mailcommon
#include <filtermanager.h>
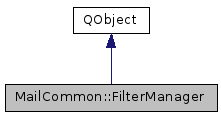
Public Types | |
enum | FilterSet { NoSet = 0x0, Inbound = 0x1, Outbound = 0x2, Explicit = 0x4, BeforeOutbound = 0x8, All = Inbound|BeforeOutbound|Outbound|Explicit } |
Signals | |
void | filtersChanged () |
void | loadingFiltersDone () |
void | tagListingFinished () |
Public Member Functions | |
void | appendFilters (const QList< MailCommon::MailFilter * > &filters, bool replaceIfNameExists=false) |
void | beginUpdate () |
QString | createUniqueFilterName (const QString &name) const |
void | endUpdate () |
void | filter (const Akonadi::Item &item, const QString &identifier, const QString &resourceId) const |
void | filter (const Akonadi::Item &item, FilterSet set=Inbound, bool account=false, const QString &resourceId=QString()) const |
void | filter (const Akonadi::Item::List &messages, FilterSet set=Explicit) const |
void | filter (const Akonadi::Item::List &messages, SearchRule::RequiredPart requiredPart, const QStringList &listFilters) const |
QList< MailCommon::MailFilter * > | filters () const |
bool | initialized () const |
bool | isValid () const |
void | removeFilter (MailCommon::MailFilter *filter) |
void | setFilters (const QList< MailCommon::MailFilter * > &filters) |
void | showFilterLogDialog (qlonglong windowId) |
QMap< QUrl, QString > | tagList () const |
Static Public Member Functions | |
static FilterActionDict * | filterActionDict () |
static FilterManager * | instance () |
Detailed Description
A wrapper class that allows easy access to the mail filters.
This class communicates with the mailfilter agent via DBus.
Definition at line 47 of file filtermanager.h.
Member Enumeration Documentation
Describes the list of filters.
Enumerator | |
---|---|
NoSet | |
Inbound | |
Outbound | |
Explicit | |
BeforeOutbound | |
All |
Definition at line 55 of file filtermanager.h.
Member Function Documentation
void FilterManager::appendFilters | ( | const QList< MailCommon::MailFilter * > & | filters, |
bool | replaceIfNameExists = false |
||
) |
Manage filters interface.
Appends the list of filters
to the current list of filters and write everything back into the configuration. The filter manager takes ownership of the filters in the list.
Definition at line 282 of file filtermanager.cpp.
void FilterManager::beginUpdate | ( | ) |
Should be called at the beginning of an filter list update.
Definition at line 310 of file filtermanager.cpp.
QString FilterManager::createUniqueFilterName | ( | const QString & | name | ) | const |
Checks for existing filters with the name
and extend the "name" to "name (i)" until no match is found for i=1..n.
Definition at line 229 of file filtermanager.cpp.
void FilterManager::endUpdate | ( | ) |
Should be called at the end of an filter list update.
Definition at line 314 of file filtermanager.cpp.
void FilterManager::filter | ( | const Akonadi::Item & | item, |
const QString & | identifier, | ||
const QString & | resourceId | ||
) | const |
Apply filters interface.
Applies filter with the given identifier
on the message item
.
- Returns
true
on success,false
otherwise.
Definition at line 239 of file filtermanager.cpp.
void FilterManager::filter | ( | const Akonadi::Item & | item, |
FilterSet | set = Inbound , |
||
bool | account = false , |
||
const QString & | resourceId = QString() |
||
) | const |
Process given message item by applying the filter rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
- Parameters
-
item The message item to process. set Select the filter set to use. account true
if an account id is specified elsefalse
accountId The id of the resource that the message was retrieved from
Definition at line 244 of file filtermanager.cpp.
void FilterManager::filter | ( | const Akonadi::Item::List & | messages, |
FilterManager::FilterSet | set = Explicit |
||
) | const |
Process given messages
by applying the filter rules one by one.
You can select which set of filters (incoming or outgoing) should be used.
- Parameters
-
item The message item to process. set Select the filter set to use.
Definition at line 249 of file filtermanager.cpp.
void FilterManager::filter | ( | const Akonadi::Item::List & | messages, |
SearchRule::RequiredPart | requiredPart, | ||
const QStringList & | listFilters | ||
) | const |
Definition at line 260 of file filtermanager.cpp.
|
static |
Returns the global filter action dictionary.
Definition at line 109 of file filtermanager.cpp.
QList< MailCommon::MailFilter * > FilterManager::filters | ( | ) | const |
Returns the filter list of the manager.
Definition at line 277 of file filtermanager.cpp.
|
signal |
This signal is emitted whenever the filter list has been updated.
bool FilterManager::initialized | ( | ) | const |
Definition at line 169 of file filtermanager.cpp.
|
static |
Returns the global filter manager object.
Definition at line 101 of file filtermanager.cpp.
bool FilterManager::isValid | ( | ) | const |
Returns whether the filter manager is in a usable state.
Definition at line 224 of file filtermanager.cpp.
|
signal |
void FilterManager::removeFilter | ( | MailCommon::MailFilter * | filter | ) |
Removes the given filter
from the list.
The filter object is not deleted.
Definition at line 303 of file filtermanager.cpp.
void FilterManager::setFilters | ( | const QList< MailCommon::MailFilter * > & | filters | ) |
Replace the list of filters of the filter manager with the given list of filters
.
The manager takes ownership of the filters.
Definition at line 269 of file filtermanager.cpp.
void FilterManager::showFilterLogDialog | ( | qlonglong | windowId | ) |
Shows the filter log dialog.
This is used to debug problems with filters.
Definition at line 234 of file filtermanager.cpp.
QMap< QUrl, QString > FilterManager::tagList | ( | ) | const |
Definition at line 219 of file filtermanager.cpp.
|
signal |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:15 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.