korganizer
#include <kodaymatrix.h>
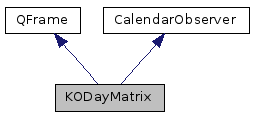
Public Slots | |
void | recalculateToday () |
void | resourcesChanged () |
void | updateView () |
Signals | |
void | incidenceDropped (const Akonadi::Item &item, const QDate &dt) |
void | incidenceDroppedMove (const Akonadi::Item &item, const QDate &dt) |
void | newEventSignal (const QDate &date) |
void | newJournalSignal (const QDate &date) |
void | newTodoSignal (const QDate &date) |
void | selected (const KCalCore::DateList &daylist) |
Public Member Functions | |
KODayMatrix (QWidget *parent) | |
~KODayMatrix () | |
void | addSelectedDaysTo (KCalCore::DateList &) |
void | calendarIncidenceAdded (const KCalCore::Incidence::Ptr &incidence) |
void | calendarIncidenceChanged (const KCalCore::Incidence::Ptr &incidence) |
void | calendarIncidenceDeleted (const KCalCore::Incidence::Ptr &incidence) |
void | clearSelection () |
const QDate & | getDate (int offset) const |
QString | getHolidayLabel (int offset) const |
bool | isBeginningOfMonth () const |
bool | isEndOfMonth () const |
bool | isTodayVisible () const |
void | setCalendar (const Akonadi::ETMCalendar::Ptr &) |
void | setHighlightMode (bool highlightEvents, bool highlightTodos, bool highlightJournals) |
void | setSelectedDaysFrom (const QDate &start, const QDate &end) |
void | setUpdateNeeded () |
void | updateIncidences () |
void | updateView (const QDate &actdate) |
Static Public Member Functions | |
static QPair< QDate, QDate > | matrixLimits (const QDate &month) |
Protected Member Functions | |
void | dragEnterEvent (QDragEnterEvent *e) |
void | dragLeaveEvent (QDragLeaveEvent *e) |
void | dragMoveEvent (QDragMoveEvent *e) |
void | dropEvent (QDropEvent *e) |
bool | event (QEvent *e) |
void | mouseMoveEvent (QMouseEvent *e) |
void | mousePressEvent (QMouseEvent *e) |
void | mouseReleaseEvent (QMouseEvent *e) |
void | paintEvent (QPaintEvent *ev) |
void | resizeEvent (QResizeEvent *) |
Detailed Description
Replacement for kdpdatebuton.cpp that used 42 widgets for the day matrix to be displayed.
Cornelius thought this was a waste of memory and a lot of overhead. In addition the selection was not very intuitive so I decided to rewrite it using a QFrame that draws the labels and allows for dragging selection while maintaining nearly full compatibility in behavior with its predecessor.
The following functionality has been changed:
o when shifting events in the agenda view from one day to another the day matrix is updated now
o dragging an event to the matrix will MOVE not COPY the event to the new date.
o no support for Ctrl+click to create groups of dates (This has not really been supported in the predecessor. It was not very intuitive nor was it user friendly.) This feature has been replaced with dragging a selection on the matrix. The matrix will automatically choose the appropriate selection (e.g. you are not any longer able to select two distinct groups of date selections as in the old class)
o now that you can select more than a week it can happen that not all selected days are displayed in the matrix. However this is preferred to the alternative which would mean to adjust the selection and leave some days undisplayed while scrolling through the months
day matrix widget of the KDateNavigator
Definition at line 69 of file kodaymatrix.h.
Constructor & Destructor Documentation
|
explicit |
constructor to create a day matrix widget.
- Parameters
-
parent widget that is the parent of the day matrix. Normally this should be a KDateNavigator
Definition at line 53 of file kodaymatrix.cpp.
KODayMatrix::~KODayMatrix | ( | ) |
destructor that deallocates all dynamically allocated private members.
Definition at line 98 of file kodaymatrix.cpp.
Member Function Documentation
void KODayMatrix::addSelectedDaysTo | ( | KCalCore::DateList & | selDays | ) |
Adds all actual selected days from mSelStart to mSelEnd to the supplied DateList.
Definition at line 108 of file kodaymatrix.cpp.
void KODayMatrix::calendarIncidenceAdded | ( | const KCalCore::Incidence::Ptr & | incidence | ) |
Reimplemented from Akonadi::ETMCalendar They set mPendingChanges to true.
Definition at line 435 of file kodaymatrix.cpp.
void KODayMatrix::calendarIncidenceChanged | ( | const KCalCore::Incidence::Ptr & | incidence | ) |
Definition at line 441 of file kodaymatrix.cpp.
void KODayMatrix::calendarIncidenceDeleted | ( | const KCalCore::Incidence::Ptr & | incidence | ) |
Definition at line 447 of file kodaymatrix.cpp.
void KODayMatrix::clearSelection | ( | ) |
Clear all selections.
Definition at line 147 of file kodaymatrix.cpp.
|
protected |
Definition at line 607 of file kodaymatrix.cpp.
|
protected |
Definition at line 632 of file kodaymatrix.cpp.
|
protected |
Definition at line 622 of file kodaymatrix.cpp.
|
protected |
Definition at line 639 of file kodaymatrix.cpp.
|
protected |
Definition at line 477 of file kodaymatrix.cpp.
const QDate & KODayMatrix::getDate | ( | int | offset | ) | const |
Returns the QDate object associated with day indexed by the supplied offset.
Definition at line 412 of file kodaymatrix.cpp.
QString KODayMatrix::getHolidayLabel | ( | int | offset | ) | const |
Returns the official name of this holy day or 0 if there is no label for this day.
Definition at line 420 of file kodaymatrix.cpp.
|
signal |
Emitted if the user has dropped an incidence (event or todo) inside the matrix.
- Parameters
-
incidence the dropped calendar incidence dt QDate that has been selected
|
signal |
Emitted if the user has dropped an event inside the matrix and chose to move it instead of copy.
- Parameters
-
oldincidence the new calendar incidence dt QDate that has been selected
|
inline |
If today is visible, then we can find out if today is near the beginning or the end of the month.
This is dependent on today remaining the index in the array of visible dates and going from top left (0) to bottom right (41).
Definition at line 155 of file kodaymatrix.h.
|
inline |
Definition at line 156 of file kodaymatrix.h.
|
inline |
Is today visible in the view? Keep this in sync with the values today (below) can take.
Definition at line 146 of file kodaymatrix.h.
|
static |
returns the first and last date of the 6*7 matrix that displays month
- Parameters
-
month The month we want to get matrix boundaries
Definition at line 886 of file kodaymatrix.cpp.
|
protected |
Definition at line 570 of file kodaymatrix.cpp.
|
protected |
Definition at line 500 of file kodaymatrix.cpp.
|
protected |
Definition at line 533 of file kodaymatrix.cpp.
|
signal |
|
signal |
|
signal |
|
protected |
Definition at line 712 of file kodaymatrix.cpp.
|
slot |
Calculates which square in the matrix should be hiighted to indicate the square is on "today".
Definition at line 152 of file kodaymatrix.cpp.
|
protected |
Definition at line 878 of file kodaymatrix.cpp.
|
slot |
Handle resource changes.
Definition at line 468 of file kodaymatrix.cpp.
|
signal |
Emitted if the user selects a block of days with the mouse by dragging a rectangle inside the matrix.
- Parameters
-
daylist list of days that have been selected by the user
void KODayMatrix::setCalendar | ( | const Akonadi::ETMCalendar::Ptr & | calendar | ) |
Associate a calendar with this day matrix.
If there is a calendar, the day matrix will accept drops and days with events will be highlighted.
Definition at line 70 of file kodaymatrix.cpp.
void KODayMatrix::setHighlightMode | ( | bool | highlightEvents, |
bool | highlightTodos, | ||
bool | highlightJournals | ||
) |
Sets which incidences should be highlighted.
Definition at line 453 of file kodaymatrix.cpp.
void KODayMatrix::setSelectedDaysFrom | ( | const QDate & | start, |
const QDate & | end | ||
) |
Sets the actual to be displayed selection in the day matrix starting from start and ending with end.
Theview must be manually updated by calling repaint. (?)
- Parameters
-
start start of the new selection end end date of the new selection
Definition at line 139 of file kodaymatrix.cpp.
void KODayMatrix::setUpdateNeeded | ( | ) |
Definition at line 177 of file kodaymatrix.cpp.
void KODayMatrix::updateIncidences | ( | ) |
Update incidence states of dates.
Depending of the preferences days with incidences are highlighted in some way.
Definition at line 249 of file kodaymatrix.cpp.
void KODayMatrix::updateView | ( | const QDate & | actdate | ) |
updates the day matrix to start with the given date.
Does all the necessary checks for holidays or events on a day and stores them for display later on. Does NOT update the view visually. Call repaint() for this.
- Parameters
-
actdate recalculates the day matrix to show NUMDAYS starting from this date.
Definition at line 182 of file kodaymatrix.cpp.
|
slot |
Recalculates all the flags of the days in the matrix like holidays or events on a day (Actually calls above method with the actual startdate).
Definition at line 172 of file kodaymatrix.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:56:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.