knode
#include <knarticle.h>
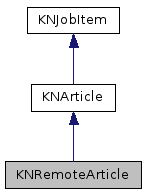
Public Types | |
typedef QList < KNRemoteArticle::Ptr > | List |
typedef boost::shared_ptr < KNRemoteArticle > | Ptr |
![]() | |
enum | articleType { ATmimeContent, ATremote, ATlocal } |
typedef QList< KNArticle::Ptr > | List |
typedef boost::shared_ptr < KNArticle > | Ptr |
![]() | |
typedef boost::shared_ptr < KNJobItem > | Ptr |
Public Member Functions | |
KNRemoteArticle (boost::shared_ptr< KNGroup > g) | |
~KNRemoteArticle () | |
int | articleNumber () const |
QColor | color () const |
void | decNewFollowUps (unsigned short s=1) |
void | decUnreadFollowUps (unsigned short s=1) |
KNRemoteArticle::Ptr | displayedReference () |
bool | filterResult () |
bool | getReadFlag () |
bool | hasChanged () |
bool | hasNewFollowUps () |
bool | hasUnreadFollowUps () |
bool | hasVisibleFollowUps () |
int | idRef () |
void | incNewFollowUps (unsigned short s=1) |
void | incUnreadFollowUps (unsigned short s=1) |
void | initListItem () |
bool | isExpired () |
bool | isFiltered () |
bool | isIgnored () |
bool | isKept () |
bool | isNew () |
bool | isRead () |
bool | isWatched () |
unsigned short | newFollowUps () |
virtual void | parse () |
void | propagateThreadChangedDate () |
short | score () |
void | setArticleNumber (int number) |
void | setChanged (bool b=true) |
void | setColor (const QColor &c) |
void | setDisplayedReference (KNRemoteArticle::Ptr dr) |
void | setExpired (bool b=true) |
void | setFiltered (bool b=true) |
void | setFilterResult (bool b=true) |
virtual void | setForceDefaultCharset (bool b) |
void | setIdRef (int i) |
void | setIgnored (bool b=true) |
void | setKept (bool b=true) |
void | setNew (bool b=true) |
void | setNewFollowUps (unsigned short s) |
void | setRead (bool b=true) |
void | setScore (short s) |
void | setSubThreadChangeDate (time_t date) |
void | setThreadingLevel (unsigned char l) |
void | setThreadMode (bool b=true) |
void | setUnreadFollowUps (unsigned short s) |
void | setVisibleFollowUps (bool b=true) |
void | setWatched (bool b=true) |
time_t | subThreadChangeDate () |
void | thread (List &f) |
unsigned char | threadingLevel () |
bool | threadMode () |
articleType | type () const |
unsigned short | unreadFollowUps () |
void | updateListItem () |
![]() | |
KNArticle (boost::shared_ptr< KNArticleCollection > c) | |
~KNArticle () | |
virtual void | clear () |
boost::shared_ptr < KNArticleCollection > | collection () const |
int | id () const |
bool | isLocked () |
bool | isNotUnloadable () |
bool | isOrphant () const |
KNHdrViewItem * | listItem () const |
void | setCollection (boost::shared_ptr< KNArticleCollection > c) |
void | setId (int i) |
void | setListItem (KNHdrViewItem *i, KNArticle::Ptr a) |
void | setLocked (bool b=true) |
void | setNotUnloadable (bool b=true) |
![]() | |
KNJobItem () | |
virtual | ~KNJobItem () |
virtual QString | prepareForExecution () |
Protected Attributes | |
int | a_rticleNumber |
QColor | c_olor |
KNRemoteArticle::Ptr | d_ref |
int | i_dRef |
unsigned short | n_ewFups |
short | s_core |
time_t | s_ubThreadChangeDate |
unsigned char | t_hrLevel |
unsigned short | u_nreadFups |
![]() | |
boost::shared_ptr < KNArticleCollection > | c_ol |
KMime::BoolFlags | f_lags |
int | i_d |
KNHdrViewItem * | i_tem |
Detailed Description
KNRemoteArticle represents an article, whos body has to be retrieved from a remote host or from the local cache.
All articles in a newsgroup are stored in instances of this class.
Definition at line 103 of file knarticle.h.
Member Typedef Documentation
List of remote articles.
Definition at line 109 of file knarticle.h.
typedef boost::shared_ptr<KNRemoteArticle> KNRemoteArticle::Ptr |
Shared pointer to a KNRemoteArticle. To be used instead of raw KNRemoteArticle*.
Definition at line 107 of file knarticle.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 76 of file knarticle.cpp.
KNRemoteArticle::~KNRemoteArticle | ( | ) |
Definition at line 88 of file knarticle.cpp.
Member Function Documentation
|
inline |
Definition at line 121 of file knarticle.h.
|
inline |
Definition at line 181 of file knarticle.h.
|
inline |
Definition at line 159 of file knarticle.h.
|
inline |
Definition at line 164 of file knarticle.h.
|
inline |
Definition at line 147 of file knarticle.h.
|
inline |
Definition at line 168 of file knarticle.h.
|
inline |
Definition at line 127 of file knarticle.h.
|
inline |
Definition at line 134 of file knarticle.h.
|
inline |
Definition at line 156 of file knarticle.h.
|
inline |
Definition at line 161 of file knarticle.h.
|
inline |
Definition at line 172 of file knarticle.h.
|
inline |
Definition at line 142 of file knarticle.h.
|
inline |
Definition at line 158 of file knarticle.h.
|
inline |
Definition at line 163 of file knarticle.h.
void KNRemoteArticle::initListItem | ( | ) |
Definition at line 113 of file knarticle.cpp.
|
inline |
Definition at line 130 of file knarticle.h.
|
inline |
Definition at line 170 of file knarticle.h.
|
inline |
Definition at line 136 of file knarticle.h.
|
inline |
Definition at line 132 of file knarticle.h.
|
inline |
Definition at line 125 of file knarticle.h.
|
inline |
Definition at line 128 of file knarticle.h.
|
inline |
Definition at line 138 of file knarticle.h.
|
inline |
Definition at line 155 of file knarticle.h.
|
virtual |
Definition at line 92 of file knarticle.cpp.
void KNRemoteArticle::propagateThreadChangedDate | ( | ) |
Definition at line 212 of file knarticle.cpp.
|
inline |
Definition at line 153 of file knarticle.h.
|
inline |
Definition at line 122 of file knarticle.h.
|
inline |
Definition at line 135 of file knarticle.h.
|
inline |
Definition at line 182 of file knarticle.h.
|
inline |
Definition at line 148 of file knarticle.h.
|
inline |
Definition at line 131 of file knarticle.h.
|
inline |
Definition at line 171 of file knarticle.h.
|
inline |
Definition at line 169 of file knarticle.h.
|
virtual |
Definition at line 201 of file knarticle.cpp.
|
inline |
Definition at line 143 of file knarticle.h.
|
inline |
Definition at line 137 of file knarticle.h.
|
inline |
Definition at line 133 of file knarticle.h.
|
inline |
Definition at line 126 of file knarticle.h.
|
inline |
Definition at line 157 of file knarticle.h.
|
inline |
Definition at line 129 of file knarticle.h.
|
inline |
Definition at line 154 of file knarticle.h.
|
inline |
Definition at line 185 of file knarticle.h.
|
inline |
Definition at line 152 of file knarticle.h.
|
inline |
Definition at line 150 of file knarticle.h.
|
inline |
Definition at line 162 of file knarticle.h.
|
inline |
Definition at line 173 of file knarticle.h.
|
inline |
Definition at line 139 of file knarticle.h.
|
inline |
Definition at line 184 of file knarticle.h.
void KNRemoteArticle::thread | ( | List & | f | ) |
Definition at line 169 of file knarticle.cpp.
|
inline |
Definition at line 151 of file knarticle.h.
|
inline |
Definition at line 149 of file knarticle.h.
|
inlinevirtual |
Reimplemented from KNArticle.
Definition at line 115 of file knarticle.h.
|
inline |
Definition at line 160 of file knarticle.h.
|
virtual |
Reimplemented from KNArticle.
Definition at line 130 of file knarticle.cpp.
Member Data Documentation
|
protected |
Definition at line 190 of file knarticle.h.
|
protected |
Definition at line 195 of file knarticle.h.
|
protected |
Definition at line 192 of file knarticle.h.
|
protected |
Definition at line 191 of file knarticle.h.
|
protected |
Definition at line 196 of file knarticle.h.
|
protected |
Definition at line 194 of file knarticle.h.
|
protected |
Definition at line 198 of file knarticle.h.
|
protected |
Definition at line 193 of file knarticle.h.
|
protected |
Definition at line 196 of file knarticle.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.