akonadi
#include <itemfetchjob.h>
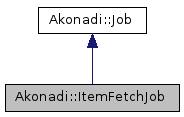
Signals | |
void | itemsReceived (const Akonadi::Item::List &items) |
![]() | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
Public Member Functions | |
ItemFetchJob (const Collection &collection, QObject *parent=0) | |
ItemFetchJob (const Item &item, QObject *parent=0) | |
ItemFetchJob (const Item::List &items, QObject *parent=0) | |
ItemFetchJob (const QList< Item::Id > &items, QObject *parent=0) | |
virtual | ~ItemFetchJob () |
void | clearItems () |
ItemFetchScope & | fetchScope () |
Item::List | items () const |
void | setCollection (const Collection &collection) |
void | setFetchScope (ItemFetchScope &fetchScope) |
void | setFetchScope (const ItemFetchScope &fetchScope) |
![]() | |
Job (QObject *parent=0) | |
virtual | ~Job () |
virtual QString | errorString () const |
void | start () |
Protected Member Functions | |
virtual void | doHandleResponse (const QByteArray &tag, const QByteArray &data) |
virtual void | doStart () |
![]() | |
virtual bool | addSubjob (KJob *job) |
virtual bool | doKill () |
void | emitWriteFinished () |
virtual bool | removeSubjob (KJob *job) |
Additional Inherited Members | |
![]() | |
enum | Error { ConnectionFailed = UserDefinedError, ProtocolVersionMismatch, UserCanceled, Unknown, UserError = UserDefinedError + 42 } |
typedef QList< Job * > | List |
![]() | |
virtual void | slotResult (KJob *job) |
Detailed Description
Job that fetches items from the Akonadi storage.
This class is used to fetch items from the Akonadi storage. Which parts of the items (e.g. headers only, attachments or all) can be specified by the ItemFetchScope.
Note that ItemFetchJob does not refresh the Akonadi storage from the backend; this is unnecessary due to the fact that backend updates automatically trigger an update to the Akonadi database whenever they occur (unless the resource is offline).
Note that items can not be created in the root collection (Collection::root()) and therefore can not be fetched from there either. That is - an item fetch in the root collection will yield an empty list.
Example:
Definition at line 82 of file itemfetchjob.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new item fetch job that retrieves all items inside the given collection.
- Parameters
-
collection The parent collection to fetch all items from. parent The parent object.
Definition at line 140 of file itemfetchjob.cpp.
|
explicit |
Creates a new item fetch job that retrieves the specified item.
If the item has a uid set, this is used to identify the item on the Akonadi server. If only a remote identifier is available, that is used. However, as remote identifiers are not necessarily globally unique, you need to specify the resource and/or collection to search in in that case, using setCollection() or Akonadi::ResourceSelectJob.
- Parameters
-
item The item to fetch. parent The parent object.
Definition at line 150 of file itemfetchjob.cpp.
|
explicit |
Creates a new item fetch job that retrieves the specified items.
If the items have a uid set, this is used to identify the item on the Akonadi server. If only a remote identifier is available, that is used. However, as remote identifiers are not necessarily globally unique, you need to specify the resource and/or collection to search in in that case, using setCollection() or Akonadi::ResourceSelectJob.
- Parameters
-
items The items to fetch. parent The parent object.
- Since
- 4.4
|
explicit |
Convenience ctor equivalent to ItemFetchJob( const Item::List &items, QObject *parent = 0 )
- Since
- 4.8
|
virtual |
Destroys the item fetch job.
Definition at line 178 of file itemfetchjob.cpp.
Member Function Documentation
void ItemFetchJob::clearItems | ( | ) |
Save memory by clearing the fetched items.
- Since
- 4.12
Definition at line 235 of file itemfetchjob.cpp.
|
protectedvirtual |
This method should be reimplemented in the concrete jobs in case you want to handle incoming data.
It will be called on received data from the backend. The default implementation does nothing.
- Parameters
-
tag The tag of the corresponding command, empty if this is an untagged response. data The received data.
Reimplemented from Akonadi::Job.
Definition at line 201 of file itemfetchjob.cpp.
|
protectedvirtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implements Akonadi::Job.
Definition at line 182 of file itemfetchjob.cpp.
ItemFetchScope & ItemFetchJob::fetchScope | ( | ) |
Returns the item fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the ItemFetchScope documentation for an example.
- Returns
- a reference to the current item fetch scope
- See also
- setFetchScope() for replacing the current item fetch scope
Definition at line 256 of file itemfetchjob.cpp.
Item::List ItemFetchJob::items | ( | ) | const |
Returns the fetched items.
- Note
- The items are invalid before the result( KJob* ) signal has been emitted or if an error occurred.
Definition at line 228 of file itemfetchjob.cpp.
|
signal |
This signal is emitted whenever new items have been fetched completely.
- Note
- This is an optimization; instead of waiting for the end of the job and calling items(), you can connect to this signal and get the items incrementally.
- Parameters
-
items The fetched items.
void ItemFetchJob::setCollection | ( | const Collection & | collection | ) |
Specifies the collection the item is in.
This is only required when retrieving an item based on its remote id which might not be unique globally.
- See also
- Akonadi::ResourceSelectJob
Definition at line 263 of file itemfetchjob.cpp.
void ItemFetchJob::setFetchScope | ( | ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
The ItemFetchScope controls how much of an item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- fetchScope()
Definition at line 242 of file itemfetchjob.cpp.
void ItemFetchJob::setFetchScope | ( | const ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
The ItemFetchScope controls how much of an item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- fetchScope()
- Since
- 4.4
Definition at line 249 of file itemfetchjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.