messagelist
#include <widget.h>
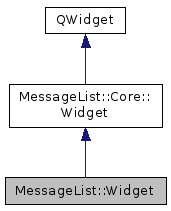
Signals | |
void | messageActivated (const Akonadi::Item &item) |
void | messageSelected (const Akonadi::Item &item) |
void | messageStatusChangeRequest (const Akonadi::Item &item, const Akonadi::MessageStatus &set, const Akonadi::MessageStatus &clear) |
void | selectionChanged () |
![]() | |
void | fullSearchRequest () |
void | statusMessage (const QString &message) |
Public Member Functions | |
Widget (QWidget *parent) | |
~Widget () | |
bool | canAcceptDrag (const QDropEvent *e) |
Akonadi::Collection | currentCollection () const |
QString | currentFilterSearchString () const |
Akonadi::MessageStatus | currentFilterStatus () const |
Akonadi::Item | currentItem () const |
KMime::Message::Ptr | currentMessage () const |
QList< Akonadi::Item > | currentThreadAsMessageList () const |
MessageList::Core::MessageItemSetReference | currentThreadAsPersistentSet () const |
void | deletePersistentSet (MessageList::Core::MessageItemSetReference ref) |
bool | focusNextMessageItem (MessageList::Core::MessageTypeFilter messageTypeFilter, bool centerItem, bool loop) |
bool | focusPreviousMessageItem (MessageList::Core::MessageTypeFilter messageTypeFilter, bool centerItem, bool loop) |
void | focusQuickSearch () |
bool | getSelectionStats (Akonadi::Item::List &selectedSernums, Akonadi::Item::List &selectedVisibleSernums, bool *allSelectedBelongToSameThread, bool includeCollapsedChildren=true) const |
bool | isThreaded () const |
QList< Akonadi::Item > | itemListFromPersistentSet (MessageList::Core::MessageItemSetReference ref) |
void | markMessageItemsAsAboutToBeRemoved (MessageList::Core::MessageItemSetReference ref, bool bMark) |
void | selectAll () |
bool | selectFirstMessageItem (MessageList::Core::MessageTypeFilter messageTypeFilter, bool centerItem) |
void | selectFocusedMessageItem (bool centerItem) |
QList< Akonadi::Item::Id > | selectionAsListMessageId (bool includeCollapsedChildren) const |
QList< Akonadi::Item > | selectionAsMessageItemList (bool includeCollapsedChildren=true) const |
QVector< qlonglong > | selectionAsMessageItemListId (bool includeCollapsedChildren) const |
QList< KMime::Message::Ptr > | selectionAsMessageList (bool includeCollapsedChildren=true) const |
MessageList::Core::MessageItemSetReference | selectionAsPersistentSet (bool includeCollapsedChildren=true) const |
bool | selectionEmpty () const |
bool | selectLastMessageItem (MessageList::Core::MessageTypeFilter messageTypeFilter, bool centerItem) |
bool | selectNextMessageItem (MessageList::Core::MessageTypeFilter messageTypeFilter, MessageList::Core::ExistingSelectionBehaviour existingSelectionBehaviour, bool centerItem, bool loop) |
bool | selectPreviousMessageItem (MessageList::Core::MessageTypeFilter messageTypeFilter, MessageList::Core::ExistingSelectionBehaviour existingSelectionBehaviour, bool centerItem, bool loop) |
void | setAllGroupsExpanded (bool expand) |
void | setAllThreadsExpanded (bool expand) |
void | setCurrentThreadExpanded (bool expand) |
void | setXmlGuiClient (KXMLGUIClient *xmlGuiClient) |
![]() | |
Widget (QWidget *parent) | |
~Widget () | |
void | aggregationMenuAboutToShow (KMenu *menu) |
QString | currentFilterSearchString () const |
Akonadi::MessageStatus | currentFilterStatus () const |
QString | currentFilterTagId () const |
Core::MessageItem * | currentMessageItem () const |
void | focusQuickSearch () |
bool | isThreaded () const |
KLineEdit * | quickSearch () const |
void | saveCurrentSelection () |
bool | searchEditHasFocus () const |
bool | selectionEmpty () const |
void | setCurrentFolder (const Akonadi::Collection &collection) |
void | setStorageModel (StorageModel *storageModel, PreSelectionMode preSelectionMode=PreSelectLastSelected) |
void | sortOrderMenuAboutToShow (KMenu *menu) |
StorageModel * | storageModel () const |
void | themeMenuAboutToShow (KMenu *menu) |
View * | view () const |
Protected Member Functions | |
virtual void | fillMessageTagCombo (KComboBox *combo) |
virtual void | viewDragEnterEvent (QDragEnterEvent *e) |
virtual void | viewDragMoveEvent (QDragMoveEvent *e) |
virtual void | viewDropEvent (QDropEvent *e) |
virtual void | viewGroupHeaderContextPopupRequest (MessageList::Core::GroupHeaderItem *group, const QPoint &globalPos) |
virtual void | viewMessageActivated (MessageList::Core::MessageItem *msg) |
virtual void | viewMessageListContextPopupRequest (const QList< MessageList::Core::MessageItem * > &selectedItems, const QPoint &globalPos) |
virtual void | viewMessageSelected (MessageList::Core::MessageItem *msg) |
virtual void | viewMessageStatusChangeRequest (MessageList::Core::MessageItem *msg, const Akonadi::MessageStatus &set, const Akonadi::MessageStatus &clear) |
virtual void | viewSelectionChanged () |
virtual void | viewStartDragRequest () |
![]() | |
void | tagIdSelected (const QVariant &data) |
virtual void | viewJobBatchStarted () |
virtual void | viewJobBatchTerminated () |
Additional Inherited Members | |
![]() | |
void | changeQuicksearchVisibility (bool) |
void | populateStatusFilterCombo () |
![]() | |
void | aggregationMenuAboutToShow () |
void | aggregationsChanged () |
void | aggregationSelected (bool) |
void | configureThemes () |
void | groupSortDirectionSelected (QAction *action) |
void | groupSortingSelected (QAction *action) |
void | messageSortDirectionSelected (QAction *action) |
void | messageSortingSelected (QAction *action) |
void | resetFilter () |
void | searchEditClearButtonClicked () |
void | searchEditTextEdited (const QString &text) |
void | searchTimerFired () |
void | setPrivateSortOrderForStorage () |
void | slotLockSearchClicked (bool b) |
void | slotViewHeaderSectionClicked (int logicalIndex) |
void | sortOrderMenuAboutToShow () |
void | statusSelected (int index) |
void | themeMenuAboutToShow () |
void | themesChanged () |
void | themeSelected (bool) |
Detailed Description
The Akonadi specific implementation of the Core::Widget.
When a KXmlGuiWindow is passed to setXmlGuiClient, the XMLGUI defined context menu akonadi_messagelist_contextmenu
is used if available.
Constructor & Destructor Documentation
|
explicit |
Create a new message list widget.
Definition at line 85 of file widget.cpp.
Widget::~Widget | ( | ) |
Definition at line 101 of file widget.cpp.
Member Function Documentation
bool Widget::canAcceptDrag | ( | const QDropEvent * | e | ) |
Returns true if this drag can be accepted by the underlying view.
Definition at line 111 of file widget.cpp.
Akonadi::Collection Widget::currentCollection | ( | ) | const |
Definition at line 709 of file widget.cpp.
QString Widget::currentFilterSearchString | ( | ) | const |
Returns the search term in the current quicksearch field.
Definition at line 617 of file widget.cpp.
Akonadi::MessageStatus Widget::currentFilterStatus | ( | ) | const |
Returns the Akonadi::MessageStatus in the current quicksearch field.
Definition at line 612 of file widget.cpp.
Item Widget::currentItem | ( | ) | const |
Returns the current message for the list as Akonadi::Item.
May return an invalid Item if there is no current message or no current folder.
Definition at line 522 of file widget.cpp.
KMime::Message::Ptr Widget::currentMessage | ( | ) | const |
Returns the current message for the list as KMime::Message::Ptr.
May return 0 if there is no current message or no current folder.
Definition at line 533 of file widget.cpp.
QList< Akonadi::Item > Widget::currentThreadAsMessageList | ( | ) | const |
Returns the Akonadi::Item bound to the current StorageModel that are part of the current thread.
The current thread is the thread that contains currentMessageItem(). The list may be empty if there is no currentMessageItem() or no StorageModel.
The returned list is guaranteed to be valid only until you return control to the main even loop. Don't store it for any longer. If you need to reference this set of messages at a later stage then take a look at createPersistentSet().
Definition at line 598 of file widget.cpp.
MessageList::Core::MessageItemSetReference Widget::currentThreadAsPersistentSet | ( | ) | const |
Return a persistent set from current thread.
Definition at line 700 of file widget.cpp.
void Widget::deletePersistentSet | ( | MessageList::Core::MessageItemSetReference | ref | ) |
Deletes the persistent set pointed by the specified reference.
If the set does not exist anymore, nothing happens.
Definition at line 666 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 209 of file widget.cpp.
bool Widget::focusNextMessageItem | ( | MessageList::Core::MessageTypeFilter | messageTypeFilter, |
bool | centerItem, | ||
bool | loop | ||
) |
Focuses the next message item in the view without actually selecting it.
messageTypeFilter can be used to restrict the selection to only certain message types.
If centerItem is true then the specified item will be positioned at the center of the view, if possible. If loop is true then the "next" algorithm will restart from the beginning of the list if the end is reached, otherwise it will just stop returning false.
Definition at line 157 of file widget.cpp.
bool Widget::focusPreviousMessageItem | ( | MessageList::Core::MessageTypeFilter | messageTypeFilter, |
bool | centerItem, | ||
bool | loop | ||
) |
Focuses the previous message item in the view without actually selecting it.
messageTypeFilter can be used to restrict the selection to only certain message types.
If centerItem is true then the specified item will be positioned at the center of the view, if possible. If loop is true then the "previous" algorithm will restart from the end of the list if the beginning is reached, otherwise it will just stop returning false.
Definition at line 162 of file widget.cpp.
void Widget::focusQuickSearch | ( | ) |
Sets the focus on the quick search line of the currently active tab.
Definition at line 203 of file widget.cpp.
bool Widget::getSelectionStats | ( | Akonadi::Item::List & | selectedSernums, |
Akonadi::Item::List & | selectedVisibleSernums, | ||
bool * | allSelectedBelongToSameThread, | ||
bool | includeCollapsedChildren = true |
||
) | const |
Fills the lists of the selected message serial numbers and of the selected+visible ones.
Returns true if the returned stats are valid (there is a current folder after all) and false otherwise. This is called by KMMainWidget in a single place so we optimize by making it a single sweep on the selection.
If includeCollapsedChildren is true then the children of the selected but collapsed items are also included in the stats
Definition at line 633 of file widget.cpp.
bool Widget::isThreaded | ( | ) | const |
Returns true if the current Aggregation is threaded, false otherwise (or if there is no current Aggregation).
Definition at line 623 of file widget.cpp.
QList< Akonadi::Item > Widget::itemListFromPersistentSet | ( | MessageList::Core::MessageItemSetReference | ref | ) |
Return Akonadi::Item from messageItemReference.
Definition at line 678 of file widget.cpp.
void Widget::markMessageItemsAsAboutToBeRemoved | ( | MessageList::Core::MessageItemSetReference | ref, |
bool | bMark | ||
) |
If bMark is true this function marks the messages as "about to be removed" so they appear dimmer and aren't selectable in the view.
If bMark is false then this function clears the "about to be removed" state for the specified MessageItems.
Definition at line 671 of file widget.cpp.
|
signal |
Emitted when a message is doubleclicked or activated by other input means.
|
signal |
Emitted when a message is selected (that is, single clicked and thus made current in the view) Note that this message CAN be 0 (when the current item is cleared, for example).
This signal is emitted when a SINGLE message is selected in the view, probably by clicking on it or by simple keyboard navigation. When multiple items are selected at once (by shift+clicking, for example) then you will get this signal only for the last clicked message (or at all, if the last shift+clicked thing is a group header...). You should handle selection changed in this case.
|
signal |
Emitted when a message wants its status to be changed.
void Widget::selectAll | ( | ) |
Selects all the items in the current folder.
Definition at line 182 of file widget.cpp.
bool Widget::selectFirstMessageItem | ( | MessageList::Core::MessageTypeFilter | messageTypeFilter, |
bool | centerItem | ||
) |
Selects the first message item in the view that matches the specified Core::MessageTypeFilter.
If centerItem is true then the specified item will be positioned at the center of the view, if possible.
If the current view is already loaded then the request will be satisfied immediately (well... if an unread message exists at all). If the current view is still loading then the selection of the first message will be scheduled to be executed when loading terminates.
So this function doesn't actually guarantee that an unread or new message was selected when the call returns. Take care :)
The function returns true if a message was selected and false otherwise.
Definition at line 172 of file widget.cpp.
void Widget::selectFocusedMessageItem | ( | bool | centerItem | ) |
Selects the currently focused message item.
May do nothing if the focused message item is already selected (which is very likely). If centerItem is true then the specified item will be positioned at the center of the view, if possible.
Definition at line 167 of file widget.cpp.
QList< Akonadi::Item::Id > Widget::selectionAsListMessageId | ( | bool | includeCollapsedChildren | ) | const |
Definition at line 585 of file widget.cpp.
QList< Akonadi::Item > Widget::selectionAsMessageItemList | ( | bool | includeCollapsedChildren = true | ) | const |
Returns the currently selected Items (bound to current StorageModel).
The list may be empty if there are no selected messages or no StorageModel.
If includeCollapsedChildren is true then the children of the selected but collapsed items are also added to the list.
The returned list is guaranteed to be valid only until you return control to the main even loop. Don't store it for any longer. If you need to reference this set of messages at a later stage then take a look at createPersistentSet().
Definition at line 558 of file widget.cpp.
QVector< qlonglong > Widget::selectionAsMessageItemListId | ( | bool | includeCollapsedChildren | ) | const |
Returns the currently selected Items id (bound to current StorageModel).
The list may be empty if there are no selected messages or no StorageModel.
If includeCollapsedChildren is true then the children of the selected but collapsed items are also added to the list.
The returned list is guaranteed to be valid only until you return control to the main even loop. Don't store it for any longer. If you need to reference this set of messages at a later stage then take a look at createPersistentSet().
Definition at line 571 of file widget.cpp.
QList< KMime::Message::Ptr > Widget::selectionAsMessageList | ( | bool | includeCollapsedChildren = true | ) | const |
Returns the currently selected KMime::Message::Ptr (bound to current StorageModel).
The list may be empty if there are no selected messages or no StorageModel.
If includeCollapsedChildren is true then the children of the selected but collapsed items are also added to the list.
The returned list is guaranteed to be valid only until you return control to the main even loop. Don't store it for any longer. If you need to reference this set of messages at a later stage then take a look at createPersistentSet().
Definition at line 545 of file widget.cpp.
MessageList::Core::MessageItemSetReference Widget::selectionAsPersistentSet | ( | bool | includeCollapsedChildren = true | ) | const |
Return a persistent set from current selection.
Definition at line 691 of file widget.cpp.
|
signal |
Emitted when the selection in the view changes.
bool Widget::selectionEmpty | ( | ) | const |
Fast function that determines if the selection is empty.
Definition at line 628 of file widget.cpp.
bool Widget::selectLastMessageItem | ( | MessageList::Core::MessageTypeFilter | messageTypeFilter, |
bool | centerItem | ||
) |
Selects the last message item in the view that matches the specified Core::MessageTypeFilter.
If centerItem is true then the specified item will be positioned at the center of the view, if possible.
The function returns true if a message was selected and false otherwise.
Definition at line 177 of file widget.cpp.
bool Widget::selectNextMessageItem | ( | MessageList::Core::MessageTypeFilter | messageTypeFilter, |
MessageList::Core::ExistingSelectionBehaviour | existingSelectionBehaviour, | ||
bool | centerItem, | ||
bool | loop | ||
) |
Selects the next message item in the view.
messageTypeFilter can be used to restrict the selection to only certain message types.
existingSelectionBehaviour specifies how the existing selection is manipulated. It may be cleared, expanded or grown/shrinked.
If centerItem is true then the specified item will be positioned at the center of the view, if possible. If loop is true then the "next" algorithm will restart from the beginning of the list if the end is reached, otherwise it will just stop returning false.
Definition at line 141 of file widget.cpp.
bool Widget::selectPreviousMessageItem | ( | MessageList::Core::MessageTypeFilter | messageTypeFilter, |
MessageList::Core::ExistingSelectionBehaviour | existingSelectionBehaviour, | ||
bool | centerItem, | ||
bool | loop | ||
) |
Selects the previous message item in the view.
If centerItem is true then the specified item will be positioned at the center of the view, if possible.
messageTypeFilter can be used to restrict the selection to only certain message types.
existingSelectionBehaviour specifies how the existing selection is manipulated. It may be cleared, expanded or grown/shrinked.
If loop is true then the "previous" algorithm will restart from the end of the list if the beginning is reached, otherwise it will just stop returning false.
Definition at line 149 of file widget.cpp.
void Widget::setAllGroupsExpanded | ( | bool | expand | ) |
If expand is true then it expands all the groups (only the toplevel group item: inner threads are NOT expanded).
If expand is false then it collapses all the groups. If no grouping is in effect then this function does nothing.
Definition at line 198 of file widget.cpp.
void Widget::setAllThreadsExpanded | ( | bool | expand | ) |
If expand is true then it expands all the threads, otherwise collapses them.
Definition at line 193 of file widget.cpp.
void Widget::setCurrentThreadExpanded | ( | bool | expand | ) |
If expand is true then it expands the current thread, otherwise collapses it.
Definition at line 188 of file widget.cpp.
void Widget::setXmlGuiClient | ( | KXMLGUIClient * | xmlGuiClient | ) |
Sets the XML GUI client which the view is used in.
This is needed if you want to use the built-in context menu. Passing 0 is ok and will disable the builtin context menu.
- Parameters
-
xmlGuiClient The KXMLGUIClient the view is used in.
Definition at line 106 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 355 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 365 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 382 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 321 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 250 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 287 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 230 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 303 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 279 of file widget.cpp.
|
protectedvirtual |
Reimplemented from MessageList::Core::Widget.
Reimplemented from MessageList::Core::Widget.
Definition at line 445 of file widget.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:55:32 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.