akonadi
#include <calendarbase.h>
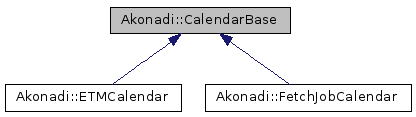
Public Types | |
typedef QSharedPointer < CalendarBase > | Ptr |
Signals | |
void | createFinished (bool success, const QString &errorMessage) |
void | deleteFinished (bool success, const QString &errorMessage) |
void | modifyFinished (bool success, const QString &errorMessage) |
Public Member Functions | |
CalendarBase (QObject *parent=0) | |
~CalendarBase () | |
bool | addEvent (const KCalCore::Event::Ptr &event) |
bool | addIncidence (const KCalCore::Incidence::Ptr &incidence) |
bool | addJournal (const KCalCore::Journal::Ptr &journal) |
bool | addTodo (const KCalCore::Todo::Ptr &todo) |
KCalCore::Incidence::List | childIncidences (const QString &parentUid) const |
KCalCore::Incidence::List | childIncidences (const Akonadi::Item::Id &parentId) const |
Akonadi::Item::List | childItems (const QString &parentUid) const |
Akonadi::Item::List | childItems (const Akonadi::Item::Id &parentId) const |
void | deleteAllEvents () |
void | deleteAllJournals () |
void | deleteAllTodos () |
bool | deleteEvent (const KCalCore::Event::Ptr &event) |
bool | deleteIncidence (const KCalCore::Incidence::Ptr &) |
bool | deleteJournal (const KCalCore::Journal::Ptr &journal) |
bool | deleteTodo (const KCalCore::Todo::Ptr &todo) |
void | endBatchAdding () |
Akonadi::IncidenceChanger * | incidenceChanger () const |
Akonadi::Item | item (const QString &uid) const |
Akonadi::Item | item (const KCalCore::Incidence::Ptr &incidence) const |
Akonadi::Item | item (Akonadi::Item::Id) const |
Akonadi::Item::List | itemList (const KCalCore::Incidence::List &incidenceList) const |
Akonadi::Item::List | items () const |
Akonadi::Item::List | items (Akonadi::Collection::Id) const |
bool | modifyIncidence (const KCalCore::Incidence::Ptr &newIncidence) |
void | setWeakPointer (const QWeakPointer< Akonadi::CalendarBase > &pointer) |
void | startBatchAdding () |
QWeakPointer< CalendarBase > | weakPointer () const |
Protected Member Functions | |
CalendarBase (CalendarBasePrivate *const d, QObject *parent) | |
Protected Attributes | |
QScopedPointer < CalendarBasePrivate > | d_ptr |
Detailed Description
The base class for all akonadi aware calendars.
Because it inherits KCalCore::Calendar, it provides seamless integration with KCalCore and KCalUtils libraries eliminating any need for adapter ( akonadi<->KCalCore ) classes.
- See also
- ETMCalendar
- FetchJobCalendar
- Since
- 4.11
Definition at line 50 of file calendarbase.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a CalendarBase object.
Definition at line 371 of file calendarbase.cpp.
CalendarBase::~CalendarBase | ( | ) |
Destroys the calendar.
Definition at line 386 of file calendarbase.cpp.
Member Function Documentation
bool CalendarBase::addEvent | ( | const KCalCore::Event::Ptr & | event | ) |
Adds an Event to the calendar.
It's added to akonadi in the background
- See also
- createFinished().
- Parameters
-
event the event to be addedreimp
Definition at line 525 of file calendarbase.cpp.
bool CalendarBase::addIncidence | ( | const KCalCore::Incidence::Ptr & | incidence | ) |
Adds an incidence to the calendar.
It's added to akonadi in the background
- See also
- createFinished().
- Parameters
-
incidence the incidence to addreimp
Definition at line 573 of file calendarbase.cpp.
bool CalendarBase::addJournal | ( | const KCalCore::Journal::Ptr & | journal | ) |
Adds a Journal to the calendar.
It's added to akonadi in the background
- See also
- createFinished().
- Parameters
-
journal the journal to addreimp
Definition at line 557 of file calendarbase.cpp.
bool CalendarBase::addTodo | ( | const KCalCore::Todo::Ptr & | todo | ) |
Adds a Todo to the calendar.
It's added to akonadi in the background
- See also
- createFinished().
- Parameters
-
todo the todo to addreimp
Definition at line 541 of file calendarbase.cpp.
KCalCore::Incidence::List CalendarBase::childIncidences | ( | const QString & | parentUid | ) | const |
Returns the child incidences of the parent identified by parentUid
.
Only the direct childs are returned
- Parameters
-
parentUid identifier of the parent incidence
Definition at line 475 of file calendarbase.cpp.
KCalCore::Incidence::List CalendarBase::childIncidences | ( | const Akonadi::Item::Id & | parentId | ) | const |
Returns the child incidences of the parent identified by parentId
.
Only the direct childs are returned
- Parameters
-
parentId identifier of the parent item
Definition at line 455 of file calendarbase.cpp.
Akonadi::Item::List CalendarBase::childItems | ( | const QString & | parentUid | ) | const |
Returns the child items of the parent identified by parentUid
.
Only the direct childs are returned
- Parameters
-
parentUid identifier of the parent incidence
Definition at line 510 of file calendarbase.cpp.
Akonadi::Item::List CalendarBase::childItems | ( | const Akonadi::Item::Id & | parentId | ) | const |
Returns the child items of the parent identified by parentId
.
Only the direct childs are returned
- Parameters
-
parentId identifier of the parent item
Definition at line 490 of file calendarbase.cpp.
|
signal |
This signal is emitted when an incidence is created in akonadi through add{Incidence,Event,Todo,Journal}.
- Parameters
-
success the success of the operation errorMessage if success
is false, contains the error message
void CalendarBase::deleteAllEvents | ( | ) |
Reimplementation of KCalCore::Calendar::deleteAllEvents() that does nothing.
Do not use this.
A convenience function that makes it easy to have a massive data-loss is a bad idea.
reimp
Definition at line 535 of file calendarbase.cpp.
void CalendarBase::deleteAllJournals | ( | ) |
Reimplementation of KCalCore::Calendar::deleteAllJournals() that does nothing.
Do not use this.
A convenience function that makes it easy to have a massive data-loss is a bad idea.
reimp
Definition at line 567 of file calendarbase.cpp.
void CalendarBase::deleteAllTodos | ( | ) |
Reimplementation of KCalCore::Calendar::deleteAllTodos() that does nothing.
Do not use this.
A convenience function that makes it easy to have a massive data-loss is a bad idea.
reimp
Definition at line 551 of file calendarbase.cpp.
bool CalendarBase::deleteEvent | ( | const KCalCore::Event::Ptr & | event | ) |
Deletes an Event from the calendar.
It's removed from akonadi in the background
- See also
- deleteFinished().
- Parameters
-
event the event to be deletedreimp
Definition at line 530 of file calendarbase.cpp.
|
signal |
This signal is emitted when an incidence is deleted in akonadi through delete{Incidence,Event,Todo,Journal} or deleteAll{Events,Todos,Journals}.
- Parameters
-
success the success of the operation errorMessage if success
is false, contains the error message
bool CalendarBase::deleteIncidence | ( | const KCalCore::Incidence::Ptr & | incidence | ) |
Deletes an incidence from the calendar.
It's removed from akonadi in the background
- See also
- deleteFinished().
- Parameters
-
incidence the incidence to deletereimp
Definition at line 613 of file calendarbase.cpp.
bool CalendarBase::deleteJournal | ( | const KCalCore::Journal::Ptr & | journal | ) |
Deletes a Journal from the calendar.
It's removed from akonadi in the background
- See also
- deleteFinished().
- Parameters
-
journal the journal to deletereimp
Definition at line 562 of file calendarbase.cpp.
bool CalendarBase::deleteTodo | ( | const KCalCore::Todo::Ptr & | todo | ) |
Deletes a Todo from the calendar.
It's removed from akonadi in the background
- See also
- deleteFinished().
- Parameters
-
todo the todo to deletereimp
Definition at line 546 of file calendarbase.cpp.
void CalendarBase::endBatchAdding | ( | ) |
Tells the Calendar that you stoped adding a batch of incidences.
- See also
- startBatchAdding()reimp
Definition at line 653 of file calendarbase.cpp.
IncidenceChanger * CalendarBase::incidenceChanger | ( | ) | const |
Returns the IncidenceChanger used by this calendar to make changes in akonadi.
Use this if you need the defaults used by CalendarBase.
Definition at line 642 of file calendarbase.cpp.
Akonadi::Item CalendarBase::item | ( | const QString & | uid | ) | const |
Returns the Item containing the incidence with uid uid
or an invalid Item if the incidence isn't found.
- See also
- Use item(Incidence::Ptr) instead where possible. This function doesn't take exceptions (recurrenceId) into account (and thus always returns the main event).
Definition at line 402 of file calendarbase.cpp.
Akonadi::Item Akonadi::CalendarBase::item | ( | const KCalCore::Incidence::Ptr & | incidence | ) | const |
Returns the Item containing the incidence with uid uid
or an invalid Item if the incidence isn't found.
Akonadi::Item CalendarBase::item | ( | Akonadi::Item::Id | id | ) | const |
Returns the Item with id
or an invalid Item if not found.
Definition at line 390 of file calendarbase.cpp.
Akonadi::Item::List CalendarBase::itemList | ( | const KCalCore::Incidence::List & | incidenceList | ) | const |
Returns the item list that corresponds to the incidenceList
.
Definition at line 440 of file calendarbase.cpp.
Akonadi::Item::List CalendarBase::items | ( | ) | const |
Returns the list of items contained in this calendar.
- See also
- incidences()
Definition at line 428 of file calendarbase.cpp.
Akonadi::Item::List CalendarBase::items | ( | Akonadi::Collection::Id | id | ) | const |
Returns the list of items contained in this calendar that belong to the specified collection.
- See also
- incidences() // TODO_KDE5: merge with items() overload
- Since
- 4.12
Definition at line 434 of file calendarbase.cpp.
|
signal |
This signal is emitted when an incidence is modified in akonadi through modifyIncidence().
- Parameters
-
success the success of the operation errorMessage if success
is false, contains the error message
bool CalendarBase::modifyIncidence | ( | const KCalCore::Incidence::Ptr & | newIncidence | ) |
Modifies an incidence.
The incidence with the same uid as newIncidence
will be updated with the contents of
- Parameters
-
newIncidence the incidence to modify
Definition at line 621 of file calendarbase.cpp.
void CalendarBase::setWeakPointer | ( | const QWeakPointer< Akonadi::CalendarBase > & | pointer | ) |
Sets the weak pointer that's associated with this instance.
Use this if later on you need to cast sender() into a QSharedPointer
- See also
- weakPointer()
Definition at line 630 of file calendarbase.cpp.
void CalendarBase::startBatchAdding | ( | ) |
Call this to tell the calendar that you're adding a batch of incidences.
So it doesn't, for example, ask the destination for each incidence.
- See also
- endBatchAdding()reimp
Definition at line 648 of file calendarbase.cpp.
QWeakPointer< CalendarBase > CalendarBase::weakPointer | ( | ) | const |
Returns the weak pointer set with setWeakPointer().
The default is an invalid weak pointer.
- See also
- setWeakPointer()
Definition at line 636 of file calendarbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.