ktimetracker
#include <timetrackerstorage.h>
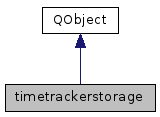
Public Member Functions | |
timetrackerstorage () | |
~timetrackerstorage () | |
void | addComment (const Task *task, const QString &comment) |
QString | addTask (const Task *task, const Task *parent=0) |
bool | allEventsHaveEndTiMe (Task *task) |
bool | allEventsHaveEndTiMe () |
QString | buildTaskView (const KTimeTracker::KTTCalendar::Ptr &calendar, TaskView *view) |
QString | buildTaskView (TaskView *view) |
KTimeTracker::KTTCalendar::Ptr | calendar () const |
void | changeTime (const Task *task, const long deltaSeconds) |
void | closeStorage () |
QString | deleteAllEvents () |
QString | icalfile () |
bool | isEmpty () |
QString | load (TaskView *taskview, const QString &fileName) |
KCalCore::Event::List | rawevents () |
KCalCore::Todo::List | rawtodos () |
QString | removeEvent (QString uid) |
bool | removeTask (Task *task) |
bool | removeTask (QString taskid) |
QString | report (TaskView *taskview, const ReportCriteria &rc) |
QString | save (TaskView *taskview) |
void | setName (const Task *task, const QString &oldname) |
QString | setTaskParent (Task *task, Task *parent) |
void | startTimer (const Task *task, const KDateTime &when=KDateTime::currentLocalDateTime()) |
void | startTimer (QString taskID) |
void | stopTimer (const Task *task, const QDateTime &when=QDateTime::currentDateTime()) |
Task * | task (const QString &uid, TaskView *view) |
QStringList | taskidsfromname (QString taskname) |
QStringList | taskNames () const |
Detailed Description
Class to store/retrieve KTimeTracker data to/from persistent storage.
The storage is an iCalendar file. Its name is contained in this class in the variable _icalfile and can be read using the function icalfile(). The name gets set by the load() operation.
All logic that deals with getting and saving data should go here.
This program uses iCalendar to store its data. There are tasks and events. Events have a start and a end date and an associated task.
Logic that gets and stores KTimeTracker data to disk.
Definition at line 57 of file timetrackerstorage.h.
Constructor & Destructor Documentation
timetrackerstorage::timetrackerstorage | ( | ) |
Definition at line 90 of file timetrackerstorage.cpp.
timetrackerstorage::~timetrackerstorage | ( | ) |
Definition at line 94 of file timetrackerstorage.cpp.
Member Function Documentation
void timetrackerstorage::addComment | ( | const Task * | task, |
const QString & | comment | ||
) |
Log a new comment for this task.
iCal allows multiple comment tags. So we just add a new comment to the todo for this task and write the calendar.
- Parameters
-
task The task that gets the comment comment The comment
Definition at line 558 of file timetrackerstorage.cpp.
Add this task from iCalendar file.
Create a new KCalCore::Todo object and load with task information. If parent is not zero, then set the RELATED-TO attribute for this Todo.
- Parameters
-
task The task to be removed. parent The parent of this task. Must have a uid() that is in the existing calendar. If zero, this task is considered a root task.
- Returns
- The unique ID for the new VTODO. Return an null QString if there was an error creating the new calendar object.
Definition at line 442 of file timetrackerstorage.cpp.
bool timetrackerstorage::allEventsHaveEndTiMe | ( | Task * | task | ) |
Deliver if all events of a task have an endtime.
If ktimetracker has been quitted with one task running, it needs to resumeRunning(). This function delivers if an enddate of an event has not yet been stored.
- Parameters
-
task The task to be examined
Definition at line 332 of file timetrackerstorage.cpp.
bool timetrackerstorage::allEventsHaveEndTiMe | ( | ) |
Deliver if all events of the actual calendar have an endtime.
If ktimetracker has been quitted with one task running, it needs to resumeRunning(). This function delivers if an enddate of an event has not yet been stored.
Definition at line 346 of file timetrackerstorage.cpp.
QString timetrackerstorage::buildTaskView | ( | const KTimeTracker::KTTCalendar::Ptr & | calendar, |
TaskView * | view | ||
) |
Build up the taskview.
This is needed if the iCal file has been modified.
Definition at line 232 of file timetrackerstorage.cpp.
QString timetrackerstorage::buildTaskView | ( | TaskView * | view | ) |
Build up the taskview.
This is needed if a subtask has been deleted.
Definition at line 303 of file timetrackerstorage.cpp.
KTTCalendar::Ptr timetrackerstorage::calendar | ( | ) | const |
Definition at line 1073 of file timetrackerstorage.cpp.
void timetrackerstorage::changeTime | ( | const Task * | task, |
const long | deltaSeconds | ||
) |
Log the change in a task's time.
This is also called when a timer is stopped. We create an iCalendar event to store each change. The event start date is set to the current datetime. If time is added to the task, the task end date is set to start time + delta. If the time is negative, the end date is set to the start time.
In both cases (postive or negative delta), we create a custom iCalendar property that stores the delta (in seconds). This property is called X-KDE-ktimetracker-duration.
Note that the ktimetracker UI allows the user to change both the session and the total task time, and this routine does not account for all posibile cases. For example, it is possible for the user to do something crazy like add 10 minutes to the session time and subtract 50 minutes from the total time. Although this change violates a basic law of physics, it is allowed.
For now, you should pass in the change to the total task time.
- Parameters
-
task The task the change is for. delta Change in task time, in seconds. Can be negative.
Definition at line 955 of file timetrackerstorage.cpp.
void timetrackerstorage::closeStorage | ( | ) |
Close calendar and clear view.
Release lock if holding one.
Definition at line 309 of file timetrackerstorage.cpp.
QString timetrackerstorage::deleteAllEvents | ( | ) |
Definition at line 358 of file timetrackerstorage.cpp.
QString timetrackerstorage::icalfile | ( | ) |
Return the name of the iCal file.
Definition at line 226 of file timetrackerstorage.cpp.
bool timetrackerstorage::isEmpty | ( | ) |
Check if the iCalendar file currently loaded has any Todos in it.
- Returns
- true if iCalendar file has any todos
Definition at line 432 of file timetrackerstorage.cpp.
QString timetrackerstorage::load | ( | TaskView * | taskview, |
const QString & | fileName | ||
) |
Load the list view with tasks read from iCalendar file.
Parses iCalendar file, builds list view items in the proper hierarchy, and loads them into the list view widget.
If the file name passed in is the same as the last file name that was loaded, this method does nothing.
This method considers any of the following conditions errors:
- the iCalendar file does not exist
- the iCalendar file is not readable
- the list group currently has list items
- an iCalendar todo has no related to attribute
- a todo is related to another todo which does not exist
- Parameters
-
taskview The list group used in the TaskView fileName Override preferences' filename
- Returns
- empty string if success, error message if error.
Definition at line 99 of file timetrackerstorage.cpp.
KCalCore::Event::List timetrackerstorage::rawevents | ( | ) |
list of all events
Definition at line 320 of file timetrackerstorage.cpp.
KCalCore::Todo::List timetrackerstorage::rawtodos | ( | ) |
list of all todos
Definition at line 326 of file timetrackerstorage.cpp.
QString timetrackerstorage::removeEvent | ( | QString | uid | ) |
Definition at line 494 of file timetrackerstorage.cpp.
bool timetrackerstorage::removeTask | ( | Task * | task | ) |
Remove this task from iCalendar file.
Removes task as well as all event history for this task.
- Parameters
-
task The task to be removed.
- Returns
- true if change was saved, false otherwise
Definition at line 510 of file timetrackerstorage.cpp.
bool timetrackerstorage::removeTask | ( | QString | taskid | ) |
Remove this task from iCalendar file.
Removes task as well as all event history for this task.
- Parameters
-
task The task to be removed.
- Returns
- true if change was saved, false otherwise
Definition at line 533 of file timetrackerstorage.cpp.
QString timetrackerstorage::report | ( | TaskView * | taskview, |
const ReportCriteria & | rc | ||
) |
Output a report based on contents of ReportCriteria.
Definition at line 575 of file timetrackerstorage.cpp.
QString timetrackerstorage::save | ( | TaskView * | taskview | ) |
Save all tasks and their totals to an iCalendar file.
All tasks must have an associated VTODO object already created in the calendar file; that is, the task->uid() must refer to a valid VTODO in the calendar. Delivers empty string if successful, else error msg.
- Parameters
-
taskview The list group used in the TaskView
Definition at line 367 of file timetrackerstorage.cpp.
|
inline |
Log a change to a task name.
For iCalendar storage, there is no need to log an Event for this event, since unique id's are used to link Events to Todos. No matter how many times you change a task's name, the uid stays the same.
- Parameters
-
task The task oldname The old name of the task. The new name is in the task object already.
Definition at line 199 of file timetrackerstorage.h.
Definition at line 394 of file timetrackerstorage.cpp.
void timetrackerstorage::startTimer | ( | const Task * | task, |
const KDateTime & | when = KDateTime::currentLocalDateTime() |
||
) |
Log the event that a timer has started for a task.
For the iCalendar storage, there is no need to log anything for this event. We log an event when the timer is stopped.
- Parameters
-
task The task the timer was started for.
Definition at line 897 of file timetrackerstorage.cpp.
void timetrackerstorage::startTimer | ( | QString | taskID | ) |
Start the timer for a given task ID.
- Parameters
-
taskID The task ID of the task to be started
Definition at line 907 of file timetrackerstorage.cpp.
void timetrackerstorage::stopTimer | ( | const Task * | task, |
const QDateTime & | when = QDateTime::currentDateTime() |
||
) |
Log the event that the timer has stopped for this task.
The task stores the last time a timer was started, so we log a new iCal Event with the start and end times for this task.
- See also
- timetrackerstorage::changeTime
- Parameters
-
task The task the timer was stopped for. when When the timer stopped.
Definition at line 929 of file timetrackerstorage.cpp.
Find the task with the given uid.
- Parameters
-
uid The uid that identifies the task view The TaskView that contains the task
- Returns
- the task identified by uid, residing in the TaskView view
Definition at line 208 of file timetrackerstorage.cpp.
QStringList timetrackerstorage::taskidsfromname | ( | QString | taskname | ) |
Return a list of all task ids for taskname.
Definition at line 470 of file timetrackerstorage.cpp.
QStringList timetrackerstorage::taskNames | ( | ) | const |
Return a list of all task names.
Definition at line 484 of file timetrackerstorage.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.