akonadi
#include <itemsync.h>
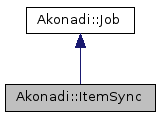
Public Types | |
enum | TransactionMode { SingleTransaction, MultipleTransactions, NoTransaction } |
![]() | |
enum | Error { ConnectionFailed = UserDefinedError, ProtocolVersionMismatch, UserCanceled, Unknown, UserError = UserDefinedError + 42 } |
typedef QList< Job * > | List |
Public Member Functions | |
ItemSync (const Collection &collection, QObject *parent=0) | |
~ItemSync () | |
void | deliveryDone () |
ItemFetchScope & | fetchScope () |
void | rollback () |
void | setFetchScope (ItemFetchScope &fetchScope) |
void | setFullSyncItems (const Item::List &items) |
void | setIncrementalSyncItems (const Item::List &changedItems, const Item::List &removedItems) |
void | setStreamingEnabled (bool enable) |
void | setTotalItems (int amount) |
void | setTransactionMode (TransactionMode mode) |
![]() | |
Job (QObject *parent=0) | |
virtual | ~Job () |
virtual QString | errorString () const |
void | start () |
Protected Member Functions | |
void | doStart () |
void | slotResult (KJob *job) |
virtual bool | updateItem (const Item &storedItem, Item &newItem) |
![]() | |
virtual bool | addSubjob (KJob *job) |
virtual void | doHandleResponse (const QByteArray &tag, const QByteArray &data) |
virtual bool | doKill () |
void | emitWriteFinished () |
virtual bool | removeSubjob (KJob *job) |
Additional Inherited Members | |
![]() | |
void | aboutToStart (Akonadi::Job *job) |
void | writeFinished (Akonadi::Job *job) |
![]() |
Detailed Description
Syncs between items known to a client (usually a resource) and the Akonadi storage.
Remote Id must only be set by the resource storing the item, other clients should leave it empty, since the resource responsible for the target collection will be notified about the addition and then create a suitable remote Id.
There are two different forms of ItemSync usage:
- Full-Sync: meaning the client provides all valid items, i.e. any item not part of the list but currently stored in Akonadi will be removed
- Incremental-Sync: meaning the client provides two lists, one for items which are new or modified and one for items which should be removed. Any item not part of either list but currently stored in Akonadi will not be changed.
- Note
- This is provided for convenience to implement "save all" like behavior, however it is strongly recommended to use single item jobs whenever possible, e.g. ItemCreateJob, ItemModifyJob and ItemDeleteJob
Definition at line 53 of file itemsync.h.
Member Enumeration Documentation
Transaction mode used by ItemSync.
- Since
- 4.6
Definition at line 158 of file itemsync.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new item synchronizer.
- Parameters
-
collection The collection we are syncing. parent The parent object.
Definition at line 130 of file itemsync.cpp.
ItemSync::~ItemSync | ( | ) |
Destroys the item synchronizer.
Definition at line 137 of file itemsync.cpp.
Member Function Documentation
void ItemSync::deliveryDone | ( | ) |
Notify ItemSync that all remote items have been delivered.
Only call this in streaming mode.
Definition at line 439 of file itemsync.cpp.
|
protectedvirtual |
This method must be reimplemented in the concrete jobs.
It will be called after the job has been started and a connection to the Akonadi backend has been established.
Implements Akonadi::Job.
Definition at line 194 of file itemsync.cpp.
ItemFetchScope & ItemSync::fetchScope | ( | ) |
Returns the item fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the ItemFetchScope documentation for an example.
- Returns
- a reference to the current item fetch scope
- See also
- setFetchScope() for replacing the current item fetch scope
Definition at line 189 of file itemsync.cpp.
void ItemSync::rollback | ( | ) |
Aborts the sync process and rolls back all not yet committed transactions.
Use this if an external error occurred during the sync process (such as the user canceling it).
- Since
- 4.5
Definition at line 461 of file itemsync.cpp.
void ItemSync::setFetchScope | ( | ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
The ItemFetchScope controls how much of an item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- fetchScope()
Definition at line 184 of file itemsync.cpp.
void ItemSync::setFullSyncItems | ( | const Item::List & | items | ) |
Sets the full item list for the collection.
Usually the result of a full item listing.
- Warning
- If the client using this is a resource, all items must have a valid remote identifier.
- Parameters
-
items A list of items.
Definition at line 142 of file itemsync.cpp.
void ItemSync::setIncrementalSyncItems | ( | const Item::List & | changedItems, |
const Item::List & | removedItems | ||
) |
Sets the item lists for incrementally syncing the collection.
Usually the result of an incremental remote item listing.
- Warning
- If the client using this is a resource, all items must have a valid remote identifier.
- Parameters
-
changedItems A list of items added or changed by the client. removedItems A list of items deleted by the client.
Definition at line 170 of file itemsync.cpp.
void ItemSync::setStreamingEnabled | ( | bool | enable | ) |
Enable item streaming.
Item streaming means that the items delivered by setXItems() calls are delivered in chunks and you manually indicate when all items have been delivered by calling deliveryDone().
- Parameters
-
enable true
to enable item streaming
Definition at line 434 of file itemsync.cpp.
void ItemSync::setTotalItems | ( | int | amount | ) |
Set the amount of items which you are going to return in total by using the setFullSyncItems() method.
- Parameters
-
amount The amount of items in total.
Definition at line 156 of file itemsync.cpp.
void ItemSync::setTransactionMode | ( | ItemSync::TransactionMode | mode | ) |
Set the transaction mode to use for this sync.
- Note
- You must call this method before starting the sync, changes afterwards lead to undefined results.
- Parameters
-
mode the transaction mode to use
- Since
- 4.6
Definition at line 470 of file itemsync.cpp.
|
protectedvirtual |
Reimplement this method to customize the synchronization algorithm.
- Parameters
-
storedItem the item as it is now newItem the item as it should be You can update the newItem
according to thestoredItem
before it gets committed.
Definition at line 205 of file itemsync.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:29 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.