNow () |
void | slotSpellcheckDoneClearStatus () |
void | slotTextModeChanged (KRichTextEdit::Mode) |
void | slotToggleMarkup () |
void | slotWordWrapToggled (bool) |
![]() | |
virtual void | autoSaveMessage (bool force=false)=0 |
virtual void | setModified (bool modified)=0 |
virtual void | slotSendNow ()=0 |
virtual void | slotWordWrapToggled (bool)=0 |
![]() | |
virtual void | setCaption (const QString &caption) |
Signals | |
void | identityChanged (const KPIMIdentities::Identity &identity) |
Public Member Functions | |
void | addAttach (KMime::Content *msgPart) |
void | addExtraCustomHeaders (const QMap< QByteArray, QString > &header) |
QString | dbusObjectPath () const |
void | disableForgottenAttachmentsCheck () |
void | disableWordWrap () |
void | forceDisableHtml () |
const KPIMIdentities::Identity & | identity () const |
void | ignoreStickyFields () |
bool | insertFromMimeData (const QMimeData *source, bool forceAttachment=false) |
bool | isComposing () const |
KAction * | lowerCaseAction () const |
void | setCollectionForNewMessage (const Akonadi::Collection &folder) |
void | setCurrentReplyTo (const QString &) |
void | setCurrentTransport (int transportId) |
void | setCustomTemplate (const QString &customTemplate) |
void | setFcc (const QString &idString) |
void | setFocusToEditor () |
void | setFocusToSubject () |
void | setFolder (const Akonadi::Collection &aFolder) |
void | setMessage (const KMime::Message::Ptr &newMsg, bool lastSignState=false, bool lastEncryptState=false, bool mayAutoSign=true, bool allowDecryption=false, bool isModified=false) |
void | setSigningAndEncryptionDisabled (bool v) |
void | setTextSelection (const QString &selection) |
QString | smartQuote (const QString &msg) |
KAction * | upperCaseAction () const |
![]() | |
virtual void | addAttachment (const KUrl &url, const QString &comment)=0 |
virtual void | addAttachment (const QString &name, KMime::Headers::contentEncoding cte, const QString &charset, const QByteArray &data, const QByteArray &mimeType)=0 |
virtual void | addAttachmentsAndSend (const KUrl::List &urls, const QString &comment, int how)=0 |
virtual void | send (int how)=0 |
virtual void | setAutoSaveFileName (const QString &fileName)=0 |
![]() | |
SecondaryWindow (const char *name=0) | |
~SecondaryWindow () | |
Static Public Member Functions | |
static Composer * | create (const KMime::Message::Ptr &msg, bool lastSignState, bool lastEncryptState, TemplateContext context=NoTemplate, uint identity=0, const QString &textSelection=QString(), const QString &customTemplate=QString()) |
Additional Inherited Members | |
![]() | |
enum | TemplateContext { New, Reply, ReplyToAll, Forward, NoTemplate } |
enum | VisibleHeaderFlag { HDR_FROM = 0x01, HDR_REPLY_TO = 0x02, HDR_SUBJECT = 0x20, HDR_NEWSGROUPS = 0x40, HDR_FOLLOWUP_TO = 0x80, HDR_IDENTITY = 0x100, HDR_TRANSPORT = 0x200, HDR_FCC = 0x400, HDR_DICTIONARY = 0x800, HDR_ALL = 0xfff } |
typedef QFlags< VisibleHeaderFlag > | VisibleHeaderFlags |
![]() | |
Composer (const char *name=0) | |
![]() | |
virtual void | closeEvent (QCloseEvent *) |
Detailed Description
Definition at line 106 of file kmcomposewin.h.
Member Function Documentation
|
virtual |
Add an attachment to the list.
Implements KMail::Composer.
Definition at line 1970 of file kmcomposewin.cpp.
|
slot |
Definition at line 576 of file kmcomposewin.cpp.
|
slot |
Definition at line 582 of file kmcomposewin.cpp.
|
slot |
Definition at line 564 of file kmcomposewin.cpp.
|
virtual |
Implements KMail::Composer.
Definition at line 3530 of file kmcomposewin.cpp.
|
slot |
Definition at line 1917 of file kmcomposewin.cpp.
|
static |
Definition at line 177 of file kmcomposewin.cpp.
|
virtual |
Implements KMail::Composer.
Definition at line 533 of file kmcomposewin.cpp.
|
virtual |
Don't check for forgotten attachments for a mail, eg.
when sending out invitations.
Implements KMail::Composer.
Definition at line 2582 of file kmcomposewin.cpp.
|
virtual |
Disables word wrap completely.
No wrapping at all will occur, not even at the right end of the editor. This is useful when sending invitations.
Implements KMail::Composer.
Definition at line 2568 of file kmcomposewin.cpp.
|
virtual |
Disables HTML completely.
It disables HTML at the point of calling this and disables it again when sending the message, to be sure. Useful when sending invitations. This will not remove the actions for activating HTML mode again, it is only meant for automatic invitation sending. Also calls
- See also
- disableHtml() internally.
Implements KMail::Composer.
Definition at line 2574 of file kmcomposewin.cpp.
|
slot |
Definition at line 3041 of file kmcomposewin.cpp.
const KPIMIdentities::Identity & KMComposeWin::identity | ( | ) | const |
Definition at line 1956 of file kmcomposewin.cpp.
|
signal |
End of D-Bus callable stuff.
|
virtual |
Ignore the "sticky" setting of the transport combo box and prefer the X-KMail-Transport header field of the message instead.
Do the same for the identity combo box, don't obey the "sticky" setting but use the X-KMail-Identity header field instead.
This is useful when sending out invitations, since you don't see the GUI and want the identity and transport to be set to the values stored in the messages.
Implements KMail::Composer.
Definition at line 2587 of file kmcomposewin.cpp.
bool KMComposeWin::insertFromMimeData | ( | const QMimeData * | source, |
bool | forceAttachment = false |
||
) |
Definition at line 2137 of file kmcomposewin.cpp.
|
inlinevirtual |
Returns true
while the message composing is in progress.
Implements KMail::Composer.
Definition at line 193 of file kmcomposewin.h.
|
inline |
Definition at line 233 of file kmcomposewin.h.
|
slot |
Start of D-Bus callable stuff.
The D-Bus methods need to be public slots, otherwise they can't be accessed.
Definition at line 548 of file kmcomposewin.cpp.
|
virtual |
Implements KMail::Composer.
Definition at line 1077 of file kmcomposewin.cpp.
|
virtual |
Implements KMail::Composer.
Definition at line 1565 of file kmcomposewin.cpp.
|
virtual |
Implements KMail::Composer.
Definition at line 1560 of file kmcomposewin.cpp.
|
virtual |
Set custom template to be used for the message.
Implements KMail::Composer.
Definition at line 1797 of file kmcomposewin.cpp.
|
virtual |
Use the given folder as sent-mail folder if the given folder exists.
Else show an error message and use the default sent-mail folder as sent-mail folder.
Implements KMail::Composer.
Definition at line 1803 of file kmcomposewin.cpp.
|
virtual |
Sets the focus to the edit-widget.
Implements KMail::Composer.
Definition at line 3239 of file kmcomposewin.cpp.
|
virtual |
Sets the focus to the subject line edit.
For use when creating a message to a known recipient.
Implements KMail::Composer.
Definition at line 3246 of file kmcomposewin.cpp.
|
inlinevirtual |
If this folder is set, the original message is inserted back after canceling.
Implements KMail::Composer.
Definition at line 214 of file kmcomposewin.h.
|
virtual |
Set the message the composer shall work with.
This discards previous messages without calling applyChanges() on them before.
Implements KMail::Composer.
Definition at line 1573 of file kmcomposewin.cpp.
|
slot |
Set whether the message should be treated as modified or not.
Definition at line 1832 of file kmcomposewin.cpp.
|
inlinevirtual |
Disabled signing and encryption completely for this composer window.
Implements KMail::Composer.
Definition at line 206 of file kmcomposewin.h.
|
virtual |
Set the text selection the message is a response to.
Implements KMail::Composer.
Definition at line 1791 of file kmcomposewin.cpp.
|
slot |
Definition at line 2257 of file kmcomposewin.cpp.
|
slot |
Definition at line 2903 of file kmcomposewin.cpp.
|
slot |
Definition at line 3074 of file kmcomposewin.cpp.
|
slot |
Definition at line 3032 of file kmcomposewin.cpp.
|
slot |
Definition at line 3026 of file kmcomposewin.cpp.
|
slot |
Switch wordWrap on/off.
Definition at line 2559 of file kmcomposewin.cpp.
QString KMComposeWin::smartQuote | ( | const QString & | msg | ) |
Definition at line 2131 of file kmcomposewin.cpp.
|
inline |
Definition at line 234 of file kmcomposewin.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.