kmail
#include <kmkernel.h>
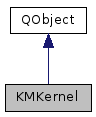
Public Slots | |
Q_SCRIPTABLE QStringList | accounts () |
Q_SCRIPTABLE bool | canQueryClose () |
Q_SCRIPTABLE void | checkAccount (const QString &account) |
Q_SCRIPTABLE void | checkMail () |
void | dumpDeadLetters () |
Q_SCRIPTABLE bool | handleCommandLine (bool noArgsOpensReader) |
Q_SCRIPTABLE void | makeResourceOnline (MessageViewer::Viewer::ResourceOnlineMode mode) |
Q_SCRIPTABLE QDBusObjectPath | newMessage (const QString &to, const QString &cc, const QString &bcc, bool hidden, bool useFolderId, const QString &messageFile, const QString &attachURL) |
Q_SCRIPTABLE int | openComposer (const QString &to, const QString &cc, const QString &bcc, const QString &subject, const QString &body, bool hidden, const QString &messageFile, const QStringList &attachmentPaths, const QStringList &customHeaders, const QString &replyTo=QString(), const QString &inReplyTo=QString()) |
Q_SCRIPTABLE int | openComposer (const QString &to, const QString &cc, const QString &bcc, const QString &subject, const QString &body, bool hidden, const QString &attachName, const QByteArray &attachCte, const QByteArray &attachData, const QByteArray &attachType, const QByteArray &attachSubType, const QByteArray &attachParamAttr, const QString &attachParamValue, const QByteArray &attachContDisp, const QByteArray &attachCharset, unsigned int identity) |
Q_SCRIPTABLE QDBusObjectPath | openComposer (const QString &to, const QString &cc, const QString &bcc, const QString &subject, const QString &body, bool hidden) |
Q_SCRIPTABLE void | openReader () |
Q_SCRIPTABLE void | pauseBackgroundJobs () |
Q_SCRIPTABLE void | resumeBackgroundJobs () |
Q_SCRIPTABLE void | resumeNetworkJobs () |
Q_SCRIPTABLE bool | selectFolder (const QString &folder) |
Q_SCRIPTABLE void | setSystrayIconNotificationsEnabled (bool enabled) |
Q_SCRIPTABLE void | showFolder (const QString &collectionId) |
Q_SCRIPTABLE bool | showMail (quint32 serialNumber, const QString &messageId) |
void | slotConfigChanged () |
void | slotRequestConfigSync () |
void | slotRunBackgroundTasks () |
void | slotShowConfigurationDialog () |
void | slotSyncConfig () |
Q_SCRIPTABLE void | stopNetworkJobs () |
Q_SCRIPTABLE void | updateConfig () |
void | updatedTemplates () |
void | updateSystemTray () |
Q_SCRIPTABLE int | viewMessage (const QString &messageFile) |
Signals | |
void | configChanged () |
void | customTemplatesChanged () |
void | endCheckMail () |
void | onlineStatusChanged (GlobalSettings::EnumNetworkState::type) |
void | startCheckMail () |
Public Member Functions | |
KMKernel (QObject *parent=0, const char *name=0) | |
~KMKernel () | |
void | action (bool mailto, bool check, const QString &to, const QString &cc, const QString &bcc, const QString &subj, const QString &body, const KUrl &messageFile, const KUrl::List &attach, const QStringList &customHeaders, const QString &replyTo, const QString &inReplyTo) |
Akonadi::Collection::List | allFolders () const |
void | checkFolderFromResources (const Akonadi::Collection::List &collectionList) |
void | checkMailOnStartup () |
void | cleanup (void) |
void | closeAllKMailWindows () |
qreal | closeToQuotaThreshold () |
Akonadi::EntityMimeTypeFilterModel * | collectionModel () const |
MessageComposer::ComposerAutoCorrection * | composerAutoCorrection () |
KSharedConfig::Ptr | config () |
void | createFilter (const QByteArray &field, const QString &value) |
QStringList | customTemplates () |
bool | doSessionManagement () |
Akonadi::EntityTreeModel * | entityTreeModel () const |
bool | excludeImportantMailFromExpiry () |
void | expireAllFoldersNow () |
bool | firstInstance () const |
bool | firstStart () const |
void | firstStartDone () |
Akonadi::ChangeRecorder * | folderCollectionMonitor () const |
KMMainWidget * | getKMMainWidget () |
bool | haveSystemTrayApplet () const |
KPIMIdentities::IdentityManager * | identityManager () |
void | init () |
bool | isImapFolder (const Akonadi::Collection &, bool &isOnline) const |
MailCommon::JobScheduler * | jobScheduler () const |
Akonadi::Collection::Id | lastSelectedFolder () |
KMainWindow * | mainWin () |
MessageComposer::MessageSender * | msgSender () |
QTextCodec * | networkCodec () |
void | openFilterDialog (bool createDummyFilter=true) |
QString | previousVersion () const |
void | quit () |
void | raise () |
void | readConfig () |
void | recoverDeadLetters () |
void | savePaneSelection () |
void | selectCollectionFromId (const Akonadi::Collection::Id id) |
void | setAccountStatus (bool) |
void | setFirstInstance (bool value) |
void | setLastSelectedFolder (const Akonadi::Collection::Id &col) |
void | setShuttingDown (bool flag) |
void | setStartingUp (bool flag) |
void | setupDBus () |
void | setXmlGuiInstance (const KComponentData &instance) |
bool | showPopupAfterDnD () |
bool | shuttingDown () const |
bool | startingUp () const |
void | stopAgentInstance () |
void | syncConfig () |
void | toggleSystemTray () |
const QAbstractItemModel * | treeviewModelSelection () |
UndoStack * | undoStack () |
void | updatePaneTagComboBox () |
int | wrapCol () const |
const KComponentData & | xmlGuiInstance () |
Static Public Member Functions | |
static bool | askToGoOnline () |
static bool | isOffline () |
static QString | localDataPath () |
static KMKernel * | self () |
Protected Member Functions | |
void | agentInstanceBroken (const Akonadi::AgentInstance &instance) |
Detailed Description
Central point of coordination in KMail.
The KMKernel class represents the core of KMail, where the different parts come together and are coordinated. It is currently also the class which exports KMail's main D-BUS interfaces.
The kernel is responsible for creating various (singleton) objects such as the identity manager and the message sender.
The kernel also creates an Akonadi Session, Monitor and EntityTreeModel. These are shared so that other objects in KMail have access to it. Having only one EntityTreeModel instead of many reduces the overall communication with the Akonadi server.
The kernel also manages some stuff that should be factored out:
- default collection handling, like inboxCollectionFolder()
- job handling, like jobScheduler()
- handling of some config settings, like wrapCol()
- various other stuff
Definition at line 103 of file kmkernel.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 124 of file kmkernel.cpp.
KMKernel::~KMKernel | ( | ) |
Definition at line 236 of file kmkernel.cpp.
Member Function Documentation
|
slot |
Definition at line 470 of file kmkernel.cpp.
void KMKernel::action | ( | bool | mailto, |
bool | check, | ||
const QString & | to, | ||
const QString & | cc, | ||
const QString & | bcc, | ||
const QString & | subj, | ||
const QString & | body, | ||
const KUrl & | messageFile, | ||
const KUrl::List & | attach, | ||
const QStringList & | customHeaders, | ||
const QString & | replyTo, | ||
const QString & | inReplyTo | ||
) |
Definition at line 1316 of file kmkernel.cpp.
|
protected |
Definition at line 1733 of file kmkernel.cpp.
Akonadi::Collection::List KMKernel::allFolders | ( | ) | const |
Returns a list of all currently loaded folders.
Since folders are loaded async, this is empty at startup.
Definition at line 1562 of file kmkernel.cpp.
|
static |
A static helper function that asks the user if they want to go online.
- Returns
- true if the user wants to go online
- false if the user wants to stay offline
Definition at line 988 of file kmkernel.cpp.
|
slot |
Definition at line 1572 of file kmkernel.cpp.
|
slot |
Checks the account with the specified name for new mail.
If the account name is empty, all accounts not excluded from manual mail check will be checked.
Definition at line 483 of file kmkernel.cpp.
void KMKernel::checkFolderFromResources | ( | const Akonadi::Collection::List & | collectionList | ) |
Definition at line 1863 of file kmkernel.cpp.
|
slot |
Start of D-Bus callable stuff.
The D-Bus methods need to be public slots, otherwise they can't be accessed.
Definition at line 436 of file kmkernel.cpp.
void KMKernel::checkMailOnStartup | ( | ) |
End of D-Bus callable stuff.
Definition at line 964 of file kmkernel.cpp.
void KMKernel::cleanup | ( | void | ) |
Definition at line 1250 of file kmkernel.cpp.
void KMKernel::closeAllKMailWindows | ( | ) |
Definition at line 1218 of file kmkernel.cpp.
qreal KMKernel::closeToQuotaThreshold | ( | ) |
Definition at line 1825 of file kmkernel.cpp.
Akonadi::EntityMimeTypeFilterModel * KMKernel::collectionModel | ( | ) | const |
Returns a model of all folders in KMail.
This is basically the same as entityTreeModel(), but with items filtered out, the model contains only collections.
Definition at line 261 of file kmkernel.cpp.
MessageComposer::ComposerAutoCorrection * KMKernel::composerAutoCorrection | ( | ) |
Definition at line 2004 of file kmkernel.cpp.
KSharedConfig::Ptr KMKernel::config | ( | ) |
Definition at line 1456 of file kmkernel.cpp.
|
signal |
void KMKernel::createFilter | ( | const QByteArray & | field, |
const QString & | value | ||
) |
Definition at line 1856 of file kmkernel.cpp.
QStringList KMKernel::customTemplates | ( | ) |
Definition at line 1840 of file kmkernel.cpp.
|
signal |
bool KMKernel::doSessionManagement | ( | ) |
Definition at line 1201 of file kmkernel.cpp.
|
slot |
Save contents of all open composer widnows to ~/dead.letter.
Definition at line 1296 of file kmkernel.cpp.
|
signal |
Akonadi::EntityTreeModel * KMKernel::entityTreeModel | ( | ) | const |
Returns the main model, which contains all folders and the items of recently opened folders.
Definition at line 256 of file kmkernel.cpp.
bool KMKernel::excludeImportantMailFromExpiry | ( | ) |
Definition at line 1820 of file kmkernel.cpp.
void KMKernel::expireAllFoldersNow | ( | ) |
Expire all folders, used for the gui action.
Definition at line 1567 of file kmkernel.cpp.
|
inline |
Definition at line 335 of file kmkernel.h.
|
inline |
Definition at line 366 of file kmkernel.h.
|
inline |
Mark first start as done.
Definition at line 368 of file kmkernel.h.
Akonadi::ChangeRecorder * KMKernel::folderCollectionMonitor | ( | ) | const |
Definition at line 251 of file kmkernel.cpp.
KMMainWidget * KMKernel::getKMMainWidget | ( | ) |
Get first mainwidget.
Definition at line 1515 of file kmkernel.cpp.
|
slot |
Definition at line 285 of file kmkernel.cpp.
bool KMKernel::haveSystemTrayApplet | ( | ) | const |
Returns true if we have a system tray applet.
This is needed in order to know whether the application should be allowed to exit in case the last visible composer or separate message window is closed.
Definition at line 1406 of file kmkernel.cpp.
KPIMIdentities::IdentityManager * KMKernel::identityManager | ( | ) |
return the pointer to the identity manager
Definition at line 1418 of file kmkernel.cpp.
void KMKernel::init | ( | ) |
Definition at line 1152 of file kmkernel.cpp.
bool KMKernel::isImapFolder | ( | const Akonadi::Collection & | col, |
bool & | isOnline | ||
) | const |
Definition at line 1772 of file kmkernel.cpp.
|
static |
Checks if the current network state is online or offline.
- Returns
- true if the network state is offline
- false if the network state is online
Definition at line 952 of file kmkernel.cpp.
|
inline |
Definition at line 359 of file kmkernel.h.
Akonadi::Entity::Id KMKernel::lastSelectedFolder | ( | ) |
Definition at line 1830 of file kmkernel.cpp.
|
static |
Returns the full path of the user's local data directory for KMail.
The path ends with '/'.
Definition at line 1399 of file kmkernel.cpp.
KMainWindow * KMKernel::mainWin | ( | ) |
returns a reference to the first Mainwin or a temporary Mainwin
Definition at line 1426 of file kmkernel.cpp.
|
slot |
Definition at line 1992 of file kmkernel.cpp.
MessageComposer::MessageSender * KMKernel::msgSender | ( | ) |
Definition at line 1603 of file kmkernel.cpp.
|
inline |
Definition at line 386 of file kmkernel.h.
|
slot |
Opens a composer window and prefills it with different message parts.
- Returns
- The DBus object path for the composer.
- Parameters
-
to A comma separated list of To addresses. cc A comma separated list of CC addresses. bcc A comma separated list of BCC addresses. subject The message subject. body The message body. hidden Whether the composer window shall initially be hidden. useFolderId The id of the folder whose associated identity will be used. messageFile A message file that will be used as message body. attachURL The URL to the file that will be attached to the message.
Definition at line 788 of file kmkernel.cpp.
|
signal |
|
slot |
Opens a composer window and prefills it with different message parts.
- Returns
- The id of composer if more are opened.
- Parameters
-
to A comma separated list of To addresses. cc A comma separated list of CC addresses. bcc A comma separated list of BCC addresses. subject The message subject. body The message body. hidden Whether the composer window shall initially be hidden. messageFile A message file that will be used as message body. attachmentPaths A list of files that will be attached to the message. customHeaders A list of custom headers.
Definition at line 534 of file kmkernel.cpp.
|
slot |
Opens a composer window and prefills it with different message parts.
- Returns
- The id of composer if more are opened.
- Parameters
-
to A comma separated list of To addresses. cc A comma separated list of CC addresses. bcc A comma separated list of BCC addresses. subject The message subject. body The message body. hidden Whether the composer window shall initially be hidden. attachName The name of the attachment. attachCte The content transfer encoding of the attachment. attachData The raw data of the attachment. attachType The mime type of the attachment. attachSubType The sub mime type of the attachment. attachParamAttr The parameter attribute of the attachment. attachParamValue The parameter value of the attachment. attachContDisp The content display type of the attachment. attachCharset The charset of the attachment. identity The identity identifier which will be used as sender identity.
Definition at line 633 of file kmkernel.cpp.
|
slot |
Opens a composer window and prefills it with different message parts.
- Returns
- The DBus object path for the composer.
- Parameters
-
to A comma separated list of To addresses. cc A comma separated list of CC addresses. bcc A comma separated list of BCC addresses. subject The message subject. body The message body. hidden Whether the composer window shall initially be hidden.
Definition at line 747 of file kmkernel.cpp.
void KMKernel::openFilterDialog | ( | bool | createDummyFilter = true | ) |
Definition at line 1845 of file kmkernel.cpp.
|
inlineslot |
Definition at line 119 of file kmkernel.h.
|
slot |
Pauses all background jobs and does not allow new background jobs to be started.
Definition at line 887 of file kmkernel.cpp.
|
inline |
Definition at line 369 of file kmkernel.h.
void KMKernel::quit | ( | ) |
Definition at line 1040 of file kmkernel.cpp.
void KMKernel::raise | ( | ) |
Definition at line 842 of file kmkernel.cpp.
void KMKernel::readConfig | ( | ) |
Definition at line 1191 of file kmkernel.cpp.
void KMKernel::recoverDeadLetters | ( | ) |
Definition at line 1094 of file kmkernel.cpp.
|
slot |
Resumes all background jobs and allows new jobs to be started.
Definition at line 893 of file kmkernel.cpp.
|
slot |
Resumes all network related jobs and enter online mode New network jobs can be started.
Definition at line 931 of file kmkernel.cpp.
void KMKernel::savePaneSelection | ( | ) |
Definition at line 1963 of file kmkernel.cpp.
void KMKernel::selectCollectionFromId | ( | const Akonadi::Collection::Id | id | ) |
Definition at line 1486 of file kmkernel.cpp.
|
slot |
Definition at line 1499 of file kmkernel.cpp.
|
static |
normal control stuff
Definition at line 1451 of file kmkernel.cpp.
void KMKernel::setAccountStatus | ( | bool | goOnline | ) |
Definition at line 912 of file kmkernel.cpp.
|
inline |
Definition at line 336 of file kmkernel.h.
void KMKernel::setLastSelectedFolder | ( | const Akonadi::Collection::Id & | col | ) |
Definition at line 1835 of file kmkernel.cpp.
|
inline |
Definition at line 373 of file kmkernel.h.
|
inline |
Definition at line 371 of file kmkernel.h.
|
slot |
Enables/disables systray icon changing when mail arrives.
With this disabled the systray icon will always be the same.
Definition at line 463 of file kmkernel.cpp.
void KMKernel::setupDBus | ( | ) |
Definition at line 266 of file kmkernel.cpp.
|
inline |
Definition at line 348 of file kmkernel.h.
|
slot |
Definition at line 2031 of file kmkernel.cpp.
|
slot |
Definition at line 857 of file kmkernel.cpp.
bool KMKernel::showPopupAfterDnD | ( | ) |
Definition at line 1815 of file kmkernel.cpp.
|
inline |
Definition at line 372 of file kmkernel.h.
|
slot |
Definition at line 1390 of file kmkernel.cpp.
|
slot |
Call this slot instead of directly KConfig::sync() to minimize the overall config writes.
Calling this slot will schedule a sync of the application config file using a timer, so that many consecutive calls can be condensed into a single sync, which is more efficient.
Definition at line 1338 of file kmkernel.cpp.
|
slot |
Definition at line 1529 of file kmkernel.cpp.
|
slot |
Definition at line 1361 of file kmkernel.cpp.
|
slot |
Sync the config immediatley.
Definition at line 1344 of file kmkernel.cpp.
|
signal |
|
inline |
Definition at line 370 of file kmkernel.h.
void KMKernel::stopAgentInstance | ( | ) |
Definition at line 1781 of file kmkernel.cpp.
|
slot |
Stops all network related jobs and enter offline mode New network jobs cannot be started.
Definition at line 899 of file kmkernel.cpp.
void KMKernel::syncConfig | ( | ) |
Definition at line 1480 of file kmkernel.cpp.
void KMKernel::toggleSystemTray | ( | ) |
Definition at line 2009 of file kmkernel.cpp.
const QAbstractItemModel * KMKernel::treeviewModelSelection | ( | ) |
Definition at line 1902 of file kmkernel.cpp.
|
inline |
Definition at line 350 of file kmkernel.h.
|
slot |
Definition at line 1356 of file kmkernel.cpp.
|
slot |
Custom templates have changed, so all windows using them need to regenerate their menus.
Definition at line 1766 of file kmkernel.cpp.
void KMKernel::updatePaneTagComboBox | ( | ) |
Definition at line 1971 of file kmkernel.cpp.
|
slot |
Definition at line 1411 of file kmkernel.cpp.
|
slot |
Definition at line 834 of file kmkernel.cpp.
|
inline |
Definition at line 364 of file kmkernel.h.
|
inline |
Definition at line 347 of file kmkernel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:52 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.