mailcommon
#include <searchpattern.h>
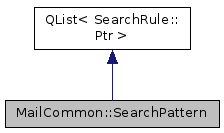
Public Types | |
enum | Operator { OpAnd, OpOr, OpAll } |
enum | SparqlQueryError { NoError = 0, MissingCheck, FolderEmptyOrNotIndexed, EmptyResult, NotEnoughCharacters } |
Public Member Functions | |
SearchPattern () | |
SearchPattern (const KConfigGroup &config) | |
~SearchPattern () | |
SparqlQueryError | asSparqlQuery (QString &queryStr, const KUrl::List &url=KUrl::List()) const |
QString | asString () const |
void | deserialize (const QByteArray &) |
void | generateSieveScript (QStringList &requires, QString &code) |
bool | matches (const Akonadi::Item &item, bool ignoreBody=false) const |
QString | name () const |
SearchPattern::Operator | op () const |
QDataStream & | operator<< (QDataStream &s) |
const SearchPattern & | operator= (const SearchPattern &aPattern) |
QDataStream & | operator>> (QDataStream &s) const |
void | purify () |
void | readConfig (const KConfigGroup &config) |
SearchRule::RequiredPart | requiredPart () const |
QByteArray | serialize () const |
void | setName (const QString &newName) |
void | setOp (SearchPattern::Operator aOp) |
void | writeConfig (KConfigGroup &config) const |
Detailed Description
This class is an abstraction of a search over messages.
It is intended to be used inside a KFilter (which adds KFilterAction's), as well as in KMSearch. It can read and write itself into a KConfig group and there is a constructor, mainly used by KMFilter to initialize from a preset KConfig-Group.
From a class hierarchy point of view, it is a QPtrList of SearchRule's that adds the boolean operators (see Operator) 'and' and 'or' that connect the rules logically, and has a name under which it could be stored in the config file.
As a QPtrList with autoDelete enabled, it assumes that it is the central repository for the rules it contains. So if you want to reuse a rule in another pattern, make a deep copy of that rule.
An abstraction of a search over messages.
Definition at line 595 of file searchpattern.h.
Member Enumeration Documentation
Boolean operators that connect the return values of the individual rules.
A pattern with OpAnd
will match iff all it's rules match, whereas a pattern with OpOr
will match if any of it's rules matches.
Enumerator | |
---|---|
OpAnd | |
OpOr | |
OpAll |
Definition at line 605 of file searchpattern.h.
Enumerator | |
---|---|
NoError | |
MissingCheck | |
FolderEmptyOrNotIndexed | |
EmptyResult | |
NotEnoughCharacters |
Definition at line 612 of file searchpattern.h.
Constructor & Destructor Documentation
SearchPattern::SearchPattern | ( | ) |
Constructor which provides a pattern with minimal, but sufficient initialization.
Unmodified, such a pattern will fail to match any KMime::Message. You can query for such an empty rule by using isEmpty, which is inherited from QPtrList.
Definition at line 1510 of file searchpattern.cpp.
|
explicit |
Constructor that initializes from a given KConfig group, if given.
This feature is mainly (solely?) used in KMFilter, as we don't allow to store search patterns in the config (yet).
Definition at line 1516 of file searchpattern.cpp.
SearchPattern::~SearchPattern | ( | ) |
Member Function Documentation
MailCommon::SearchPattern::SparqlQueryError SearchPattern::asSparqlQuery | ( | QString & | queryStr, |
const KUrl::List & | url = KUrl::List() |
||
) | const |
Returns the pattern as a SPARQL query.
Definition at line 1755 of file searchpattern.cpp.
QString SearchPattern::asString | ( | ) | const |
void SearchPattern::deserialize | ( | const QByteArray & | str | ) |
Constructs the pattern from a byte array serialization.
Definition at line 1849 of file searchpattern.cpp.
void SearchPattern::generateSieveScript | ( | QStringList & | requires, |
QString & | code | ||
) |
Definition at line 1894 of file searchpattern.cpp.
bool SearchPattern::matches | ( | const Akonadi::Item & | item, |
bool | ignoreBody = false |
||
) | const |
The central function of this class.
Tries to match the set of rules against a KMime::Message. It's virtual to allow derived classes with added rules to reimplement it, yet reimplemented methods should and (&&) the result of this function with their own result or else most functionality is lacking, or has to be reimplemented, since the rules are private to this class.
- Returns
- true if the match was successful, false otherwise.
Definition at line 1526 of file searchpattern.cpp.
|
inline |
Returns the name of the search pattern.
Definition at line 688 of file searchpattern.h.
|
inline |
Returns the filter operator.
Definition at line 705 of file searchpattern.h.
QDataStream & SearchPattern::operator<< | ( | QDataStream & | s | ) |
Definition at line 1875 of file searchpattern.cpp.
const SearchPattern & SearchPattern::operator= | ( | const SearchPattern & | aPattern | ) |
Overloaded assignment operator.
Makes a deep copy.
Definition at line 1822 of file searchpattern.cpp.
QDataStream & SearchPattern::operator>> | ( | QDataStream & | s | ) | const |
Definition at line 1855 of file searchpattern.cpp.
void SearchPattern::purify | ( | ) |
Removes all empty rules from the list.
You should call this method whenever the user had had control of the rules outside of this class. (e.g. after editing it with SearchPatternEdit).
Definition at line 1579 of file searchpattern.cpp.
void SearchPattern::readConfig | ( | const KConfigGroup & | config | ) |
Reads a search pattern from a KConfigGroup.
If it does not find a valid saerch pattern in the preset group, initializes the pattern as if it were constructed using the default constructor.
For backwards compatibility with previous versions of KMail, it checks for old-style filter rules (e.g. using OpIgnore
) in config
und converts them to the new format on writeConfig.
Derived classes reimplementing readConfig() should also call this method, or else the rules will not be loaded.
Definition at line 1594 of file searchpattern.cpp.
SearchRule::RequiredPart SearchPattern::requiredPart | ( | ) | const |
Returns the required part from the item that is needed for the search to operate.
See RequiredPart
Definition at line 1566 of file searchpattern.cpp.
QByteArray SearchPattern::serialize | ( | ) | const |
Writes the pattern into a byte array for persistance purposes.
Definition at line 1841 of file searchpattern.cpp.
|
inline |
Sets the name of the search pattern.
KMFilter uses this to store it's own name, too.
Definition at line 697 of file searchpattern.h.
|
inline |
Sets the filter operator.
Definition at line 713 of file searchpattern.h.
void SearchPattern::writeConfig | ( | KConfigGroup & | config | ) | const |
Writes itself into config
.
Tries to delete old-style keys by overwriting them with QString().
Derived classes reimplementing writeConfig() should also call this method, or else the rules will not be stored.
Definition at line 1674 of file searchpattern.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:15 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.