akonadi
#include <collection.h>
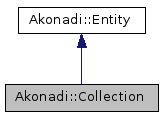
Public Types | |
typedef QList< Collection > | List |
enum | Right { ReadOnly = 0x0, CanChangeItem = 0x1, CanCreateItem = 0x2, CanDeleteItem = 0x4, CanChangeCollection = 0x8, CanCreateCollection = 0x10, CanDeleteCollection = 0x20, CanLinkItem = 0x40, CanUnlinkItem = 0x80, AllRights } |
enum | UrlType { UrlShort = 0, UrlWithName = 1 } |
![]() | |
enum | CreateOption { AddIfMissing } |
typedef qint64 | Id |
Public Member Functions | |
Collection () | |
Collection (Id id) | |
Collection (const Collection &other) | |
~Collection () | |
CachePolicy | cachePolicy () const |
QStringList | contentMimeTypes () const |
QString | displayName () const |
bool | isVirtual () const |
QString | name () const |
AKONADI_DEPRECATED Id | parent () const |
AKONADI_DEPRECATED QString | parentRemoteId () const |
QString | resource () const |
Rights | rights () const |
void | setCachePolicy (const CachePolicy &policy) |
void | setContentMimeTypes (const QStringList &types) |
void | setName (const QString &name) |
AKONADI_DEPRECATED void | setParent (Id parent) |
AKONADI_DEPRECATED void | setParent (const Collection &collection) |
AKONADI_DEPRECATED void | setParentRemoteId (const QString &identifier) |
void | setResource (const QString &identifier) |
void | setRights (Rights rights) |
void | setStatistics (const CollectionStatistics &statistics) |
void | setVirtual (bool isVirtual) |
CollectionStatistics | statistics () const |
KUrl | url () const |
KUrl | url (UrlType type) const |
![]() | |
void | addAttribute (Attribute *attribute) |
Attribute * | attribute (const QByteArray &name) const |
template<typename T > | |
T * | attribute (CreateOption option) |
template<typename T > | |
T * | attribute () const |
Attribute::List | attributes () const |
void | clearAttributes () |
bool | hasAttribute (const QByteArray &name) const |
template<typename T > | |
bool | hasAttribute () const |
Id | id () const |
bool | isValid () const |
bool | operator!= (const Entity &other) const |
bool | operator< (const Entity &other) const |
Entity & | operator= (const Entity &other) |
bool | operator== (const Entity &other) const |
Collection | parentCollection () const |
Collection & | parentCollection () |
QString | remoteId () const |
QString | remoteRevision () const |
void | removeAttribute (const QByteArray &name) |
template<typename T > | |
void | removeAttribute () |
void | setId (Id identifier) |
void | setParentCollection (const Collection &parent) |
void | setRemoteId (const QString &id) |
void | setRemoteRevision (const QString &revision) |
Static Public Member Functions | |
static Collection | fromUrl (const KUrl &url) |
static QString | mimeType () |
static Collection | root () |
static QString | virtualMimeType () |
Additional Inherited Members | |
![]() | |
Entity (const Entity &other) | |
~Entity () | |
Detailed Description
Represents a collection of PIM items.
This class represents a collection of PIM items, such as a folder on a mail- or groupware-server.
Collections are hierarchical, i.e., they may have a parent collection.
Definition at line 75 of file collection.h.
Member Typedef Documentation
typedef QList<Collection> Akonadi::Collection::List |
Describes a list of collections.
Definition at line 81 of file collection.h.
Member Enumeration Documentation
Describes rights of a collection.
Definition at line 86 of file collection.h.
Describes the type of url which is returned in url().
- Since
- 4.7
Enumerator | |
---|---|
UrlShort |
A short url which contains the identifier only (equivalent to url()) |
UrlWithName |
A url with identifier and name. |
Definition at line 264 of file collection.h.
Constructor & Destructor Documentation
Collection::Collection | ( | ) |
Creates an invalid collection.
Definition at line 59 of file collection.cpp.
|
explicit |
Create a new collection.
- Parameters
-
id The unique identifier of the collection.
Definition at line 67 of file collection.cpp.
Collection::~Collection | ( | ) |
Destroys the collection.
Definition at line 77 of file collection.cpp.
Collection::Collection | ( | const Collection & | other | ) |
Creates a collection from an other
collection.
Definition at line 72 of file collection.cpp.
Member Function Documentation
CachePolicy Collection::cachePolicy | ( | ) | const |
Returns the cache policy of the collection.
Definition at line 249 of file collection.cpp.
QStringList Collection::contentMimeTypes | ( | ) | const |
Returns a list of possible content mimetypes, e.g.
message/rfc822, x-akonadi/collection for a mail folder that supports sub-folders.
Definition at line 115 of file collection.cpp.
QString Collection::displayName | ( | ) | const |
Returns the display name (EntityDisplayAttribute::displayName()) if set, and Collection::name() otherwise.
For human-readable strings this is preferred over Collection::name().
- Since
- 4.11
Definition at line 86 of file collection.cpp.
|
static |
Creates a collection from the given url
.
Definition at line 172 of file collection.cpp.
bool Collection::isVirtual | ( | ) | const |
Returns whether the collection is virtual, for example a search collection.
- Since
- 4.6
Definition at line 261 of file collection.cpp.
|
static |
Returns the mimetype used for collections.
Definition at line 197 of file collection.cpp.
QString Collection::name | ( | ) | const |
Returns the i18n'ed name of the collection.
Definition at line 81 of file collection.cpp.
Collection::Id Collection::parent | ( | ) | const |
Returns the identifier of the parent collection.
Definition at line 129 of file collection.cpp.
QString Collection::parentRemoteId | ( | ) | const |
Returns the parent remote identifier.
- Note
- This usually returns nothing for collections retrieved from the backend.
Definition at line 144 of file collection.cpp.
QString Collection::resource | ( | ) | const |
Returns the identifier of the resource owning the collection.
Definition at line 207 of file collection.cpp.
Collection::Rights Collection::rights | ( | ) | const |
Returns the rights the user has on the collection.
Definition at line 99 of file collection.cpp.
|
static |
Returns the root collection.
Definition at line 192 of file collection.cpp.
void Collection::setCachePolicy | ( | const CachePolicy & | policy | ) |
Sets the cache policy
of the collection.
Definition at line 254 of file collection.cpp.
void Collection::setContentMimeTypes | ( | const QStringList & | types | ) |
Sets the list of possible content mime types
.
Definition at line 120 of file collection.cpp.
void Collection::setName | ( | const QString & | name | ) |
Sets the i18n'ed name of the collection.
- Parameters
-
name The new collection name.
Definition at line 93 of file collection.cpp.
void Collection::setParent | ( | Id | parent | ) |
Sets the identifier of the parent
collection.
- Parameters
-
parent the parent identifier to set
Definition at line 134 of file collection.cpp.
void Collection::setParent | ( | const Collection & | collection | ) |
Sets the parent collection
.
- Parameters
-
collection the parent collection to set
Definition at line 139 of file collection.cpp.
void Collection::setParentRemoteId | ( | const QString & | identifier | ) |
Sets the parent's remote identifier
.
- Parameters
-
identifier the parent's remote identifier to set
Definition at line 149 of file collection.cpp.
void Collection::setResource | ( | const QString & | identifier | ) |
Sets the identifier
of the resource owning the collection.
Definition at line 212 of file collection.cpp.
void Collection::setRights | ( | Rights | rights | ) |
Sets the rights
the user has on the collection.
Definition at line 109 of file collection.cpp.
void Collection::setStatistics | ( | const CollectionStatistics & | statistics | ) |
Sets the collection statistics
for the collection.
Definition at line 243 of file collection.cpp.
void Akonadi::Collection::setVirtual | ( | bool | isVirtual | ) |
Sets whether the collection is virtual or not.
Virtual collections can't be converted to non-virtual and vice versa.
- Parameters
-
isVirtual virtual collection if true
, otherwise a normal collection
- Since
- 4.10
Definition at line 266 of file collection.cpp.
CollectionStatistics Collection::statistics | ( | ) | const |
Returns the collection statistics of the collection.
Definition at line 238 of file collection.cpp.
KUrl Collection::url | ( | ) | const |
Returns the url of the collection.
- Todo:
- KDE5 remove in favor of url( UrlType type = UrlShort ).
Definition at line 154 of file collection.cpp.
KUrl Collection::url | ( | UrlType | type | ) | const |
Returns the url of the collection.
- Parameters
-
type the type of url
- Since
- 4.7
Definition at line 159 of file collection.cpp.
|
static |
Returns the mimetype used for virtual collections.
- Since
- 4.11
Definition at line 202 of file collection.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.