korganizer
#include <actionmanager.h>
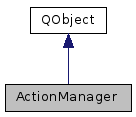
Public Slots | |
void | exportHTML () |
void | exportHTML (KOrg::HTMLExportSettings *settings, bool autoMode=false) |
void | goDate (const QDate &) |
void | goDate (const QString &) |
void | importCalendar (const KUrl &url) |
bool | importURL (const KUrl &url, bool merge) |
void | keyBindings () |
void | loadParts () |
void | openEventEditor (const QString &) |
void | openEventEditor (const QString &summary, const QString &description, const QStringList &attachments) |
void | openEventEditor (const QString &summary, const QString &description, const QStringList &attachments, const QStringList &attendees) |
void | openEventEditor (const QString &summary, const QString &description, const QString &uri, const QString &file, const QStringList &attendees, const QString &attachmentMimetype) |
void | openEventEditor (const QString &summary, const QString &description, const QStringList &attachmentUris, const QStringList &attendees, const QStringList &attachmentMimetypes, bool attachmentIsInline) |
void | openJournalEditor (const QDate &date) |
void | openJournalEditor (const QString &text, const QDate &date) |
void | openJournalEditor (const QString &text) |
void | openTodoEditor (const QString &) |
void | openTodoEditor (const QString &summary, const QString &description, const QStringList &attachments) |
void | openTodoEditor (const QString &summary, const QString &description, const QStringList &attachments, const QStringList &attendees) |
void | openTodoEditor (const QString &summary, const QString &description, const QString &uri, const QString &file, const QStringList &attendees, const QString &attachmentMimetype) |
void | openTodoEditor (const QString &summary, const QString &description, const QStringList &attachmentUris, const QStringList &attendees, const QStringList &attachmentMimetypes, bool attachmentIsInline) |
void | processIncidenceSelection (const Akonadi::Item &item, const QDate &date) |
void | readProperties (const KConfigGroup &) |
void | readSettings () |
bool | saveAsURL (const KUrl &kurl) |
void | saveProperties (KConfigGroup &) |
bool | saveURL () |
void | showDate (const QDate &date) |
void | showEventView () |
void | showJournalView () |
void | showTodoView () |
void | toggleMenubar (bool dontShowWarning=false) |
void | updateConfig () |
void | writeSettings () |
Signals | |
void | configChanged () |
void | toggleMenuBar () |
Public Member Functions | |
ActionManager (KXMLGUIClient *client, CalendarView *widget, QObject *parent, KOrg::MainWindow *mainWindow, bool isPart, KMenuBar *menuBar=0) | |
virtual | ~ActionManager () |
bool | addIncidence (const QString &ical) |
void | createCalendarAkonadi () |
virtual bool | deleteIncidence (Akonadi::Item::Id id, bool force=false) |
bool | editIncidence (Akonadi::Item::Id id) |
QString | getCurrentURLasString () const |
bool | handleCommandLine () |
void | init () |
QString | localFileName () |
bool | mergeURL (const QString &url) |
bool | openURL (const QString &url) |
bool | queryClose () |
bool | saveAsURL (const QString &url) |
bool | showIncidence (Akonadi::Item::Id id) |
bool | showIncidenceContext (Akonadi::Item::Id id) |
KUrl | url () const |
CalendarView * | view () const |
Static Public Member Functions | |
static KOrg::MainWindow * | findInstance (const KUrl &url) |
Protected Slots | |
void | checkAutoExport () |
void | configureDateTime () |
void | downloadNewStuff () |
void | file_archive () |
void | file_icalimport () |
void | file_import () |
void | file_open () |
void | file_open (const KUrl &url) |
void | setItems (const QStringList &, int) |
void | setTitle () |
void | showTip () |
void | showTipOnStart () |
void | slotAutoArchive () |
void | slotAutoArchivingSettingsModified () |
void | toggleDateNavigator () |
void | toggleEventViewer () |
void | toggleResourceView () |
void | toggleTodoView () |
void | updateUndoRedoActions () |
Protected Member Functions | |
QWidget * | dialogParent () |
KUrl | getSaveURL () |
Detailed Description
The ActionManager creates all the actions in KOrganizer.
This class is shared between the main application and the part so all common actions are in one location. It also provides D-Bus interfaces.
Definition at line 66 of file actionmanager.h.
Constructor & Destructor Documentation
ActionManager::ActionManager | ( | KXMLGUIClient * | client, |
CalendarView * | widget, | ||
QObject * | parent, | ||
KOrg::MainWindow * | mainWindow, | ||
bool | isPart, | ||
KMenuBar * | menuBar = 0 |
||
) |
Definition at line 97 of file actionmanager.cpp.
|
virtual |
Definition at line 118 of file actionmanager.cpp.
Member Function Documentation
bool ActionManager::addIncidence | ( | const QString & | ical | ) |
Add an incidence to the active calendar.
- Parameters
-
ical A calendar in iCalendar format containing the incidence.
Definition at line 1403 of file actionmanager.cpp.
|
protectedslot |
called by the autoExportTimer to automatically export the calendar
Definition at line 1919 of file actionmanager.cpp.
|
signal |
When change is made to options dialog, the topwidget will catch this and emit this signal which notifies all widgets which have registered for notification to update their settings.
|
protectedslot |
Open kcontrol module for configuring date and time formats.
Definition at line 1250 of file actionmanager.cpp.
void ActionManager::createCalendarAkonadi | ( | ) |
Create Calendar object based on the akonadi framework and set it on the view.
Definition at line 211 of file actionmanager.cpp.
|
virtual |
Delete the incidence with the given unique id from current calendar.
- Parameters
-
uid UID of the incidence to delete. force If true, all recurrences and sub-todos (if applicable) will be deleted without prompting for confirmation.
Definition at line 1398 of file actionmanager.cpp.
|
protected |
Return widget used as parent for dialogs and message boxes.
Definition at line 1914 of file actionmanager.cpp.
|
protectedslot |
Definition at line 1408 of file actionmanager.cpp.
bool ActionManager::editIncidence | ( | Akonadi::Item::Id | id | ) |
Definition at line 1348 of file actionmanager.cpp.
|
slot |
Export the calendar to an HTML file.
Reads up the user settings as needed. Intended to be used as part of the auto HTML export feature.
Definition at line 1010 of file actionmanager.cpp.
|
slot |
Export the calendar to an HTML file, per the user settings.
- Parameters
-
settings is a pointer to an KOrg::HTMLExportSettings instance. autoMode if true, indicates that this export is for an autosave; if false, then the export is explicitly user invoked.
Definition at line 1032 of file actionmanager.cpp.
|
protectedslot |
delete or archive old entries in your calendar for speed/space.
Definition at line 932 of file actionmanager.cpp.
|
protectedslot |
import a non-ics calendar from another program like ical.
Definition at line 872 of file actionmanager.cpp.
|
protectedslot |
import a generic ics file
Definition at line 922 of file actionmanager.cpp.
|
protectedslot |
open new window
open a file, load it into the calendar.
Definition at line 844 of file actionmanager.cpp.
|
protectedslot |
open a file from the list of recent files.
Also called from file_open() after the URL is obtained from the user.
- Parameters
-
url the URL to open
Definition at line 852 of file actionmanager.cpp.
|
static |
Is there a instance with this URL?
Definition at line 1273 of file actionmanager.cpp.
QString ActionManager::getCurrentURLasString | ( | ) | const |
Get current URL as QString.
Definition at line 1343 of file actionmanager.cpp.
|
protected |
|
slot |
Definition at line 1811 of file actionmanager.cpp.
|
slot |
Definition at line 1816 of file actionmanager.cpp.
bool ActionManager::handleCommandLine | ( | ) |
Called by KOrganizerUniqueAppHandler in the kontact plugin Returns true if the command line was successfully handled false otherwise.
Definition at line 1363 of file actionmanager.cpp.
|
slot |
Definition at line 1854 of file actionmanager.cpp.
|
slot |
Definition at line 937 of file actionmanager.cpp.
void ActionManager::init | ( | ) |
Peform initialization that requires this* to be full constructed.
Definition at line 153 of file actionmanager.cpp.
|
slot |
Definition at line 1583 of file actionmanager.cpp.
|
slot |
Definition at line 1599 of file actionmanager.cpp.
QString ActionManager::localFileName | ( | ) |
Definition at line 1453 of file actionmanager.cpp.
bool ActionManager::mergeURL | ( | const QString & | url | ) |
Open calendar file from URL.
Definition at line 1333 of file actionmanager.cpp.
|
slot |
Definition at line 1609 of file actionmanager.cpp.
|
slot |
Definition at line 1614 of file actionmanager.cpp.
|
slot |
Definition at line 1621 of file actionmanager.cpp.
|
slot |
Definition at line 1629 of file actionmanager.cpp.
|
slot |
Definition at line 1948 of file actionmanager.cpp.
|
slot |
Definition at line 1781 of file actionmanager.cpp.
|
slot |
Definition at line 1786 of file actionmanager.cpp.
|
slot |
Definition at line 1791 of file actionmanager.cpp.
|
slot |
Definition at line 1716 of file actionmanager.cpp.
|
slot |
Definition at line 1721 of file actionmanager.cpp.
|
slot |
Definition at line 1727 of file actionmanager.cpp.
|
slot |
Definition at line 1735 of file actionmanager.cpp.
|
slot |
Definition at line 1932 of file actionmanager.cpp.
bool ActionManager::openURL | ( | const QString & | url | ) |
Open calendar file from URL.
Definition at line 1286 of file actionmanager.cpp.
|
slot |
Definition at line 1529 of file actionmanager.cpp.
bool ActionManager::queryClose | ( | ) |
Definition at line 1849 of file actionmanager.cpp.
|
slot |
Definition at line 1220 of file actionmanager.cpp.
|
slot |
Using the KConfig associated with the kapp variable, read in the settings from the config file.
Definition at line 791 of file actionmanager.cpp.
|
slot |
Save calendar file to URL.
Definition at line 1097 of file actionmanager.cpp.
bool ActionManager::saveAsURL | ( | const QString & | url | ) |
Save calendar file to URL.
Definition at line 1338 of file actionmanager.cpp.
|
slot |
Definition at line 1210 of file actionmanager.cpp.
|
slot |
Save calendar file to URL of current calendar.
Definition at line 960 of file actionmanager.cpp.
|
protectedslot |
Definition at line 728 of file actionmanager.cpp.
|
protectedslot |
Definition at line 1604 of file actionmanager.cpp.
|
slot |
Definition at line 1821 of file actionmanager.cpp.
|
slot |
Definition at line 1806 of file actionmanager.cpp.
bool ActionManager::showIncidence | ( | Akonadi::Item::Id | id | ) |
Definition at line 1353 of file actionmanager.cpp.
bool ActionManager::showIncidenceContext | ( | Akonadi::Item::Id | id | ) |
Show an incidence in context.
This means showing the todo, agenda or journal view (as appropriate) and scrolling it to show the incidence.
- Parameters
-
uid Unique ID of the incidence to show.
Definition at line 1358 of file actionmanager.cpp.
|
slot |
Definition at line 1796 of file actionmanager.cpp.
|
protectedslot |
Show tip of the day.
Definition at line 1261 of file actionmanager.cpp.
|
protectedslot |
Show tip of the day.
Definition at line 1266 of file actionmanager.cpp.
|
slot |
Definition at line 1801 of file actionmanager.cpp.
|
protectedslot |
called by the auto archive timer to automatically delete/archive events
Definition at line 1899 of file actionmanager.cpp.
|
protectedslot |
connected to CalendarView's signal which comes from the ArchiveDialog
Definition at line 1890 of file actionmanager.cpp.
|
protectedslot |
Definition at line 1297 of file actionmanager.cpp.
|
protectedslot |
Definition at line 1313 of file actionmanager.cpp.
|
slot |
Definition at line 134 of file actionmanager.cpp.
|
signal |
Emitted when the "New" action is activated.
|
protectedslot |
Definition at line 1321 of file actionmanager.cpp.
|
protectedslot |
Definition at line 1305 of file actionmanager.cpp.
|
slot |
Options dialog made a changed to the configuration.
we catch this and notify all widgets which need to update their configuration.
Definition at line 1226 of file actionmanager.cpp.
|
protectedslot |
Definition at line 1826 of file actionmanager.cpp.
|
inline |
Get current URL.
Definition at line 111 of file actionmanager.h.
|
inline |
Definition at line 78 of file actionmanager.h.
|
slot |
Write current state to config file.
Definition at line 806 of file actionmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:19 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.