akonadi
#include <etmcalendar.h>
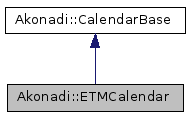
Public Types | |
enum | CollectionColumn { CollectionTitle = 0, CollectionColumnCount } |
typedef QSharedPointer < ETMCalendar > | Ptr |
![]() | |
typedef QSharedPointer < CalendarBase > | Ptr |
Signals | |
void | calendarChanged () |
void | collectionChanged (const Akonadi::Collection &, const QSet< QByteArray > &attributeNames) |
void | collectionsAdded (const Akonadi::Collection::List &collection) |
void | collectionsRemoved (const Akonadi::Collection::List &collection) |
![]() | |
void | createFinished (bool success, const QString &errorMessage) |
void | deleteFinished (bool success, const QString &errorMessage) |
void | modifyFinished (bool success, const QString &errorMessage) |
Public Member Functions | |
ETMCalendar (QObject *parent=0) | |
ETMCalendar (const QStringList &mimeTypes, QObject *parent=0) | |
~ETMCalendar () | |
KCalCore::Alarm::List | alarms (const KDateTime &from, const KDateTime &to, bool excludeBlockedAlarms) const |
KCheckableProxyModel * | checkableProxyModel () const |
Akonadi::Collection | collection (Akonadi::Collection::Id) const |
bool | collectionFilteringEnabled () const |
Akonadi::EntityTreeModel * | entityTreeModel () const |
bool | hasRight (const Akonadi::Item &item, Akonadi::Collection::Right right) const |
bool | hasRight (const QString &uid, Akonadi::Collection::Right right) const |
QAbstractItemModel * | model () const |
void | setCollectionFilteringEnabled (bool enable) |
![]() | |
CalendarBase (QObject *parent=0) | |
~CalendarBase () | |
bool | addEvent (const KCalCore::Event::Ptr &event) |
bool | addIncidence (const KCalCore::Incidence::Ptr &incidence) |
bool | addJournal (const KCalCore::Journal::Ptr &journal) |
bool | addTodo (const KCalCore::Todo::Ptr &todo) |
KCalCore::Incidence::List | childIncidences (const QString &parentUid) const |
KCalCore::Incidence::List | childIncidences (const Akonadi::Item::Id &parentId) const |
Akonadi::Item::List | childItems (const QString &parentUid) const |
Akonadi::Item::List | childItems (const Akonadi::Item::Id &parentId) const |
void | deleteAllEvents () |
void | deleteAllJournals () |
void | deleteAllTodos () |
bool | deleteEvent (const KCalCore::Event::Ptr &event) |
bool | deleteIncidence (const KCalCore::Incidence::Ptr &) |
bool | deleteJournal (const KCalCore::Journal::Ptr &journal) |
bool | deleteTodo (const KCalCore::Todo::Ptr &todo) |
void | endBatchAdding () |
Akonadi::IncidenceChanger * | incidenceChanger () const |
Akonadi::Item | item (const QString &uid) const |
Akonadi::Item | item (const KCalCore::Incidence::Ptr &incidence) const |
Akonadi::Item | item (Akonadi::Item::Id) const |
Akonadi::Item::List | itemList (const KCalCore::Incidence::List &incidenceList) const |
Akonadi::Item::List | items () const |
Akonadi::Item::List | items (Akonadi::Collection::Id) const |
bool | modifyIncidence (const KCalCore::Incidence::Ptr &newIncidence) |
void | setWeakPointer (const QWeakPointer< Akonadi::CalendarBase > &pointer) |
void | startBatchAdding () |
QWeakPointer< CalendarBase > | weakPointer () const |
Additional Inherited Members | |
![]() | |
CalendarBase (CalendarBasePrivate *const d, QObject *parent) | |
![]() | |
QScopedPointer < CalendarBasePrivate > | d_ptr |
Detailed Description
A KCalCore::Calendar that uses an EntityTreeModel to populate itself.
All non-idempotent KCalCore::Calendar methods interact with Akonadi. If you need a need a non-persistent calendar use FetchJobCalendar.
ETMCalendar allows to be populated with only a subset of your calendar items, by using a KCheckableProxyModel to specify which collections to be used and/or by setting a KCalCore::CalFilter.
- See also
- FetchJobCalendar
- CalendarBase
- Since
- 4.11
Definition at line 54 of file etmcalendar.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a new ETMCalendar.
Loading begins immediately, asynchronously.
Definition at line 453 of file etmcalendar.cpp.
|
explicit |
Constructs a new ETMCalendar that will only load the specified mime types.
Use this ctor to ignore journals or to-dos for example. If no mime types are specified, all mime types will be used.
Definition at line 459 of file etmcalendar.cpp.
ETMCalendar::~ETMCalendar | ( | ) |
Destroys this ETMCalendar.
Definition at line 466 of file etmcalendar.cpp.
Member Function Documentation
|
signal |
Emitted whenever an Item is inserted, removed or modified.
KCheckableProxyModel * ETMCalendar::checkableProxyModel | ( | ) | const |
Returns the KCheckableProxyModel used to select from which collections should the calendar be populated from.
Definition at line 497 of file etmcalendar.cpp.
Akonadi::Collection ETMCalendar::collection | ( | Akonadi::Collection::Id | id | ) | const |
Returns the collection having id
.
Use this instead of creating a new collection, the returned collection will have the correct right, name, display name, etc all set.
Definition at line 471 of file etmcalendar.cpp.
|
signal |
This signal is emitted if a collection has been changed (properties or attributes).
- Parameters
-
collection The changed collection. attributeNames The names of the collection attributes that have been changed.
bool ETMCalendar::collectionFilteringEnabled | ( | ) | const |
Returns whether collection filtering is enabled.
Default is true.
- See also
- setCollectionFilteringEnabled()
Definition at line 595 of file etmcalendar.cpp.
|
signal |
This signal is emitted when one or more collections are added to the ETM.
- Parameters
-
collection non empty list of collections
|
signal |
This signal is emitted when one or more collections are deleted from the ETM.
- Parameters
-
collection non empty list of collections
Akonadi::EntityTreeModel * ETMCalendar::entityTreeModel | ( | ) | const |
Returns the underlying EntityTreeModel.
For most uses, you'll want model() or the KCalCore::Calendar interface instead.
It contains every item and collection with calendar mime type, doesn't have KCalCore filtering and doesn't honour any collection selection.
This method is exposed for performance reasons only, so you can reuse it, since it's resource savy.
- See also
- model()
Definition at line 571 of file etmcalendar.cpp.
bool ETMCalendar::hasRight | ( | const Akonadi::Item & | item, |
Akonadi::Collection::Right | right | ||
) | const |
Returns true if the collection owning incidence has
righ right
.
Definition at line 482 of file etmcalendar.cpp.
bool ETMCalendar::hasRight | ( | const QString & | uid, |
Akonadi::Collection::Right | right | ||
) | const |
This is an overloaded function.
- Parameters
-
uid the identifier for the incidence to check for rights right the access right to check for
- See also
- hasRight()
Definition at line 477 of file etmcalendar.cpp.
QAbstractItemModel * ETMCalendar::model | ( | ) | const |
Convenience method to access the contents of this KCalCore::Calendar through a QAIM interface.
Like through calendar interface, the model only items of selected collections. To select or unselect collections, see checkableProxyModel().
Definition at line 491 of file etmcalendar.cpp.
void ETMCalendar::setCollectionFilteringEnabled | ( | bool | enable | ) |
Enable or disable collection filtering.
If true, the calendar will only contain items of selected collections.
- Parameters
-
enable enables collection filtering if set as true
Definition at line 577 of file etmcalendar.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.