knode
#include <knjobdata.h>
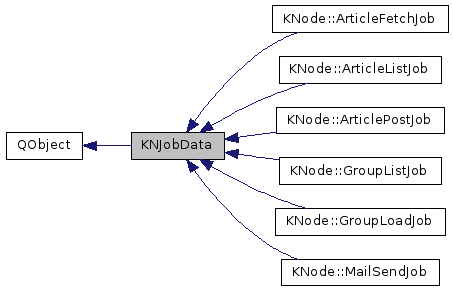
Public Types | |
enum | jobType { JTLoadGroups =1, JTFetchGroups, JTfetchNewHeaders, JTfetchArticle, JTpostArticle, JTmail, JTfetchSource } |
Signals | |
void | finished (KNJobData *) |
Public Member Functions | |
KNJobData (jobType t, KNJobConsumer *c, KNServerInfo::Ptr a, KNJobItem::Ptr i) | |
~KNJobData () | |
KNServerInfo::Ptr | account () const |
void | cancel () |
bool | canceled () const |
void | createProgressItem () |
KNJobItem::Ptr | data () const |
int | error () const |
QString | errorString () const |
virtual void | execute ()=0 |
void | notifyConsumer () |
void | prepareForExecution () |
KPIM::ProgressItem * | progressItem () const |
void | setComplete () |
void | setError (int err, const QString &errMsg) |
void | setProgress (unsigned int progress) |
void | setStatus (const QString &msg) |
bool | success () const |
jobType | type () const |
Protected Member Functions | |
KUrl | baseUrl () const |
void | emitFinished () |
void | setupKIOJob (KIO::Job *job) |
void | setupKJob (KJob *job) |
Protected Attributes | |
KNServerInfo::Ptr | a_ccount |
KNJobConsumer * | c_onsumer |
KNJobItem::Ptr | d_ata |
bool | mCanceled |
int | mError |
QString | mErrorString |
QPointer< KJob > | mJob |
KPIM::ProgressItem * | mProgressItem |
jobType | t_ype |
Detailed Description
Abstract base class for all KNode internal jobs.
This class takes care of:
- progress/status reporting and user interaction (cancellation).
- error handling/reporting.
- easy handling of associated KIO jobs. To imlpement a new job class, you need to sub-class this class and implement the execute() method.
Definition at line 101 of file knjobdata.h.
Member Enumeration Documentation
enum KNJobData::jobType |
Enumerator | |
---|---|
JTLoadGroups | |
JTFetchGroups | |
JTfetchNewHeaders | |
JTfetchArticle | |
JTpostArticle | |
JTmail | |
JTfetchSource |
Definition at line 109 of file knjobdata.h.
Constructor & Destructor Documentation
KNJobData::KNJobData | ( | jobType | t, |
KNJobConsumer * | c, | ||
KNServerInfo::Ptr | a, | ||
KNJobItem::Ptr | i | ||
) |
Definition at line 75 of file knjobdata.cpp.
KNJobData::~KNJobData | ( | ) |
Definition at line 88 of file knjobdata.cpp.
Member Function Documentation
|
inline |
Definition at line 122 of file knjobdata.h.
|
protected |
Returns a correctly set up KUrl according to the encryption and authentication settings for KIO slave operations.
Definition at line 185 of file knjobdata.cpp.
void KNJobData::cancel | ( | ) |
Cancels this job.
If the job is currently active, this cancels the associated KIO job and emits the finished signal.
Definition at line 105 of file knjobdata.cpp.
|
inline |
Returns true if the job has been canceled by the user.
Definition at line 132 of file knjobdata.h.
void KNJobData::createProgressItem | ( | ) |
Creates a KPIM::ProgressItem for this job.
Definition at line 149 of file knjobdata.cpp.
|
inline |
Definition at line 123 of file knjobdata.h.
|
protected |
Emits the finished() signal via a single-shot timer.
Definition at line 119 of file knjobdata.cpp.
|
inline |
Returns the error code (see KIO::Error).
Definition at line 126 of file knjobdata.h.
|
inline |
Returns the error message.
Definition at line 128 of file knjobdata.h.
|
pure virtual |
Performs the actual operation of a job, needs to be reimplemented for every job.
Note that a job might be executed multiple times e.g. in case of an authentication error.
Implemented in KNode::ArticlePostJob, KNode::ArticleFetchJob, KNode::ArticleListJob, KNode::GroupLoadJob, KNode::GroupListJob, and KNode::MailSendJob.
|
signal |
Emitted when a job has been finished.
It's recommended to to emit it via emitFinished().
void KNJobData::notifyConsumer | ( | ) |
Definition at line 96 of file knjobdata.cpp.
|
inline |
Definition at line 146 of file knjobdata.h.
|
inline |
Returns the progress item for this job.
Definition at line 157 of file knjobdata.h.
|
inline |
Tells the progress item to indicate that the job has finished if available.
This causes the destruction of the progress item.
Definition at line 172 of file knjobdata.h.
void KNJobData::setError | ( | int | err, |
const QString & | errMsg | ||
) |
Set job error information.
- Parameters
-
err The error code (see KIO::Error). errMsg A translated error message.
Definition at line 201 of file knjobdata.cpp.
|
inline |
Set the progress value of the progress item if available.
- Parameters
-
progress The new progress value.
Definition at line 168 of file knjobdata.h.
|
inline |
Set the status message of the progress item if available.
- Parameters
-
msg The new status message.
Definition at line 164 of file knjobdata.h.
|
protected |
Sets TLS metadata and connects the given KIO job to the progress item.
- Parameters
-
job The KIO job to setup.
Definition at line 135 of file knjobdata.cpp.
|
protected |
Connects progress signals.
- Parameters
-
job The KJob to setup.
Definition at line 124 of file knjobdata.cpp.
|
inline |
Returns true if the job finished successfully.
Definition at line 130 of file knjobdata.h.
|
inline |
Definition at line 120 of file knjobdata.h.
Member Data Documentation
|
protected |
Definition at line 203 of file knjobdata.h.
|
protected |
Definition at line 210 of file knjobdata.h.
|
protected |
Definition at line 202 of file knjobdata.h.
|
protected |
Cancel status flag.
Definition at line 209 of file knjobdata.h.
|
protected |
The job error code (see KIO::Error).
Definition at line 205 of file knjobdata.h.
|
protected |
The error message.
Definition at line 207 of file knjobdata.h.
|
protected |
An associated KJob.
Definition at line 212 of file knjobdata.h.
|
protected |
The progress item representing this job to the user.
Definition at line 214 of file knjobdata.h.
|
protected |
Definition at line 201 of file knjobdata.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.