akonadi
#include <job.h>
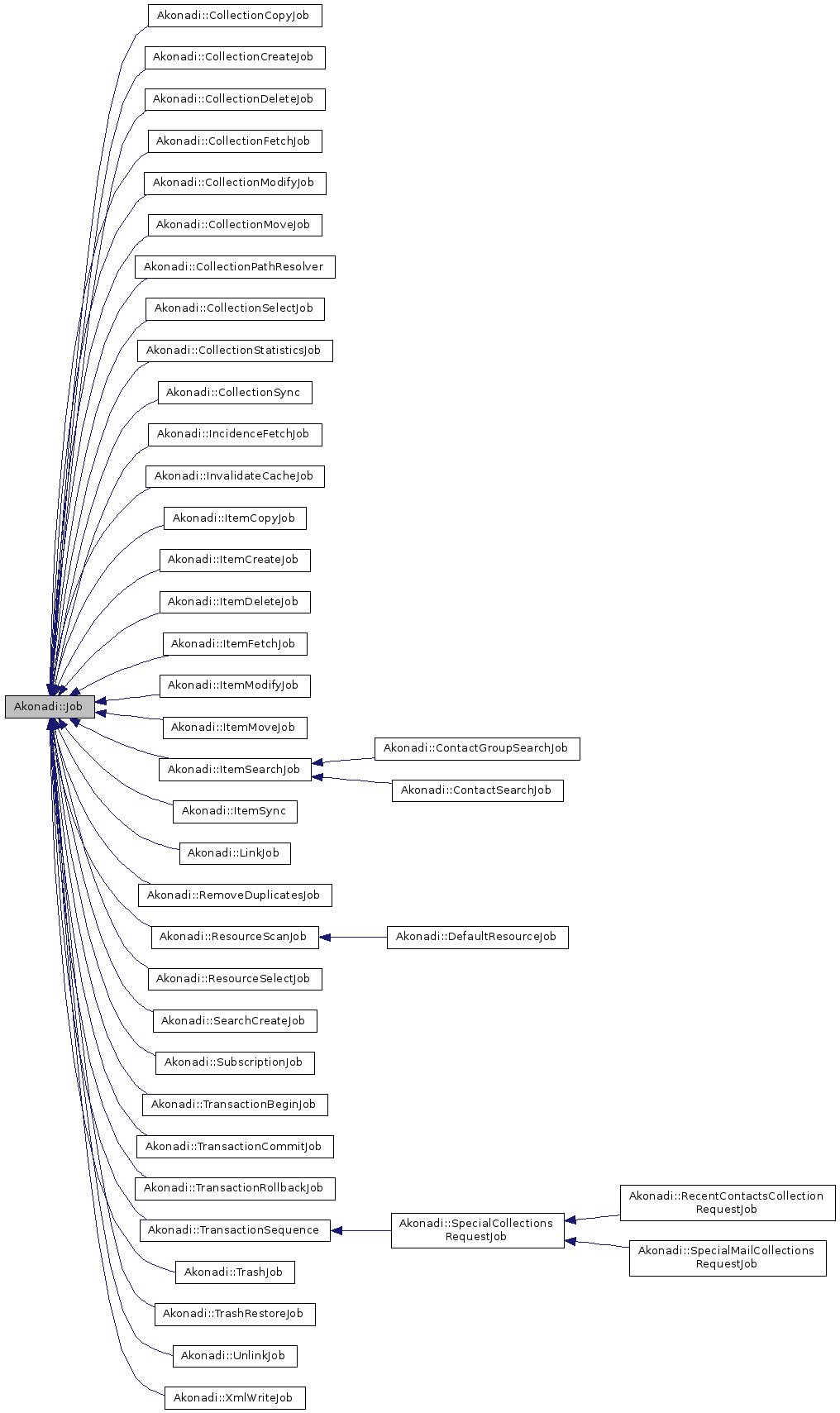
Public Types | |
enum | Error { ConnectionFailed = UserDefinedError, ProtocolVersionMismatch, UserCanceled, Unknown, UserError = UserDefinedError + 42 } |
typedef QList< Job * > | List |
Signals | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
Public Member Functions | |
Job (QObject *parent=0) | |
virtual | ~Job () |
virtual QString | errorString () const |
void | start () |
Protected Slots | |
virtual void | slotResult (KJob *job) |
Protected Member Functions | |
virtual bool | addSubjob (KJob *job) |
virtual void | doHandleResponse (const QByteArray &tag, const QByteArray &data) |
virtual bool | doKill () |
virtual void | doStart ()=0 |
void | emitWriteFinished () |
virtual bool | removeSubjob (KJob *job) |
Detailed Description
Base class for all actions in the Akonadi storage.
This class encapsulates a request to the pim storage service, the code looks like
The job is queued for execution as soon as the event loop is entered again.
And the slotResult is usually at least:
With the synchronous interface the code looks like
- Warning
- Using the synchronous method is error prone, use this only if the asynchronous access is not possible. See the documentation of KJob::exec() for more details.
Subclasses must reimplement doStart().
- Note
- KJob-derived objects delete itself, it is thus not possible to create job objects on the stack!
Member Typedef Documentation
typedef QList<Job*> Akonadi::Job::List |
Member Enumeration Documentation
enum Akonadi::Job::Error |
Describes the error codes that can be emitted by this class.
Subclasses can provide additional codes, starting from UserError onwards
Enumerator | |
---|---|
ConnectionFailed |
The connection to the Akonadi server failed. |
ProtocolVersionMismatch |
The server protocol version is too old or too new. |
UserCanceled |
The user canceld this job. |
Unknown |
Unknown error. |
UserError |
Starting point for error codes defined by sub-classes. |
Constructor & Destructor Documentation
|
explicit |
Member Function Documentation
|
signal |
This signal is emitted directly before the job will be started.
- Parameters
-
job The started job.
|
protectedvirtual |
Adds the given job as a subjob to this job.
This method is automatically called if you construct a job using another job as parent object. The base implementation does the necessary setup to share the network connection with the backend.
- Parameters
-
job The new subjob.
Reimplemented in Akonadi::TransactionSequence.
|
protectedvirtual |
This method should be reimplemented in the concrete jobs in case you want to handle incoming data.
It will be called on received data from the backend. The default implementation does nothing.
- Parameters
-
tag The tag of the corresponding command, empty if this is an untagged response. data The received data.
Reimplemented in Akonadi::ItemFetchJob, Akonadi::ItemModifyJob, Akonadi::CollectionFetchJob, Akonadi::ItemSearchJob, Akonadi::SearchCreateJob, Akonadi::ItemCreateJob, Akonadi::CollectionStatisticsJob, Akonadi::CollectionCreateJob, and Akonadi::SubscriptionJob.
|
protectedvirtual |
Kills the execution of the job.
Reimplemented in Akonadi::RemoveDuplicatesJob.
|
protectedpure virtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implemented in Akonadi::ItemFetchJob, Akonadi::ItemModifyJob, Akonadi::CollectionFetchJob, Akonadi::ItemSync, Akonadi::DefaultResourceJob, Akonadi::ItemSearchJob, Akonadi::TransactionCommitJob, Akonadi::TrashJob, Akonadi::TransactionSequence, Akonadi::SpecialCollectionsRequestJob, Akonadi::ItemDeleteJob, Akonadi::CollectionSync, Akonadi::CollectionModifyJob, Akonadi::TransactionRollbackJob, Akonadi::ItemCreateJob, Akonadi::SearchCreateJob, Akonadi::ResourceSelectJob, Akonadi::CollectionStatisticsJob, Akonadi::ItemMoveJob, Akonadi::ItemCopyJob, Akonadi::CollectionDeleteJob, Akonadi::CollectionPathResolver, Akonadi::LinkJob, Akonadi::ResourceScanJob, Akonadi::UnlinkJob, Akonadi::TrashRestoreJob, Akonadi::CollectionCreateJob, Akonadi::CollectionCopyJob, Akonadi::RemoveDuplicatesJob, Akonadi::SubscriptionJob, Akonadi::CollectionMoveJob, Akonadi::TransactionBeginJob, Akonadi::CollectionSelectJob, Akonadi::IncidenceFetchJob, Akonadi::InvalidateCacheJob, and Akonadi::XmlWriteJob.
|
protected |
Call this method to indicate that this job will not call writeData() again.
- See also
- writeFinished()
|
virtual |
|
protectedvirtual |
void Job::start | ( | ) |
|
signal |
This signal is emitted if the job has finished all write operations, ie.
if this signal is emitted, the job guarantees to not call writeData() again. Do not emit this signal directly, call emitWriteFinished() instead.
- Parameters
-
job This job.
- See also
- emitWriteFinished()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.