akonadi
#include <collectionfetchjob.h>
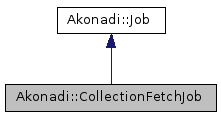
Public Types | |
enum | Type { Base, FirstLevel, Recursive, NonOverlappingRoots } |
![]() | |
enum | Error { ConnectionFailed = UserDefinedError, ProtocolVersionMismatch, UserCanceled, Unknown, UserError = UserDefinedError + 42 } |
typedef QList< Job * > | List |
Signals | |
void | collectionsReceived (const Akonadi::Collection::List &collections) |
![]() | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
Public Member Functions | |
CollectionFetchJob (const Collection &collection, Type type=FirstLevel, QObject *parent=0) | |
CollectionFetchJob (const Collection::List &collections, QObject *parent=0) | |
CollectionFetchJob (const Collection::List &collections, Type type, QObject *parent=0) | |
CollectionFetchJob (const QList< Collection::Id > &collections, Type type=Base, QObject *parent=0) | |
virtual | ~CollectionFetchJob () |
Collection::List | collections () const |
CollectionFetchScope & | fetchScope () |
AKONADI_DEPRECATED void | includeStatistics (bool include=true) |
AKONADI_DEPRECATED void | includeUnsubscribed (bool include=true) |
void | setFetchScope (const CollectionFetchScope &fetchScope) |
AKONADI_DEPRECATED void | setResource (const QString &resource) |
![]() | |
Job (QObject *parent=0) | |
virtual | ~Job () |
virtual QString | errorString () const |
void | start () |
Protected Member Functions | |
virtual void | doHandleResponse (const QByteArray &tag, const QByteArray &data) |
virtual void | doStart () |
![]() | |
virtual bool | addSubjob (KJob *job) |
virtual bool | doKill () |
void | emitWriteFinished () |
virtual bool | removeSubjob (KJob *job) |
Additional Inherited Members | |
![]() | |
virtual void | slotResult (KJob *job) |
Detailed Description
Job that fetches collections from the Akonadi storage.
This class can be used to retrieve the complete or partial collection tree from the Akonadi storage. This fetches collection data, not item data.
Definition at line 53 of file collectionfetchjob.h.
Member Enumeration Documentation
Describes the type of fetch depth.
Definition at line 61 of file collectionfetchjob.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new collection fetch job.
If the given base collection has a unique identifier, this is used to identify the collection in the Akonadi server. If only a remote identifier is avaiable the collection is identified using that, provided that a resource search context has been specified. There are two ways of doing that: by calling setResource(), or globally using Akonadi::ResourceSelectJob.
- Parameters
-
collection The base collection for the listing. type The type of fetch depth. parent The parent object.
Definition at line 114 of file collectionfetchjob.cpp.
|
explicit |
Creates a new collection fetch job to retrieve a list of collections.
The same rules for identifiers apply as noted in the constructor description.
- Parameters
-
collections A list of collections to fetch. Must not be empty. parent The parent object.
Definition at line 124 of file collectionfetchjob.cpp.
CollectionFetchJob::CollectionFetchJob | ( | const Collection::List & | collections, |
Type | type, | ||
QObject * | parent = 0 |
||
) |
Creates a new collection fetch job to retrieve a list of collections.
The same rules for identifiers apply as noted in the constructor description.
- Parameters
-
collections A list of collections to fetch. Must not be empty. type The type of fetch depth. parent The parent object.
- Todo:
- KDE5 merge with ctor above.
- Since
- 4.7
Definition at line 139 of file collectionfetchjob.cpp.
|
explicit |
Convenience ctor equivalent to CollectionFetchJob( const Collection::List &collections, Type type, QObject *parent = 0 )
- Since
- 4.8
- Parameters
-
collections list of collection ids type fetch job type parent parent object
Definition at line 154 of file collectionfetchjob.cpp.
|
virtual |
Destroys the collection fetch job.
Definition at line 171 of file collectionfetchjob.cpp.
Member Function Documentation
Akonadi::Collection::List CollectionFetchJob::collections | ( | ) | const |
Returns the list of fetched collection.
Definition at line 175 of file collectionfetchjob.cpp.
|
signal |
This signal is emitted whenever the job has received collections.
- Parameters
-
collections The received collections.
|
protectedvirtual |
This method should be reimplemented in the concrete jobs in case you want to handle incoming data.
It will be called on received data from the backend. The default implementation does nothing.
- Parameters
-
tag The tag of the corresponding command, empty if this is an untagged response. data The received data.
Reimplemented from Akonadi::Job.
Definition at line 301 of file collectionfetchjob.cpp.
|
protectedvirtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implements Akonadi::Job.
Definition at line 182 of file collectionfetchjob.cpp.
CollectionFetchScope & CollectionFetchJob::fetchScope | ( | ) |
Returns the collection fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the CollectionFetchScope documentation for an example.
- Returns
- a reference to the current collection fetch scope
- See also
- setFetchScope() for replacing the current collection fetch scope
- Since
- 4.4
Definition at line 437 of file collectionfetchjob.cpp.
void CollectionFetchJob::includeStatistics | ( | bool | include = true | ) |
Include also statistics about the collections.
- Since
- 4.3
- Deprecated:
- Use CollectionFetchScope instead.
- Parameters
-
include whether to also fetch statistics
Definition at line 424 of file collectionfetchjob.cpp.
void CollectionFetchJob::includeUnsubscribed | ( | bool | include = true | ) |
Include also unsubscribed collections.
- Deprecated:
- Use CollectionFetchScope instead.
- Parameters
-
include whether to also fetch unsubscribed collections
Definition at line 417 of file collectionfetchjob.cpp.
void CollectionFetchJob::setFetchScope | ( | const CollectionFetchScope & | fetchScope | ) |
Sets the collection fetch scope.
The CollectionFetchScope controls how much of a collection's data is fetched from the server as well as a filter to select which collections to fetch.
- Parameters
-
fetchScope The new scope for collection fetch operations.
- See also
- fetchScope()
- Since
- 4.4
Definition at line 431 of file collectionfetchjob.cpp.
void CollectionFetchJob::setResource | ( | const QString & | resource | ) |
Sets a resource identifier to limit collection listing to one resource.
- Parameters
-
resource The resource identifier.
- Deprecated:
- Use CollectionFetchScope instead.
Definition at line 327 of file collectionfetchjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.