knode
#include <knfolder.h>
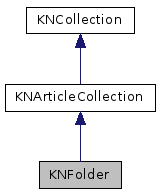
Classes | |
class | DynData |
Public Types | |
typedef QList< KNFolder::Ptr > | List |
typedef boost::shared_ptr < KNFolder > | Ptr |
![]() | |
typedef QList < KNArticleCollection::Ptr > | List |
typedef boost::shared_ptr < KNArticleCollection > | Ptr |
![]() | |
enum | collectionType { CTnntpAccount, CTgroup, CTfolder, CTcategory, CTvirtualGroup } |
typedef boost::shared_ptr < KNCollection > | Ptr |
Protected Member Functions | |
void | closeFiles () |
Protected Attributes | |
int | i_d |
bool | i_ndexDirty |
QFile | i_ndexFile |
QString | i_nfoPath |
QFile | m_boxFile |
int | p_arentId |
bool | w_asOpen |
![]() | |
int | c_ount |
KNCollectionViewItem * | l_istItem |
QString | n_ame |
KNCollection::Ptr | p_arent |
Detailed Description
Representation of a folder.
This includes:
- Information about the folder (eg. name, parent)
- Methods to load the folder content from a mbox file.
- Methods to store the folder content in a mbox file.
Definition at line 30 of file knfolder.h.
Member Typedef Documentation
typedef QList<KNFolder::Ptr> KNFolder::List |
List of folders.
Definition at line 42 of file knfolder.h.
typedef boost::shared_ptr<KNFolder> KNFolder::Ptr |
Shared pointer to a KNFolder.
To be used instead of raw KNFolder*.
Definition at line 38 of file knfolder.h.
Constructor & Destructor Documentation
KNFolder::KNFolder | ( | ) |
Definition at line 39 of file knfolder.cpp.
KNFolder::KNFolder | ( | int | id, |
const QString & | name, | ||
KNFolder::Ptr | parent = KNFolder::Ptr() |
||
) |
Definition at line 46 of file knfolder.cpp.
KNFolder::KNFolder | ( | int | id, |
const QString & | name, | ||
const QString & | prefix, | ||
KNFolder::Ptr | parent = KNFolder::Ptr() |
||
) |
Definition at line 65 of file knfolder.cpp.
KNFolder::~KNFolder | ( | ) |
Definition at line 84 of file knfolder.cpp.
Member Function Documentation
|
inline |
Definition at line 70 of file knfolder.h.
|
inline |
Definition at line 72 of file knfolder.h.
|
inline |
Definition at line 74 of file knfolder.h.
|
protected |
Definition at line 537 of file knfolder.cpp.
void KNFolder::deleteAll | ( | ) |
Definition at line 489 of file knfolder.cpp.
void KNFolder::deleteFiles | ( | ) |
Definition at line 505 of file knfolder.cpp.
|
inline |
Definition at line 53 of file knfolder.h.
|
inline |
Definition at line 57 of file knfolder.h.
|
inline |
Definition at line 56 of file knfolder.h.
bool KNFolder::loadArticle | ( | KNLocalArticle::Ptr | a | ) |
Load the full content of an article.
- Parameters
-
a the article to load.
- Returns
- true if the article is successfully loaded.
Definition at line 314 of file knfolder.cpp.
bool KNFolder::loadHdrs | ( | ) |
Definition at line 169 of file knfolder.cpp.
|
inline |
Definition at line 55 of file knfolder.h.
|
virtual |
Implements KNCollection.
Definition at line 100 of file knfolder.cpp.
|
virtual |
Load the properties/settings of this collection.
Implements KNCollection.
Definition at line 109 of file knfolder.cpp.
bool KNFolder::readInfo | ( | ) |
Definition at line 140 of file knfolder.cpp.
void KNFolder::removeArticles | ( | KNLocalArticle::List & | l, |
bool | del = true |
||
) |
Definition at line 449 of file knfolder.cpp.
bool KNFolder::saveArticles | ( | KNLocalArticle::List & | l | ) |
Definition at line 355 of file knfolder.cpp.
|
inline |
Definition at line 54 of file knfolder.h.
|
virtual |
Sets the parent collection.
Reimplemented from KNCollection.
Definition at line 162 of file knfolder.cpp.
void KNFolder::syncIndex | ( | bool | force = false | ) |
Definition at line 513 of file knfolder.cpp.
|
inlinevirtual |
bool KNFolder::unloadHdrs | ( | bool | force = true | ) |
Definition at line 293 of file knfolder.cpp.
|
virtual |
Updates the listview item after the collection has changed.
Reimplemented from KNCollection.
Definition at line 90 of file knfolder.cpp.
|
inline |
Definition at line 61 of file knfolder.h.
|
virtual |
Save the properties/settings of this collection.
Implements KNCollection.
Definition at line 146 of file knfolder.cpp.
Member Data Documentation
|
protected |
Definition at line 99 of file knfolder.h.
|
protected |
Definition at line 101 of file knfolder.h.
|
protected |
Definition at line 104 of file knfolder.h.
|
protected |
Definition at line 105 of file knfolder.h.
|
protected |
Definition at line 103 of file knfolder.h.
|
protected |
Definition at line 100 of file knfolder.h.
|
protected |
Definition at line 102 of file knfolder.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.