knode
#include <knnntpaccount.h>
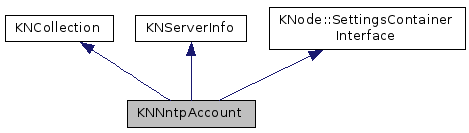
Public Types | |
typedef QList< KNNntpAccount::Ptr > | List |
typedef boost::shared_ptr < KNNntpAccount > | Ptr |
![]() | |
enum | collectionType { CTnntpAccount, CTgroup, CTfolder, CTcategory, CTvirtualGroup } |
typedef boost::shared_ptr < KNCollection > | Ptr |
![]() | |
enum | Encryption { None, SSL, TLS } |
typedef boost::shared_ptr < KNServerInfo > | Ptr |
Public Member Functions | |
KNNntpAccount () | |
~KNNntpAccount () | |
KNode::Cleanup * | activeCleanupConfig () const |
int | checkInterval () const |
KNode::Cleanup * | cleanupConfig () const |
bool | editProperties (QWidget *parent) |
bool | fetchDescriptions () const |
virtual const KPIMIdentities::Identity & | identity () const |
bool | intervalChecking () const |
QDate | lastNewFetch () const |
QString | path () |
bool | readInfo (const QString &confPath) |
void | setCheckInterval (int c) |
void | setFetchDescriptions (bool b) |
virtual void | setIdentity (const KPIMIdentities::Identity &identity) |
void | setIntervalChecking (bool b) |
void | setLastNewFetch (QDate date) |
void | setUseDiskCache (bool b) |
void | startTimer () |
collectionType | type () |
bool | useDiskCache () const |
bool | wasOpen () const |
void | writeConfig () |
![]() | |
KNCollection (KNCollection::Ptr p) | |
virtual | ~KNCollection () |
int | count () const |
void | decCount (int i) |
void | incCount (int i) |
KNCollectionViewItem * | listItem () const |
virtual const QString & | name () |
KNCollection::Ptr | parent () const |
void | setCount (int i) |
void | setListItem (KNCollectionViewItem *i) |
void | setName (const QString &s) |
virtual void | setParent (KNCollection::Ptr p) |
virtual void | updateListItem () |
![]() | |
KNServerInfo () | |
~KNServerInfo () | |
Encryption | encryption () const |
int | id () const |
bool | isEmpty () const |
bool | needsLogon () const |
bool | operator== (const KNServerInfo &s) const |
const QString & | pass () |
int | port () const |
void | readConf (KConfigGroup &conf) |
void | readPassword () |
bool | readyForLogin () const |
void | saveConf (KConfigGroup &conf) |
const QString & | server () |
void | setEncryption (Encryption enc) |
void | setId (int i) |
void | setNeedsLogon (bool b) |
void | setPass (const QString &s) |
void | setPort (int p) |
void | setServer (const QString &s) |
void | setUser (const QString &s) |
const QString & | user () |
![]() | |
virtual | ~SettingsContainerInterface () |
Protected Member Functions | |
virtual KNCollection::Ptr | selfPtr () |
![]() | |
SettingsContainerInterface () | |
Protected Attributes | |
KNNntpAccountIntervalChecking * | a_ccountIntervalChecking |
int | c_heckInterval |
bool | f_etchDescriptions |
bool | i_ntervalChecking |
QDate | l_astNewFetch |
KNode::Cleanup * | mCleanupConf |
int | mIdentityUoid |
bool | u_seDiskCache |
bool | w_asOpen |
![]() | |
int | c_ount |
KNCollectionViewItem * | l_istItem |
QString | n_ame |
KNCollection::Ptr | p_arent |
![]() | |
int | i_d |
Encryption | mEncryption |
bool | mPassLoaded |
bool | n_eedsLogon |
QString | p_ass |
bool | p_assDirty |
int | p_ort |
QString | s_erver |
QString | u_ser |
Detailed Description
Represents an account on a news server.
Definition at line 56 of file knnntpaccount.h.
Member Typedef Documentation
typedef QList<KNNntpAccount::Ptr> KNNntpAccount::List |
List of accounts.
Definition at line 66 of file knnntpaccount.h.
typedef boost::shared_ptr<KNNntpAccount> KNNntpAccount::Ptr |
Shared pointer to a KNNntpAccount.
To be used instead of raw KNNntpAccount*.
Definition at line 62 of file knnntpaccount.h.
Constructor & Destructor Documentation
KNNntpAccount::KNNntpAccount | ( | ) |
Definition at line 79 of file knnntpaccount.cpp.
KNNntpAccount::~KNNntpAccount | ( | ) |
Definition at line 90 of file knnntpaccount.cpp.
Member Function Documentation
KNode::Cleanup * KNNntpAccount::activeCleanupConfig | ( | ) | const |
Returns the cleanup configuration that should be used for this account.
Definition at line 205 of file knnntpaccount.cpp.
|
inline |
Definition at line 102 of file knnntpaccount.h.
|
inline |
Definition at line 103 of file knnntpaccount.h.
bool KNNntpAccount::editProperties | ( | QWidget * | parent | ) |
returns true when the user accepted
Definition at line 173 of file knnntpaccount.cpp.
|
inline |
Definition at line 85 of file knnntpaccount.h.
|
virtual |
Returns this server's specific identity or the null identity if there is none.
Implements KNode::SettingsContainerInterface.
Definition at line 213 of file knnntpaccount.cpp.
|
inline |
Definition at line 101 of file knnntpaccount.h.
|
inline |
Definition at line 86 of file knnntpaccount.h.
|
virtual |
Implements KNCollection.
Definition at line 160 of file knnntpaccount.cpp.
|
virtual |
tries to read information, returns false if it fails to do so
Implements KNCollection.
Definition at line 98 of file knnntpaccount.cpp.
|
protectedvirtual |
Reimplemented from KNArticleCollection::selfPtr().
Implements KNCollection.
Definition at line 227 of file knnntpaccount.cpp.
void KNNntpAccount::setCheckInterval | ( | int | c | ) |
Definition at line 199 of file knnntpaccount.cpp.
|
inline |
Definition at line 109 of file knnntpaccount.h.
|
virtual |
Sets this server's specific identity.
- Parameters
-
identity this server's identity of a null identity to unset.
Implements KNode::SettingsContainerInterface.
Definition at line 221 of file knnntpaccount.cpp.
|
inline |
Definition at line 113 of file knnntpaccount.h.
|
inline |
Definition at line 110 of file knnntpaccount.h.
|
inline |
Definition at line 111 of file knnntpaccount.h.
void KNNntpAccount::startTimer | ( | ) |
Definition at line 187 of file knnntpaccount.cpp.
|
inlinevirtual |
Returns the collection type.
Implements KNCollection.
Definition at line 71 of file knnntpaccount.h.
|
inline |
Definition at line 88 of file knnntpaccount.h.
|
inline |
Definition at line 87 of file knnntpaccount.h.
|
virtual |
Save the configuration to disk.
Implements KNode::SettingsContainerInterface.
Definition at line 125 of file knnntpaccount.cpp.
Member Data Documentation
|
protected |
helper class for news interval checking, manages the QTimer
Definition at line 137 of file knnntpaccount.h.
|
protected |
Definition at line 134 of file knnntpaccount.h.
|
protected |
use an additional "list newsgroups" command to fetch the newsgroup descriptions
Definition at line 125 of file knnntpaccount.h.
|
protected |
is interval checking enabled
Definition at line 133 of file knnntpaccount.h.
|
protected |
last use of "newgroups"
Definition at line 127 of file knnntpaccount.h.
|
protected |
account specific cleanup configuration
Definition at line 123 of file knnntpaccount.h.
|
protected |
Unique object identifier of the identity of this server.
-1 means there is no specific identity for this group (because KPIMIdentities::Identity::uoid() returns an unsigned int.
Definition at line 121 of file knnntpaccount.h.
|
protected |
cache fetched articles on disk
Definition at line 131 of file knnntpaccount.h.
|
protected |
was the server open in the listview on the last shutdown?
Definition at line 129 of file knnntpaccount.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.