knode
#include <treewidget.h>
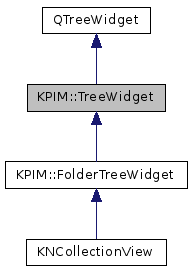
Signals | |
void | columnVisibilityChanged (int logicalIndex) |
Public Member Functions | |
TreeWidget (QWidget *parent, const char *name=0) | |
int | addColumn (const QString &label, int headerAlignment=Qt::AlignLeft|Qt::AlignVCenter) |
virtual bool | fillHeaderContextMenu (KMenu *menu, const QPoint &clickPoint) |
QTreeWidgetItem * | firstItem () const |
bool | isColumnHidden (int logicalIndex) const |
QTreeWidgetItem * | lastItem () const |
bool | manualColumnHidingEnabled () const |
bool | restoreLayout (KConfigGroup &group, const QString &keyName=QString()) |
bool | restoreLayout (KConfig *config, const QString &groupName, const QString &keyName=QString()) |
bool | saveLayout (KConfigGroup &group, const QString &keyName=QString()) const |
bool | saveLayout (KConfig *config, const QString &groupName, const QString &keyName=QString()) const |
void | setColumnHidden (int logicalIndex, bool hide) |
bool | setColumnText (int columnIndex, const QString &label) |
void | setManualColumnHidingEnabled (bool enable) |
void | toggleColumn (int logicalIndex) |
Protected Member Functions | |
virtual void | changeEvent (QEvent *e) |
Detailed Description
A QTreeWidget with expanded capabilities.
This class extends the functionality provided by the standard QTreeWidget by adding toggleable columns and a layout save/restore facility.
At the time of writing the usage of QTreeWidget is a bit against the reccomended Qt4 approach. On the other hand in many cases you just want a simple tree of items and don't want to care about anything complex like a "data model".
Definition at line 56 of file treewidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a TreeWidget.
Sets up the default values for properties.
Definition at line 39 of file treewidget.cpp.
Member Function Documentation
int KPIM::TreeWidget::addColumn | ( | const QString & | label, |
int | headerAlignment = Qt::AlignLeft | Qt::AlignVCenter |
||
) |
Convenience function that adds a column to this tree widget and returns its logical index.
Please note that in fact this function manipulates the header item which in turn plays with the underlying data model.
The alignment flags refer to the header text only. For individual items you must set the alignment manually.
- Parameters
-
label The label for the column alignment The alignment of the header text for this column
Definition at line 279 of file treewidget.cpp.
|
protectedvirtual |
Reimplemented in order to catch style change events and explicitly disable animations.
Definition at line 57 of file treewidget.cpp.
|
signal |
This signal is emitted when an existing column is shown or hidden either by the means of the popup menu or by a call to setColumnHidden().
See also setManualColumnHidingEnabled().
|
virtual |
Called by this class to fill the contextual menu shown when the user right clicks on one of the header columns and column hiding is enabled.
If you override this, remember to call the base class implementation if you want to keep column hiding working.
You should return true if you want the menu to be shown or false if you don't want it to be shown. The default implementation returns true unless you have no columns at all, column hiding is disabled or you pass some weird parameter.
Definition at line 212 of file treewidget.cpp.
QTreeWidgetItem * KPIM::TreeWidget::firstItem | ( | ) | const |
Convenience function that returns the first item in the view.
This, in fact, is roughly equivalent to topLevelItem( 0 ). It is included for symmetry with lastItem().
Definition at line 311 of file treewidget.cpp.
bool KPIM::TreeWidget::isColumnHidden | ( | int | logicalIndex | ) | const |
Returns true if the column specified by logicalIndex is actually hidden.
Definition at line 207 of file treewidget.cpp.
QTreeWidgetItem * KPIM::TreeWidget::lastItem | ( | ) | const |
Convenience function that returns the last item in the view.
Definition at line 318 of file treewidget.cpp.
|
inline |
- Returns
- true if manual column hiding is currently enabled, false otherwise.
Definition at line 169 of file treewidget.h.
bool KPIM::TreeWidget::restoreLayout | ( | KConfigGroup & | group, |
const QString & | keyName = QString() |
||
) |
Attempts to restore the layout of this tree from the specified key of the specified KConfigGroup.
The layout must have been previously saved by a call to saveLayout().
Please note that you must call this function after having added all the relevant columns to the view. On the other hand if you're setting some of the view properties programmatically (like default section size) then you should set them after restoring the layout because otherwise they might get overwritten by the stored data. The proper order is: add columns, restore layout, set header properties.
- Parameters
-
group The KConfigGroup to read the layout data from. keyName The key to use, "TreeWidgetLayout" by default
- Returns
- true if the layout data has been successfully read and restored. Returns false if the specified key of the config group is empty, or the data contained inside is not valid.
Definition at line 91 of file treewidget.cpp.
bool KPIM::TreeWidget::restoreLayout | ( | KConfig * | config, |
const QString & | groupName, | ||
const QString & | keyName = QString() |
||
) |
Attempts to restore the layout of this tree from the specified key of the specified group.
The layout must have been previously saved by a call to saveLayout().
Please note that you must call this function after having added all the relevant columns to the view. On the other hand if you're setting some of the view properties programmatically (like default section size) then you should set them after restoring the layout because otherwise they might get overwritten by the stored data. The proper order is: add columns, restore layout, set header properties.
- Parameters
-
configGroup The name of the KConfig group to read the layout data from. keyName The key to use, "TreeWidgetLayout" by default
- Returns
- true if the layout data has been successfully read and restored. Returns false if the specified key of the config group is empty, or the data contained inside is not valid.
Definition at line 170 of file treewidget.cpp.
bool KPIM::TreeWidget::saveLayout | ( | KConfigGroup & | group, |
const QString & | keyName = QString() |
||
) | const |
Stores the layout of this tree view to the specified KConfigGroup.
The stored data includes visible columns, column width and order.
- Parameters
-
group The KConfigGroup to write the layout data to. keyName The key to use, "TreeWidgetLayout" by default
- Returns
- true on success and false on failure (if keyName is 0, for example).
Definition at line 72 of file treewidget.cpp.
bool KPIM::TreeWidget::saveLayout | ( | KConfig * | config, |
const QString & | groupName, | ||
const QString & | keyName = QString() |
||
) | const |
Stores the layout of this tree view to the specified key of the specified config group.
The stored data includes visible columns, column width and order.
- Parameters
-
configGroup The KConfig group to write the layout data to. keyName The key to use, "TreeWidgetLayout" by default
- Returns
- true on success and false on failure (if you can't write to the configGroup).
Definition at line 82 of file treewidget.cpp.
void KPIM::TreeWidget::setColumnHidden | ( | int | logicalIndex, |
bool | hide | ||
) |
Hides or shows the column specified by logicalIndex.
The column is hidden if hide is true and shown otherwise.
Definition at line 198 of file treewidget.cpp.
bool KPIM::TreeWidget::setColumnText | ( | int | columnIndex, |
const QString & | label | ||
) |
Convenience function that changes the header text for the specified column.
Returns true if the text can be successfully changed or false if the specified column index is out of range.
Definition at line 300 of file treewidget.cpp.
void KPIM::TreeWidget::setManualColumnHidingEnabled | ( | bool | enable | ) |
Enables or disables manual column hiding by the means of a contextual menu.
All columns but the first can be hidden by the user by toggling the check on the popup menu that is displayed by right clicking the header of this view.
By default column hiding is enabled.
Definition at line 182 of file treewidget.cpp.
void KPIM::TreeWidget::toggleColumn | ( | int | logicalIndex | ) |
Toggle the visibility state of the specified column.
Definition at line 244 of file treewidget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:58:37 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.